【Java并发中的原子操作】:CAS和原子类的使用,专家教你3大技巧
发布时间: 2024-08-29 14:42:11 阅读量: 30 订阅数: 28 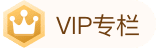
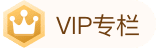
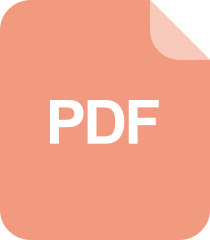
Java中的原子操作:深入探索AtomicInteger的实现与应用
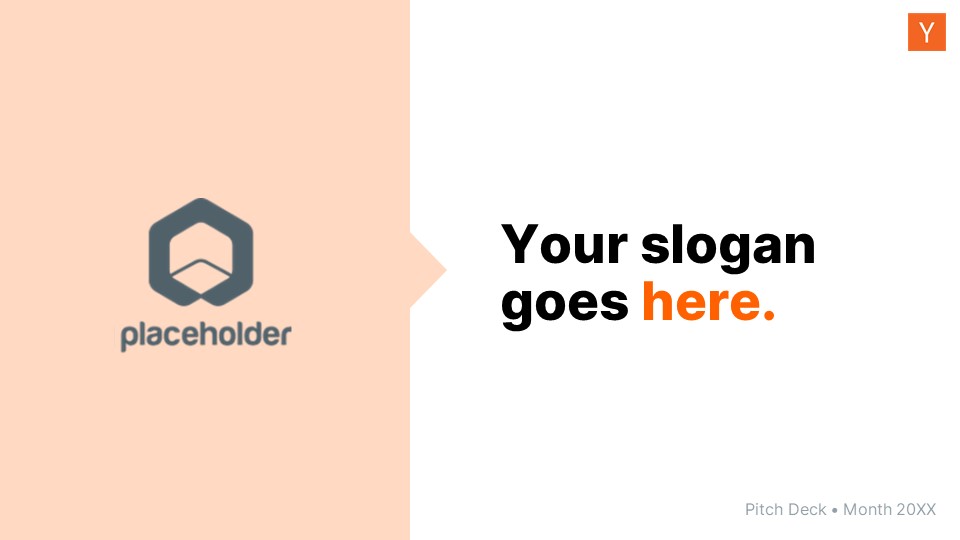
# 1. Java并发基础与原子操作概述
Java并发编程是构建现代高性能应用的核心之一,而在并发编程中,原子操作发挥着至关重要的作用。本章我们将概览Java并发的基础知识以及原子操作的基本概念,为深入理解后续内容打下坚实的基础。
## 1.1 Java并发编程的重要性
Java并发编程允许我们设计能够利用多核处理器性能的应用程序,提高程序的执行效率。它涉及到线程、进程、锁、同步机制等多个方面,是现代软件开发中不可或缺的一部分。
## 1.2 并发与并行的区别
在深入研究并发之前,我们需要区分并发与并行的概念。**并发**指的是多个任务在同一时间段内开始运行,但不一定是同时运行;而**并行**则是指多个任务在同一时刻同时运行。在多核处理器上,多个线程可以真正做到并行执行,这大大提高了程序的性能。
## 1.3 原子操作的必要性
在并发环境中,数据的一致性和完整性至关重要,原子操作是保证并发编程安全的基础。原子操作是指在多线程环境下,被序列化执行的操作,其结果要么完全执行,要么完全不执行,不会存在中间状态。Java通过一系列的并发工具类来实现原子操作,如`AtomicInteger`、`AtomicLong`等。
在接下来的章节中,我们将详细探讨CAS机制以及如何在Java中应用原子类来提升并发编程的效率和安全性。
# 2. 理解CAS机制
### 2.1 CAS的基本概念
#### 2.1.1 CAS的定义和原理
CAS(Compare-And-Swap)是一种无锁的同步机制,用于实现多线程环境下的原子操作。在执行CAS操作时,会先比较内存中的值与预期的值是否一致,如果一致则将内存中的值更新为新值。这个过程是原子的,即在操作过程中不会被其他线程中断。CAS操作成功则返回true,表示更新成功;否则返回false,表示更新失败。
CAS的原理可以理解为一种乐观锁的实现方式,它假设多个线程在操作共享变量时不会发生冲突,只有在真正更新变量时,才进行冲突检测。如果检测到冲突,即有其他线程更改了该变量,当前线程则放弃更新并可以重新尝试或者采取其他策略。
#### 2.1.2 CAS在Java中的实现
在Java中,CAS是由`sun.misc.Unsafe`类中的`compareAndSwap`方法实现的,该类提供了硬件级别的原子操作能力。而在Java 8之后,推荐使用`java.util.concurrent.atomic`包中的原子类来使用CAS操作,这些类包括`AtomicInteger`、`AtomicLong`等。通过这些类的方法,如`getAndIncrement`,开发者可以轻松地实现CAS操作而无需直接操作`Unsafe`类。
```java
import java.util.concurrent.atomic.AtomicInteger;
public class CasExample {
private AtomicInteger atomicInteger = new AtomicInteger(0);
public void increment() {
int current, newValue;
do {
current = atomicInteger.get();
newValue = current + 1;
} while (!***pareAndSet(current, newValue));
}
}
```
在上述代码中,`increment`方法会尝试将`AtomicInteger`的值原子性地增加1。它首先获取当前值,然后计算新值,接着使用`compareAndSet`方法来更新。如果在尝试更新时发现值已经被其他线程改变,则循环重试直到成功。
### 2.2 CAS的优缺点分析
#### 2.2.1 CAS的优势
- **无阻塞**:由于CAS是一种无锁操作,它避免了传统锁可能导致的阻塞问题,提高了系统的并发性能。
