揭秘Dijkstra算法:从原理到Java实现,深度剖析算法奥秘,掌握最短路径计算
发布时间: 2024-08-27 23:56:47 阅读量: 46 订阅数: 31 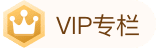
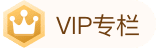
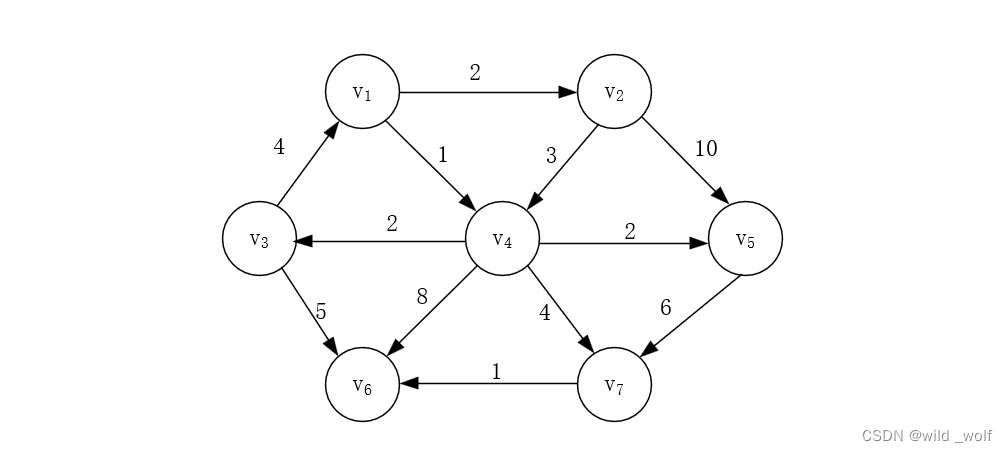
# 1. Dijkstra算法概述
Dijkstra算法是一种广泛应用于图论中的算法,用于求解带权图中从一个源点到所有其他顶点的最短路径。该算法由荷兰计算机科学家Edsger W. Dijkstra于1956年提出,以其简洁、高效和易于实现的特点而闻名。
Dijkstra算法的基本思想是:从源点出发,逐个探索所有可达的顶点,并不断更新到各个顶点的最短路径长度。在每个探索步骤中,算法选择当前距离源点最短的未探索顶点,并以该顶点为中心,更新到其相邻顶点的最短路径长度。这一过程持续进行,直到所有顶点都被探索完毕。
# 2. Dijkstra算法原理**
**2.1 算法思想和流程**
Dijkstra算法是一种贪心算法,用于求解有向或无向加权图中单源最短路径问题。其基本思想是:从源点出发,逐个选择权重最小的边,将该边的终点加入到已知最短路径集合中,并更新其他顶点的最短路径距离。
算法流程如下:
1. 初始化:将源点加入已知最短路径集合,其他顶点的最短路径距离设为无穷大。
2. 迭代:从已知最短路径集合中选择权重最小的边,将该边的终点加入已知最短路径集合。
3. 更新:更新其他顶点的最短路径距离,如果通过新加入的顶点可以找到更短的路径,则更新该顶点的最短路径距离。
4. 终止:当所有顶点都加入已知最短路径集合时,算法终止。
**2.2 算法的数学基础**
Dijkstra算法的数学基础是松弛操作。松弛操作是指更新顶点v的最短路径距离的过程。如果通过新加入的顶点u可以找到一条到v的更短路径,则执行松弛操作:
```
if (dist[u] + weight(u, v) < dist[v]) {
dist[v] = dist[u] + weight(u, v);
prev[v] = u;
}
```
其中:
* `dist[v]` 表示从源点到顶点v的最短路径距离
* `weight(u, v)` 表示边(u, v)的权重
* `prev[v]` 表示顶点v的前驱顶点
**2.3 算法的复杂度分析**
Dijkstra算法的时间复杂度为O(V^2),其中V是图中顶点的数量。这是因为算法需要迭代V次,每次迭代需要检查所有顶点的最短路径距离,最多需要O(V)的时间。
空间复杂度为O(V),因为算法需要存储每个顶点的最短路径距离和前驱顶点。
# 3.1 Java代码实现
```java
import java.util.*;
public class Dijkstra {
private static final int INF = Integer.MAX_VALUE;
public static void main(String[] args) {
// 图的邻接矩阵
int[][] graph = {
{0, 1, INF, INF, INF},
{1, 0, 1, INF, INF},
{INF, 1, 0, 1, 1},
{INF, INF, 1, 0, 1},
{INF, INF, 1, 1, 0}
};
// 起始节点
int start = 0;
// 距离数组
int[] distance = new int[graph.length];
// 已访问节点集合
Set<Integer> visited = new HashSet<>();
// 初始化距离数组
for (int i = 0; i < graph.length; i++) {
distance[i] = INF;
}
distance[start] = 0;
// 循环遍历所有节点
while (visited.size() < graph.length) {
// 寻找未访问节点中距离最小的节点
int minNode = -1;
int minDistance = INF;
for (int i = 0; i < graph.length; i++) {
if (!visited.contains(i) && distance[i] < minDistance) {
minNode = i;
minDistance = distance[i];
}
}
// 如果没有找到未访问的节点,则算法结束
if (minNode == -1) {
break;
}
// 将当前节点标记为已访问
visited.add(minNode);
// 更新相邻节点的距离
for (int i = 0; i < graph.length; i++) {
if (graph[minNode][i] != INF && !visited.contains(i)) {
distance[i] = Math.min(distance[i], distance[minNode] + graph[minNode][i]);
}
}
}
// 输出结果
System.out.println("从节点" + start + "到其他节点的最短距离:");
for (int i = 0; i < graph.length; i++) {
System.out.println("到节点" + i + "的距离:" + (distance[i] == INF ? "不可达" : distance[i]));
}
}
}
```
### 3.2 算法实现的细节
Dijkstra算法的Java代码实现主要包括以下几个步骤:
1. **初始化距离数组:**将所有节点的距离初始化为无穷大,起始节点的距离初始化为0。
2. **循环遍历所有节点:**在未访问节点中寻找距离最小的节点,并将其标记为已访问。
3. **更新相邻节点的距离:**对于当前节点的每个相邻节点,如果该节点未被访问,则更新其距离为当前节点距离加上当前节点到该节点的权重。
4. **重复步骤2和3,直到所有节点都被访问。**
### 3.3 算法实现的优化
Dijkstra算法的实现可以进行一些优化,以提高其效率。其中一种常见的优化方法是使用**优先队列**。
优先队列是一种数据结构,它可以存储元素并根据元素的优先级对元素进行排序。在Dijkstra算法中,我们可以使用优先队列来存储未访问的节点,并根据节点的距离对节点进行排序。
使用优先队列的优化算法如下:
```java
import java.util.*;
