Java最大公约数算法:深入剖析复杂度和时间效率
发布时间: 2024-08-27 22:31:34 阅读量: 29 订阅数: 29 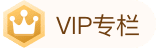
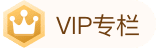
# 1. Java最大公约数算法概述
最大公约数(Greatest Common Divisor,GCD)是两个或多个整数中最大的公约数。在Java中,计算最大公约数有两种常用的算法:辗转相除法和扩展欧几里得算法。
辗转相除法是一种简单有效的算法,它通过不断求余数来计算最大公约数。其基本原理是:两个整数的最大公约数等于其中较小整数和它们余数的最大公约数。该算法的复杂度为O(log min(a, b)),其中a和b是两个输入整数。
扩展欧几里得算法是一种更通用的算法,它不仅可以计算最大公约数,还可以求解线性同余方程。其基本原理是:两个整数的最大公约数可以表示为它们两个系数的线性组合。该算法的复杂度为O(log max(a, b)),其中a和b是两个输入整数。
# 2. 最大公约数算法的理论基础
### 2.1 辗转相除法
#### 2.1.1 算法原理
辗转相除法是一种求最大公约数的经典算法,其原理如下:
给定两个正整数 `a` 和 `b`,如果 `b` 为 0,则 `a` 即为 `a` 和 `b` 的最大公约数;否则,计算 `a` 和 `b` 的余数 `r`,即 `a % b`,然后将 `b` 赋值为 `r`,重复此过程,直到 `b` 为 0。此时,`a` 的值即为 `a` 和 `b` 的最大公约数。
#### 2.1.2 复杂度分析
辗转相除法的平均时间复杂度为 `O(log min(a, b))`,其中 `min(a, b)` 表示 `a` 和 `b` 中较小的一个。这是因为在每次迭代中,`a` 和 `b` 的值都会减小,直到 `b` 为 0。
### 2.2 扩展欧几里得算法
#### 2.2.1 算法原理
扩展欧几里得算法是一种求解最大公约数和贝祖等式的算法。贝祖等式是一个线性方程,其形式为 `ax + by = gcd(a, b)`,其中 `a` 和 `b` 是给定的正整数,`x` 和 `y` 是整数解。
扩展欧几里得算法的原理如下:
给定两个正整数 `a` 和 `b`,如果 `b` 为 0,则 `a` 即为 `a` 和 `b` 的最大公约数,且 `x = 1`,`y = 0` 满足贝祖等式。否则,计算 `a` 和 `b` 的余数 `r`,即 `a % b`,然后递归调用扩展欧几里得算法求解 `b` 和 `r` 的最大公约数 `g` 和贝祖等式的解 `x'` 和 `y'`. 根据递归的结果,可以计算出 `a` 和 `b` 的最大公约数 `g` 和贝祖等式的解 `x` 和 `y`:
```
g = gcd(a, b)
x = x'
y = y' - (a / b) * x'
```
#### 2.2.2 复杂度分析
扩展欧几里得算法的时间复杂度为 `O(log min(a, b))`,与辗转相除法相同。这是因为扩展欧几里得算法也是通过递归调用来求解最大公约数的,每次递归调用都会减少 `a` 和 `b` 的值,直到 `b` 为 0。
# 3. Java实现最大公约数算法
### 3.1 辗转相除法实现
#### 3.1.1 代码示例
```java
public static int gcdEuclidean(int a, int b) {
if (b == 0) {
return a;
}
return gcdEuclidean(b, a % b);
}
```
#### 3.1.2 性能分析
辗转相除法的时间复杂度为 O(log min(a, b)),其中 min(a, b) 表示 a 和 b 中较小的一个。这是因为在每次递归中,较小的数字都会减半,直到它变成 0。
### 3.2 扩展欧几里得算法实现
#### 3.2.1 代码示例
```java
public static int[] gcdExtended(int a, int b) {
if (b == 0) {
return new int[]{1, 0, a};
}
int[] result = gcdExtended(b, a % b);
int x = result[1];
int y = result[0] - (a / b) * result[1];
return new int[]{y, x, result[2]};
}
```
#### 3.2.2 性能分析
扩展欧几里得算法的时间复杂度也为 O(log min(a, b)),与辗转相除法相同。
### 3.3 性能比较
下表比较了辗转相除法和扩展欧几里得算法的性能:
| 算法 | 时间复杂度 |
|---|---|
| 辗转相除法 | O(log min(a, b)) |
| 扩展欧几里得算法 | O(log min(a, b)) |
从表中可以看出,这两种算法的性能在理论上是相同的。然而,在实践中,扩展欧几里得算法可能比辗转相除法稍慢,因为它的计算量更大。
### 3.4 应用场景
辗转相除法和扩展欧几里得算法在许多应用中都有用,包括:
* **密码学:**最大公约数算法用于计算 RSA 和 ECC 等加密算法中的密钥。
* **数据结构:**最大公约数算法用于计算哈希表和并查集等数据结构中的哈希值。
* **数学:**最大公约数算法用于解决许多数学问题,如求解线性方程组和计算分数的简化形式。
# 4. 最大公约数算法的应用
### 4.1 密码学中的应用
最大公约数算法在密码学中有着广泛的应用,特别是在公钥密码体制中。
