【PHP连接MySQL数据库的终极指南】:从小白到大师的进阶之路
发布时间: 2024-07-24 00:16:11 阅读量: 16 订阅数: 18 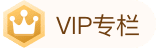
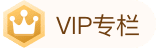

# 1. PHP与MySQL数据库简介**
PHP是一种流行的服务器端脚本语言,广泛用于Web开发。MySQL是一种关系型数据库管理系统(RDBMS),以其高性能、可扩展性和可靠性而闻名。PHP和MySQL的结合提供了强大的平台,用于构建动态、数据驱动的Web应用程序。
本指南将介绍PHP与MySQL数据库的集成,涵盖从建立连接到执行查询和管理数据的各个方面。通过循序渐进的示例和深入的解释,您将掌握使用PHP有效连接和操作MySQL数据库所需的技能。
# 2. PHP连接MySQL数据库的实践技巧
### 2.1 建立数据库连接
#### 2.1.1 使用mysqli_connect()函数
`mysqli_connect()` 函数用于建立与 MySQL 数据库的连接。其语法如下:
```php
mysqli_connect(host, username, password, dbname, port, socket)
```
| 参数 | 描述 |
|---|---|
| host | 数据库服务器主机名或 IP 地址 |
| username | 数据库用户名 |
| password | 数据库密码 |
| dbname | 要连接的数据库名称 |
| port | 数据库服务器端口号(可选,默认为 3306) |
| socket | 数据库服务器套接字文件路径(可选,默认为 /tmp/mysql.sock) |
**代码示例:**
```php
$servername = "localhost";
$username = "root";
$password = "";
$dbname = "myDB";
// 建立数据库连接
$conn = mysqli_connect($servername, $username, $password, $dbname);
// 检查连接是否成功
if (!$conn) {
die("连接失败:" . mysqli_connect_error());
}
```
**逻辑分析:**
* 第 2 行:指定数据库服务器主机名或 IP 地址。
* 第 3 行:指定数据库用户名。
* 第 4 行:指定数据库密码。
* 第 5 行:指定要连接的数据库名称。
* 第 7-9 行:使用 `mysqli_connect()` 函数建立数据库连接。
* 第 11 行:使用 `mysqli_connect_error()` 函数检查连接是否成功。
#### 2.1.2 处理连接错误
如果数据库连接失败,`mysqli_connect()` 函数将返回 `false`。可以通过 `mysqli_connect_error()` 函数获取连接错误信息。
**代码示例:**
```php
if (!$conn) {
die("连接失败:" . mysqli_connect_error());
}
```
**逻辑分析:**
* 第 2 行:检查数据库连接是否成功。
* 第 3 行:如果连接失败,使用 `mysqli_connect_error()` 函数获取连接错误信息并输出。
### 2.2 执行SQL查询
#### 2.2.1 使用mysqli_query()函数
`mysqli_query()` 函数用于执行 SQL 查询。其语法如下:
```php
mysqli_query(connection, query)
```
| 参数 | 描述 |
|---|---|
| connection | 数据库连接 |
| query | 要执行的 SQL 查询 |
**代码示例:**
```php
// 执行 SQL 查询
$result = mysqli_query($conn, "SELECT * FROM users");
// 检查查询是否成功
if (!$result) {
die("查询失败:" . mysqli_error($conn));
}
```
**逻辑分析:**
* 第 2 行:使用 `mysqli_query()` 函数执行 SQL 查询。
* 第 4 行:使用 `mysqli_error()` 函数检查查询是否成功。
#### 2.2.2 处理查询结果
`mysqli_query()` 函数返回一个结果集对象。可以通过以下方法处理查询结果:
* `mysqli_fetch_assoc()`:以关联数组的形式获取一行查询结果。
* `mysqli_fetch_row()`:以数字数组的形式获取一行查询结果。
* `mysqli_fetch_object()`:以对象的形式获取一行查询结果。
**代码示例:**
```php
// 循环遍历查询结果
while ($row = mysqli_fetch_assoc($result)) {