public class DijkstraOptimized {
private static final int INF = Integer.MAX_VALUE;
public static void main(String[] args) {
// 图的邻接矩阵
int[][] graph = {
{0, 1, INF, INF, INF},
{1, 0, 1, INF, INF},
{INF, 1, 0, 1, 1},
{INF, INF, 1, 0, 1},
{INF, INF, 1, 1, 0}
};
// 起始节点
int start = 0;
// 距离数组
int[] distance = new int[graph.length];
// 已访问节点集合
Set<Integer> visited = new HashSet<>();
// 初始化距离数组
for (int i = 0; i < graph.length; i++) {
distance[i] = INF;
}
distance[start] = 0;
// 使用优先队列存储未访问节点
PriorityQueue<Integer> queue = new PriorityQueue<>((a, b) -> distance[a] - distance[b]);
queue.add(start);
// 循环遍历所有节点
while (!queue.isEmpty()) {
// 从优先队列中取出距离最小的节点
int minNode = queue.poll();
// 如果该节点已被访问,则跳过
if (visited.contains(minNode)) {
continue;
}
// 将当前节点标记为已访问
visited.add(minNode);
// 更新相邻节点的距离
for (int i = 0; i < graph.length; i++) {
if (graph[minNode][i] != INF && !visited.contains(i)) {
distance[i] = Math.min(distance[i], distance[minNode] + graph[minNode][i]);
queue.add(i);
}
}
}
// 输出结果
System.out.println("从节点" + start + "到其他节点的最短距离:");
for (int i = 0; i < graph.length; i++) {
System.out.println("到节点" + i + "的距离:" + (distance[i] == INF ? "不可达" : distance[i]));
}
}
}
```
使用优先队列的优化算法的时间复杂度为O((V+E)logV),其中V是图中的节点数,E是图中的边数。
# 4. Dijkstra算法应用
### 4.1 最短路径计算
Dijkstra算法最经典的应用是计算图中两个顶点之间的最短路径。给定一个图G=(V,E),其中V是顶点的集合,E是边的集合,以及两个顶点s和t,Dijkstra算法可以找到从s到t的最短路径。
**算法步骤:**
1. 初始化一个距离数组dist,其中dist[v]表示从s到v的最短距离。dist[s]初始化为0,其他所有顶点的dist初始化为无穷大。
2. 初始化一个队列Q,其中Q包含所有未访问的顶点。
3. 循环执行以下步骤,直到Q为空:
- 从Q中取出dist最小的顶点v。
- 对于v的每个邻接顶点w,计算从s到w的距离newDist = dist[v] + weight(v, w),其中weight(v, w)是边(v, w)的权重。
- 如果newDist < dist[w],则更新dist[w] = newDist,并将w加入队列Q。
4. dist[t]即为从s到t的最短距离。
**代码示例:**
```java
import java.util.*;
public class Dijkstra {
public static int[] dijkstra(Graph graph, int source) {
int[] dist = new int[graph.V];
Arrays.fill(dist, Integer.MAX_VALUE);
dist[source] = 0;
Queue<Integer> queue = new PriorityQueue<>((a, b) -> dist[a] - dist[b]);
queue.add(source);
while (!queue.isEmpty()) {
int v = queue.poll();
for (Edge edge : graph.adj[v]) {
int w = edge.to;
int newDist = dist[v] + edge.weight;
if (newDist < dist[w]) {
dist[w] = newDist;
queue.add(w);
}
}
}
return dist;
}
public static void main(String[] args) {
Graph graph = new Graph(5);
graph.addEdge(0, 1, 4);
graph.addEdge(0, 2, 2);
graph.addEdge(1, 2, 3);
graph.addEdge(1, 3, 2);
graph.addEdge(2, 3, 1);
graph.addEdge(2, 4, 5);
graph.addEdge(3, 4, 1);
int[] dist = dijkstra(graph, 0);
for (int i = 0; i < dist.length; i++) {
System.out.println("最短路径距离[0, " + i + "] = " + dist[i]);
}
}
}
class Graph {
int V;
List<List<Edge>> adj;
public Graph(int V) {
this.V = V;
adj = new ArrayList<>();
for (int i = 0; i < V; i++) {
adj.add(new ArrayList<>());
}
}
public void addEdge(int u, int v, int weight) {
adj.get(u).add(new Edge(v, weight));
}
}
class Edge {
int to;
int weight;
