【Java线程通信机制】:揭秘wait、notify和join的5大使用秘诀
发布时间: 2024-08-29 14:45:59 阅读量: 23 订阅数: 39 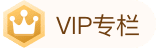
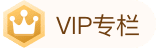
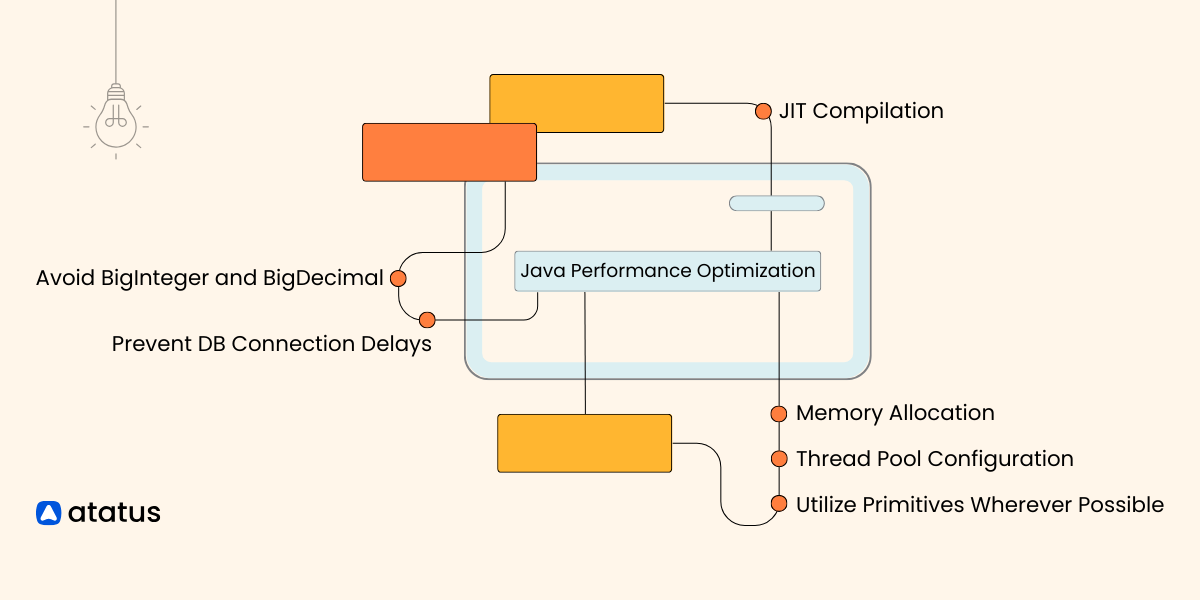
# 1. Java线程通信机制概述
在现代计算机系统中,多线程编程是提高软件执行效率的关键技术之一。Java作为一种广泛使用的编程语言,提供了丰富的线程通信机制来协调线程间的执行顺序和资源共享,确保数据的一致性和线程的安全性。Java中的线程通信机制主要包括wait、notify、notifyAll以及join等方法,它们通过Java虚拟机(JVM)内核层面的支持,实现线程间的协作。
## 1.1 Java线程通信机制的重要性
线程通信机制解决了多线程环境下的同步问题。当多个线程需要访问共享资源时,正确的线程通信可以避免资源竞争和数据冲突,从而避免产生数据不一致的问题。线程通信主要通过监视器锁(Monitor Lock)实现,而wait、notify和notifyAll则是实现线程间通信的关键方法。
## 1.2 Java线程通信的基本元素
- **Wait方法**:使当前线程释放锁并进入等待状态,直到其他线程调用相同对象的notify或notifyAll方法,或者经过指定的时间后自动醒来。
- **Notify方法**:用于选择性地唤醒等待同一监视器的线程,这些线程正在等待该监视器的notify方法。
- **Join方法**:使当前线程等待其他线程完成操作后才能继续执行,类似于线程之间的协调执行顺序。
在接下来的章节中,我们将深入探讨这些线程通信机制的内部工作原理,应用场景,以及高级应用和最佳实践。
# 2. 深入理解wait机制
Java线程的wait机制是多线程编程中实现线程间通信和协调的一种重要方式。它允许一个线程在某些条件下主动放弃处理器的执行,并让出对共享资源的控制权,从而促使其他线程得以继续执行。本章将深入探讨wait机制的工作原理、应用以及高级用法。
## 2.1 wait方法的基本概念
### 2.1.1 wait方法的工作原理
wait方法是Object类中提供的一个关键方法,任何对象都可以调用此方法来使当前线程进入等待状态。当一个线程调用了某个对象的wait方法,它将会释放对该对象锁的控制权,并进入对象的等待集合中。这样做的目的是为了等待其他线程执行到该对象的notify()或notifyAll()方法时,重新获得执行的机会。
wait方法的执行可以分为以下几个步骤:
1. 调用wait方法的线程必须拥有该对象的锁。
2. 线程执行wait方法后,会释放该对象的锁,并进入等待集合。
3. 等待集合中的线程将会一直等待,直到被其他线程使用notify或notifyAll方法唤醒。
4. 一旦被唤醒,线程需要重新竞争到锁后才能继续执行。
### 2.1.2 wait方法在同步代码块中的应用
在同步代码块中使用wait方法时,通常与其他同步机制一起使用以确保线程间的正确通信。一个常见的场景是使用synchronized关键字来同步代码块,以保证共享资源的安全访问。
```java
synchronized (lock) {
while (condition) {
lock.wait();
}
// 线程安全地处理业务逻辑
}
```
在上述代码中,`lock.wait();` 语句使得当前线程在进入等待状态之前首先释放了`lock`对象的锁。在其他线程中,一旦完成某些操作,可能会调用`lock.notify()`或`lock.notifyAll()`来通知等待的线程,从而唤醒它们继续执行后续的业务逻辑。
