Common Methods for Numerical Solution of Ordinary Differential Equations
发布时间: 2024-09-14 22:49:47 阅读量: 23 订阅数: 18 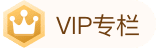
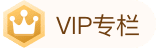
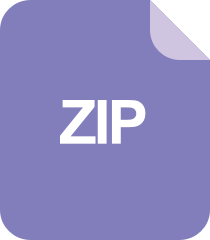
SUMS50 Numerical Methods for Ordinary Differential Equations.zip
# 1. Overview of Ordinary Differential Equations
## Definition of Ordinary Differential Equations
An ordinary differential equation is an equation that describes the relationship between the derivative(s) of an unknown function with respect to a single independent variable and the function itself. It is a fundamental theory in mathematics, physics, engineering, and other fields, often used to describe the laws of change in natural phenomena. Ordinary differential equations are commonly used to establish mathematical models, allowing predictions or explanations of various phenomena through equation solving.
## Classification of Ordinary Differential Equations
Depending on characteristics such as order, linearity, coefficient type, and equation type, ordinary differential equations can be classified into various types, including first-order equations, higher-order equations, linear equations, nonlinear equations, constant coefficient equations, and variable coefficient equations.
## Applications of Ordinary Differential Equations
Ordinary differential equations are widely applied in physics, engineering, biology, economics, and other fields. For example, Newton's second law can be expressed as an ordinary differential equation, and the change in current in a circuit can also be described by an ordinary differential equation. In biology, phenomena such as population growth and species competition can be modeled and predicted using ordinary differential equations.
# 2. Overview of Numerical Solution Methods
Numerical solution methods are an essential part of numerical solutions for ordinary differential equations. This chapter will introduce the basic principles, classifications, and advantages and disadvantages of numerical methods.
### Basic Principles of Numerical Methods
The basic principle of numerical methods is to transform continuous ordinary differential equations into discrete systems of equations and solve them approximately using computers. The core idea is to discretize continuous variables such as time and space, transforming the problem into calculations at a finite number of discrete points.
### Classification of Numerical Solution Methods
Depending on their basic principles and operational methods, numerical solution methods can be divided into several categories:
1. **Single-step methods**: ***mon single-step methods include the Euler method and the modified Euler method.
2. **Multistep methods**: ***mon multistep methods include the Adams-Bashforth method and the Adams-Moulton method.
3. **Runge-Kutta methods**: These methods use information from multiple intermediate points to calculate the value of the next point, achieving higher accuracy and stability.
4. **Adaptive step size methods**: These methods automatically adjust the step size based on the current solution's accuracy and stability, improving the efficiency and accuracy of the solution.
### Advantages and Disadvantages of Numerical Methods
Numerical solution methods have the following advantages:
- **Flexibility**: Numerical methods can be applied to various types of ordinary differential equations and can be adjusted and optimized according to specific problems.
- **Reliability**: Numerical methods have been extensively researched and practiced over the years and have been widely applied and verified, ensuring high reliability.
- **Efficiency**: Numerical methods can efficiently solve problems using computers, significantly reducing the time required for solutions.
Numerical solution methods also have some disadvantages:
- **Accumulation of errors**: Numerical methods introduce certain errors in each iteration, and as the number of iterations increases, these errors can accumulate, affecting the accuracy of the results.
- **Boundary problems**: Some numerical methods are not flexible enough in handling boundary problems, potentially leading to inaccurate solutions.
- **Convergence issues**: Some numerical methods may experience convergence problems under certain conditions, requiring careful selection and adjustment of parameters.
In summary, numerical solution methods are an important part of numerical solutions for ordinary differential equations. Different numerical methods have different applications and characteristics, requiring selection and optimization based on specific problems. In practical applications, it is necessary to consider factors such as solution accuracy, computational efficiency, and stability to choose the appropriate numerical solution method.
