Java算法可视化工具性能优化:渲染效率提升的终极指南
发布时间: 2024-08-30 05:11:55 阅读量: 55 订阅数: 25 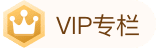
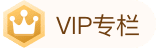
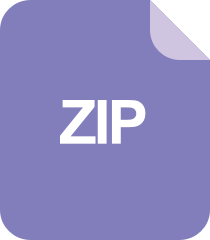
AlgorithmVisualizer:用Java swing制作的算法可视化工具
# 1. Java算法可视化工具基础
在信息技术飞速发展的今天,Java算法可视化工具作为帮助开发者和学习者直观理解算法运行过程的辅助工具,已经成为教学和开发中不可或缺的一部分。本章将介绍这些工具的基本概念和使用原理,为后续章节深入探讨性能挑战和优化策略打下坚实的基础。
## 1.1 算法可视化的概念与作用
算法可视化是指通过图形化的方式展示算法的执行过程,包括数据结构的改变、算法的运行步骤等。通过这种方式,复杂算法的逻辑和效率可以被直观地展示出来,从而提高学习效率和代码质量。例如,排序算法可视化能直观展示不同排序算法在执行时数组元素的变动情况。
## 1.2 Java算法可视化工具的实现原理
Java算法可视化工具一般通过两个主要部分实现:算法执行部分和图形展示部分。算法执行部分负责运行算法逻辑,而图形展示部分则负责将算法执行的中间状态转换为图形界面。Java通过其丰富的API支持多样的图形界面设计,如Swing或JavaFX,可创建出动态变化的图形界面。
在接下来的章节中,我们将深入探讨在使用这些工具时可能遇到的性能挑战,并提供相应的优化策略和工具使用技巧。
# 2. 算法可视化中的性能挑战
在构建和使用Java算法可视化工具时,性能挑战无处不在。从渲染效率到系统资源管理,每一个环节都可能影响最终用户体验和工具的稳定性。本章将深入探讨这些性能挑战,以及如何使用性能分析工具和方法来识别和解决这些挑战。
## 2.1 渲染效率的重要性
渲染效率在任何图形界面密集型应用中都至关重要,算法可视化工具尤其如此。它直接关系到用户能否实时、流畅地观察算法的运行过程。
### 2.1.1 渲染效率对用户体验的影响
用户体验是评价任何工具成功与否的重要指标。在算法可视化工具中,渲染效率的高低决定了动画的平滑程度和信息更新的即时性。如果渲染效率低下,用户可能会观察到卡顿、延迟,甚至是渲染错误。这些问题会打断用户的思维连贯性,降低学习和理解算法的效果。
### 2.1.2 渲染效率对系统资源的影响
除了用户体验之外,渲染效率还直接影响到系统资源的使用。高效的渲染可以减少CPU和GPU的负载,降低内存的消耗。这对于运行在低配置设备上的可视化工具尤为重要。高效率的渲染能够保证算法可视化的流畅,同时为其他后台任务保留更多资源。
## 2.2 性能分析工具和方法
为了识别和改善性能瓶颈,性能分析工具和方法是不可或缺的。这些工具和方法可以帮助开发者监控程序运行状态,定位性能瓶颈,并实施相应的优化措施。
### 2.2.1 性能监控工具的使用
Java提供了多种性能监控工具,如JVisualVM、JProfiler和Java Mission Control等。这些工具能够监控内存使用情况、CPU负载、线程状态等关键性能指标。通过分析这些数据,开发者可以了解程序运行时的资源分配和使用情况,从而对可能的性能问题进行预测和诊断。
### 2.2.2 瓶颈定位与性能测试方法
确定了性能瓶颈之后,下一步就是进行针对性的性能测试。这通常涉及压力测试、回归测试和基准测试等方法。例如,可以通过压力测试来评估算法在高负载下的表现,或者使用回归测试来确保优化措施不会引入新的问题。基准测试则可以帮助开发者比较不同优化策略的效果,选择最优解。
### Java性能测试工具实例
```java
// 一个简单的性能测试类示例,使用JMH进行基准测试
import org.openjdk.jmh.annotations.*;
import org.openjdk.jmh.results.format.ResultFormatType;
import org.openjdk.jmh.runner.Runner;
import org.openjdk.jmh.runner.RunnerException;
import org.openjdk.jmh.runner.options.Options;
import org.openjdk.jmh.runner.options.OptionsBuilder;
@State(Scope.Thread)
@BenchmarkMode(Mode.AverageTime)
@OutputTimeUnit(TimeUnit.NANOSECONDS)
public class SortBenchmark {
private int[] array = new int[1024];
@Setup(Level.Iteration)
public void prepare() {
// 初始化测试数据
}
@Benchmark
public void benchmarkSort() {
// 执行排序算法
}
public static void main(String[] args) throws RunnerException {
Options opt = new OptionsBuilder()
.include(SortBenchmark.class.getSimpleName())
.forks(1)
.warmupIterations(5)
.measurementIterations(5)
.resultFormat(ResultFormatType.CSV)
.build();
new Runner(opt).run();
}
}
```
在上述代码中,我们定义了一个性能测试基准类`SortBenchmark`,使用JMH(Java Microbenchmark Harness)来测量排序算法的性能。通过`@Benchmark`注解定义了基准测试方法,并通过`@Setup`注解来初始化测试环境。最终,通过`Runner`类来执行测试,并输出CSV格式的测试结果。
性能测试是提高渲染效率和整体系统性能的关键步骤。开发者需要定期执行这些测试,并根据测试结果来调整和优化代码。通过性能测试,可以确保算法可视化工具在不同的使用场景和硬件配置下都能提供良好的用户体验。
# 3. 优化Java算法可视化工具的技术路径
## 3.1 算法层面的优化策略
### 3.1.1 算法选择与优化
在Java算法可视化工具中,选择合适的算法对于提高性能至关重要。当处理大量数据时,简单直观的算法可能会导致性能瓶颈。例如,在排序操作中,传统的冒泡排序虽然易于实现,但时间复杂度为O(n^2),在面对大规模数据集时,性能明显不足。因此,优化策略之一是选择更高效的算法,比如快速排序或者归并排序,它们的时间复杂度为O(nlogn),可以显著提升处理速度。
除了选择高效算法外,对现有算法进行优化也很重要。例如,对快速排序进行优化,可以采用随机选基准元素的方法来减少最坏情况下的性能损失。此外,针对特定问题的算法改造,如使用哈希表优化查找操作,可以将时间复杂度从O(n)降低到O(1)。
```java
// 示例代码:快速排序优化版本
public static void quickSort(int[] array, int low, int high) {
if (low < high) {
int pivot = partition(array, low, high);
quickSort(array, low, pivot - 1);
quickSort(array, pivot + 1, high);
}
}
private static int partition(int[] array, int low, int high) {
int pivot = array[high];
int i = (low - 1);
for (int j = low; j < high; j++) {
if (array[j] < pivot) {
i++;
swap(array, i, j);
}
}
swap(array, i + 1, high);
return (i + 1);
}
private static
```
0
0
相关推荐
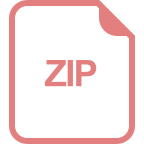
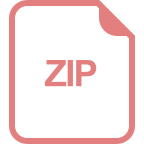
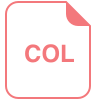
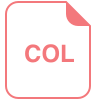
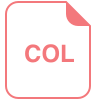
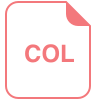
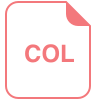
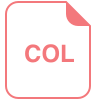