【Java并发编程高级技巧】:避开10大并发陷阱,提升程序稳定性
发布时间: 2024-08-29 14:21:10 阅读量: 49 订阅数: 39 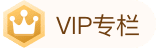
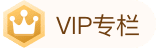
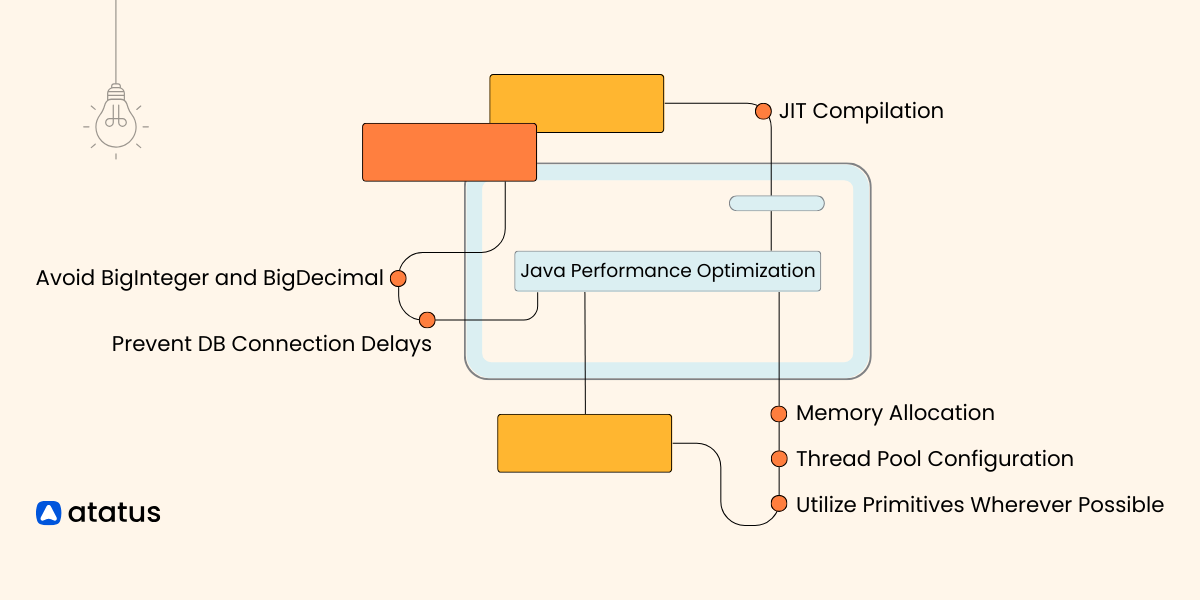
# 1. Java并发编程基础概念
## 1.1 并发编程的重要性
并发编程是现代软件开发的核心之一,特别是在构建需要高效利用多核处理器资源的高性能应用时尤为重要。通过并发,我们可以同时执行多个任务,提升程序的整体吞吐量。Java作为支持多线程的编程语言,提供了丰富的并发工具和API,帮助开发者设计出既高效又易于维护的并发应用程序。
## 1.2 Java中的线程与进程
在Java中,线程是执行任务的最小单位。进程可以包含多个线程,而线程则是独立于其他线程运行的代码片段。Java虚拟机(JVM)通过线程调度器来管理线程的生命周期。Java中的Thread类和Runnable接口是创建线程的两种常见方式。
```java
class MyThread extends Thread {
public void run() {
// 线程执行的任务代码
}
}
class MyRunnable implements Runnable {
public void run() {
// 线程执行的任务代码
}
}
public class Main {
public static void main(String[] args) {
MyThread t = new MyThread();
t.start(); // 启动线程
MyRunnable r = new MyRunnable();
new Thread(r).start(); // 创建线程并启动
}
}
```
## 1.3 同步与并发控制基础
并发控制是确保多个线程按预期顺序执行的关键。Java提供了多种同步机制,包括`synchronized`关键字和显式锁(如`ReentrantLock`)。这些机制可以防止多个线程同时访问共享资源时发生冲突和数据不一致的问题。理解同步机制是掌握并发编程的前提。
在后续章节中,我们将深入探讨并发编程中的高级概念,并通过实际案例分析来掌握它们的应用技巧。
# 2. 并发陷阱解析与解决方案
## 2.1 线程同步的常见问题
### 2.1.1 死锁的识别与预防
死锁是多线程编程中常见的一种陷阱,它发生在两个或多个线程在相互等待对方释放资源的情况下,导致这些线程永远无法继续执行。识别死锁通常需要借助于系统的日志输出、线程转储(thread dump)或者专门的监控工具。
为了预防死锁,我们可以采取以下策略:
- 使用固定的顺序来获取锁,并确保所有线程都按照这一顺序来请求锁。
- 限制锁的持有时间,确保线程在合理时间内释放锁。
- 对共享资源实现锁定策略,例如使用锁定超时机制。
### 2.1.2 活锁与饥饿现象分析
活锁是死锁的另一种形式,其中线程虽然保持活跃状态并尝试处理问题,但是由于一些原因(比如频繁的重试和撤销操作)无法向前推进。饥饿现象则是指由于线程优先级设置不当或者资源分配策略导致某些线程长时间得不到执行的机会。
解决活锁和饥饿的策略:
- 在处理消息时,引入随机延迟来打乱线程重试的顺序,避免系统进入活锁状态。
- 通过线程优先级调整或确保公平的锁分配策略,例如,使用公平锁来避免饥饿现象的发生。
## 2.2 并发集合类的陷阱
### 2.2.1 不恰当的集合使用导致的并发问题
在使用并发集合类时,如果不正确地使用或者不了解其内部工作原理,很容易造成线程安全问题。以 HashMap 为例,它并不是线程安全的。在多线程环境中直接使用 HashMap,可能会导致数据不一致的问题。
为避免这类问题,应当:
- 使用线程安全的集合,比如 Collections.synchronizedMap 包装的 HashMap。
- 使用 Java 提供的并发集合类,如 ConcurrentHashMap,它为多线程操作提供了高效的线程安全的映射结构。
### 2.2.2 如何选择正确的并发集合
选择正确的并发集合对于确保应用性能和线程安全至关重要。以下是选择并发集合时可以考虑的因素:
- 考虑集合操作的类型,例如是否需要频繁地进行更新操作。
- 了解各个并发集合类的内部实现,以便为具体的应用场景选择合适的集合。
- 关注集合的扩展性,尤其在高并发的场景下。
## 2.3 线程池使用的误区
### 2.3.1 线程池配置不当导致的性能问题
线程池的配置至关重要,不恰当的配置会导致线程资源得不到合理利用,从而影响程序性能。比如,核心线程数和最大线程数设置不合理,可能会造成CPU使用率不均衡或者队列积压严重。
避免这类问题的方法有:
- 根据CPU数量合理设置线程池的线程数。
- 使用适当的队列类型和大小,比如 ArrayBlockingQueue、LinkedBlockingQueue 或者 SynchronousQueue。
### 2.3.2 线程池的优雅关闭与资源管理
在多线程应用中,优雅地关闭线程池并管理相关资源是确保程序稳定运行的重要部分。当系统关闭时,需要处理好正在执行的任务和队列中等待的任务。
实现线程池的优雅关闭通常遵循以下步骤:
- 调用线程池的 shutdown() 方法,拒绝接受新任务,等待已提交的任务执行完毕。
- 调用 shutdownNow() 方法,尝试停止所有正在执行的任务,并返回队列中等待的任务。
- 对于长时间运行的任务,应当定期检查并更新任务的执行状态,确保能够及时响应系统关闭请求。
# 3. 并发工具类的高级应用
## 3.1 锁的高级特性与应用
### 3.1.1 ReentrantLock与synchronized的选择
在Java并发编程中,锁是保证线程安全的关键机制之一。`ReentrantLock` 和 `synchronized` 是Java提供的两种常见同步机制。它们在功能上有所重叠,但各自有独特的用法场景和高级特性。
`ReentrantLock` 是一个可重入的互斥锁。它提供了比 `synchronized` 更灵活的功能,如尝试获取锁的限时操作(`tryLock(long timeout, TimeUnit unit)`)、可中断的锁获取(`lockInterruptibly()`),以及公平锁机制。公平锁保证了等待时间最长的线程最先获得锁,而 `synchronized` 不提供这样的保证。
```java
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class ReentrantLockExample {
private final Lock lock = new ReentrantLock();
public void performTask() {
lock.lock();
try {
// 临界区:执行任务
} finally {
lock.unlock(); // 确保锁总是会被释放
}
}
}
```
从上述代码中可以看到,`ReentrantLock` 必须显式获取和释放锁。这增加了灵活性,但也增加了错误的风险。相反,`synchronized` 提供了一种内置的锁机制,代码编写更加简洁,但它不允许尝试获取锁或在等待中响应中断。
### 3.1.2 公平锁与非公平锁的性能对比
公平锁确保线程按照请求锁的顺序获得锁,而非公平锁允许“饥饿”发生,即等待时间长的线程可能会被后来的线程超越。非公平锁在大多数情况下都比公平锁有更高的性能,因为它们减少了上下文切换的开销。
```java
import java.util.concurrent.locks.ReentrantLock;
public class FairnessLockExample {
public static void main(String[] args) {
ReentrantLock fairLock = new ReentrantLock(true); // 公平锁
ReentrantLock unfairLock = new ReentrantLock(false); // 非公平锁
// 测试公平锁和非公平锁的性能
}
}
```
在高并发场景下,使用公平锁可能会影响性能,因为每个线程都必须按顺序获取锁,而这个顺序可能是由线程调度的开销所决定的。然而,在资源紧张的情况下,例如线程数量远远大于CPU核心数,公平锁可以保证更合理的资源分配。