# 3. Euler's Method
Euler's method is a commonly used numerical solution method for ordinary differential equations, based on the principle of discretizing differential equations. The following will introduce the algorithm, stability, and convergence analysis of Euler's method.
#### Principles of Euler's Method
The principle of Euler's method is to replace the derivative in the differential equation with a finite difference, transforming the differential equation into a recursive iterative format. For a first-order ordinary differential equation dy/dx = f(x, y), Euler's method can be used to approximate the solution. First, discretize the range of the independent variable x, then iterate using the formula y_{n+1} = y_n + h*f(x_n, y_n), where h is the step size and n is the number of iterations. This allows for the step-by-step calculation of the differential equation's solution.
#### Algorithm of Euler's Method
The Euler method's algorithm can be simply described as follows:
```
1. Initialize the initial values of the independent variable x_0 and the dependent variable y_0.
2. Set the step size h.
3. For each iteration step n:
- Calculate the next independent variable value x_{n+1} = x_n + h.
- Calculate the next dependent variable value y_{n+1} = y_n + h*f(x_n, y_n).
4. Obtain the sequence of numerical solutions {(x_0, y_0), (x_1, y_1), (x_2, y_2), ...}.
```
#### Stability and Convergence Analysis of Euler's Method
The stability and convergence of Euler's method depend on the choice of step size h. Typically, Euler's method is unstable, especially when dealing with stiff equations or higher-order equations. Moreover, Euler's method has poor convergence properties and may require an extremely small step size to achieve accurate numerical solutions. Therefore, Euler's method often needs to be combined with adaptive step size techniques in practical applications to improve the accuracy and stability of numerical solutions.
I hope this provides a preliminary understanding of Euler's method.
# 4. Improved Euler's Method
### Principles of Improved Euler's Method
The improved Euler's method is a commonly used numerical method for solving ordinary differential equations, representing an improvement and optimization of the Euler method. The improved Euler's method uses a more accurate approximation method to calculate the value of the next step, thereby increasing the accuracy of the numerical solution.
### Algorithm of Improved Euler's Method
The basic algorithm for the improved Euler's method is as follows:
1. Calculate the initial values based on the initial conditions.
2. For each step, use the following formula to calculate the value of the next step:
`y[i+1] = y[i] + h * f(x[i] + h/2, y[i] + (h/2) * f(x[i], y[i]))`
Where h is the step size, x[i] and y[i] are the values of the current step, and f is the derivative function of the equation.
3. Repeat step 2 until reaching the specified termination condition.
### Example Application of Improved Euler's Method
Below is a simple example of using the improved Euler's method to solve an ordinary differential equation.
```python
import math
def f(x, y):
return x**2 + y
def improved_euler_method(h, x0, y0, xn):
num_steps = int((xn - x0) / h)
x_values = [x0]
y_values = [y0]
for i in range(num_steps):
x = x_values[i]
y = y_values[i]
y_mid = y + (h/2) * f(x, y)
x_next = x + h
y_next = y + h * f(x + h/2, y_mid)
x_values.append(x_next)
y_values.append(y_next)
return x_values, y_values
# Setting initial conditions and step size
h = 0.1
x0 = 0
y0 = 1
xn = 1
# Solving using the improved Euler's method
x_values, y_values = improved_euler_method(h, x0, y0, xn)
# Outputting results
for i in range(len(x_values)):
print(f"x = {x_values[i]}, y = {y_values[i]}")
```
**Code Explanation:**
- First, a derivative function f(x, y) is defined to calculate the derivative of the equation.
- Then, the improved Euler's method function improved_euler_method is used to solve the problem, inputting the step size h, initial values x0, y0, and termination value xn.
- In the function, the required number of steps num_steps is first calculated, and then the value of each step is computed through a loop. In each step, the intermediate value y_mid of y is calculated using the improved Euler's method formula, and the next step's value y_next is calculated using this intermediate value. Finally, all step x and y values are stored in x_values and y_values lists and returned as results.
