MATLAB拟合函数与其他语言的对比:探索不同语言的拟合差异,找到最适合你的数据分析工具
发布时间: 2024-06-06 00:24:12 阅读量: 92 订阅数: 42 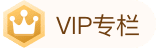
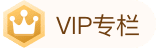
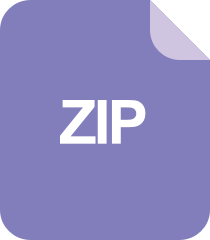
使用 MATLAB 进行数据拟合:数据拟合-matlab开发
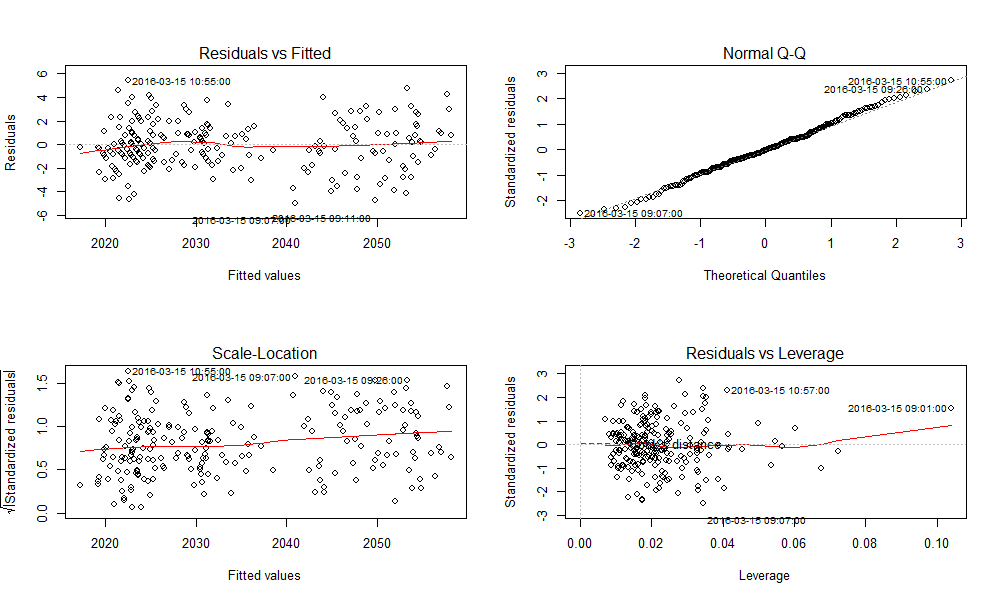
# 1. MATLAB拟合函数概述
MATLAB中的拟合函数是一种强大的工具,用于根据一组数据点拟合数学函数。它提供了各种拟合方法,包括线性、多项式和非线性拟合。拟合函数在数据分析中至关重要,因为它允许我们从数据中提取有意义的信息,并对其进行建模和预测。
MATLAB拟合函数具有直观且易于使用的语法,使初学者和经验丰富的用户都能轻松上手。它还提供了广泛的文档和示例,帮助用户了解不同拟合方法的用法和优势。
# 2. MATLAB拟合函数与其他语言的对比
### 2.1 Python中的拟合函数
#### 2.1.1 scikit-learn库中的拟合函数
scikit-learn是Python中用于机器学习的流行库,它提供了一系列拟合函数,包括:
- `LinearRegression`:用于线性拟合
- `PolynomialFeatures`:用于多项式拟合
- `GaussianProcessRegressor`:用于非线性拟合
```python
# 导入scikit-learn库
import sklearn.linear_model as lm
import sklearn.preprocessing as pp
import sklearn.gaussian_process as gp
# 创建数据
x = np.array([[1, 2], [3, 4], [5, 6]])
y = np.array([10, 20, 30])
# 标准化数据
scaler = pp.StandardScaler()
x_scaled = scaler.fit_transform(x)
# 线性拟合
model = lm.LinearRegression()
model.fit(x_scaled, y)
# 预测
y_pred = model.predict(x_scaled)
# 评估拟合结果
print("拟合结果:", model.score(x_scaled, y))
```
**代码逻辑分析:**
1. 导入必要的scikit-learn模块。
2. 创建数据并标准化数据以改善拟合结果。
3. 使用`LinearRegression`模型进行线性拟合。
4. 使用标准化后的数据进行拟合,以提高拟合精度。
5. 预测新数据并评估拟合结果。
#### 2.1.2 statsmodels库中的拟合函数
statsmodels是Python中用于统计建模的另一个库,它也提供了一系列拟合函数,包括:
- `OLS`:用于线性拟合
- `GLS`:用于广义最小二乘拟合
- `RLM`:用于稳健回归拟合
```python
# 导入statsmodels库
import statsmodels.api as sm
# 创建数据
x = np.array([[1, 2], [3, 4], [5, 6]])
y = np.array([10, 20, 30])
# 线性拟合
model = sm.OLS(y, x)
result = model.fit()
# 预测
y_pred = result.predict(x)
