揭秘PHP数据库缓存机制:深入理解缓存原理与实现
发布时间: 2024-08-02 05:01:37 阅读量: 24 订阅数: 26 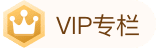
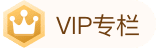
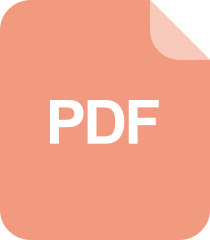
Java实现LRU缓存机制:深入解析与高效编码实践
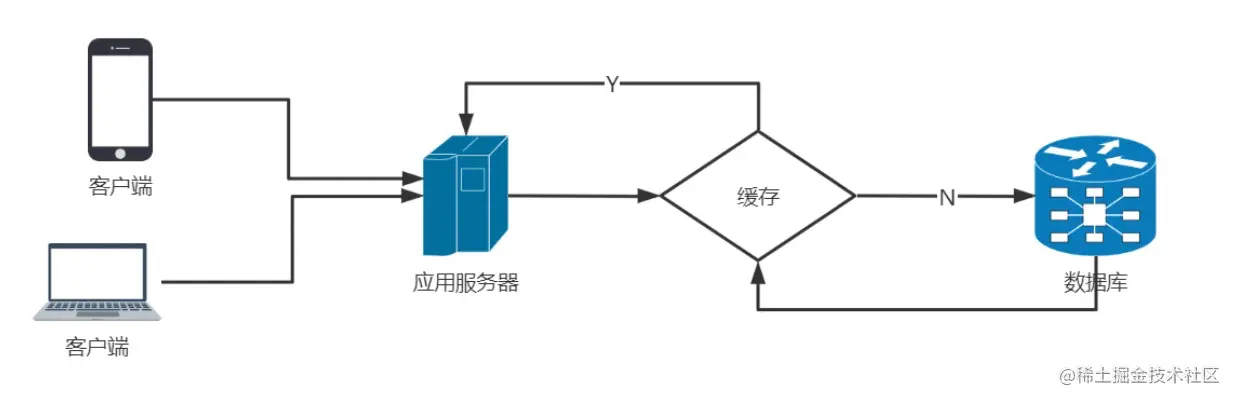
# 1. PHP数据库缓存概述
PHP数据库缓存是一种技术,用于将频繁访问的数据库查询结果存储在内存中,以减少对数据库的访问次数,从而提高应用程序的性能。它通过将数据副本保存在缓存中,当需要时直接从缓存中读取数据,而不是从数据库中查询,从而避免了对数据库的访问开销。
缓存可以有效地提高应用程序的响应时间,特别是对于那些需要频繁访问数据库的应用程序。它还可以减少数据库的负载,提高数据库的可用性和稳定性。此外,缓存还可以降低数据库的成本,因为可以减少对数据库的访问次数,从而降低数据库的硬件和维护成本。
# 2. PHP数据库缓存原理
### 2.1 缓存机制的分类和特点
缓存机制根据其存储位置和访问方式的不同,可以分为以下几类:
| 缓存类型 | 存储位置 | 访问方式 | 特点 |
|---|---|---|---|
| 内存缓存 | 服务器内存 | 直接访问 | 访问速度快,容量受限 |
| 文件缓存 | 硬盘 | 文件读写 | 访问速度慢,容量大 |
| 数据库缓存 | 数据库 | 数据库查询 | 访问速度介于内存和文件缓存之间,容量受数据库限制 |
| 分布式缓存 | 多台服务器 | 网络通信 | 容量大,高可用性 |
每种缓存机制都有其自身的特点和适用场景。内存缓存访问速度最快,但容量受限,适合存储频繁访问的小型数据。文件缓存容量大,但访问速度较慢,适合存储不经常访问的大型数据。数据库缓存访问速度介于内存和文件缓存之间,容量受数据库限制,适合存储与数据库查询相关的缓存数据。分布式缓存容量大,高可用性,适合存储大规模的共享数据。
### 2.2 缓存的实现方式和存储结构
缓存的实现方式主要有以下几种:
| 实现方式 | 存储结构 | 特点 |
|---|---|---|
| 哈希表 | 键值对 | 快速查找,空间占用大 |
| 链表 | 节点列表 | 顺序访问,插入删除效率高 |
| LRU缓存 | 双向链表 + 哈希表 | 近期最少使用原则,空间占用小 |
| LFU缓存 | 计数器 + 哈希表 | 访问频率最高原则,命中率高 |
哈希表是一种基于键值对的存储结构,查找效率高,但空间占用较大。链表是一种基于节点列表的存储结构,顺序访问效率高,但插入删除效率较低。LRU缓存(最近最少使用)是一种基于双向链表和哈希表的存储结构,遵循最近最少使用原则,空间占用小,命中率高。LFU缓存(访问频率最高)是一种基于计数器和哈希表的存储结构,遵循访问频率最高原则,命中率更高。
选择合适的缓存实现方式和存储结构,需要根据实际的应用场景和性能要求进行权衡。
# 3. PHP数据库缓存实现
### 3.1 PHP原生缓存函数的使用
#### 3.1.1 memcache函数的使用
memcache是PHP原生支持的一种分布式内存缓存系统,它提供了高效的键值存储功能,可以有效地提高数据库查询速度。memcache函数的使用非常简单,下面是一个示例代码:
```php
<?php
// 连接到memcache服务器
$memcache = new Memcache;
$memcache->connect('localhost', 11211);
// 设置缓存值
$memcache->set('key', 'value', 0, 3600);
// 获取缓存值
$value = $memcache->get('key');
// 关闭连接
$memcache->close();
?>
```
**逻辑分析:**
* `connect()`方法用于连接到memcache服务器,参数分别为服务器地址和端口。
* `set()`方法用于设置缓存值,参数分别为键、值、过期时间(单位为秒)、压缩标志。
* `get()`方法用于获取缓存值,参数为键。
* `close()`方法用于关闭连接。
**参数说明:**
* `$memcache`:memcache对象。
* `'key'`:缓存键。
* `'value'`:缓存值。
* `0`:过期时间为0表示永不过期。
* `3600`:过期时间为3600秒(1小时)。
* `$value`:获取的缓存值。
#### 3.1.2 redis函数的使用
redis也是一种分布式内存缓存系统,它提供了丰富的键值存储类型和灵活的数据结构,可以满足各种缓存需求。redis函数的使用也比较简单,下面是一个示例代码:
```php
<?php
// 连接到redis服务器
$redis = new Redis;