## 2.2 wait方法的高级应用
### 2.2.1 使用wait实现生产者消费者模型
生产者消费者问题是计算机科学中的一个经典问题,它描述了两个或多个线程之间的协调工作关系。在Java中,可以利用wait和notify机制来实现这一模型。
生产者消费者模型的实现可以分为以下几个步骤:
1. 创建一个共享队列(Buffer),用于存放产品。
2. 生产者线程生产产品并放入队列中,若队列已满,则线程进入等待状态。
3. 消费者线程从队列中取出产品消费,若队列为空,则线程进入等待状态。
4. 生产者或消费者线程在操作完成后,使用notify方法唤醒其他等待的线程。
```java
class Buffer {
private final Queue<Product> queue = new LinkedList<>();
private final int capacity;
public Buffer(int capacity) {
this.capacity = capacity;
}
public synchronized void produce(Product product) throws InterruptedException {
while (queue.size() == capacity) {
wait();
}
queue.add(product);
notifyAll();
}
public synchronized Product consume() throws InterruptedException {
while (queue.isEmpty()) {
wait();
}
return queue.poll();
}
}
```
### 2.2.2 处理wait中的中断异常
当一个线程处于等待状态时,它可能会被其他线程中断。在这种情况下,wait方法会抛出一个InterruptedException异常。在实际应用中,我们需要合理处理这种中断信号。
处理InterruptedException通常有两种策略:
1. 在捕获异常后直接退出线程。
2. 将中断异常作为状态的一部分,并在适当的时机进行处理。
下面是一个处理InterruptedException的示例:
```java
synchronized void safeWait() {
while (condition) {
try {
lock.wait();
} catch (InterruptedException e) {
// 重新设置线程中断状态
Thread.currentThread().interrupt();
// 根据需要处理中断情况
return;
}
}
}
```
在这个例子中,通过调用`Thread.currentThread().interrupt();`恢复线程的中断状态,确保线程能够根据中断状态进行适当的逻辑处理。
## 2.3 wait与notify的配合使用
### 2.3.1 notify和notifyAll方法介绍
与wait方法配合使用的是notify和notifyAll方法。这两种方法都定义在Object类中,用于唤醒等待该对象锁的线程。
- notify(): 随机唤醒在此对象上等待的一个线程。
- notifyAll(): 唤醒在此对象上等待的所有线程。
这两种方法只能在同步方法或同步代码块中被调用,否则会抛出IllegalMonitorStateException异常。
### 2.3.2 理解notify与wait的同步关系
为了确保线程间的正确通信,通常会将wait与notify放在一对循环中使用。wait通常放在循环的判断条件中,而notify则放在更新条件后调用。
