Numerical Optimization Algorithms and Practical Problem Solving
发布时间: 2024-09-14 22:57:34 阅读量: 9 订阅数: 11 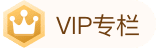
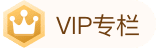
# 1. Overview of Numerical Optimization Algorithms
## 1.1 Definition and Role of Numerical Optimization Algorithms
Numerical optimization algorithms are a class of methods that optimize mathematical models to find optimal or near-optimal solutions. They have extensive applications in solving practical problems, including but not limited to engineering design, production scheduling, electronic circuit design, and urban planning.
The main role of numerical optimization algorithms is to search for the optimal solution in the possible solution space based on given objective functions and constraints. They can help us optimize designs, improve production efficiency, reduce costs, and enhance the accuracy and effectiveness of decision-making to some extent.
## 1.2 Classification of Common Numerical Optimization Algorithms
Numerical optimization algorithms can be categorized in different ways. Depending on the form of the algorithm, they can be divided into deterministic algorithms and stochastic algorithms.
Deterministic algorithms mainly include gradient descent and Newton's method. These methods use the gradient information of the objective function during the search process to guide the search direction and update parameters, gradually approaching the optimal solution.
Stochastic algorithms mainly include genetic algorithms, ant colony algorithms, and particle swarm optimization. These algorithms simulate processes such as natural biological evolution and group behavior, searching the possible solution space with a certain degree of randomness to find an approximate optimal solution.
## 1.3 Case Studies of Numerical Optimization Algorithms in Practical Problems
Numerical optimization algorithms are widely applied in practical problems. Here are some specific case studies:
- In the field of engineering design, numerical optimization algorithms can be used to optimize structural design, material selection, and parameter adjustment to improve product performance and reduce costs.
- In production scheduling, numerical optimization algorithms can help rationally plan production, optimize material delivery routes, and improve production efficiency and resource utilization.
- In electronic circuit design, numerical optimization algorithms can be used for circuit parameter adjustment and filter design to enhance circuit performance and stability.
- In urban planning, numerical optimization algorithms can be used for traffic flow optimization and urban layout planning to improve traffic efficiency and the quality of life for residents.
These case studies fully demonstrate the value and importance of numerical optimization algorithms in solving practical problems. By reasonably selecting and using numerical optimization algorithms, we can better solve various complex problems in real life.
# 2. Principles and Fundamental Concepts of Numerical Optimization Algorithms
Numerical optimization algorithms are a method for solving optimization problems frequently encountered in practical applications. This chapter will introduce the principles and fundamental concepts of several common numerical optimization algorithms and analyze their advantages and disadvantages.
### 2.1 Gradient Descent Method
Gradient descent is a common numerical optimization algorithm that iteratively seeks the minimum point of a function based on its gradient information. The specific steps are as follows:
1. Start with an initial point x_0;
2. Calculate the gradient g(x_k) of the function at the current point;
3. Update the position of the point x_{k+1} = x_k - α g(x_k), where α is the learning rate, controlling the step size of each iteration.
The advantage of the gradient descent method is its simplicity and ease of implementation, but it may get stuck in local minima and is sensitive to parameter selection.
Here is the Python implementation of the gradient descent method:
```python
def gradient_descent(f, df, x0, learning_rate, max_iter):
x = x0
for i in range(max_iter):
gradient = df(x)
x -= learning_rate * gradient
return x
```
Code explanation:
- `f` is the objective function to be optimized;
- `df` is the gradient function of the objective function;
- `x0` is the initial position of the point;
- `learning_rate` is the learning rate;
- `max_iter` is the maximum number of iterations.
### 2.2 Newton's Method
Newton's method is another commonly used numerical optimization algorithm that iteratively approximates the minimum point of a function using the first and second derivatives of the function. The specific steps are as follows:
1. Start with an initial point x_0;
2. Calculate the first derivative f'(x_k) and the second derivative f''(x_k) of the function at the current point;
3. Update the position of the point x_{k+1} = x_k - f'(x_k) / f''(x_k).
The advantage of Newton's method is its fast convergence rate and good performance when near the minimum point, but it may be influenced by the choice of initial point and the non-positivity of the Hessian matrix.