- **上下文切换减少**:因为没有锁竞争,所以减少了线程上下文切换的开销。
- **更好的性能**:尤其是在高竞争环境中,CAS通常比使用传统锁具有更好的性能表现。
#### 2.2.2 CAS的潜在风险
- **ABA问题**:在CAS操作中,如果变量V从A变到B,又变回A,CAS操作会误判没有改变,导致数据更新失败。
- **循环时间长**:在高争用条件下,CAS可能导致长时间循环,导致性能下降。
- **只能保证单个变量的原子操作**:对于涉及多个变量的复合操作,需要其他机制来保证原子性。
### 2.3 CAS在并发编程中的应用案例
#### 2.3.1 使用CAS实现无锁计数器
无锁计数器是并发编程中的一个典型应用。与传统的使用`synchronized`关键字或`ReentrantLock`实现的计数器不同,无锁计数器能够避免锁带来的性能开销,提高计数操作的效率。
```java
import java.util.concurrent.atomic.AtomicInteger;
public class LockFreeCounter {
private AtomicInteger count = new AtomicInteger(0);
public void increment() {
count.incrementAndGet();
}
public int getCount() {
return count.get();
}
}
```
在这个例子中,`increment`方法使用`incrementAndGet`来增加计数,这是一个原子操作。由于`AtomicInteger`内部利用CAS实现,因此该计数器在多线程环境下能够保持正确的计数。
#### 2.3.2 CAS在并发集合中的运用
Java的并发集合类,如`ConcurrentHashMap`,`ConcurrentLinkedQueue`等,内部大量使用了CAS操作。以`ConcurrentHashMap`为例,其使用分段锁技术,并通过CAS来实现无锁的键值对更新。
```java
import java.util.concurrent.ConcurrentHashMap;
public class ConcurrentHashMapExample {
private ConcurrentHashMap<String, String> map = new ConcurrentHashMap<>();
public void put(String key, String value) {
map.put(key, value);
}
public String get(String key) {
return map.get(key);
}
}
```
`ConcurrentHashMap`通过维护一个分段锁,将数据分割到不同的段,每个段独立维护自己的锁,然后使用CAS来保证在没有锁竞争的情况下,数据的更新操作。这使得`ConcurrentHashMap`在高并发下表现优异。
在本章节中,我们深入探讨了CAS机制的基本概念、优缺点以及其在并发编程中的应用案例。通过这些内容,读者应当对CAS机制有了较为全面的理解,为后面章节探讨原子类和并发性能优化打下基础。
# 3. 深入探索Java中的原子类
## 3.1 原子类的分类和功能
### 3.1.1 原子变量类简介
在Java中,原子类是一组为解决多线程环境下共享变量的原子操作而设计的类。这些类位于 `java.util.concurrent.atomi
0
0
相关推荐
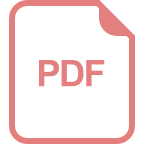
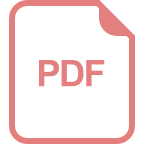
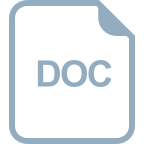
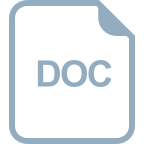
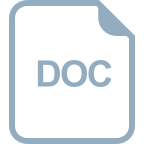
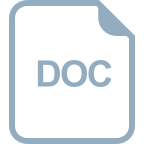
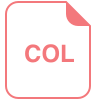
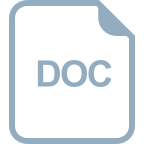