**4.1.1 RSA算法**
RSA算法是一种非对称加密算法,其安全性基于求解大整数的因数分解问题。RSA算法使用两个大素数p和q,生成公钥(e, n)和私钥(d, n),其中n=pq。加密时,明文M使用公钥(e, n)加密为密文C,解密时,密文C使用私钥(d, n)解密为明文M。
最大公约数算法在RSA算法中用于计算私钥d。已知公钥(e, n)和p、q,可以使用扩展欧几里得算法计算d,满足de ≡ 1 (mod (p-1)(q-1))。
**4.1.2 ECC算法**
ECC算法是一种基于椭圆曲线的公钥密码算法。ECC算法的安全性基于求解椭圆曲线上的离散对数问题。ECC算法使用一个有限域上的椭圆曲线,以及一个基点G。公钥为点Q=kG,其中k是私钥。
最大公约数算法在ECC算法中用于计算基点G的阶。已知椭圆曲线和一个点P,可以使用辗转相除法计算P的阶,即P的最小正整数倍数n,使得nP=O(无穷远点)。
### 4.2 数据结构中的应用
最大公约数算法在数据结构中也有着重要的应用。
**4.2.1 哈希表**
哈希表是一种数据结构,用于快速查找和插入数据。哈希表使用哈希函数将键映射到哈希值,然后将数据存储在哈希值对应的桶中。
最大公约数算法可以用于计算哈希函数。例如,可以使用辗转相除法计算字符串的哈希值,将字符串转换为数字,然后计算数字的余数。
**4.2.2 并查集**
并查集是一种数据结构,用于维护一组元素的连通性信息。并查集使用两个数组parent和rank,parent数组记录每个元素的父节点,rank数组记录每个元素所在树的深度。
最大公约数算法可以用于优化并查集的find操作。find操作用于查找一个元素的根节点。使用辗转相除法可以将find操作的时间复杂度从O(log n)优化到O(α(n)),其中α(n)是阿克曼函数的反函数。
```java
// 使用辗转相除法优化并查集的find操作
public int find(int x) {
if (parent[x] == x) {
return x;
}
return parent[x] = find(parent[x]);
}
```
### 代码示例
**4.2.3 辗转相除法计算哈希值**
```java
// 使用辗转相除法计算字符串的哈希值
public int hash(String s) {
int h = 0;
for (char c : s.toCharArray()) {
h = (h * 31 + c) % MOD;
}
return h;
}
```
**4.2.4 并查集优化find操作**
```java
// 使用辗转相除法优化并查集的find操作
public int find(int x) {
if (parent[x] == x) {
return x;
}
return parent[x] = find(parent[x]);
}
```
**4.2.5 辗转相除法计算椭圆曲线点阶**
```java
// 使用辗转相除法计算椭圆曲线点阶
public int order(Point P) {
int n = 0;
Point Q = P;
while (Q != O) {
n++;
Q = Q.add(P);
}
return n;
}
```
# 5.1 算法优化技巧
### 5.1.1 二进制辗转相除法
二进制辗转相除法是一种优化辗转相除法的算法,它利用了二进制数的特性来减少运算次数。具体步骤如下:
```java
public static int gcdBinary(int a, int b) {
if (b == 0) {
return a;
}
int shift = 0;
// 将b变为奇数
while ((b & 1) == 0) {
b >>= 1;
shift++;
}
// 若a是偶数
while ((a & 1) == 0) {
a >>= 1;
}
// 辗转相除
while (a != b) {
if ((a & 1) == 0 && (b & 1) == 0) {
a >>= 1;
b >>= 1;
} else if ((a & 1) == 0) {
a >>= 1;
} else if ((b & 1) == 0) {
b >>= 1;
} else {
if (a > b) {
a -= b;
} else {
b -= a;
}
}
}
return a << shift;
}
```
### 5.1.2 快速模幂
快速模幂是一种优化模幂运算的算法,它利用了二进制数的特性来减少运算次数。具体步骤如下:
```java
public static long fastPow(long a, long b, long mod) {
long res = 1;
while (b > 0) {
if ((b & 1) == 1) {
res = (res * a) % mod;
}
a = (a * a) % mod;
b >>= 1;
}
return res;
}
```
0
0
相关推荐
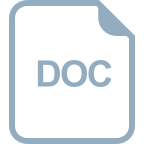
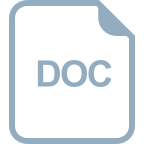
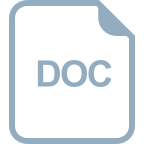
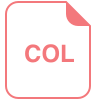
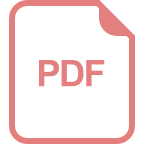
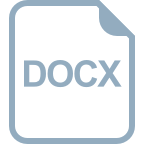
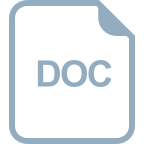
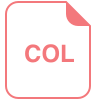