Android散列表应用深度解析:探索哈希表的奥秘
发布时间: 2024-09-10 03:07:03 阅读量: 143 订阅数: 79 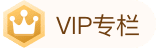
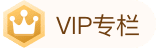
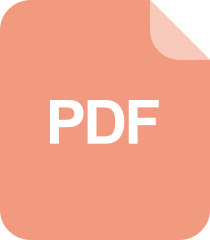
代码随想录:哈希表的应用与优化
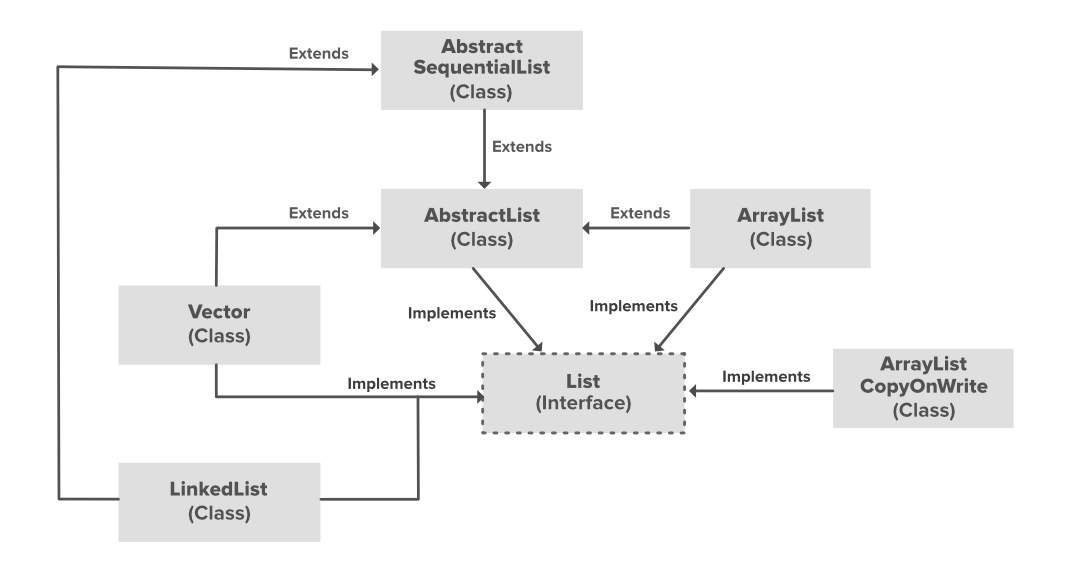
# 1. 散列表与哈希表的基础概念
在现代计算机科学中,散列表(Hash Table)是一种从关键码(Key)到值(Value)的映射表,通过哈希函数(Hash Function)将关键码映射到表中一个位置来访问记录,以加快信息的检索速度。这种数据结构提供了非常高的存取效率,常用于实现关联数组和数据库索引。
## 1.1 哈希表的特点
散列表的主要特点包括高效率的平均查找速度、动态数据结构以及实现简单。哈希表在插入和查询操作上通常能达到常数时间复杂度O(1)。但值得注意的是,在最坏的情况下,哈希表的时间复杂度可以退化到O(n),比如在哈希函数设计不当导致大量冲突时。
## 1.2 哈希表的基本组成
哈希表主要由哈希函数、存储空间以及处理冲突的机制组成。哈希函数负责将关键码转换为数组的索引,存储空间通常是一个数组或链表的集合,而处理冲突的机制用于解决多个关键码映射到同一个索引的情况。
```java
// 示例:Java中的HashMap类,用于演示哈希表的基本使用
import java.util.HashMap;
public class HashTableExample {
public static void main(String[] args) {
HashMap<String, Integer> map = new HashMap<>();
map.put("key1", 1);
map.put("key2", 2);
Integer value = map.get("key1");
System.out.println(value); // 输出: 1
}
}
```
哈希表的实现和应用广泛,下一章将详细探讨其内部工作机制。
# 2. 哈希表的内部工作机制
## 2.1 哈希函数的设计原理
哈希函数是哈希表的核心组成部分,负责将输入的键转换成数组索引。一个良好的哈希函数应当能够尽可能均匀地将键映射到数组的不同位置,以减少哈希冲突的发生。
### 2.1.1 哈希函数的选择标准
选择或设计哈希函数时,我们需要考虑以下标准:
1. **计算效率**:哈希函数的计算需要尽可能快速,以提高整体数据结构的操作效率。
2. **空间效率**:哈希函数应该尽量紧凑,避免不必要的内存开销。
3. **均匀分布**:理想情况下,哈希函数应能够将键均匀地分布在哈希表的存储空间内,以减少冲突。
### 2.1.2 常见哈希算法解析
常见的哈希算法包括:
- **除法取余法**:使用一个质数对键的值进行取余操作,生成哈希值。
- **乘法取余法**:通过乘以一个常数并取余的方式来生成哈希值。
- **数字分析法**:利用键值中的特定位或数字来进行哈希运算。
这里,我们以乘法取余法为例,分析其设计原理:
```csharp
// 伪代码:乘法取余法哈希函数示例
int hash(int key, int size) {
// size为哈希表的大小
return (key * a) % size;
}
```
在这个例子中,`a` 是一个常数,它和 `key` 相乘后,取模操作得到的结果即为哈希值。通过调整 `a` 的值,我们可以改变哈希值的分布情况。
