Discretization Methods and Numerical Simulation
发布时间: 2024-09-14 22:51:36 阅读量: 14 订阅数: 14 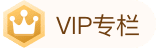
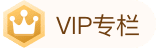
# Introduction
## Background and Research Significance
In scientific research and engineering practice, numerical simulation and computation of various physical phenomena are often required. Numerical simulation transforms real-world continuous problems into discrete numerical ones through mathematical models and discretization methods, and then solves them using computer algorithms. The applications of numerical simulation are extensive, covering multiple fields such as fluid dynamics, structural mechanics, electromagnetism, and more.
Discretization methods are an important part of numerical simulation, converting continuous physical problems into discrete mathematical ones. By discretizing physical problems in space, they can be transformed into a series of algebraic equations, thereby allowing for computer solutions. The choice and use of discretization methods directly affect the accuracy and efficiency of numerical simulations. Therefore, researching and applying appropriate discretization methods are crucial for improving the accuracy and computational efficiency of numerical simulations.
## Article Structure Overview
This article will introduce the principles and applications of discretization methods in numerical simulation. First, we will briefly introduce the basic concepts and common methods of discretization, including finite difference, finite element, and finite volume methods. Then, we will discuss the fundamental principles of numerical simulation, including the concept, basic steps, and error and accuracy control. Next, we will elaborate on the specific applications of discretization methods in numerical simulation, including fields such as fluid dynamics, structural mechanics, and electromagnetism. Additionally, this article will present case studies demonstrating the applications of discretization methods in different fields. Finally, we will summarize the relationship between discretization methods and numerical simulation, look forward to the development trends of discretization methods, and propose prospects for future numerical simulation research.
Upon reading this article, readers will understand the importance and application value of discretization methods in numerical simulation, grasp the basic principles and steps of numerical simulation, become aware of the applications of discretization methods in different fields, and gain insights and prospects for future numerical simulation research.
# Discretization Methods Introduction
Discretization methods are mathematical approaches that transform continuous problems into discrete ones. In numerical simulation, discretization methods are often used for numerical calculations of continuous physical phenomena. This chapter will introduce the basic concepts and common applications of discretization methods.
### What is a Discretization Method
A discretization method is a common strategy in numerical computation, dividing the continuous spatial or temporal domain into discrete units, thereby transforming a continuous problem into a discrete one. By performing numerical calculations on these discrete units, we can approximate the solution to the continuous problem and obtain a numerical solution for the system.
### Common Discretization Methods
Among discretization methods, common approaches include the finite difference, finite element, and finite volume methods. These methods are applicable to different types of physical phenomena and mathematical models, each with its own advantages and disadvantages.
#### Finite Difference Method
The finite difference method is a technique for converting differential equations into difference equations. It divides the solution area into discrete grid points and uses difference approximations to replace differential operations. By constructing a system of difference equations, we can compute the numerical solution at each grid point. The finite difference method is suitable for one-dimensional and two-dimensional partial differential equations and is often applied in fluid dynamics, heat conduction, and other fields.
```python
# Example of a one-dimensional heat conduction equation, discretized using the finite difference method
import numpy as np
# Define the grid
Nx = 10 # Number of grid points
L = 1.0 # Length of the domain
dx = L / (Nx - 1) # Grid spacing
x = np.linspace(0, L, Nx)
# Initialize the temperature field
T = np.zeros(Nx)
T[0] = 100 # Left boundary temperature
T[-1] = 0 # Right boundary temperature
# Compute the difference equation
for i in range(1, Nx-1):
T[i] = T[i] + (T[i-1] - 2*T[i] + T[i+1]) * dt / dx**2
print(T)
```
In the above code, we use the finite difference method to discretize a one-dimensional heat conduction equation and compute the temperature at each grid point.
#### Finite Element Method
The finite element method is a technique for converting continuous problems into discrete ones, widely used in structural mechanics, earthquake dynamics, and other fields. The finite element method divides the solution area into discrete finite elements and approximates the solution using basis functions. By integrating and approximating the equations within the finite elements, we can obtain a discrete system of equations, and by solving this system, we can find the numerical solution.
