最短路径算法的复杂度分析:理论与实践,深入理解
发布时间: 2024-07-10 18:46:18 阅读量: 66 订阅数: 28 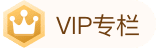
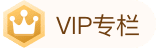
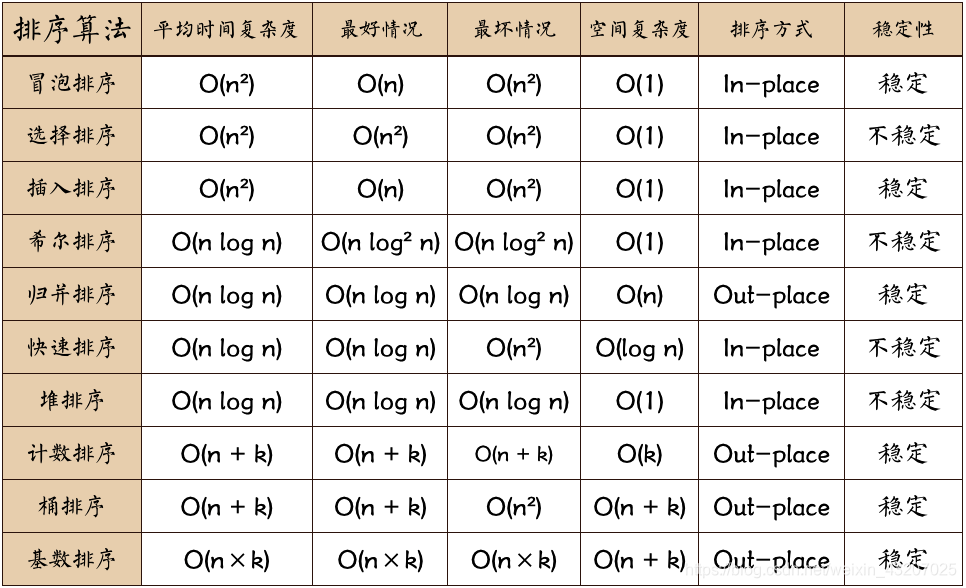
# 1. 最短路径算法的理论基础**
最短路径算法是一种计算机算法,用于在加权图或网络中找到从一个节点到另一个节点的最短路径。最短路径可以根据权重(例如距离、时间或成本)来衡量。
最短路径算法的基础是图论,图论是数学的一个分支,用于研究图的结构和性质。图由节点(也称为顶点)和连接节点的边组成。边的权重表示从一个节点到另一个节点的成本。
最短路径算法的工作原理是系统地探索图,从起始节点开始,并根据权重对路径进行评估。算法通过迭代过程逐步构建最短路径,直到找到从起始节点到目标节点的最优路径。
# 2. 最短路径算法的复杂度分析
### 2.1 算法的时间复杂度分析
算法的时间复杂度衡量算法在最坏情况下执行所需的时间。对于最短路径算法,时间复杂度通常取决于图的顶点数和边数。
#### 2.1.1 Dijkstra算法
Dijkstra算法的时间复杂度为O(V^2),其中V是图中的顶点数。该算法使用优先队列来维护未访问顶点的距离,并在每次迭代中选择距离最小的顶点进行访问。
**代码块:**
```python
import heapq
def dijkstra(graph, source):
dist = [float('inf')] * len(graph)
dist[source] = 0
pq = [(0, source)]
while pq:
current_dist, current_vertex = heapq.heappop(pq)
if current_dist > dist[current_vertex]:
continue
for neighbor in graph[current_vertex]:
distance = current_dist + graph[current_vertex][neighbor]
if distance < dist[neighbor]:
dist[neighbor] = distance
heapq.heappush(pq, (distance, neighbor))
return dist
```
**逻辑分析:**
* 初始化距离数组dist,将源顶点的距离设为0。
* 使用优先队列pq维护未访问顶点的距离。
* 每次从pq中弹出距离最小的顶点,并更新其相邻顶点的距离。
* 算法在所有顶点都被访问后结束。
#### 2.1.2 Floyd-Warshall算法
Floyd-Warshall算法的时间复杂度为O(V^3),其中V是图中的顶点数。该算法使用动态规划来计算所有顶点对之间的最短路径。
**代码块:**
```python
def floyd_warshall(graph):
dist = [[float('inf')] * len(graph) for _ in range(len(graph))]
for i in range(len(graph)):
dist[i][i] = 0
for k in range(len(graph)):
for i in range(len(graph)):
for j in range(len(graph)):
if dist[i][j] > dist[i][k] + dist[k][j]:
dist[i][j] = dist[i][k] + dist[k][j]
return dist
```
**逻辑分析:**
* 初始化距离矩阵dist,将对角线元素设为0。
* 使用三层循环迭代所有顶点对。
* 对于每个顶点对,检查是否存在通过中间顶点k的更短路径。
* 算法在所有顶点对的距离都更新后结束。
### 2.2 算法的空间复杂度分析
算法的空间复杂度衡量算法在执行过程中所需的最大内存空间。对于最短路径算法,空间复杂度通常取决于图的顶点数和边数。
#### 2.2.1 Dijkstra算法
Dijkstra算法的空间复杂度为O(V),其中V是图中的顶点数。该算法使用优先队列和距离数组,它们的大小与顶点数成正比。
#### 2.2.2 Floyd-Warshall算法
Floyd-Warshall算法的空间复杂度为O(V^2),其中V是图中的顶点数。该算法使用距离矩阵,其大小与顶点数的平方成正比。