## 2.2 哈希冲突的解决策略
在哈希表中,哈希冲突是无法完全避免的,因此需要有一系列策略来解决冲突。
### 2.2.1 开放定址法
开放定址法是一种处理冲突的策略,它在发现冲突时,按照一定的规则找到下一个空槽位。最简单的开放定址法是线性探测法,按照固定步长进行探测。
### 2.2.2 链表法
链表法是另一种常见的解决冲突的方法,它在每个数组位置上保存一个链表,用于存放所有具有相同哈希值的元素。这种方法的缺点是额外的内存开销。
### 2.2.3 双重散列法
双重散列法使用两个哈希函数,当发生冲突时,使用第二个哈希函数计算新的索引位置。双重散列能够减少聚集的问题,提高查找效率。
## 2.3 哈希表的性能分析
哈希表的性能主要受时间复杂度和空间复杂度的影响,而负载因子对哈希表的性能有着直接的影响。
### 2.3.1 时间复杂度和空间复杂度
理想情况下,哈希表的查找、插入和删除操作的时间复杂度为 O(1)。但在最坏情况下,如果哈希函数设计不当或负载因子过高,时间复杂度可能退化到 O(n)。
### 2.3.2 负载因子的影响与调整
负载因子是哈希表已填入元素的数量与哈希表大小的比值,它是衡量哈希表负载情况的一个重要指标。随着负载因子的增加,冲突的概率也随之增加,因此适时调整哈希表的大小,可以维持高效的性能。
## 表格和流程图
以下是哈希表性能分析的表格:
| 操作 | 最好情况 | 平均情况 | 最坏情况 |
| ------------ | -------- | -------- | -------- |
| 查找 | O(1) | O(1) | O(n) |
| 插入 | O(1) | O(1) | O(n) |
| 删除 | O(1) | O(1) | O(n) |
下面是一个展示哈希冲突解决策略选择的 mermaid 流程图:
```mermaid
graph TD
A[开始哈希冲突] --> B{冲突解决策略}
B -->|开放定址法| C[线性探测法]
B -->|链表法| D[在链表中添加元素]
B -->|双重散列法| E[使用第二个哈希函数计算索引]
```
通过这些分析,我们可以看出哈希表的设计与实现不仅要关注其核心算法,还需要考虑实际应用中的性能优化以及应对哈希冲突的策略。这样,我们才能构建出既快速又高效的哈希表数据结构。
# 3. Android中的散列表实现
## 3.1 Android内置的散列表类
### 3.1.1 HashMap的使用和特性
`HashMap`是Android开发中常用的一种散列表实现,其关键特性是存储键值对,且基于散列实现快速的元素查找。`HashMap`不保证映射的顺序,特别适合于需要以常数时间进行插入、删除和查找操作的场景。Android中的`HashMap`是通过哈希表来实现的,这使得它在平均情况下具有常数时间复杂度的性能表现。
为了更深入理解`HashMap`的使用和特性,让我们看以下例子:
```java
import java.util.HashMap;
public class HashMapExample {
public static void main(String[] args) {
HashMap<String, Integer> hashMap = new HashMap<>();
// 添加元素
hashMap.put("One", 1);
hashMap.put("Two", 2);
hashMap.put("Three", 3);
// 查找元素
Integer value = hashMap.get("Two");
// 遍历HashMap
for (Map.Entry<String, Integer> entry : hashMap.entrySet()) {