```python
# Example of a two-dimensional elasticity problem, discretized using the finite element method
import numpy as np
from scipy.sparse import coo_matrix
# Define the grid and elements
Nx = 10 # Number of grid points in the x-axis
Ny = 10 # Number of grid points in the y-axis
Lx = 1.0 # Length of the x-axis domain
Ly = 1.0 # Length of the y-axis domain
dx = Lx / (Nx - 1) # Grid spacing in the x-axis
dy = Ly / (Ny - 1) # Grid spacing in the y-axis
x = np.linspace(0, Lx, Nx)
y = np.linspace(0, Ly, Ny)
# Create numbering for finite elements and nodes
n_nodes = Nx * Ny # Total number of nodes
n_elements = (Nx - 1) * (Ny - 1) # Total number of elements
node_nums = np.arange(n_nodes).reshape((Nx, Ny))
element_nums = np.arange(n_elements).reshape((Nx - 1, Ny - 1))
# Construct the stiffness matrix and load vector
K = coo_matrix((n_nodes, n_nodes))
F = np.zeros(n_nodes)
# Iterate over each element to construct the stiffness matrix and load vector
for i in range(Nx - 1):
for j in range(Ny - 1):
element_nodes = [node_nums[i, j], node_nums[i+1, j], node_nums[i, j+1], node_nums[i+1, j+1]]
# Construct the element stiffness matrix and load vector
Ke, Fe = element_stiffness(element_nodes)
# Add the element stiffness matrix and load vector to the overall stiffness matrix and load vector
for m in range(4):
for n in range(4):
K[element_nodes[m], element_nodes[n]] += Ke[m, n]
F[element_nodes[m]] += Fe[m]
# Solve the linear system
U = np.linalg.solve(K, F)
print(U)
```
In the above code, we use the finite element method to discretize a two-dimensional elasticity problem and compute the displacement at each node.
#### Finite Volume Method
The finite volume method is a discretization method based on control volumes, widely used in fluid dynamics and heat and mass transfer. The finite volume method divides the solution area into discrete control volumes and integrates the physical quantities within the control volumes using conservation equations such as mass conservation and momentum conservation. By solving the discrete conservation equation system, we can obtain the numerical solution.
```python
# Example of a two-dimensional convection-diffusion equation, discretized using the finite volume method
import numpy as np
# Define the grid and elements
Nx = 10 # Number of grid points in the x-axis
Ny = 10 # Number of grid points in the y-axis
Lx = 1.0 # Length of the domain
Ly = 1.0 # Length of the y-axis domain
dx = Lx / (Nx - 1) # Grid spacing in the x-axis
dy = Ly / (Ny - 1) # Grid spacing in the y-axis
x = np.linspace(0, Lx, Nx)
y = np.linspace(0, Ly, Ny)
# Create numbering for control volumes and nodes
n_nodes = Nx * Ny # Total number of nodes
n_cells = (Nx - 2) * (Ny - 2) # Total number of control volumes
node_nums = np.arange(n_nodes).reshape((Nx, Ny))
cell_nums = np.arange(n_cells).reshape((Nx - 2, Ny - 2))
# Initialize physical quantities
phi = np.zeros((Nx, Ny))
phi[0, :] = 1.0 # Left boundary set to 1
# Define parameters
alpha = 0.01 # Diffusion coefficient
u = 1.0 # Velocity in the x-direction
# Iterative solution
for k in range(100):
phi_new = np.zeros((Nx, Ny))
for i in range(1, Nx - 1):
for j in range(1, Ny - 1):
# Calculate diffusion flux within the control volume
flux_x = -alpha * (phi[i, j] - phi[i-1, j]) / dx
flux_y = -alpha * (phi[i, j] - phi[i, j-1]) / dy
# Calculate convection flux within the control volume
conv_flux_x = u * phi[i, j]
conv_flux_y = u * phi[i, j]
# Calculate the source term within the control volume
source = 0.0
# Update physical quantities
phi_new[i, j] = phi[i, j] + (flux_x - flux_x + conv_flux_x - conv_flux_y + source) * dt / (dx * dy)
phi = phi_new
print(phi)
```
In the above code, we use the finite volume method to discretize a two-dimensional convection-diffusion equation and calculate the physical quantities within each control volume.
With this introduction, we understand the basic concepts and common methods of discretization methods. In numerical simulation, choosing the appropriate discretization method to discretize the problem is a very important step. In the following chapters, we will introduce the basic principles of numerical simulation and the applications of discretization methods in different fields.
