Python中有向图的最大流算法详解
发布时间: 2024-03-28 15:33:58 阅读量: 13 订阅数: 21 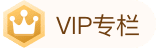
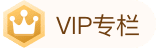
# 1. 图论基础概述
- 1.1 图的基本概念
- 1.2 有向图的定义与性质
- 1.3 最大流算法介绍
# 2. 最大流问题概述
- 2.1 最大流问题的定义
- 2.2 最大流问题的实际应用
- 2.3 最大流问题求解方法
# 3. Ford-Fulkerson算法原理
- **3.1 Ford-Fulkerson算法基本思想**
- Ford-Fulkerson算法的核心是不断通过增广路径来找到最大流量的流网络。其基本思想是:从源节点到汇点通过增广路径进行流量增加,直到无法找到增广路径为止。
- **3.2 增广路径的寻找**
- 增广路径是指从源节点到汇点的一条路径,并且每条路径上的流量还没有达到边的容量上限。常用的方法有DFS(深度优先搜索)和BFS(广度优先搜索)。
- **3.3 Ford-Fulkerson算法的具体实现**
- Ford-Fulkerson算法的具体实现步骤:
1. 初始化残余网络:将每条边的流量设置为0;
2. 不断寻找增广路径:通过增广路径找到可增加的最大流量;
3. 更新残余网络:更新残余网络中的边的流量;
4. 重复步骤2和步骤3,直到无法找到增广路径。
```python
def ford_fulkerson(graph, source, sink):
# Step 1: Initialize residual graph with capacities and flows
residual_graph = initialize_residual_graph(graph)
# Step 2: Find augmenting paths in the residual graph
while True:
path = bfs(residual_graph, source, sink)
if not path:
break
# Find the bottleneck capacity of the path
bottleneck = get_bottleneck_capacity(path, residual_graph)
# Update residual capacities along the augmenting path
update_residual_capacities(path, bottleneck, residual_graph)
# Step 3: Calculate the maximum flow
max_flow = calculate_max_flow(residual_graph, source)
return max_flow
# Code Summary:
# 1. Initialize residual graph with capacities and flows.
# 2. Find augmenting paths using BFS in the residual graph.
# 3. Update residual capacities
```
0
0
相关推荐
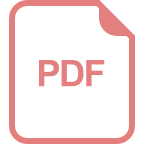
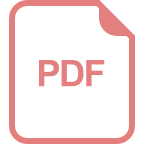
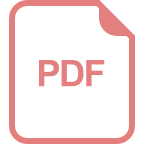
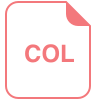
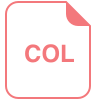
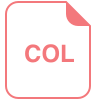
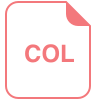