- Lastly, in the main program, the improved Euler's method function is called with the initial conditions and step size, and the results are printed.
**Result Explanation:**
This example calculates the results of the improved Euler's method over the interval [0, 1] with a step size of 0.1. Each line of the output shows the x and y values for a step, and it can be observed that as the steps increase, the y values gradually approach the accurate solution.
# 5. Runge-Kutta Method
Among the methods for numerically solving ordinary differential equations, the Runge-Kutta method is a very commonly used one. It approximates the true solution by converting differential equations into difference equations.
#### Principles of the Runge-Kutta Method
The basic principle of the Runge-Kutta method is to use Taylor series expansions to approximate the solution of differential equations. By employing different weighting coefficients, Runge-Kutta methods of varying orders can be obtained.
#### Algorithm of the Runge-Kutta Method
Here is the algorithm for a commonly used fourth-order Runge-Kutta method:
```python
def runge_kutta(f, h, t0, T, y0):
n = int((T - t0) / h)
t = [t0]
y = [y0]
for i in range(n):
k1 = f(t[i], y[i])
k2 = f(t[i] + h/2, y[i] + h/2 * k1)
k3 = f(t[i] + h/2, y[i] + h/2 * k2)
k4 = f(t[i] + h, y[i] + h * k3)
y_next = y[i] + h/6 * (k1 + 2*k2 + 2*k3 + k4)
y.append(y_next)
t_next = t[i] + h
t.append(t_next)
return t, y
```
#### Higher-order Forms of the Runge-Kutta Method
In addition to the fourth-order Runge-Kutta method, there are higher-order forms such as the fifth and sixth-order Runge-Kutta methods.
Higher-order Runge-Kutta methods can approximate the solutions of differential equations more accurately, but the computational effort will also increase accordingly. Therefore, in practical applications, there must be a trade-off between accuracy and computational efficiency.
This concludes the discussion on the Runge-Kutta method, which is one of the common methods for numerically solving ordinary differential equations. By mastering the Runge-Kutta method, one can more flexibly handle various problems of solving ordinary differential equations and obtain more accurate numerical solutions.
# 6. Other Numerical Solution Methods
In the process of numerically solving ordinary differential equations, apart from the Euler and Runge-Kutta methods, there are other numerical solution methods. These methods can be selected and used based on the needs of actual problems and the characteristics of numerical computation.
### Runge-Kutta Methods
The Runge-Kutta method (a variant of the Runge-Kutta method) ***pared to the Euler method, it offers higher precision and stability. The Runge-Kutta method usually approaches the exact solution through iterative calculations.
Below is a classic example of a fourth-order Runge-Kutta method implemented in Python:
```python
def runge_kutta(f, x0, y0, h, n):
"""
Function for solving ordinary differential equations using the Runge-Kutta method.
:param f: The right-hand side function of the differential equation.
:param x0: Initial value of the independent variable.
:param y0: Initial value of the dependent variable.
:param h: Step size.
:param n: Number of iterations.
:return: The list of results after iteration.
"""
result = [y0]
for i in range(n):
k1 = h * f(x0 + i * h, result[i])
k2 = h * f(x0 + i * h + h/2, result[i] + k1/2)
k3 = h * f(x0 + i * h + h/2, result[i] + k2/2)
k4 = h * f(x0 + i * h + h, result[i] + k3)
y_next = result[i] + (k1 + 2*k2 + 2*k3 + k4) / 6
result.append(y_next)
return result
```
In the code, the `runge_kutta` function takes a function `f` as a parameter, representing the right-hand side function of the differential equation to be solved. Then, through iterative calculation, it computes the values of the dependent variable for each time step one by one. Finally, all the computed results are stored in a list and returned.
### Multistep Methods
Multistep methods are numerical solution methods that rely on historical data to predict the current solution. Multistep methods usually require multiple initial values, so during iterative calculations, it is necessary to compute the values of the dependent variable for each time step in sequence.