# Fundamental Principles of Numerical Simulation
Numerical simulation is a method of simulating and solving real-world problems using a computer. It transforms real-world problems into mathematical models and uses discretization methods to process these models, followed by numerical algorithms for computation. This chapter will introduce the basic principles of numerical simulation, including the concept of numerical simulation, basic steps, and error and accuracy control.
#### Concept of Numerical Simulation
Numerical simulation is the process of using a computer to find numerical solutions to real-world problems. It constructs mathematical models by turning real-world problems into mathematical problems, and then obtains approximate solutions through numerical calculations. Numerical simulation can be applied to various scientific and engineering fields, such as fluid dynamics, structural mechanics, electromagnetism, and more.
#### Basic Steps of Numerical Simulation
The basic steps of numerical simulation include establishing a mathematical model, selecting an appropriate discretization method, writing numerical algorithms, and performing numerical calculations.
##### Establishing a Mathematical Model
Establishing a mathematical model is the first step in numerical simulation. A mathematical model is the mathematical form that abstracts and describes real-world problems. When establishing a mathematical model, it is necessary to consider the physical and mathematical characteristics of the problem and choose the appropriate mathematical equations or systems to describe it.
##### Selecting an Appropriate Discretization Method
Discretization methods are used to discretize the mathematical model. Discretization methods can be divided into finite difference, finite element, and finite volume methods, among others. When selecting a discretization method, it is necessary to consider the characteristics of the problem and the requirements of numerical computation.
##### Writing Numerical Algorithms
Numerical algorithms are used to compute the discretized model. Depending on the choice of discretization method, corresponding numerical algorithms can be written. Numerical algorithms need to consider computational stability, convergence, and computational efficiency.
##### Performing Numerical Calculations
Performing numerical calculations is the final step in numerical simulation. By inputting the mathematical model and numerical algorithms into a computer, numerical calculations can obtain an approximate solution to the problem. The results of numerical calculations can be displayed and analyzed using visualization methods.
#### Error and Accuracy Control in Numerical Simulation
The results of numerical simulation differ from real-world problems. These errors come from the simplification of mathematical models, the approximation of discretization methods, and the precision of computer calculations. To control errors and improve computational accuracy, ***mon methods of error control include mesh convergence analysis, time step control, and numerical format improvement.
This chapter introduces the basic principles of numerical simulation, including the concept of numerical simulation, basic steps, and error and accuracy control. The next chapter will introduce the applications of discretization methods in numerical simulation.
# Applications of Discretization Methods in Numerical Simulation
Discretization methods are a key step in numerical simulation, transforming continuous problems into discrete forms so that computers can process them. In numerical simulation, discretization methods are widely applied, involving multiple fields such as fluid dynamics, structural mechanics, and electromagnetism. The following will introduce the applications of discretization methods in these fields.
#### Discretization Methods in Fluid Dynamics
In fluid dynamics, discretization methods are often used to describe the motion state of fluids and the distribution of flow fields. Finite difference, finite element, and finite volume methods are common discretization methods suitable for different types of fluid dynamics problems. These methods divide the fluid domain into discrete units, using numerical approximation techniques, can simulate and analyze fluid dynamics problems, such as fluid velocity fields, pressure field distribution, and fluid dynamics characteristic parameters.
#### Discretization Methods in Structural Mechanics
In structural mechanics, discretization methods are often used to describe the forces and deformations of structures. The finite element method is one of the most common discretization methods in structural mechanics. It divides the structure into discrete finite elements, using the principle of numerical approximation, and can analyze and solve structural stress, strain, and vibration characteristics. The application of discretization methods in structural mechanics enables engineers to more accurately assess the stability, safety, and reliability of structures.
#### Discretization Methods in Electromagnetism
In the field of electromagnetism, discretization methods are often used to describe the distribution of electromagnetic fields and electromagnetic properties. Finite difference and finite element methods are commonly used discretization methods in electromagnetism, which can be used to simulate and analyze the propagation of electromagnetic waves, the distribution of electromagnetic fields, and the interaction of electromagnetic fields with media. The application of these methods enables engineers and researchers to delve deeper into understanding electromagnetic phenomena and to design more sophisticated electromagnetic devices and systems.
The above is the application of discretization methods in numerical simulation, providing numerical solutions to problems in different fields, and promoting progress in engineering technology and scientific research.
