7 Steps of Characteristic Method in Partial Differential Equations: Solving Equations Along Characteristic Lines
发布时间: 2024-09-14 08:53:06 阅读量: 25 订阅数: 19 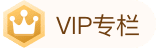
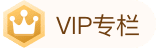
# Overview of the Method of Characteristic for Partial Differential Equations
Partial Differential Equations (PDEs) are extensively used in physics, engineering, finance, and many other fields. The method of characteristics is a powerful tool for solving PDEs that involves tracking the trajectories of characteristic lines within the equation to obtain the solution.
The main idea of the method of characteristics is to transform a PDE into a series of ordinary differential equations (ODEs) along characteristic lines. These ODEs are often easier to solve, thus enabling the explicit or approximate solution of the PDE. The method of characteristics is particularly effective for solving first- and second-order PDEs and plays a crucial role in applications such as fluid dynamics and heat conduction.
# Theoretical Basis of the Method of Characteristics
### 2.1 Characteristic Lines in PDEs
**Definition:**
The characteristic lines in a PDE refer to geometric curves that the variables satisfy, where the tangent direction is consistent with the normal direction of the solution surface of the equation.
**Characteristic Equations:**
Characteristic lines satisfy the following characteristic equations:
```
du/dt = a(u,v), dv/dt = b(u,v)
```
Here, `u` and `v` are independent variables of the PDE, and `a` and `b` are coefficients within the equation.
### 2.2 Fundamental Principles of the Method of Characteristics
The method of characteristics is a technique for solving PDEs based on the following principles:
1. **Solving the characteristic equations:** Solving the characteristic equations to obtain the set of characteristic line equations.
2. **Constructing characteristic lines:** Constructing characteristic lines along the set of characteristic line equations.
3. **Simplifying equations:** Reducing the PDE to an ODE along characteristic lines.
4. **Solving the ODE:** Solving the ODE to find the solution to the PDE.
**Advantages:**
* The method of characteristics can simplify higher-order PDEs to lower-order ODEs, making them easier to solve.
* It has geometric intuition, which aids in understanding the properties of the solution to the PDE.
**Limitations:**
* The method of characteristics is only applicable to a specific class of PDEs for which the characteristic equations are solvable.
* It is sensitive to initial conditions; small variations in initial conditions can lead to significant changes in the solution.
**Code Example:**
Solving a first-order PDE `u_t + u_x = 0`:
```python
import numpy as np
def char_line_method(u0, x0, t0, dt, dx):
"""Method of characteristics for solving first-order PDEs.
Args:
u0 (float): Initial condition u(x0, t0)
x0 (float): Initial position x
t0 (float): Initial time t
dt (float): Time step
dx (float): Space step
Returns:
u (np.ndarray): Numerical solution
"""
# Calculate characteristic line equations
a = 1
b = 1
char_line_eq = np.array([a, b])
# Construct characteristic lines along the line equations
t = np.arange(t0, t0 + dt, dt)
x = np.arange(x0, x0 + dx, dx)
X, T = np.meshgrid(x, t)
char_line = X - a * T
# Simplify the equation along the characteristic lines
u = u0 * np.ones_like(X)
# Solve the ODE
for i in range(len(t)):
for j in range(len(x)):
if char_line[i, j] >= x0:
u[i, j] = u0
return u
```
**Code Logic Analysis:**
* The `char_line_method` function takes initial conditions, initial position, time step, and space step as inputs and returns the numerical solution to the PDE.
* It calculates the characteristic line equations to obtain the set of `[a, b]`.
* It constructs the characteristic line `X - a * T` along the characteristic line equations.
* It simplifies the equation along the characteristic line, reducing the PDE to the ODE `u_t = 0`.
* It solves the ODE to obtain the numerical solution `u` to the PDE.
**Parameter Explanation:**
* `u0`: Initial condition `u(x0, t0)
0
0