$redis->connect('localhost', 6379);
// 设置缓存值
$redis->set('key', 'value');
// 获取缓存值
$value = $redis->get('key');
// 关闭连接
$redis->close();
?>
```
**逻辑分析:**
* `connect()`方法用于连接到redis服务器,参数分别为服务器地址和端口。
* `set()`方法用于设置缓存值,参数分别为键和值。
* `get()`方法用于获取缓存值,参数为键。
* `close()`方法用于关闭连接。
**参数说明:**
* `$redis`:redis对象。
* `'key'`:缓存键。
* `'value'`:缓存值。
* `$value`:获取的缓存值。
### 3.2 第三方缓存库的应用
除了PHP原生缓存函数,还有一些第三方缓存库可以提供更丰富的功能和更好的性能。下面介绍两个常用的第三方缓存库。
#### 3.2.1 APC缓存库的使用
APC(Alternative PHP Cache)是一个PHP扩展,它提供了高效的内存缓存和字节码缓存功能。APC缓存库的使用非常简单,下面是一个示例代码:
```php
<?php
// 设置缓存值
apc_store('key', 'value');
// 获取缓存值
$value = apc_fetch('key');
// 删除缓存值
apc_delete('key');
?>
```
**逻辑分析:**
* `apc_store()`方法用于设置缓存值,参数分别为键和值。
* `apc_fetch()`方法用于获取缓存值,参数为键。
* `apc_delete()`方法用于删除缓存值,参数为键。
**参数说明:**
* `'key'`:缓存键。
* `'value'`:缓存值。
* `$value`:获取的缓存值。
#### 3.2.2 Xcache缓存库的使用
Xcache也是一个PHP扩展,它提供了类似于APC的缓存功能,但性能更高。Xcache缓存库的使用也比较简单,下面是一个示例代码:
```php
<?php
// 设置缓存值
xcache_set('key', 'value');
// 获取缓存值
$value = xcache_get('key');
// 删除缓存值
xcache_unset('key');
?>
```
**逻辑分析:**
* `xcache_set()`方法用于设置缓存值,参数分别为键和值。
* `xcache_get()`方法用于获取缓存值,参数为键。
* `xcache_unset()`方法用于删除缓存值,参数为键。
**参数说明:**
* `'key'`:缓存键。
* `'value'`:缓存值。
* `$value`:获取的缓存值。
# 4. PHP数据库缓存优化
### 4.1 缓存策略的制定和选择
#### 4.1.1 缓存更新策略
缓存更新策略决定了当缓存中的数据发生变化时,如何更新缓存中的数据。常见的缓存更新策略包括:
- **写回策略:**当数据发生变化时,直接更新数据库,同时将更新后的数据写入缓存。
- **写穿策略:**当数据发生变化时,直接更新数据库,但不更新缓存。下次从缓存中读取数据时,发现数据已过期,则重新从数据库中读取数据并更新缓存。
- **延迟双写策略:**当数据发生变化时,先将更新后的数据写入缓存,然后异步地更新数据库。
#### 4.1.2 缓存失效策略
缓存失效策略决定了当缓存中的数据过期或不再有效时,如何处理缓存中的数据。常见的缓存失效策略包括:
- **固定时间失效:**设置一个固定时间,当缓存中的数据超过这个时间后,自动失效。
- **访问时间失效:**当数据被访问时,更新其失效时间。当失效时间超过当前时间后,数据失效。
- **最近最少使用(LRU):**将最近最少使用的缓存数据淘汰。
- **最近最少访问(LFU):**将最近最少访问的缓存数据淘汰。
### 4.2 缓存性能的监控和调优
#### 4.2.1 缓存命中率的监控
缓存命中率是衡量缓存性能的重要指标。缓存命中率是指从缓存中读取数据成功的次数与从数据库中读取数据成功的次数之比。缓存命中率越高,说明缓存的性能越好。
#### 4.2.2 缓存开销的优化
缓存开销是指使用缓存带来的额外开销,包括内存占用、CPU消耗和网络开销。可以通过以下方式优化缓存开销:
- **选择合适的缓存大小:**缓存大小过大会占用过多内存,过小会导致命中率降低。
- **使用高效的缓存算法:**使用高效的缓存算法,如LRU或LFU,可以减少缓存开销。
- **优化缓存的数据结构:**使用合适的缓存数据结构,如哈希表或跳跃表,可以提高缓存的性能。
### 代码示例
**缓存更新策略示例:**
```php
// 写回策略
$cache->set('key', 'value');
$db->update('table', ['key' => 'value']);
// 写穿策略
$db->update('table', ['key' => 'value']);