echo "ID: " . $row["id"] . ", Name: " . $row["name"] . "<br>";
}
```
**逻辑分析:**
* 第 2 行:使用 `mysqli_fetch_assoc()` 函数以关联数组的形式获取一行查询结果。
* 第 3 行:使用 `while` 循环遍历查询结果。
* 第 4 行:输出查询结果。
### 2.3 插入、更新和删除数据
#### 2.3.1 使用mysqli_query()函数
`mysqli_query()` 函数也可以用于插入、更新和删除数据。其语法如下:
```php
mysqli_query(connection, query)
```
| 参数 | 描述 |
|---|---|
| connection | 数据库连接 |
| query | 要执行的 SQL 查询 |
**代码示例:**
```php
// 插入数据
$sql = "INSERT INTO users (name, email) VALUES ('John Doe', 'john@example.com')";
mysqli_query($conn, $sql);
// 更新数据
$sql = "UPDATE users SET name = 'Jane Doe' WHERE id = 1";
mysqli_query($conn, $sql);
// 删除数据
$sql = "DELETE FROM users WHERE id = 1";
mysqli_query($conn, $sql);
```
**逻辑分析:**
* 第 2-4 行:执行插入数据的 SQL 查询。
* 第 6-8 行:执行更新数据的 SQL 查询。
* 第 10-12 行:执行删除数据的 SQL 查询。
#### 2.3.2 准备语句的应用
准备语句可以防止 SQL 注入攻击,提高查询效率。其语法如下:
```php
mysqli_prepare(connection, query)
```
| 参数 | 描述 |
|---|---|
| connection | 数据库连接 |
| query | 要执行的 SQL 查询 |
**代码示例:**
```php
// 准备语句
$stmt = mysqli_prepare($conn, "INSERT INTO users (name, email) VALUES (?, ?)");
// 绑定参数
mysqli_stmt_bind_param($stmt, "ss", $name, $email);
// 设置参数值
$name = "John Doe";
$email = "john@example.com";
// 执行准备语句
mysqli_stmt_execute($stmt);
```
**逻辑分析:**
* 第 2 行:使用 `mysqli_prepare()` 函数准备 SQL 查询。
* 第 4 行:使用 `mysqli_stmt_bind_param()` 函数绑定参数。
* 第 6-8 行:设置参数值。
* 第 10 行:执行准备语句。
# 3. PHP连接MySQL数据库的进阶应用
### 3.1 数据库事务处理
#### 3.1.1 事务的基本概念
事务是数据库中的一系列操作,这些操作要么全部成功,要么全部失败。事务确保数据库中的数据保持一致性,即使在操作过程中发生错误或系统故障。
#### 3.1.2 使用mysqli_begin_transaction()函数
```php
<?php
// 打开数据库连接
$mysqli = new mysqli("localhost", "username", "password", "database_name");
// 开始事务
$mysqli->begin_transaction();
// 执行查询
$sql = "INSERT INTO users (name, email) VALUES ('John Doe', 'john.doe@example.com')";
$mysqli->query($sql);
// 提交事务
$mysqli->commit();
// 关闭数据库连接
$mysqli->close();
?>
```
**逻辑分析:**
* `mysqli_begin_transaction()` 函数开启一个事务。
* `mysqli_query()` 函数执行插入查询。
* `mysqli_commit()` 函数提交事务,将更改永久保存到数据库中。
* 如果在事务过程中发生错误,`mysqli_rollback()` 函数可用于回滚更改。
### 3.2 数据库优化
#### 3.2.1 索引的创建和使用
索引是数据库表中用于快速查找数据的特殊数据结构。通过在经常查询的列上创建索引,可以显著提高查询性能。
**创建索引:**
```sql
CREATE INDEX index_name ON table_name (column_name);
```
**使用索引:**
```php
<?php
// 打开数据库连接
