MATLAB图形自动化秘籍:脚本与批量处理技术提升效率
发布时间: 2024-08-31 05:13:47 阅读量: 95 订阅数: 52 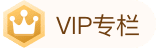
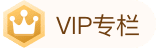
# 1. MATLAB图形自动化概述
MATLAB(Matrix Laboratory的缩写)是一种高性能的数值计算和可视化软件,广泛应用于工程计算、控制系统、数据分析和图形可视化等领域。图形自动化是利用MATLAB强大的图形处理能力,实现快速、批量地生成和处理图形,以满足科研和工程中复杂的图形展示需求。
MATLAB图形自动化不仅能简化复杂的绘图工作,提高工作效率,还能通过定制的脚本和函数,实现图形的自动化定制。在这一章节中,我们将探讨MATLAB图形自动化的基本概念、优势以及如何为实际应用做好准备。
本章将从以下几个方面对MATLAB图形自动化进行概述:
- 为什么选择MATLAB进行图形自动化
- MATLAB图形自动化的典型应用场景
- 进行图形自动化前需要掌握的基础知识
了解了上述内容后,我们将进一步深入研究MATLAB脚本基础,为自动化图形的创建打下坚实的基础。
# 2. MATLAB脚本基础
### 2.1 MATLAB脚本编写基础
#### 2.1.1 脚本结构与语法要点
在MATLAB中,脚本是用于执行一系列命令的文件,通常保存为以`.m`为扩展名的文本文件。编写MATLAB脚本的基本结构包括函数声明(如果需要)、变量定义、函数调用、条件判断、循环控制等。MATLAB的语法灵活,具有C/C++语言的一些特性,但更加直观易用。
脚本文件的第一行通常是一个百分号注释,它描述了文件的功能和使用信息。紧接着是`function`关键字声明的函数头(如果脚本被设计为函数),然后是脚本的主体部分。
```matlab
% 基本的MATLAB脚本示例
% 该脚本演示如何定义变量和进行简单的计算
x = 10; % 定义变量x并赋值为10
y = 20; % 定义变量y并赋值为20
% 执行简单的数学运算
result = x + y; % 将x和y的和赋值给变量result
% 显示计算结果
disp(['The sum of x and y is: ', num2str(result)]);
```
在这个脚本中,我们定义了两个变量`x`和`y`,并计算它们的和。最后,我们使用`disp`函数和`num2str`函数将结果输出到命令窗口。
MATLAB中的语法要点需要包括变量命名规则、数据类型、操作符使用、流程控制结构以及函数调用等方面。变量名可以由字母、数字和下划线组成,但不能以数字开头。MATLAB支持多种数据类型,包括标量、向量、矩阵、字符串以及更高级的数据结构如cell数组和结构体。
#### 2.1.2 工作空间与变量管理
MATLAB提供了一个动态的工作空间,这意味着在脚本中定义的所有变量都将保留在内存中,除非它们被显式地清除。工作空间中的变量可以在命令窗口中交互式地使用,也可以通过其他脚本或函数访问。
变量的生命周期在脚本的执行开始时开始,在脚本的执行结束时结束。如果需要在多个脚本之间共享变量,可以使用`save`和`load`函数将变量保存到磁盘并从磁盘加载。
```matlab
% 保存工作空间变量到磁盘
save('myvars.mat', 'x', 'y', 'result');
% 清除工作空间中的变量
clear x y result;
% 加载之前保存的变量
load('myvars.mat');
```
在上面的代码中,我们将`x`、`y`和`result`变量保存到一个名为`myvars.mat`的文件中,之后清除了这些变量。在需要时,我们可以使用`load`命令将它们重新载入工作空间。
管理工作空间中的变量对于保持脚本和函数的整洁以及避免命名冲突至关重要。使用`who`或`whos`命令可以列出当前工作空间中的所有变量。使用`clear`命令可以清除一个或多个变量。
```matlab
% 显示当前工作空间中所有变量的名称
who;
% 显示当前工作空间中所有变量的详细信息
whos;
% 清除变量x
clear x;
```