# Case Studies in Numerical Simulation
In this chapter, we will demonstrate the applications of discretization methods in numerical simulation through specific case studies, including applications in fluid dynamics, structural mechanics, and electromagnetism. Through these case studies, we can more intuitively understand the practical applications and effects of discretization methods in different fields.
#### Fluid Dynamics Case Study
We will introduce a common fluid dynamics case, such as the numerical simulation of fluid flow using the finite volume method, including establishing a fluid dynamics model, selecting discretization methods, writing numerical algorithms, performing numerical calculations, and analyzing results. Through this case study, readers can understand the specific applications of discretization methods in the field of fluid dynamics.
#### Structural Mechanics Case Study
We will take a typical structural mechanics case as an example to introduce the application of discretization methods in structural mechanics. By using the finite element method for numerical simulation of a structure, including establishing a structural mechanics model, selecting the appropriate discretization method, writing numerical algorithms, performing numerical calculations, and analyzing results. Readers can understand the specific applications of discretization methods in structural mechanics through this case study.
#### Electromagnetism Case Study
Finally, we will introduce an electromagnetism case, such as the numerical simulation of electromagnetic fields using the finite difference method, including establishing an electromagnetism model, selecting discretization methods, writing numerical algorithms, performing numerical calculations, and analyzing results. Through this case study, readers can understand the specific applications of discretization methods in the field of electromagnetism.
Through the above case studies, we can fully understand the applications of discretization methods in numerical simulations across different fields and more intuitively understand the practical effects of discretization methods in different fields.
# Conclusion and Prospects
In this article, we systematically introduced the applications of discretization methods in numerical simulation. Through an in-depth discussion of the introduction to discretization methods, the fundamental principles of numerical simulation, and the specific applications of discretization methods in numerical simulation, we can draw the following conclusions and prospects.
#### Summary of the Relationship Between Discretization Methods and Numerical Simulation
Discretization methods are the foundation of numerical simulation, transforming continuous mathematical models into discrete forms, making numerical solutions to real-world problems possible. Different types of numerical simulation problems require appropriate discretization methods, such as finite volume methods in fluid dynamics, finite element methods in structural mechanics, and finite difference methods in electromagnetism. Through the selection and application of discretization methods, we can better understand and solve complex physical phenomena, providing technical support for engineering and scientific fields.
#### Development Trends of Discretization Methods
With the continuous advancement of computer technology and numerical computation methods, discretization methods are also evolving. The future development trends of discretization methods are mainly reflected in the following aspects:
1. **Multiphysics Field Coupling Simulation**: Coupling simulations of different physical fields, such as fluid-structure interaction and electrothermal coupling, require more complex discretization methods to handle these coupled problems.
2. **Adaptive Mesh Technology**: To improve computational efficiency and accuracy, adaptive mesh technology will become a focus of discretization method development, capable of automatically adjusting mesh density and structure based on computational results.
3. **High-Performance Computing and Parallel Computing**: With the development of supercomputers, discretization methods need to better integrate with high-performance computing and parallel computing to address larger-scale, more complex numerical simulation problems.
#### Prospects for Future Numerical Simulation Research
In the future, with the continuous innovation and development of discretization methods and numerical simulation technology, we can look forward to progress in the following areas:
1. **More Accurate Simulation Results**: By continuously optimizing discretization methods and algorithms, more accurate simulation results closer to real-world physical phenomena can be obtained.
2. **Wider Application Fields**: Discretization methods will further expand into fields such as biomedical engineering and environmental engineering, providing more comprehensive technical support for social development and scientific research.
3. **Intelligentization and Automation**: With the development of artificial intelligence technology, discretization methods and numerical simulation will become more intelligent and automated, providing users with more convenient and efficient numerical simulation services.
0
0
相关推荐
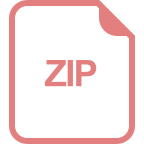
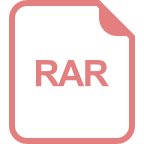
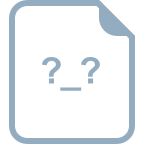
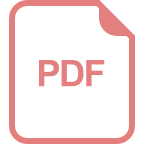
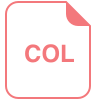
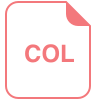
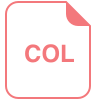
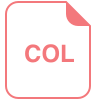
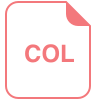