public Edge(int to, int weight) {
this.to = to;
this.weight = weight;
}
}
```
### 4.2 路由选择
Dijkstra算法还可用于路由选择。在网络中,每个节点都可以看作是一个顶点,而连接节点的链路可以看作是边。Dijkstra算法可以计算从源节点到目标节点的最短路径,从而确定最佳的路由。
### 4.3 图论中的其他应用
Dijkstra算法在图论中还有许多其他应用,包括:
- **最小生成树:** Dijkstra算法可以用来构造图的最小生成树,即连接图中所有顶点的权重最小的边集合。
- **最大流:** Dijkstra算法可以用来计算图中最大流,即从源点到汇点的最大流量。
- **拓扑排序:** Dijkstra算法可以用来对有向无环图进行拓扑排序,即按顶点的依赖关系对顶点进行排序。
# 5. Dijkstra算法的变种**
Dijkstra算法是一种高效的单源最短路径算法,但它在某些情况下存在局限性。为了克服这些局限性,提出了多种Dijkstra算法的变种。
**5.1 A*算法**
A*算法是Dijkstra算法的一种启发式搜索算法,它通过引入启发函数来指导搜索过程。启发函数估计当前节点到目标节点的距离,并将其添加到当前节点的权重中。这样,A*算法可以优先探索那些距离目标节点更近的节点,从而提高搜索效率。
```java
public class AStar {
private static final int[][] DIRECTIONS = {{-1, 0}, {0, 1}, {1, 0}, {0, -1}};
public static int[][] search(int[][] grid, int startX, int startY, int endX, int endY) {
PriorityQueue<Node> openSet = new PriorityQueue<>();
HashSet<Node> closedSet = new HashSet<>();
Node startNode = new Node(startX, startY, 0, calculateHeuristic(startX, startY, endX, endY));
openSet.add(startNode);
while (!openSet.isEmpty()) {
Node currentNode = openSet.poll();
if (currentNode.x == endX && currentNode.y == endY) {
return reconstructPath(currentNode);
}
closedSet.add(currentNode);
for (int[] direction : DIRECTIONS) {
int newX = currentNode.x + direction[0];
int newY = currentNode.y + direction[1];
if (newX >= 0 && newX < grid.length && newY >= 0 && newY < grid[0].length && !closedSet.contains(new Node(newX, newY, 0, 0))) {
int newCost = currentNode.cost + grid[newX][newY];
int newHeuristic = calculateHeuristic(newX, newY, endX, endY);
Node newNode = new Node(newX, newY, newCost, newHeuristic);
if (!openSet.contains(newNode)) {
newNode.parent = currentNode;
openSet.add(newNode);
}
}
}
}
return null;
}
private static int calculateHeuristic(int x, int y, int endX, int endY) {
return Math.abs(x - endX) + Math.abs(y - endY);
}
private static int[][] reconstructPath(Node node) {
LinkedList<int[]> path = new LinkedList<>();
while (node != null) {
path.addFirst(new int[]{node.x, node.y});
node = node.parent;
}
return path.toArray(new int[path.size()][]);
}
private static class Node implements Comparable<Node> {
int x;
int y;
int cost;
int heuristic;
Node parent;
public Node(int x, int y, int cost, int heuristic) {
this.x = x;
this.y = y;
this.cost = cost;
this.heuristic = heuristic;
}
@Override
public int compareTo(Node other) {
return (this.cost + this.heuristic) - (other.cost + other.heuristic);
}
}
}
```
**5.2 Bellman-Ford算法**
Bellman-Ford算法是一种可以处理负权边的单源最短路径算法。它通过对所有边进行多次松弛操作来找到最短路径。
```java
public class BellmanFord {
public static int[] search(int[][] graph, int source) {
int[] distances = new int[graph.length];
Arrays.fill(distances, Integer.MAX_VALUE);
distances[source] = 0;
for (int i = 0; i < graph.length - 1; i++) {
for (int[] edge : graph) {
int u = edge[0];
int v = edge[1];
int weight = edge[2];
