Java算法与云计算:算法在云端,释放无限可能
发布时间: 2024-08-27 20:45:59 阅读量: 10 订阅数: 16 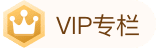
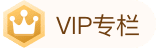
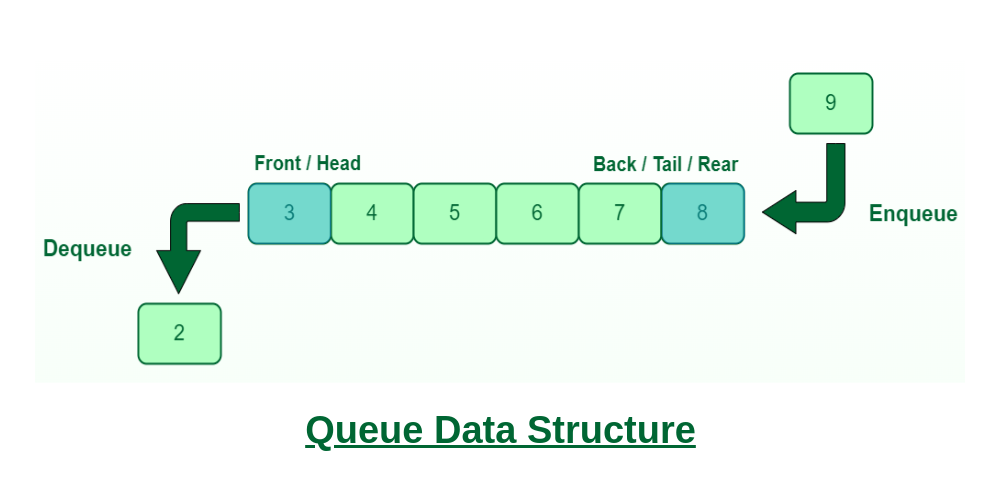
# 1. Java算法基础
**1.1 算法的概念和分类**
算法是指解决特定问题的步骤序列,它具有明确的输入、输出和有限的步骤。算法可以分为两大类:顺序算法和非顺序算法。顺序算法按照步骤顺序执行,而非顺序算法可以根据条件或输入数据动态改变执行顺序。
**1.2 Java中的算法实现**
Java提供了丰富的库和框架来支持算法实现。例如,`java.util.Arrays`类提供了排序和搜索算法,而`java.util.concurrent`包提供了并发算法。此外,Java还支持泛型编程,允许算法在不同数据类型上重用。
# 2. 云计算架构与算法应用
### 2.1 云计算基础概念
#### 2.1.1 云计算的定义和特点
云计算是一种按需付费的计算模型,它允许用户通过互联网访问共享的计算资源,如服务器、存储和网络。其主要特点包括:
- **按需自服务:** 用户可以随时随地通过自助服务门户访问云资源。
- **广泛的网络访问:** 云资源可以通过各种设备和网络连接访问。
- **资源池化:** 云资源被集中管理,并根据需求动态分配给用户。
- **快速弹性:** 云资源可以根据需求快速扩展或缩减。
- **可度量服务:** 云资源的使用情况可以按需计费,并提供详细的报告。
#### 2.1.2 云计算的服务模型和部署模型
云计算提供了多种服务模型和部署模型,以满足不同的业务需求:
**服务模型:**
- **基础设施即服务 (IaaS):** 提供底层计算、存储和网络资源。
- **平台即服务 (PaaS):** 在 IaaS 之上提供开发和部署平台。
- **软件即服务 (SaaS):** 提供预构建的应用程序,用户无需管理基础设施或平台。
**部署模型:**
- **公有云:** 云资源向公众开放。
- **私有云:** 云资源专用于单个组织。
- **混合云:** 结合公有云和私有云的特性。
### 2.2 云计算中的算法应用场景
云计算的分布式架构和强大的计算能力使其成为各种算法应用的理想平台。以下是一些常见的应用场景:
#### 2.2.1 大数据处理和分析
云计算提供了大规模数据处理和分析的解决方案。算法可以利用云端的分布式计算能力并行处理海量数据,从而提高分析效率。
#### 2.2.2 机器学习和人工智能
云计算为机器学习和人工智能算法提供了训练和部署的平台。算法可以在云端的大型数据集上训练,并利用分布式计算资源实现快速迭代和部署。
#### 2.2.3 图像和视频处理
云计算的并行处理能力和存储容量使其适用于图像和视频处理任务。算法可以在云端高效处理大量图像和视频,用于对象检测、图像增强和视频分析等应用。
**代码示例:**
```python
import numpy as np
import cv2
# 加载图像
image = cv2.imread('image.jpg')
# 使用 OpenCV 的并行处理模块进行图像灰度化
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY, async=True)
# 等待并行处理完成
gray_image.wait_for_async()
# 显示灰度化图像
cv2.imshow('Gray Image', gray_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**代码逻辑分析:**
此代码示例使用 OpenCV 库中的并行处理模块对图像进行灰度化处理。`async=True` 参数启用并行处理,允许算法在多个 CPU 核心上并行执行。`wait_for_async()` 方法等待并行处理完成,然后将结果存储在 `gray_image` 变量中。
**参数说明:**
- `image`: 输入的彩色图像。
- `cv2.COLOR_BGR2GRAY`: OpenCV 中的色彩空间转换常量,用于将 BGR 图像转换为灰度图像。
- `async`: 布尔值,指示是否启用并行处理。
# 3. Java算法在云端实践
### 3.1 云端算法开发平台
#### 3.1.1 Hadoop和Spark
**Hadoop**是一个分布式计算框架,用于处理大规模数据集。它提供了一组用于存储和处理数据的工具,包括:
- **HDFS(Hadoop分布式文件系统)**:一个分布式文件系统,用于存储大文件。