## 3.2 原子变量的使用技巧
### 3.2.1 原子类的基本原理
原子变量(Atomic Variables)是java.util.concurrent.atomic包中的类,它们提供了一种能在没有锁的情况下进行线程安全操作的手段。原子变量的内部实现通常依赖于非阻塞算法和硬件级的原子操作。
以 `AtomicInteger` 为例,它基于 `Unsafe` 类的 `compareAndSwapInt` 方法,这是一个原子操作,可以保证在多线程环境中的可见性和原子性。
```java
import java.util.concurrent.atomic.AtomicInteger;
public class AtomicIntegerExample {
private AtomicInteger atomicInteger = new AtomicInteger();
public int getAndIncrement() {
return atomicInteger.getAndIncrement();
}
}
```
在这个例子中,`getAndIncrement` 方法将原子性地增加变量的值,并返回增加前的值。这是通过在一个循环中不断尝试 `compareAndSwapInt` 操作直到成功来实现的。
### 3.2.2 高级原子操作与场景应用
除了基本的原子操作,原子变量类还提供了更多高级操作,例如 `compareAndSet`, `getAndUpdate` 和 `updateAndGet` 等。这些方法不仅更新变量的值,而且还能在操作前获取当前值,使得复杂的原子操作成为可能。
```java
import java.util.concurrent.atomic.AtomicInteger;
public class AdvancedAtomicExample {
private AtomicInteger atomicInteger = new AtomicInteger();
public void multipleUpdates() {
// 假设一个更新操作依赖于先前值的情况
atomicInteger.updateAndGet(x -> x * 2); // 获取当前值,乘以2后更新
}
}
```
这种高级操作非常适合实现无锁的计数器、累加器、计时器等。它们可以有效地减少线程间的竞争,提高并发性能。
## 3.3 并发工具类的深度挖掘
### 3.3.1 CountDownLatch、CyclicBarrier与Semaphore的使用
Java并发工具类库提供了几种用于控制线程同步的高级工具,如 `CountDownLatch`、`CyclicBarrier` 和 `Semaphore`。这些工具类通过不同的方式,帮助开发者更好地管理线程间的协调。
`CountDownLatch` 允许多个线程等待直到一定数量的事件发生。它是一次性的,一旦计数达到零,就不能重新使用。
```java
import java.util.concurrent.CountDownLatch;
public class CountDownLatchExample {
private CountDownLatch latch = new CountDownLatch(3);
public void await() throws InterruptedException {
latch.await(); // 等待计数器达到零
}
public void countDown() {
latch.countDown(); // 减少计数
}
}
```
而 `CyclicBarrier` 允许一组线程相互等待,直到所有线程都到达某个点,然后可以继续执行。与 `CountDownLatch` 不同,`Cycl
0
0
相关推荐
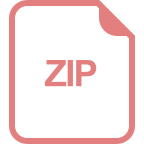
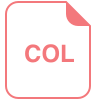
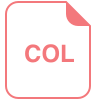
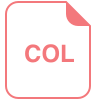
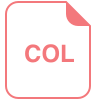


