MATLAB三维图形处理完全手册:从建模到渲染的全步骤
发布时间: 2024-08-31 04:51:40 阅读量: 97 订阅数: 61 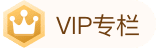
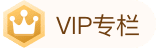
# 1. MATLAB三维图形处理概述
MATLAB作为一个强大的数学计算和图形处理工具,特别是在三维图形的处理上,提供了非常丰富的功能和方法。本章旨在向读者介绍MATLAB三维图形处理的基本概念、功能和应用,为后续章节的具体操作打下基础。
MATLAB三维图形处理不仅仅局限于静态的可视化,它还涵盖了交互式图形、动画制作、渲染技术等高级领域。我们首先会探讨三维图形处理的基本原理和技术,然后逐步深入到建模、数据处理、渲染以及高级应用实例。通过对这些内容的学习,读者将能够有效地利用MATLAB进行三维图形的创建、编辑和分析。
本章将首先从基础入手,介绍三维图形处理的基本概念和在MATLAB中的实现方式,为读者打开三维图形世界的大门。接下来的章节将带领读者深入探讨如何运用MATLAB创建复杂的三维模型,进行数据分析和优化,并最终实现高质量的图形输出和分享。让我们开始探索MATLAB三维图形处理的精彩世界吧。
# 2. 三维图形的建模基础
## 2.1 MATLAB中的三维基本图形
### 2.1.1 点、线、面的基本绘制
在MATLAB中,绘制三维图形的基本元素包括点、线和面。这些元素是构成复杂三维模型的基础。例如,点是通过其在三维空间中的坐标来定义的,线可以通过点的有序集合来表示,而面则通常由多个顶点构成的闭合多边形来定义。
要绘制一个简单的三维点,可以使用`plot3`函数:
```matlab
% 在三维空间中绘制一个点(1, 2, 3)
plot3(1, 2, 3, 'o', 'MarkerSize', 10, 'MarkerFaceColor', 'b');
```
上述代码中,`plot3`函数用于绘制三维空间中的点或线。参数`'o'`定义了点的形状,`'MarkerSize'`和`'MarkerFaceColor'`分别定义了点的大小和填充颜色。
绘制线段时,需要两个点来定义线段的起始和结束位置,例如:
```matlab
% 绘制一条连接点(1, 2, 3)和(4, 5, 6)的线段
plot3([1, 4], [2, 5], [3, 6], 'r-'); % 'r-'定义了线段的颜色为红色
```
在绘制面时,通常需要一个顶点矩阵来表示一个或多个多边形,每个多边形由顶点的顺序排列构成:
```matlab
% 定义一个四边形面的四个顶点
vertices = [1, 2, 3; 4, 5, 6; 7, 8, 9; 10, 11, 12];
% 使用patch函数绘制四边形
patch('Vertices', vertices, 'Faces', [1, 2, 3, 4], 'FaceColor', 'cyan');
```
在上述代码中,`'Vertices'`定义了顶点的坐标矩阵,`'Faces'`定义了顶点之间的连接方式,形成面的边界,而`'FaceColor'`定义了面的颜色。
### 2.1.2 坐标系统的理解和使用
MATLAB提供了多种坐标系统来支持不同的三维绘图需求,包括笛卡尔坐标系、极坐标系以及球面坐标系等。在三维图形绘制中,通常使用笛卡尔坐标系,而极坐标和球面坐标更适合于特定类型的图形展示。
笛卡尔坐标系是最基本的三维坐标系统,它通过三个互相垂直的轴来定位空间中的点:X轴、Y轴和Z轴。MATLAB默认的绘图就是使用笛卡尔坐标系。
```matlab
% 绘制多个点以观察其在笛卡尔坐标系中的位置
x = rand(5,1) * 10;
y = rand(5,1) * 10;
z = rand(5,1) * 10;
scatter3(x, y, z, 'filled');
```
在极坐标系中,点的位置由半径、角度和高度来定义。可以使用`polar3`函数将笛卡尔坐标系转换为极坐标系,从而绘制出极坐标图。
```matlab
% 在极坐标系中绘制一个点
r = 3;
theta = linspace(0, 2*pi, 100);
z = linspace(0, 1, 100);
polar3(r*cos(theta), r*sin(theta), z);
```
球面坐标系是另外一种在三维空间中描述点位置的坐标系统。它包括半径、仰角和方位角。尽管MATLAB没有直接的函数来绘制球坐标系图形,但可以通过坐标转换将球坐标转换为笛卡尔坐标系进行绘制。
```matlab
% 将球坐标系转换为笛卡尔坐标系并绘制
r = 5;
phi = linspace(0, pi, 50);
theta = linspace(0, 2*pi, 50);