System.out.println(entry.getKey() + " : " + entry.getValue());
}
}
}
```
上述代码段演示了`HashMap`的基本使用方法。其中`put`方法用于添加键值对,`get`方法用于根据键获取值,而通过`entrySet()`方法可以遍历所有的键值对。
`HashMap`类在内部通过一个数组来存储数据,并通过哈希函数对键进行散列,以达到快速定位元素的目的。在使用`HashMap`时,我们需要注意其容量(capacity)和负载因子(load factor)的概念。初始容量决定了数组的大小,而负载因子决定了何时扩容。
### 3.1.2 HashSet的使用和特性
`HashSet`是基于`HashMap`实现的,它不允许键值对中有重复的键,实际上只使用了键,并将值设置为一个虚拟对象。由于`HashSet`是基于`HashMap`实现的,它的特性与`HashMap`十分相似,操作的时间复杂度也为平均常数时间。`HashSet`特别适合用于需要快速查找元素是否存在的场景。
以下是一个`HashSet`使用的例子:
```java
import java.util.HashSet;
import java.util.Set;
public class HashSetExample {
public static void main(String[] args) {
Set<String> hashSet = new HashSet<>();
// 添加元素
hashSet.add("Apple");
hashSet.add("Banana");
hashSet.add("Cherry");
// 检查元素是否存在
boolean contains = hashSet.contains("Banana");
// 遍历HashSet
for (String item : hashSet) {
System.out.println(item);
}
}
}
```
在`HashSet`的实现中,我们可以注意到,添加元素时`add`方法会先检查待添加的元素是否已经存在。由于底层使用`HashMap`实现,所以`HashSet`的性能取决于`HashMap`的性能。
## 3.2 自定义哈希函数与键值对
### 3.2.1 设计适用于Android的哈希函数
为了优化性能和减少哈希冲突,我们可以设计并实现自定义的哈希函数。好的哈希函数应该能够尽可能地减少冲突,并且散列均匀分布。自定义哈希函数在Android中可以用于提升特定应用的性能,例如当键是自定义对象或者有特定需求的字符串时。
举个例子,我们可以为一个简单的自定义对象实现一个哈希函数:
```java
class CustomObject {
private int key;
public CustomObject(int key) {
this.key = key;
}
@Override
public int hashCode() {
retu
```
0
0
相关推荐
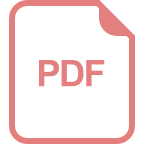
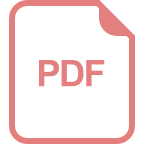
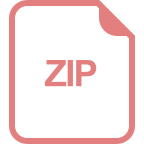
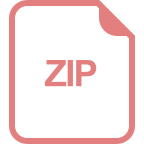
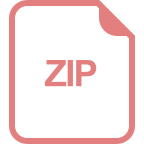
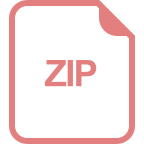
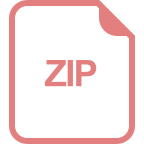
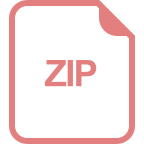