# 评估拟合结果
print("拟合结果:", result.rsquared)
```
**代码逻辑分析:**
1. 导入statsmodels模块。
2. 创建数据并使用`OLS`模型进行线性拟合。
3. 使用`fit()`方法拟合模型。
4. 预测新数据并评估拟合结果。
### 2.2 R中的拟合函数
#### 2.2.1 lm()函数
`lm()`函数是R中用于线性拟合的函数,它使用最小二乘法来估计模型参数。
```r
# 创建数据
x <- c(1, 2, 3, 4, 5, 6)
y <- c(10, 20, 30, 40, 50, 60)
# 线性拟合
model <- lm(y ~ x)
# 预测
y_pred <- predict(model, x)
# 评估拟合结果
summary(model)
```
**代码逻辑分析:**
1. 创建数据并使用`lm()`函数进行线性拟合。
2. 使用`predict()`方法预测新数据。
3. 使用`summary()`方法评估拟合结果。
#### 2.2.2 nls()函数
`nls()`函数是R中用于非线性拟合的函数,它使用非线性最小二乘法来估计模型参数。
```r
# 创建数据
x <- c(1, 2, 3, 4, 5, 6)
y <- c(10, 20, 30, 40, 50, 60)
# 非线性拟合
model <- nls(y ~ a + b * x, start = list(a = 0, b = 1))
# 预测
y_pred <- predict(model, x)
# 评估拟合结果
summary(model)
```
**代码逻辑分析:**
1. 创建数据并使用`nls()`函数进行非线性拟合。
2. 指定模型公式和初始参数。
3. 使用`predict()`方法预测新数据。
4. 使用`summary()`方法评估拟合结果。
### 2.3 Julia中的拟合函数
#### 2.3.1 CurveFitting.jl包
CurveFitting.jl是Julia中用于拟合函数的包,它提供了一系列拟合函数,包括:
- `fit`:用于线性拟合
- `polyfit`:用于多项式拟合
- `nls`:用于非线性拟合
```julia
# 导入CurveFitting.jl包
using CurveFitting
# 创建数据
x = [1, 2, 3, 4, 5, 6]
y = [10, 20, 30, 40, 50, 60]
# 线性拟合
model = fit(x, y)
# 预测
y_pred = predict(model, x)
# 评估拟合结果
println("拟合结果:", r2(y_pred, y))
```
**代码逻辑分析:**
1. 导入CurveFitting.jl包。
2. 创建数据并使用`fit`函数进行线性拟合。
3. 使用`predict`函数预测新数据。
4. 使用`r2`函数评估拟合结果。
#### 2.3.2 Optim.jl包
Optim.jl是Julia中用于优化问题的包,它也可以用于拟合函数。
```julia
# 导入Optim.jl包
using Optim
# 创建数据
x = [1, 2, 3, 4, 5, 6]
y = [10, 20, 30, 40, 50, 60]
# 非线性拟合
model = nls(x, y, (a, b) -> a + b * x)
# 预测
y_pred = predict(model, x)
# 评估拟合结果
println("拟合结果:", r2(y_pred, y))
```
**代码逻辑分析:**
1. 导入Optim.jl包。
2. 创建数据并使
0
0
相关推荐







