【最短路径算法的并行化】:提升计算效率,加速解决问题
发布时间: 2024-07-10 18:49:07 阅读量: 42 订阅数: 39 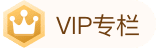
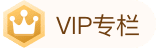
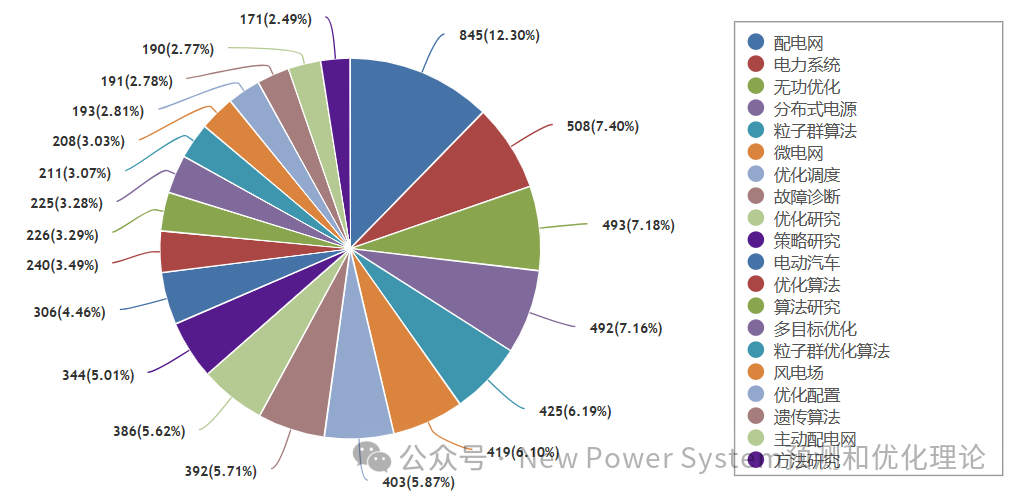
# 1. 最短路径算法概述
最短路径算法旨在找到从源点到目标点之间的一条路径,该路径的权重(例如距离、时间或成本)最小。这些算法广泛应用于各种领域,包括交通网络、通信网络和计算机图形学。
最短路径算法通常分为两类:
* **单源最短路径算法:**从一个源点到所有其他点的最短路径。
* **多源最短路径算法:**从多个源点到所有其他点的最短路径。
常见的单源最短路径算法包括 Dijkstra 算法和 Bellman-Ford 算法,而 Floyd-Warshall 算法是一种多源最短路径算法。
# 2. 并行化算法设计原理
### 2.1 并行计算的概念和优势
**并行计算**是指利用多个处理单元同时执行程序的不同部分,以提高计算效率。它与串行计算不同,后者一次只能执行一个任务。
并行计算的优势在于:
* **缩短计算时间:**通过并行执行任务,可以显著减少计算时间。
* **提高资源利用率:**并行计算可以充分利用多核处理器或多台计算机的计算能力,提高资源利用率。
* **解决复杂问题:**并行计算可以解决串行计算无法处理的大规模或复杂问题。
### 2.2 并行算法的设计模式
并行算法的设计模式是指将算法并行化的不同方法。常见的模式包括:
* **任务并行:**将任务分解成多个独立的子任务,并分配给不同的处理单元同时执行。
* **数据并行:**将数据分解成多个块,并分配给不同的处理单元同时处理。
* **管道并行:**将算法分解成一系列阶段,每个阶段由不同的处理单元执行,并将结果传递给下一个阶段。
* **混合并行:**结合上述模式,以实现最佳的并行化效果。
#### 代码示例:任务并行
```python
import concurrent.futures
def task(i):
# 执行任务
return i * i
def main():
# 创建线程池
with concurrent.futures.ThreadPoolExecutor() as executor:
# 提交任务
tasks = [executor.submit(task, i) for i in range(10)]
# 获取结果
results = [task.result() for task in tasks]
# 打印结果
print(results)
if __name__ == "__main__":
main()
```
**逻辑分析:**
* `task()` 函数定义了一个简单的任务,计算一个数字的平方。
* `main()` 函数创建了一个线程池,并使用 `executor.submit()` 提交任务。
* 线程池中的线程并行执行任务,并返回结果。
* 主线程等待所有任务完成,然后打印结果。
#### 代码示例:数据并行
```python
import numpy as np
def data_parallel(data):
# 将数据分解成块
blocks = np.array_split(data, 4)
# 创建线程池
with concurrent.futures.ThreadPoolExecutor() as executor:
# 提交任务
tasks = [executor.submit(np.sum, block) for block in blocks]
# 获取结果
results = [task.result() for task in tasks]
# 合并结果
return np.sum(results)
if __name__ == "__main__":
# 生成数据
data = np.random.rand(1000000)
# 并行计算数据和
result = data_parallel(data)
# 打印结果
print(result)
```
**逻辑分析:**
* `data_parallel()` 函数将数据分解成 4 个块。
* 创建一个线程池,并使用 `executor.submit()` 提交任务,每个任务计算一个块的和。
* 线程池中的线程并行执行任务,并返回结果。
* 主线程等待所有任务完成,并将结果合并。
# 3. Dijkstra算法的并行化
### 3.1 Dijkstra算法的串行实现
Dijkstra算法是一种经典的串行最短路径算法,用于计算加权有向图中从一个源点到所有其他顶点的最短路径。其基本思想是逐步扩展从源点出发的最短路径,直到到达所有顶点。
以下为Dijkstra算法的伪代码:
```python
def dijkstra(graph, source):
# 初始化距离和前驱节点
dist = [inf for _ in range(len(graph))]
prev = [None for _ in range(len(graph))]
dist[source] = 0
# 优先队列,按距离从小到大排序
pq = [(0, source)]
# 循环直到优先队列为空
while pq:
# 取出距离最小的顶点
current_dist, current_node = heapq.heappop(pq)
# 如果当前距离大于已知的距离,则跳过
if current_dist > dist[current_node]:
continue
# 遍历当前顶点的相邻节点
for neighbor in graph[current_node]:
```
0
0
相关推荐
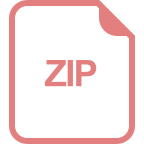
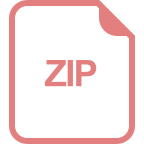
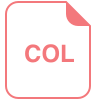
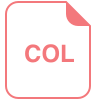
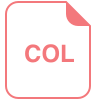
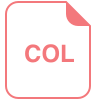