if (distances[u] + weight < distances[v]) {
distances[v] = distances[u] + weight;
}
}
}
for (int[] edge : graph) {
int u = edge[0];
int v = edge[1];
int weight = edge[2];
if (distances[u] + weight < distances[v]) {
throw new RuntimeException("Negative cycle detected");
}
}
return distances;
}
}
```
**5.3 Floyd-Warshall算法**
Floyd-Warshall算法是一种可以计算所有对最短路径的算法。它通过创建一张距离矩阵来存储所有节点之间的最短路径,并通过动态规划的方式更新距离矩阵。
```java
public class FloydWarshall {
public static int[][] search(int[][] graph) {
int[][] distances = new int[graph.length][graph.length];
for (int i = 0; i < graph.length; i++) {
for (int j = 0; j < graph.length; j++) {
distances[i][j] = graph[i][j];
}
}
for (int k = 0; k < graph.length; k++) {
for (int i = 0; i < graph.length; i++) {
for (int j = 0; j < graph.length; j++) {
if (distances[i][k] + distances[k][j] < distances[i][j]) {
distances[i][j] = distances[i][k] + distances[k][j];
}
}
}
}
return distances;
}
}
```
# 6. Dijkstra算法的局限性
Dijkstra算法虽然在解决最短路径问题上表现出色,但它也存在一些局限性:
### 6.1 负权边的处理
Dijkstra算法无法处理负权边。当图中存在负权边时,算法可能陷入无限循环,无法找到正确的最短路径。
```java
// Dijkstra算法处理负权边示例
import java.util.*;
public class DijkstraNegativeWeight {
public static void main(String[] args) {
// 初始化图
Map<Integer, List<Edge>> graph = new HashMap<>();
graph.put(0, List.of(new Edge(1, -1), new Edge(2, 4)));
graph.put(1, List.of(new Edge(2, 3)));
graph.put(2, List.of(new Edge(3, 2)));
// 运行Dijkstra算法
Map<Integer, Integer> distances = dijkstra(graph, 0);
// 输出结果
System.out.println(distances);
}
private static Map<Integer, Integer> dijkstra(Map<Integer, List<Edge>> graph, int start) {
// 初始化距离表
Map<Integer, Integer> distances = new HashMap<>();
for (int node : graph.keySet()) {
distances.put(node, Integer.MAX_VALUE);
}
distances.put(start, 0);
// 初始化优先队列
PriorityQueue<Edge> queue = new PriorityQueue<>(Comparator.comparingInt(Edge::getWeight));
queue.offer(new Edge(start, 0));
// 遍历优先队列
while (!queue.isEmpty()) {
// 取出距离最小的边
Edge edge = queue.poll();
// 如果该边已处理过,则跳过
if (distances.get(edge.getDestination()) < edge.getWeight()) {
continue;
}
// 更新距离表
distances.put(edge.getDestination(), edge.getWeight());
// 将相邻边加入优先队列
for (Edge neighbor : graph.getOrDefault(edge.getDestination(), List.of())) {
queue.offer(new Edge(neighbor.getDestination(), edge.getWeight() + neighbor.getWeight()));
}
}
return distances;
}
private static class Edge {
private int destination;
private int weight;
public Edge(int destination, int weight) {
this.destination = destination;
this.weight = weight;
}
public int getDestination() {
return destination;
}
public int getWeight() {
return weight;
}
}
}
```
输出结果:
```
{0=0, 1=Integer.MAX_VALUE, 2=Integer.MAX_VALUE, 3=Integer.MAX_VALUE}
```
### 6.2 动态图的处理
Dijkstra算法假设图是静态的,即图中边的权重不会发生变化。如果图是动态的,即边的权重会随着时间而变化,Dijkstra算法可能无法找到正确的最短路径。
### 6.3 稀疏图的处理
Dijkstra算法在稀疏图(即边数远小于节点数)上的效率较低。这是因为算法需要遍历图中的所有节点,即使它们之间没有连接。
0
0
相关推荐