Here is the Python implementation of Newton's method:
```python
def newton_method(f, df, ddf, x0, max_iter):
x = x0
for i in range(max_iter):
gradient = df(x)
hessian = ddf(x)
x -= np.linalg.inv(hessian) @ gradient
return x
```
Code explanation:
- `f` is the objective function to be optimized;
- `df` is the first derivative function of the objective function;
- `ddf` is the second derivative function of the objective function;
- `x0` is the initial position of the point;
- `max_iter` is the maximum number of iterations.
### 2.3 Genetic Algorithm
A genetic algorithm is a numerical optimization algorithm that simulates natural evolution, iteratively searching for the optimal solution by simulating genetic operations such as inheritance, mutation, and selection. The specific steps are as follows:
1. Initialize a population and randomly generate a set of initial solutions;
2. Calculate the fitness of each individual and perform selection based on fitness;
3. Generate new individuals through genetic crossover and mutation, replacing some of the original individuals;
4. Repeat steps 2 and 3 until a termination condition is met.
The advantage of genetic algorithms is that they can perform global searches and are suitable for complex optimization problems, but they require appropriate parameters to be set according to the characteristics of the problem.
Here is the Python implementation of the genetic algorithm:
```python
def genetic_algorithm(f, population_size, mutation_rate, max_iter):
population = initialize_population(population_size)
for i in range(max_iter):
fitness = evaluate_fitness(f, population)
parents = select_parents(population, fitness)
offspring = crossover(parents)
offspring = mutate(offspring, mutation_rate)
population = replace_population(population, offspring)
return get_best_solution(f, population)
```
Code explanation:
- `f` is the objective function to be optimized;
- `population_size` is the number of individuals in the population;
- `mutation_rate` is the mutation probability;
- `max_iter` is the maximum number of iterations.
### 2.4 Ant Colony Algorithm
The ant colony algorithm is a numerical optimization algorithm that simulates the foraging behavior of ants, finding the optimal solution by simulating ant information exchange and path selection. The specific steps are as follows:
1. Initialize the positions of the ants and the pheromone matrix;
2. Each ant probabilistically selects the direction of the next move and updates the pheromone matrix;
3. Repeat step 2 until a termination condition is met.
The advantage of the ant colony algorithm is its adaptability and robustness, capable of addressing complex optimization problems, but it is prone to getting stuck in local minima.
Here is the Python implementation of the ant colony algorithm:
```python
def ant_colony_algorithm(graph, num_ants, num_iterations):
ants = initialize_ants(graph, num_ants)
best_solution = None
for i in range(num_iterations):
for ant in ants:
ant.construct_solution(graph)
ant.update_pheromone(graph)
if best_solution is None or ant.get_solution_cost(graph) < best_solution.get_solution_cost(graph):
best_solution = ant.get_solution()
evaporate_pheromone(graph)
return best_solution
```
Code explanation:
- `graph` is the graph model of the problem;
- `num_ants` is the number of ants;
- `num_iterations` is the number of iterations.
### 2.5 Particle Swarm Optimization
Particle swarm optimization is a numerical optimization algorithm that simulates group behavior, searching for the optimal solution by simulating the cooperation and information exchange of bird flocks. The specific steps are as follows:
1. Initialize the positions and velocities of the particles;
2. Each particle updates its velocity and position based on its own and its neighbors' information, and updates the global optimal solution;
3. Repeat step 2 until a termination condition is met.
The advantage of the particle swarm optimization algorithm is that it can efficiently search the problem space and is suitable for continuous optimization problems, but it is sensitive to parameter choices.
Here is the Python implementation of the particle swarm optimization algorithm:
```python
def particle_swarm_algorithm(f, num_particles, max_iter):
particles = initialize_particles(num_particles)
global_best_solution = None
for i in range(max_iter):
for particle in particles:
particle.update_velocity(global_best_solution)
particle.update_position()
if global_best_solution is None or particle.get_fitness() < global_best_solution.get_fitness():
global_best_solution = particle.get_solution()
return global_best_solution
```
Code explanation:
- `f` is the objective function to be optimized;
- `num_particles` is the number of particles;
- `max_iter` is the maximum number of iterations.
### 2.6 Analysis of the Advantages and Disadvantages of Numerical Optimization Algorithms
Each numerical optimization algorithm has its unique advantages and disadvantages. Table 2.1 summarizes the advantages and disadvantages of gradient descent, Newton's method, genetic algorithms, ant colony algorithms, and particle swarm optimization.
Table 2.1 Advantages and Disadvantages of Numerical Optimization Algorithms
| Algorithm | Advantages
0
0