Three Solution Methods for Inverse Problems of Partial Differential Equations: Backward Estimation of Unknown Parameters from Observational Data
发布时间: 2024-09-14 09:05:00 阅读量: 17 订阅数: 23 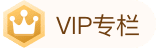
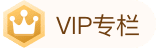
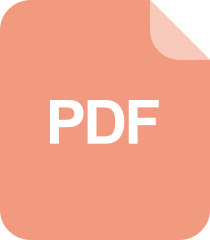
Restricted Gene Expression Programming: A New Approach for Parameter Identification Inverse Problems of Partial Differential Equation(CI, EI, 2区IF=2.784)
# An Overview of Three Solution Methods for Inverse Problems in Partial Differential Equations: Inferring Unknown Parameters from Observational Data
Partial Differential Equation (PDE) inverse problems involve deducing the unknown solutions of PDEs based on observational data. PDE inverse problems are widely applied in fields such as image processing, medical imaging, and fluid dynamics.
The key to solving PDE inverse problems lies in linking observational data with PDE models. This typically involves solving an inversion operator that maps observational data to PDE solutions. Inversion operators are often highly nonlinear, making PDE inverse problems challenging.
The main approaches to solving PDE inverse problems can be categorized into three types: optimization-based methods, variational methods, and stochastic methods. Optimization methods solve PDEs by iteratively minimizing an objective function. Variational methods transform PDE inverse problems into variational problems and obtain PDE solutions by solving variational equations. Stochastic methods use random sampling to approximate PDE solutions.
## 2. Solutions Based on Optimization Methods
### 2.1 Gradient Descent Method
#### 2.1.1 Basic Principles
The gradient descent method is an iterative optimization algorithm used to find local minima of functions. The basic idea is to iteratively update the current point in the negative direction of the function gradient until a local minimum is reached.
#### 2.1.2 Algorithm Flow and Implementation
The algorithm flow of gradient descent is as follows:
1. Initialize parameters: learning rate α, maximum number of iterations N, current point x0.
2. Iterative update:
- Compute the function gradient: ∇f(xn)
- Update the current point: xn+1 = xn - α∇f(xn)
3. Determine the termination condition:
- Maximum number of iterations N reached
- Gradient approaches zero: ‖∇f(xn)‖ < ε
```python
import numpy as np
def gradient_descent(f, x0, alpha=0.01, N=1000, epsilon=1e-6):
"""Solve for local minima using gradient descent
Args:
f: Objective function
x0: Initial point
alpha: Learning rate
N: Maximum number of iterations
epsilon: Termination condition threshold
Returns:
Local minimum
"""
x = x0
for i in range(N):
grad = np.nabla(f, x) # Compute function gradient
x -= alpha * grad # Update current point
if np.linalg.norm(grad) < epsilon: # Check termination condition
break
return x
```
### 2.2 Conjugate Gradient Method
#### 2.2.1 Basic Principles
The conjugate gradient method is an improved gradient descent method that introduces conjugate directions to accelerate convergence. Conjugate directions refer to mutually orthogonal directions, and searching along conjugate directions can effectively avoid zigzag convergence.
#### 2.2.2 Algorithm Flow and Implementation
The algorithm flow of the conjugate gradient method is as follows:
1. Initialize parameters: learning rate α, maximum number of iterations N, current point x0, conjugate direction d0 = -∇f(x0).
2. Iterative update:
- Compute conjugate direction: dn+1 = -∇f(xn) + βndn
- Compute step size: αn = (dn^T (-∇f(xn))) / (dn^T dn)
- Update current point: xn+1 = xn - αndn
3. Determine the termination condition:
- Maximum number of iterations N reached
- Gradient approaches zero: ‖∇f(xn)‖ < ε
```python
import numpy as np
def conjugate_gradient(f, x0, alpha=0.01, N=1000, epsilon=1e-6):
"""Solve for local minima using conjugate gradient method
Args:
f: Objective function
x0: Initial point
alpha: Learning rate
N: Maximum number of iterations
epsilon: Termination condition threshold
Returns:
Local minimum
"""
x = x0
d = -np.nabla(f, x) # Initialize conjugate direction
for i in range(N):
grad = np.nabla(f, x) # Compute function gradient
beta = np.dot(grad, grad) / np.dot(d, grad) # Compute conjugate direction coefficient
d = -grad + beta * d # Update conjugate direction
alpha = np.dot(d, -grad) / np.dot(d, d) # Compute step size
x -= alpha * d # Update current point
if np.linalg.norm(grad) < epsilon: # Check termination condition
break
return x
```
### 2.3 Newton's Method
#### 2.3.1 Basic Principles
Newton's method is a second-order optimization algorithm that accelerates convergence by utilizing the second derivative (Hessian matrix) of the function. Newton's method performs iterative updates at the local quadratic approximation of the function, achieving faster convergence than gradient descent and conjugate gradient methods.
#### 2.3.2 Algorithm Flow and Implementation
The algorithm flow of Newton's method is as follows:
1. Initialize parameters: maximum number of iterations N, current point x0.
2. Iterative update:
- Compute the Hessian matrix: H(xn)
- Compute Newton direction: dn = -H(xn)^-1 ∇f(xn)
- Compute step size: αn = (dn^T (-∇f(xn))) / (dn^T H(xn) dn)
- Update current point: xn+1 = xn - αndn
3. Determine the termination condition:
- Maximum number of iterations N reached
- Gradient approaches zero: ‖∇f(xn)‖ < ε
```python
import numpy as np
def newton_method(f, x0, N=1000, epsilon=1e-6):
"""Solve for local minima using Newton's method
Args:
f: Objective function
x0: Initial point
N: Maximum number of iterations
epsilon: Termination condition threshold
Returns:
Local minimum
"""
x = x0
for i in range(N):
grad = np.nabla(f, x) # Compute function gradient
hess = np.nabla(grad, x) # Compute Hessian matrix
d = -np.linalg.inv(hess) @ grad # Compute Newton direction
alpha = np.dot(d, -grad) / np.dot(d, hess @ d) # Compute step size
x -= alpha * d # Update current point
if np.linalg.norm(grad) < epsilon: # Check termination condition
break
return x
```
## 3. Solutions Based on Variational Methods
### 3.1 Variational Principle
#### 3.1.1 Basic Concepts
The variational principle is a powerful tool for solving PDE inverse problems. The fundamental idea is to transform the solution of a PDE
0
0
相关推荐
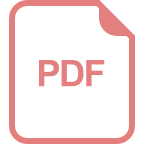
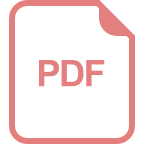
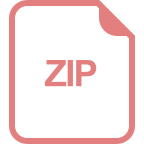
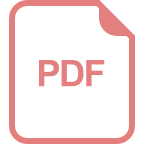
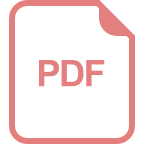
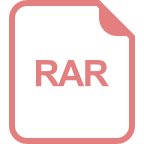
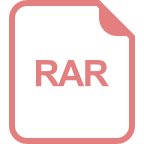
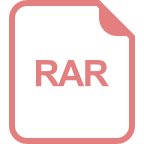