```java
synchronized void signal() {
while (!condition) {
wait();
}
// 更新共享资源状态
condition = false;
notify();
}
```
在上述示例中,signal方法中的while循环确保了只有在条件成立时,才会继续执行。若条件不成立,则线程会持续等待。一旦条件得到满足,将唤醒等待的线程。
在多线程环境中,正确理解wait和notify的同步关系至关重要。一方面,这有助于构建高效、可靠的并发应用程序;另一方面,它也是深入理解并发编程的关键所在。
至此,我们已经探讨了wait机制的方方面面,为理解Java线程通信机制打下了坚实的基础。在下一章中,我们将继续深入了解notify机制,并探索更多Java多线程编程的核心概念。
# 3. 细说notify机制
## 3.1 notify方法的原理与规则
### 3.1.1 notify方法的基本用途
在多线程协作的场景中,线程之间需要相互通信,协调各自的动作。在Java中,`notify`方法就是用于唤醒在当前对象上等待的一个线程。当一个线程调用对象的`notify`方法时,JVM会随机选择一个在此对象锁上等待的线程进行通知,被通知的线程将获得对象锁,并从`wait`调用点继续执行。
`notify`方法与`wait`方法配合使用时,可以实现线程之间的精确控制,这对于生产者和消费者模式等协作场景至关重要。生产者线程通过`notify`通知消费者线程可以消费数据了,而消费者线程通过`notify`通知生产者可以再次生产数据了。
### 3.1.2 notify方法的等待队列机制
当一个线程调用`notify`方法时,它并不会立即释放锁。JVM会将这个线程从锁的等待队列中移除,并选择另一个等待线程(如果有的话)将其加入到锁的入口队列中。这个过程通常发生在`wait`方法返回前,确保锁被正确释放并让被唤醒的线程有机会重新获取锁。
需要注意的是,`notify`只能唤醒一个等待线程。如果多个线程都在等待,那么选择哪个线程是不确定的。这就导致了`notify`可能存在效率问题,因为选择的线程可能不是最佳的执行线程,可能需要等待更长的时间。为了避免这种情况,通常使用`notifyAll`方法,它将唤醒所有等待线程,但是这又可能导致线程竞争,从而需要更复杂的逻辑来确保线程执行的顺序性。
## 3.2 notify的实践案例分析
### 3.2.1 使用notify解决多线程协作问题
假设我们有一个简单的生产者消费者场景,生产者线程生成数据,消费者线程消费数据。我们使用`notify`来确保当队列为空时消费者线程等待,当队列非空时生产者线程通知消费者线程开始消费。
```java
public class ProducerConsumerExample {
private final Queue<Integer> queue = new LinkedList<>();
private final int MAX_SIZE = 10;
public synchronized void produce(int number) {
while (queue.size() == MAX_SIZE) {
try {
wait();
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
```
0
0
相关推荐
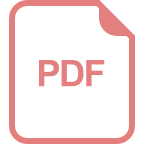
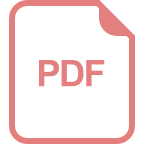
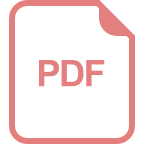