$mysqli = new mysqli("localhost", "username", "password", "database_name");
// 使用索引执行查询
$sql = "SELECT * FROM users WHERE name LIKE '%John%'";
$result = $mysqli->query($sql);
// 关闭数据库连接
$mysqli->close();
?>
```
**逻辑分析:**
* 在 `users` 表的 `name` 列上创建索引。
* `LIKE` 查询使用索引进行优化,从而提高查询速度。
#### 3.2.2 查询缓存的应用
查询缓存是一种机制,它将最近执行的查询及其结果存储在内存中。当后续查询与缓存中的查询匹配时,数据库将直接从缓存中返回结果,从而避免执行查询。
**启用查询缓存:**
```php
<?php
// 打开数据库连接
$mysqli = new mysqli("localhost", "username", "password", "database_name");
// 启用查询缓存
$mysqli->query("SET query_cache_size = 1024000");
// 执行查询
$sql = "SELECT * FROM users WHERE name LIKE '%John%'";
$result = $mysqli->query($sql);
// 关闭数据库连接
$mysqli->close();
?>
```
**逻辑分析:**
* `SET query_cache_size` 语句将查询缓存大小设置为 1MB。
* 后续查询将使用查询缓存,从而提高性能。
### 3.3 数据库安全
#### 3.3.1 SQL注入攻击的原理
SQL注入攻击是一种利用恶意输入来修改或执行未经授权的 SQL 查询的攻击。攻击者通过在用户输入中注入 SQL 语句来实现攻击。
**示例:**
```php
<?php
// 打开数据库连接
$mysqli = new mysqli("localhost", "username", "password", "database_name");
// 获取用户输入
$username = $_GET['username'];
// 构建查询
$sql = "SELECT * FROM users WHERE username = '$username'";
$result = $mysqli->query($sql);
// 关闭数据库连接
$mysqli->close();
?>
```
**逻辑分析:**
* 用户输入直接拼接在 SQL 查询中。
* 攻击者可以通过输入恶意用户名(例如 `' OR 1=1 -- `)来绕过身份验证并访问所有用户数据。
#### 3.3.2 防范SQL注入攻击的措施
* **使用参数化查询:**使用参数化查询可以防止 SQL 注入攻击,因为它将用户输入作为参数而不是直接拼接在查询中。
* **过滤用户输入:**对用户输入进行过滤,删除任何潜在的恶意字符。
* **使用白名单:**只允许用户输入预定义的有效值。
# 4. PHP连接MySQL数据库的项目实战
### 4.1 用户注册系统
#### 4.1.1 用户信息收集和验证
用户注册系统是网站中常见的模块,它负责收集和验证用户注册信息,并将其存储到数据库中。
**步骤:**
1. **创建注册表单:**使用HTML和PHP创建注册表单,收集用户的姓名、电子邮件、密码等信息。
2. **验证表单数据:**使用PHP对表单数据进行验证,确保数据格式正确且符合要求。
3. **加密密码:**使用密码哈希函数(如bcrypt)对用户密码进行加密,以增强安全性。
#### 4.1.2 数据入库和加密
**代码块:**
```php
$stmt = $conn->prepare("INSERT INTO users (name, email, password) VALUES (?, ?, ?)");
$stmt->bind_param("sss", $name, $email, $password);
$stmt->execute();
```
**逻辑分析:**
* `$stmt`:准备语句对象,用于插入数据。
* `$conn`:数据库连接对象。
* `prepare()`:准备SQL查询,防止SQL注入攻击。
* `bind_param()`:绑定参数到准备语句,确保数据类型匹配。
* `execute()`:执行准备语句,将数据插入数据库。
**参数说明:**
* `$name`:用户姓名。
* `$email`:用户电子邮件。
* `$password`:用户密码(已加密)。
### 4.2 博客管理系统
#### 4.2.1 文章发布和编辑
**代码块:**
```php
$stmt = $conn->prepare("INSERT INTO posts (title, content, author_id) VALUES (?, ?, ?)");