[Phi, Theta] = meshgrid(phi, theta);
X = r * sin(Phi) .* cos(Theta);
Y = r * sin(Phi) .* sin(Theta);
Z = r * cos(Phi);
surf(X, Y, Z);
```
理解并掌握不同的坐标系统对于有效地创建三维图形至关重要,可以利用它们各自的优势来进行复杂的三维图形绘制。
## 2.2 MATLAB中的复合三维图形
### 2.2.1 利用patch和surface创建复杂图形
在MATLAB中,创建复杂三维图形通常需要使用`patch`和`surface`函数。这两种函数都是用来绘制三维表面,但它们有各自的适用场景和特性。
`patch`函数非常适合用来创建不规则的、由多个多边形构成的复杂图形,或者需要不同填充颜色的图形。`patch`允许用户通过定义顶点和面的连接关系来构建自定义的三维图形。
```matlab
% 创建一个由多个面构成的复杂三维图形
vertices = [1, 1, 1; 2, 1, 1; 2, 2, 1; 1, 2, 1;
1, 1, 2; 2, 1, 2; 2, 2, 2; 1, 2, 2];
faces = [1, 2, 3, 4; 5, 6, 7, 8; 1, 2, 6, 5; 2, 3, 7, 6; 3, 4, 8, 7; 4, 1, 5, 8];
patch('Faces', faces, 'Vertices', vertices, 'FaceColor', 'y');
```
在这段代码中,`'Faces'`指定了顶点构成面的方式,每行代表一个面,列中的数字是顶点在`'Vertices'`中的索引。`'FaceColor'`则是每个面的颜色设置。
相对而言,`surface`函数通常用于创建规则的、连续的曲面,例如基于数据矩阵的等高线图。`surface`适合于表现连续的数据变化和曲面的光滑过渡。
```matlab
% 创建一个基于数据的曲面图
[X, Y] = meshgrid(-2:0.1:2, -2:0.1:2);
Z = X .* exp(-X.^2 - Y.^2);
surface(X, Y, Z);
```
在上述代码中,`meshgrid`函数生成了X和Y的坐标矩阵,这些矩阵被用来计算每个点的Z坐标,形成一个基于数据的曲面。
### 2.2.2 图形属性的定制和优化
为了增强三维图形的表现力和信息传递效率,需要对图形属性进行定制和优化。在MATLAB中,可以调整线宽、颜色、表面透明度等多种属性。
例如,可以增加线宽以突出图形的边界:
```matlab
% 在之前创建的曲面图形基础上增加线宽
surface(X, Y, Z);
set(gca, 'linewidth', 2); % gca获取当前坐标轴,'linewidth'设置线宽
```
颜色的调整有助于区分不同的图形部分或增强视觉效果。可以使用`colormap`函数来设置图形的颜色映射。
```matlab
% 设置颜色映射并调整透明度
colormap(jet(256)); % 使用jet颜色映射
alpha(0.7); % 设置透明度为0.7,其中1为完全不透明,0为完全透明
```
表面的光照效果也会使得图形看起来更加立体和真实。MATLAB提供了多种光照效果,如`light`、`lighting`、`diffuse`、`specular`和`material`等函数。
```matlab
% 增加光照效果和材质属性
light('Position', [10, 10, 10]);
lighting phong;
diffuse(0.7);
specular(0.5);
material shiny;
```
以上示例中,`light`函数定义了光源的位置,`lighting phong`和`material shiny`设置了光照和材质属性,而`diffuse`和`specular`则分别定义了漫反射和镜面反射的强度。
通过自定义和优化三维图形属性,可以更好地展现数据的内在特征和结构,提高图形的可读性和美观性。
## 2.3 MATLAB中的三维建模工具
### 2.3.1 PLOT3D和Meshgrid的高级应用
MATLAB中的`plot3d`函数和`meshgrid`函数是三维图形绘制和建模的重要工具。`plot3d`可以直接绘制三维点和线,而`meshgrid`在处理三维曲面图形时尤为关键,它能够生成用于定义三维网格的坐标矩阵。
`plot3d`函数用于绘制三维空间中的点集或线段。在进行复杂三维图形绘制时,`plot3d`能够通过其丰富的选项来满足不同的视觉需求。
```matlab
% 使用plot3d绘制三维空间中的多个点
x = [1, 2, 3, 4, 5];
y = [2, 3, 4, 5, 6];
z = [1,
```
0
0
相关推荐








