MATLAB Real-Time Data Update from Excel: The Secret of Dynamic Linking
发布时间: 2024-09-15 15:25:44 阅读量: 34 订阅数: 31 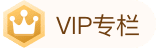
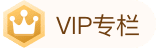
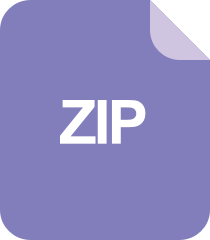
react-native-deep-linking:用于处理深层链接的简单路由匹配库
# 1. The Basics of MATLAB Interacting with Excel
The interaction between MATLAB and Excel is a common task in data analysis and processing. MATLAB provides a series of functions and tools that make it easy to read, write, and manipulate Excel data. This chapter will introduce the basics of MATLAB interacting with Excel, including data import and export, table ranges and cell references, as well as real-time data update mechanisms.
- **Data Import and Export:** MATLAB can easily import and export Excel data through the `readtable` and `writetable` functions. These functions support various file formats, including `.xlsx`, `.xls`, and `.csv`.
- **Table Ranges and Cell References:** In MATLAB, table ranges and cell references can be used to specify specific areas in an Excel worksheet. Table ranges are denoted using `A1` notation, for example, `A1:C5` represents the cell area from A1 to C5. Cell references use the `row, column` format, for example, `5, 3` represents the cell in the 5th row and 3rd column.
# 2. Real-time Reading of Excel Data
**2.1 The Principle and Method of Reading Excel Data**
**2.1.1 Data Import and Export**
Data interaction between MATLAB and Excel can be achieved through import and export operations. Importing refers to loading Excel data into the MATLAB workspace, while exporting is about writing MATLAB data into an Excel file.
```
% Import Excel data
data = xlsread('data.xlsx');
% Export MATLAB data
xlswrite('output.xlsx', data);
```
**2.1.2 Table Ranges and Cell References**
In MATLAB, table ranges and cell references can be used to specify the data to be read or written. A table range consists of a starting cell and an ending cell, while cell references specify a single cell.
```
% Read data from a specific table range
data = xlsread('data.xlsx', 'Sheet1', 'A1:C10');
% Read data from a specific cell
value = xlsread('data.xlsx', 'Sheet1', 'A1');
```
**2.2 Real-time Data Update Mechanism**
**2.2.1 Event Listening and Callback Functions**
MATLAB provides an event listening mechanism that allows the program to execute callback functions when a specific event occurs. When the data in the Excel file changes, MATLAB can listen for this event and trigger a callback function, thus achieving real-time data updates.
```
% Create an event listener
listener = addlistener(excel.Workbook, 'WorksheetModified', @updateCallback);
% Callback function
function updateCallback(~, event)
% Get the updated data
data = xlsread('data.xlsx');
% Update the data in the MATLAB workspace
assignin('base', 'data', data);
end
```
**2.2.2 Timers and Data Refresh**
Another method to achieve real-time data updates is by using a timer. Timers can periodically execute specific tasks, such as refreshing Excel data.
```
% Create a timer
timer = timer('Period', 1, 'ExecutionMode', 'fixedRate');
% Timer callback function
function timerCallback(~, ~)
% Get the updated data
data = xlsread('data.xlsx');
% Update the data in the MATLAB workspace
assignin('base', 'data', data);
end
% Start the timer
start(timer);
```
# 3. Dynamic Linking of MATLAB and Excel Data
### 3.1 Data Binding and Two-way Updates
Dynamic linking between MATLAB an
0
0
相关推荐