Below is a classic example of the Adams-Bashforth predictor-corrector fourth-order method (consisting of two three-order formulas) implemented in Python:
```python
def adams_bashforth_moulton(f, x0, y0, h, n):
"""
Function for solving ordinary differential equations using the Adams-Bashforth predictor-corrector method.
:param f: The right-hand side function of the differential equation.
:param x0: Initial value of the independent variable.
:param y0: Initial value of the dependent variable.
:param h: Step size.
:param n: Number of iterations.
:return: The list of results after iteration.
"""
result = [y0]
for i in range(n):
# Prediction
p1 = f(x0 + i*h, result[i])
p2 = f(x0 + (i-1)*h, result[i-1])
p3 = f(x0 + (i-2)*h, result[i-2])
y_predict = result[i] + h * (55*p1 - 59*p2 + 37*p3 - 9*f(x0 + (i-3)*h, result[i-3])) / 24
# Correction
c1 = f(x0 + (i+1)*h, y_predict)
c2 = f(x0 + i*h, result[i])
c3 = f(x0 + (i-1)*h, result[i-1])
c4 = f(x0 + (i-2)*h, result[i-2])
y_corrected = result[i] + h * (9*c1 + 19*c2 - 5*c3 + c4) / 24
result.append(y_corrected)
return result
```
In the code, the `adams_bashforth_moulton` function uses the predictor-corrector formula of the Adams-Bashforth method, iteratively computing and correcting the values of the dependent variable for each time step one by one.
### Adaptive Step Size Methods
Adaptive step size methods are numerical solution methods that automatically adjust the step size as needed. During the solving process, adaptive step size methods will automatically choose the size of the next time step based on the magnitude of the numerical error, ensuring the accuracy and stability of the numerical solution.
Below is an example of an adaptive step size improved Euler method implemented in Python:
```python
def adaptive_euler(f, x0, y0, h, tol, max_iter):
"""
Function for solving ordinary differential equations using the adaptive step size improved Euler method.
:param f: The right-hand side function of the differential equation.
:param x0: Initial value of the independent variable.
:param y0: Initial value of the dependent variable.
:param h: Initial step size.
:param tol: Target accuracy.
:param max_iter: Maximum number of iterations.
:return: The list of results after iteration.
"""
result = [y0]
x = x0
y = y0
h_actual = h
iter_count = 0
while iter_count < max_iter:
delta1 = h_actual * f(x, y)
delta2 = h_actual * f(x + h_actual, y + delta1)
error = abs(delta2 - delta1)
if error <= tol:
y_next = y + delta2
result.append(y_next)
x += h_actual
y = y_next
iter_count += 1
h_actual = h * (tol / error) ** 0.5
return result
```
In the code, the `adaptive_euler` function automatically adjusts the step size based on error control criteria to ensure the accuracy of the numerical solution. In each iteration, if the error is less than the target accuracy, the result calculated with the current step size is accepted; otherwise, the step size is reduced, and iteration continues.
These are examples of other commonly used numerical solution methods. Depending on the needs of actual problems and the characteristics of numerical computation, suitable numerical solution methods can be selected to solve ordinary differential equations.
0
0
相关推荐
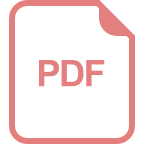
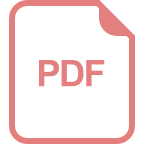
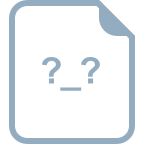
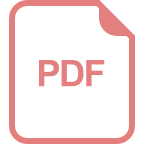
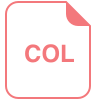
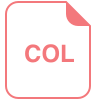
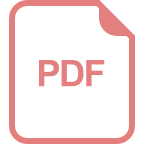
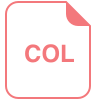