# 3.1 网络路由中的应用
**3.1.1 路由表维护**
路由表是网络设备(如路由器)中维护的一张表,用于存储到其他网络设备的最佳路径。最短路径算法在路由表维护中扮演着至关重要的角色,它负责计算和更新路由表中的最佳路径。
**Dijkstra算法在路由表维护中的应用:**
Dijkstra算法是一种贪心算法,用于在加权有向图中找到从一个源点到所有其他点的最短路径。在路由表维护中,源点通常是路由器本身,而其他点是网络中的其他设备。Dijkstra算法从源点开始,逐个迭代,计算到每个点的最短路径,并更新路由表中的相应条目。
```python
def dijkstra(graph, source):
# 初始化距离表,将所有节点的距离设为无穷大,源点的距离为 0
distance = {node: float('inf') for node in graph}
distance[source] = 0
# 初始化未访问节点集合
unvisited = set(graph)
# 循环,直到未访问节点集合为空
while unvisited:
# 找到未访问节点中距离最小的节点
current = min(unvisited, key=distance.get)
# 将当前节点标记为已访问
unvisited.remove(current)
# 遍历当前节点的邻接节点
for neighbor in graph[current]:
# 计算通过当前节点到邻接节点的距离
new_distance = distance[current] + graph[current][neighbor]
# 如果通过当前节点到邻接节点的距离小于当前存储的距离,则更新距离表
if new_distance < distance[neighbor]:
distance[neighbor] = new_distance
return distance
```
**3.1.2 最短路径计算**
当网络设备需要将数据包发送到其他设备时,它需要计算到目标设备的最短路径。最短路径算法在最短路径计算中发挥着核心作用,它负责根据路由表中的信息计算出最佳路径。
**Floyd-Warshall算法在最短路径计算中的应用:**
Floyd-Warshall算法是一种动态规划算法,用于计算加权有向图中所有节点之间的最短路径。在最短路径计算中,Floyd-Warshall算法从源点开始,逐个迭代,计算到所有其他点的最短路径,并更新路由表中的相应条目。
```python
def floyd_warshall(graph):
# 初始化距离矩阵,将所有节点之间的距离设为无穷大,对角线元素为 0
distance = [[float('inf') for _ in range(len(graph))] for _ in range(len(graph))]
for i in range(len(graph)):
distance[i][i] = 0
# 遍历图中的所有边,更新距离矩阵
for u in graph:
for v in graph[u]:
distance[u][v] = graph[u][v]
# 逐个中间节点进行松弛操作
for k in range(len(graph)):
for i in range(len(graph)):
for j in range(len(graph)):
if distance[i][k] + distance[k][j] < distance[i][j]:
distance[i][j] = distance[i][k] + distance[k][j]
return distance
```
# 4. 最短路径算法的优化
在实际应用中,最短路径算法的效率至关重要,尤其是在处理大规模图时。为了提升算法的性能,研究人员提出了各种优化技
0
0
相关推荐
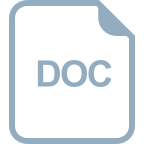
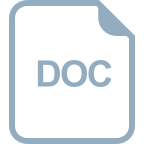
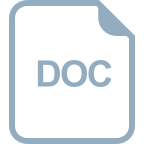
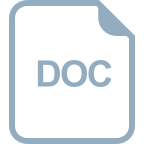
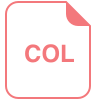
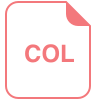
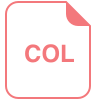
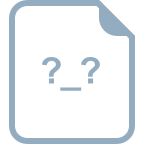
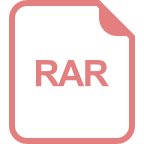