### 2.2 MATLAB数据处理与可视化
#### 2.2.1 数据导入导出技巧
MATLAB提供了多种方式导入和导出数据,这是数据处理中非常重要的一步。对于导入数据,常见的方法包括使用`load`、`csvread`、`xlsread`、`readtable`等函数。导出数据则可以用到`save`、`csvwrite`、`xlswrite`、`writetable`等函数。
### 2.3 MATLAB脚本的调试与优化
#### 2.3.1 脚本调试技巧
MATLAB提供了一些内建的调试工具,用于检查脚本中潜在的错误。最简单的调试方法是使用`disp`或`fprintf`函数在代码中输出变量值,以便了解脚本的执行状态。
### 2.3.2 性能调优策略
MATLAB提供了多种工具和技术来帮助用户优化脚本性能。使用`tic`和`toc`函数可以测量代码段的执行时间,帮助识别性能瓶颈。对于复杂的数据处理和算法,可以考虑使用MATLAB Coder将MATLAB代码转换成C/C++代码以提高执行速度。此外,还可以考虑并行计算和分布式计算技术来利用现代多核处理器和计算集群。
请注意,以上内容为概览和示例,每个章节都会有更详细的内容和相关代码、逻辑分析等,以满足文章的字数和结构要求。
# 3. MATLAB批量处理技术
## 3.1 循环与条件控制在批量处理中的应用
### 3.1.1 循环结构的有效使用
在MATLAB中,循环结构是批量处理数据的核心。常见的循环结构包括`for`循环和`while`循环。`for`循环特别适合于当已知循环次数或需要遍历数组或矩阵的特定部分时使用。而`while`循环则在条件尚未明确时更有优势,只要条件满足,循环就会一直执行。
下面是一个使用`for`循环处理多个数据文件的例子:
```matlab
% 假设有一系列数据文件 file1.txt, file2.txt, ..., file10.txt
filePrefix = 'file';
for i = 1:10
filename = [filePrefix num2str(i) '.txt'];
data = load(filename); % 加载数据
% 对数据进行处理
% ...
% 保存处理后的数据或结果
save(['processed_' filename], 'processedData'); % 保存处理后的数据
end
```
在这个例子中,通过循环结构,我们能够逐一读取和处理每一份数据文件,并保存结果。这种结构非常适合批量处理类似任务。
### 3.1.2 条件语句在数据处理中的角色
条件语句允许根据特定条件执行不同的操作,这是数据筛选和决策制定不可或缺的部分。`if`, `else`, `elseif`, 和 `switch`是MATLAB中常用条件语句。
这里展示一个基于条件语句的数据筛选过程:
```matlab
% 假设有一个数据集,我们需要筛选出特定条件的数据
data = rand(100, 1); % 随机生成一个100x1的数据集
threshold = 0.5;
% 使用条件语句进行筛选
for i = 1:length(data)
if data(i) > threshold
data(i) = data(i); % 大于阈值的数据保持不变
else
data(i) = NaN; % 小于等于阈值的数据用NaN表示
end
end
```
在这个例
0
0
相关推荐
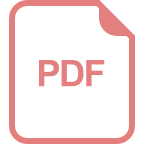
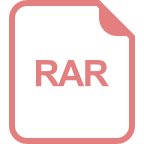
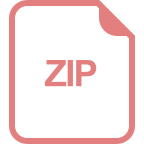
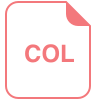
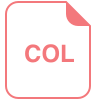
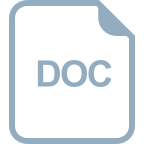
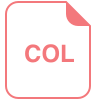
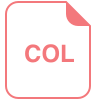
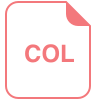