Android数据结构进阶教程:从单向到双向链表
发布时间: 2024-09-10 02:39:37 阅读量: 81 订阅数: 79 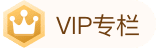
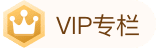
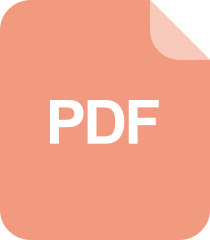
数据结构笔记:单向链表
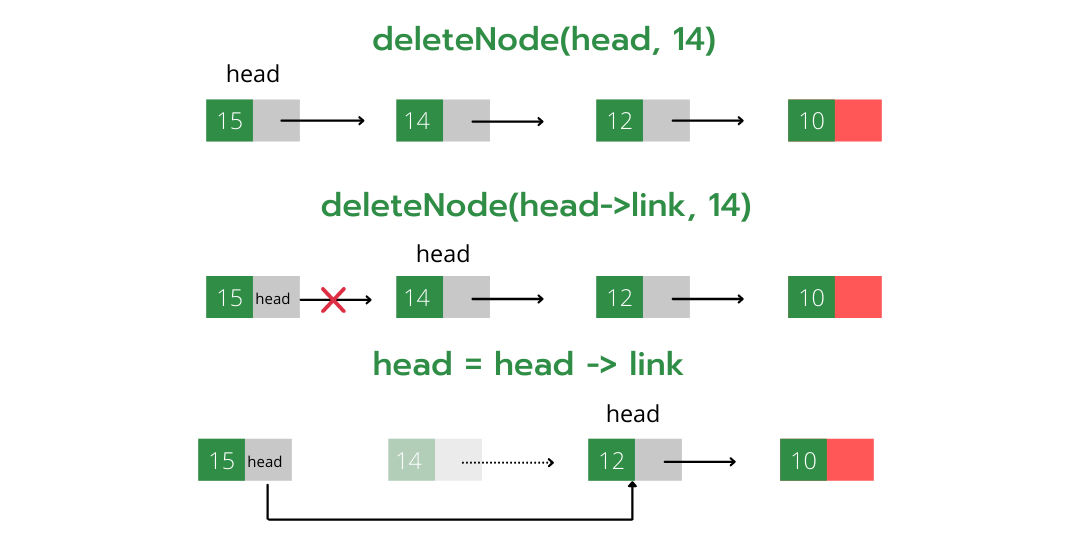
# 1. 链表基础与数据结构概述
数据结构是组织和存储数据的一种方式,使得访问和修改更加高效。在众多数据结构中,链表因其独特的动态内存分配和高效的元素插入与删除操作,成为IT行业特别是移动应用开发中的一个关键概念。本文将详细介绍链表的类型、特点和应用场景,重点放在单向链表和双向链表上,我们将从基础概念开始,逐步深入到操作细节,并结合Android平台的应用案例,为读者提供一个实用的学习指南。
## 链表的类型与特点
链表按照节点的连接方式可分为单向链表和双向链表。单向链表的节点仅向一个方向连接,适用于简单的数据存储和管理。双向链表的节点双向连接,允许从任一节点出发,既可向前也可向后遍历,提供了更灵活的数据操作。
```mermaid
graph LR
A[单向链表] -->|一个指针| B[节点]
C[双向链表] -->|两个指针| D[节点]
D -->|前驱指针| E[前一节点]
D -->|后继指针| F[后一节点]
```
## 链表与数组的对比
链表和数组都是常见的线性数据结构,但它们在内存使用和操作效率上有着显著区别。数组是静态的,需要预先分配固定大小的内存空间,适合随机访问;而链表是动态的,每个节点通过指针链接,可以灵活地增加或删除节点,但随机访问效率较低。
## 链表在Android开发中的重要性
在Android应用开发中,链表结构被广泛用于实现各种数据集合,如队列、栈等。掌握链表的使用对于优化内存管理、提升应用性能和响应速度都至关重要。在下一章中,我们将深入探讨单向链表的内部实现和操作技巧,以及如何在Android开发中高效利用链表结构。
# 2. 单向链表深入解析
## 单向链表的内部实现
### 节点结构与指针操作
单向链表是一种常见的数据结构,它由一系列节点组成,每个节点包含数据部分和指向下一个节点的指针。在Java中,我们通常使用类来定义一个链表节点,如下所示:
```java
class ListNode {
int data;
ListNode next;
ListNode(int data) {
this.data = data;
this.next = null;
}
}
```
节点的`data`字段存储数据,`next`字段则存储指向下一个节点的指针。链表的头节点(head)是链表的第一个节点,它指向链表中的下一个节点,最后一个节点的`next`指针则指向`null`,标识链表的结束。
指针操作是单向链表的核心,包括:
- **创建节点:** 创建一个节点实例,并初始化数据。
- **插入节点:** 在链表的特定位置插入一个新的节点。
- **删除节点:** 删除链表中的某个节点。
- **访问节点:** 通过遍历链表来访问特定位置的节点。
### 单向链表的创建和销毁
创建一个单向链表通常从创建一个头节点开始,然后逐个创建其余节点,并依次链接它们。以下是一个简单的单向链表的创建示例:
```java
public class SingleLinkedList {
private ListNode head;
public void add(int data) {
ListNode newNode = new ListNode(data);
if (head == null) {
head = newNode;
} else {
ListNode current = head;
while (current.next != null) {
current = current.next;