- **MapReduce**:一个编程模型,用于并行处理大数据集。
**Spark**是一个大数据处理引擎,建立在Hadoop之上。它提供了一个统一的API,用于处理结构化和非结构化数据。Spark比Hadoop更快,因为它使用内存计算,而不是将数据写入磁盘。
**代码块:**
```java
import org.apache.spark.SparkConf;
import org.apache.spark.SparkContext;
public class SparkExample {
public static void main(String[] args) {
// 创建SparkConf对象
SparkConf conf = new SparkConf().setAppName("SparkExample");
// 创建SparkContext对象
SparkContext sc = new SparkContext(conf);
// 创建RDD
RDD<String> rdd = sc.textFile("input.txt");
// 对RDD进行转换和操作
RDD<String> result = rdd.filter(line -> line.contains("error"));
// 将结果保存到文件中
result.saveAsTextFile("output.txt");
// 关闭SparkContext
sc.close();
}
}
```
**逻辑分析:**
这段代码演示了如何使用Spark处理文本文件。它首先创建了一个SparkContext对象,然后创建一个RDD(弹性分布式数据集)来读取输入文件。接下来,它使用filter()转换对RDD进行过滤,只保留包含“error”字符串的行。最后,它将结果保存到一个输出文件中。
#### 3.1.2 Google Cloud Platform和Amazon Web Services
**Google Cloud Platform(GCP)**和**Amazon Web Services(AWS)**是两大云计算提供商。它们提供各种服务,包括:
- **计算**:虚拟机、容器和无服务器功能。
- **存储**:对象存储、块存储和文件存储。
- **数据库**:关系数据库、非关系数据库和内存数据库。
- **机器学习**:机器学习平台、模型训练和部署服务。
**表格:GCP和AWS服务的比较**
| 服务 | GCP | AWS |
|---|---|---|
| 计算 | Compute Engine | EC2 |
| 存储 | Cloud Storage | S3 |
| 数据库 | Cloud SQL | RDS |
| 机器学习 | Cloud ML Engine | SageMaker |
### 3.2 云端算法实现案例
#### 3.2.1 分布式数据处理
**使用Spark进行分布式数据处理**
Spark可以用于并行处理大数据集。例如,我们可以使用Spark来处理日志文件并计算每个IP地址的请求次数。
**代码块:**
```java
import org.apache.spark.SparkConf;
import org.apache.spark.SparkContext;
public class DistributedDataProcessing {
public static void main(String[] args) {
// 创建SparkConf对象
SparkConf conf = new SparkConf().setAppName("DistributedDataProcessing");
// 创建SparkContext对象
SparkContext sc = new SparkContext(conf);
// 创建RDD
RDD<String> rdd = sc.textFile("input.txt");
// 对RDD进行转换和操作
RDD<String> result = rdd.map(line -> {
String[] parts = line.split(" ");
return parts[0]; // 提取IP地址
}).groupBy(ip -> ip).count(); // 根据IP地址分组并计数
// 将结果保存到文件中
result.saveAsTextFile("output.txt");
```
0
0
相关推荐
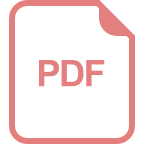
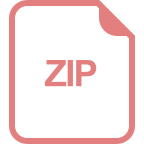
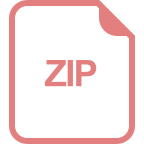





