MATLAB Reading Excel Data Cloud Computing: Distributed Processing and Scalability
发布时间: 2024-09-15 15:38:03 阅读量: 17 订阅数: 21 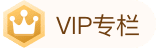
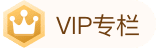
# 1. Reading Excel Data with MATLAB
## 1.1 Data Import and Preprocessing
MATLAB offers a variety of functions for importing Excel data, including `xlsread` and `readtable`. `xlsread` is used for numerical data, while `readtable` is used for tables that contain text, numbers, dates, and other data types. After importing the data, the `whos` command can be used to view data types and their sizes.
**Code Example:**
```matlab
% Import Excel file
data = xlsread('data.xlsx');
```
# 2.1 Concepts and Architecture of Distributed Computing
### 2.1.1 Parallel Computing vs. Distributed Computing
**Parallel Computing** refers to the use of multiple processing units to execute different parts of a program simultaneously to increase computation speed. There are two main types of parallel computing:
- **Shared Memory Parallel Computing:** Multiple processing units share the same memory and can access the same data.
- **Distributed Memory Parallel Computing:** Each processing unit has its own memory and can only access its own data.
**Distributed Computing** involves breaking down a computational task into smaller subtasks and executing these subtasks in parallel on different computers. The difference between distributed computing and parallel computing is that distributed computing involves different computers, while parallel computing involves multiple processing units on the same computer.
### 2.1.2 Cloud Computing Platforms and Service Models
Cloud computing platforms provide on-demand access to computational resources, including processing power, storage, and networking. The cloud service models are divided into three main types:
- **Infrastructure as a Service (IaaS):** Provides basic computing resources, such as servers, storage, and networking.
- **Platform as a Service (PaaS):** Provides a platform for developing and deploying applications on top of IaaS.
- **Software as a Service (SaaS):** Provides pre-built applications, allowing users to avoid managing the underlying infrastructure.
**Table 2.1: Cloud Computing Platforms and Service Models**
| Service Model | Description |
|---|---|
| IaaS | Provides basic computing resources |
| PaaS | Provides a platform for application development and deployment |
| SaaS | Provides pre-built applications |
**Code Block 2.1:**
```python
import cloudpickle
import multiprocessing as mp
def worker(args):
# Process subtasks
result = ...
return result
def main():
# Create a task queue
tasks = [task1, task2, task3]
# Create a process pool
pool = mp.Pool(processes=4)
# Map tasks to the process pool
results = pool.map(worker, tasks)
# Close the process pool
pool.close()
pool.join()
# Process results
...
```
**Logical Analysis:**
This code demonstrates the use of Python's `multiprocessing` module for distributed computing. The `worker` function processes each subtask, while the `main` function maps tasks to the process pool and collects results. The `Pool` class provides an interface for managing processes, and the `map` method maps functions to the process pool and returns an iterator with the results.
**Parameter Description:**
- `processes`: The number of processes in the process pool.
# 3. Practical Application of Cloud Computing
### 3.1 MATLAB Cloud Deployment
#### 3.1.1 Cloud Platform Selection and Configuration
**Cloud Platform Selection**
When choosing a cloud platform, consider the following factors:
- **Service Types:** The types of services provided by the platform, such as computing, storage, databases, etc.
- **Pricing Model:** The platform's pricing method, such as pay-as-you-go or reserved instances.
- **Availability Zones:** The availability zones in which the platform provides services, to ensure data and application accessibility.
- **Technical Support:** The level of technical support provided by the platform to resolve deployment and operational issues.
**Cloud Platform Configuration**
When configuring a cloud platform, follow these steps:
1. **Create an Account:** Register and create an account on the cloud platform.
2. **Select Region:** Choose the region where you want to deploy your application.
3. **Create a Resource Group:** Create a resource group to organize and manage cloud resources.
4. **Create a Virtual Machine:** Create a virtual machine to host your MATLAB application.
5. **Configure Networking:** Set up the virtual machine's network configurations, including IP addresses, subnets, and security groups.
#### 3.1.2 MATLAB Code Migration and Deployment
**Code Migration**
When migrating MATLAB code to the cloud, consider the following:
- **Code Compatibility:** Ensure that the MATLAB code is compati
0
0
相关推荐
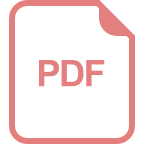
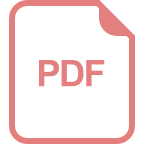
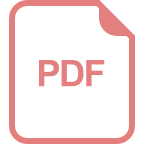
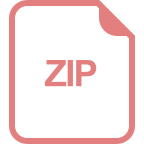
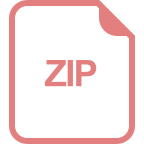
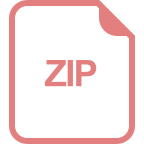
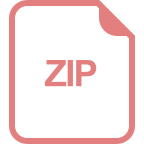
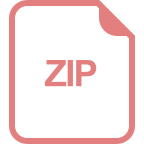
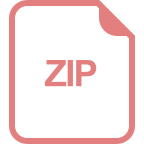