```
**缓存失效策略示例:**
```php
// 固定时间失效
$cache->set('key', 'value', 3600); // 失效时间为1小时
// 访问时间失效
$cache->set('key', 'value');
$cache->touch('key', 3600); // 更新失效时间为1小时
```
**缓存性能监控示例:**
```php
// 缓存命中率监控
$cache->getStats();
// 缓存开销监控
$cache->getMemoryUsage();
$cache->getCPUTime();
```
### 流程图
**缓存更新策略流程图:**
```mermaid
graph LR
subgraph 写回策略
A[数据发生变化] --> B[更新数据库] --> C[更新缓存]
end
subgraph 写穿策略
A[数据发生变化] --> B[更新数据库]
end
subgraph 延迟双写策略
A[数据发生变化] --> B[更新缓存] --> C[异步更新数据库]
end
```
### 表格
**缓存策略对比表:**
| 策略 | 优点 | 缺点 |
|---|---|---|
| 写回策略 | 数据一致性高 | 性能开销大 |
| 写穿策略 | 性能高 | 数据一致性低 |
| 延迟双写策略 | 兼顾数据一致性和性能 | 实现复杂 |
# 5. PHP数据库缓存案例实践
### 5.1 电商网站的商品缓存
#### 5.1.1 商品信息的缓存
对于电商网站而言,商品信息是至关重要的数据,频繁的查询会给数据库带来巨大的压力。因此,对商品信息进行缓存可以有效地提高查询效率。
**实现步骤:**
1. **确定缓存键:**商品信息的缓存键可以根据商品ID或商品SKU进行设计。
2. **获取缓存数据:**在查询商品信息时,首先从缓存中获取数据。如果缓存中存在,则直接返回缓存数据。
3. **更新缓存:**如果缓存中不存在,则从数据库中查询商品信息并将其写入缓存。
**代码示例:**
```php
// 获取商品信息
$productId = 123;
$productCacheKey = 'product_' . $productId;
$productInfo = $cache->get($productCacheKey);
// 如果缓存中不存在,则从数据库中查询
if (!$productInfo) {
$productInfo = $db->query("SELECT * FROM products WHERE id = $productId");
$cache->set($productCacheKey, $productInfo, 3600); // 缓存1小时
}
```
#### 5.1.2 商品详情页的缓存
商品详情页通常包含商品图片、描述、规格等信息,这些信息相对静态,且访问频率较高。因此,对商品详情页进行缓存可以显著提高页面加载速度。
**实现步骤:**
1. **确定缓存键:**商品详情页的缓存键可以根据商品ID或商品URL进行设计。
2. **获取缓存数据:**在加载商品详情页时,首先从缓存中获取数据。如果缓存中存在,则直接返回缓存数据。
3. **更新缓存:**如果缓存中不存在,则从数据库中查询商品详情信息并将其写入缓存。
**代码示例:**
```php
// 获取商品详情页
$productId = 123;
$productDetailCacheKey = 'product_detail_' . $productId;
$productDetail = $cache->get($productDetailCacheKey);
// 如果缓存中不存在,则从数据库中查询
if (!$productDetail) {
$productDetail = $db->query("SELECT * FROM product_details WHERE product_id = $productId");
$cache->set($productDetailCacheKey, $productDetail, 3600); // 缓存1小时
}
```
0
0
相关推荐
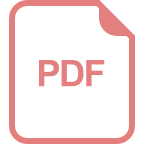
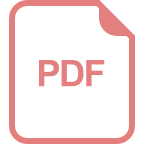
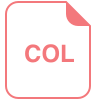
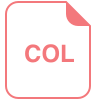
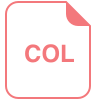
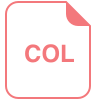
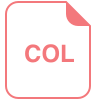
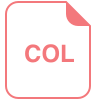