$stmt->bind_param("sss", $title, $content, $author_id);
$stmt->execute();
```
**逻辑分析:**
* `$stmt`:准备语句对象,用于插入数据。
* `$conn`:数据库连接对象。
* `prepare()`:准备SQL查询,防止SQL注入攻击。
* `bind_param()`:绑定参数到准备语句,确保数据类型匹配。
* `execute()`:执行准备语句,将数据插入数据库。
**参数说明:**
* `$title`:文章标题。
* `$content`:文章内容。
* `$author_id`:作者ID。
#### 4.2.2 评论管理和回复
**代码块:**
```php
$stmt = $conn->prepare("SELECT * FROM comments WHERE post_id = ?");
$stmt->bind_param("i", $post_id);
$stmt->execute();
```
**逻辑分析:**
* `$stmt`:准备语句对象,用于查询数据。
* `$conn`:数据库连接对象。
* `prepare()`:准备SQL查询,防止SQL注入攻击。
* `bind_param()`:绑定参数到准备语句,确保数据类型匹配。
* `execute()`:执行准备语句,获取查询结果。
**参数说明:**
* `$post_id`:文章ID。
### 4.3 在线购物系统
#### 4.3.1 商品管理和库存
**代码块:**
```php
$stmt = $conn->prepare("UPDATE products SET quantity = ? WHERE id = ?");
$stmt->bind_param("ii", $quantity, $product_id);
$stmt->execute();
```
**逻辑分析:**
* `$stmt`:准备语句对象,用于更新数据。
* `$conn`:数据库连接对象。
* `prepare()`:准备SQL查询,防止SQL注入攻击。
* `bind_param()`:绑定参数到准备语句,确保数据类型匹配。
* `execute()`:执行准备语句,更新数据库中的数据。
**参数说明:**
* `$quantity`:商品数量。
* `$product_id`:商品ID。
#### 4.3.2 订单处理和支付
**代码块:**
```php
$stmt = $conn->prepare("INSERT INTO orders (user_id, product_id, quantity, total_price) VALUES (?, ?, ?, ?)");
$stmt->bind_param("iiii", $user_id, $product_id, $quantity, $total_price);
$stmt->execute();
```
**逻辑分析:**
* `$stmt`:准备语句对象,用于插入数据。
* `$conn`:数据库连接对象。
* `prepare()`:准备SQL查询,防止SQL注入攻击。
* `bind_param()`:绑定参数到准备语句,确保数据类型匹配。
* `execute()`:执行准备语句,将数据插入数据库。
**参数说明:**
* `$user_id`:用户ID。
* `$product_id`:商品ID。
* `$quantity`:商品数量。
* `$total_price`:订单总价。
# 5. PHP连接MySQL数据库的常见问题与解决方案**
**5.1 连接失败**
**5.1.1 检查数据库配置**
* 确认数据库服务器地址、用户名、密码和数据库名称是否正确。
* 检查数据库服务器是否正在运行。
* 检查PHP脚本是否具有连接数据库的权限。
**5.1.2 检查网络连接**
* 确保PHP脚本可以访问数据库服务器。
* 检查防火墙或其他网络设备是否阻止了连接。
* 使用ping命令测试与数据库服务器的连接。
**5.2 查询出错**
**5.2.1 检查SQL语法**
* 确保SQL查询语法正确无误。
* 使用调试工具或IDE检查查询中的语法错误。
* 尝试使用更简单的查询来排除语法问题。
**5.2.2 检查数据类型匹配**
* 确保SQL查询中的数据类型与数据库表中的字段数据类型相匹配。
* 使用类型转换函数或强制转换来解决数据类型不匹配的问题。
**5.3 数据安全隐患**
**5.3.1 使用参数化查询**
* 使用参数化查询来防止SQL注入攻击。
* 使用mysqli_prepare()和mysqli_bind_param()函数来准备和绑定查询参数。
**5.3.2 过滤用户输入**
* 使用htmlspecialchars()或其他过滤函数来过滤用户输入,防止恶意代码注入。
* 对用户输入进行类型检查和范围验证,以确保数据的完整性和安全性。
0
0
相关推荐
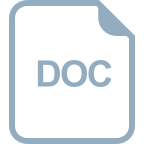