}
current.next = newNode;
}
}
public void printList() {
ListNode current = head;
while (current != null) {
System.out.print(current.data + " -> ");
current = current.next;
}
System.out.println("null");
}
}
```
在这个例子中,`add`方法用于向链表末尾添加一个新的节点。`printList`方法用于打印整个链表,从头节点开始,沿着`next`指针遍历到链表末尾。
销毁一个单向链表意味着需要遍历整个链表,并且逐个删除其中的节点。这通常在Java中通过垃圾回收机制自动完成,但在C或C++等语言中需要程序员手动实现。例如,在C中销毁链表的代码如下:
```c
void freeLinkedList(ListNode *head) {
ListNode *temp;
while (head != NULL) {
temp = head;
head = head->next;
free(temp);
}
}
```
这里使用`free`函数来释放每个节点所占用的内存,直到链表为空。
## 单向链表的操作技巧
### 常用操作:插入、删除、查找
#### 插入
在单向链表中,插入操作根据插入位置的不同,分为头部插入、尾部插入和中间插入。以下是一个简单的中间插入方法示例:
```java
public void insert(int data, int position) {
ListNode newNode = new ListNode(data);
if (position == 0) {
newNode.next = head;
head = newNode;
return;
}
ListNode current = head;
int index = 0;
while (current != null && index < position - 1) {
current = current.next;
index++;
}
if (current == null) {
throw new IndexOutOfBoundsException("Position " + position + " is out of bounds");
}
newNode.next = current.next;
current.next = newNode;
}
```
#### 删除
删除节点需要小心处理,以确保不会丢失对链表其余部分的链接:
```java
public void delete(int position) {
if (head == null) {
throw new IllegalStateException("List is empty");
}
if (position == 0) {
head = head.next;
return;
}
ListNode current = head;
int index = 0;
while (current.next != null && index
```
0
0
相关推荐
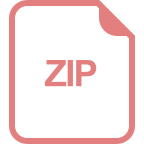
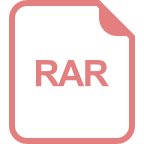
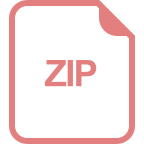
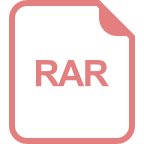
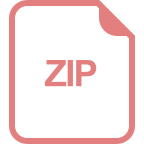
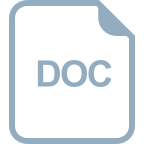
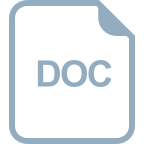
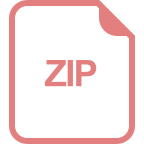