Python读取MySQL特定列:灵活提取所需数据的秘诀
发布时间: 2024-07-31 09:54:58 阅读量: 25 订阅数: 16 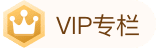
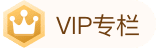
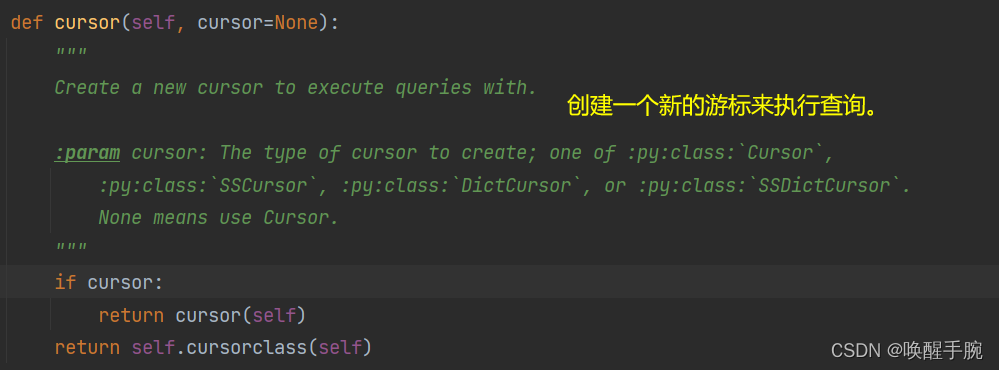
# 1. Python连接MySQL数据库
在Python中连接MySQL数据库需要使用第三方库,如PyMySQL或mysqlclient。以下示例演示如何使用PyMySQL连接MySQL数据库:
```python
import pymysql
# 连接数据库
connection = pymysql.connect(
host='localhost',
user='root',
password='password',
database='database_name'
)
```
其中,`host`是数据库服务器地址,`user`和`password`是数据库用户名和密码,`database_name`是数据库名称。
# 2. Python读取MySQL特定列
在本章节中,我们将深入探究如何使用Python读取MySQL数据库中的特定列。我们将介绍三种不同的方法:使用SELECT语句指定特定列、使用WHERE子句过滤数据以及使用ORDER BY子句排序数据。
### 2.1 使用SELECT语句指定特定列
#### 2.1.1 基本语法和示例
SELECT语句用于从数据库中检索数据。要指定要检索的特定列,请在SELECT子句中列出列名。例如,以下查询将从名为"customers"的表中检索"name"和"email"列:
```python
import mysql.connector
mydb = mysql.connector.connect(
host="localhost",
user="username",
password="password",
database="mydatabase"
)
mycursor = mydb.cursor()
mycursor.execute("SELECT name, email FROM customers")
myresult = mycursor.fetchall()
for x in myresult:
print(x)
```
**代码逻辑逐行解读:**
1. 导入必要的MySQL连接器库。
2. 使用`mysql.connector`模块建立与MySQL数据库的连接。
3. 创建一个游标对象,用于执行SQL查询。
4. 使用`execute()`方法执行SELECT查询,并指定要检索的列名。
5. 使用`fetchall()`方法获取查询结果,并将结果存储在`myresult`变量中。
6. 使用一个循环迭代查询结果,并打印每一行。
#### 2.1.2 通配符的使用
通配符可用于检索表中的所有列。以下查询将从"customers"表中检索所有列:
```python
mycursor.execute("SELECT * FROM customers")
```
**代码逻辑逐行解读:**
1. 使用`*`通配符表示检索所有列。
### 2.2 使用WHERE子句过滤数据
#### 2.2.1 基本语法和示例
WHERE子句用于根据指定条件过滤查询结果。例如,以下查询将从"customers"表中检索"name"和"email"列,但仅限于"city"列等于"London"的行:
```python
mycursor.execute("SELECT name, email FROM customers WHERE city = 'London'")
```
**代码逻辑逐行解读:**
1. 在SELECT语句中添加WHERE子句,并指定过滤条件。
#### 2.2.2 比较运算符和逻辑运算符
WHERE子句支持各种比较运算符和逻辑运算符,用于构建复杂的过滤条件。以下是一些常见的运算符:
| 运算符 | 描述 |
|---|---|
| = | 等于 |
| != | 不等于 |
| > | 大于 |
| < | 小于 |
| >= | 大于或等于 |
| <= | 小于或等于 |
| AND | 逻辑与 |
| OR | 逻辑或 |
| NOT | 逻辑非 |
例如,以下查询将从"customers"表中检索"name"和"email"列,但仅限于"city"列等于"London"或"city"列等于"Paris"的行:
```python
mycursor.execute("SELECT name, email FROM customers WHERE city = 'London' OR city = 'Paris'")
```
**代码逻辑逐行解读:**
1. 在WHERE子句中使用OR运算符组合多个过滤条件。
### 2.3 使用ORDER BY子句排序数据
#### 2.3.1 基本语法和示例
ORDER BY子句用于根据指定列对查询结果进行排序。例如,以下查询将从"customers"表中检索"name"和"email"列,并按"name"列升序排序:
```python
mycursor.execute("SELECT name, email FROM customers ORDER BY name")
```
**代码逻辑逐行解读:**
1. 在SELECT语句中添加ORDER BY子句,并指定排序列。
#### 2.3.2 排序规则和排序方向
ORDER BY子句支持ASC和DESC关键字来指定排序规则和排序方向。ASC表示升序(从最小到最大),而DESC表示降序(从最大到最小)。例如,以下查询将按"name"列降序排序:
```python
mycursor.execute("SELECT name, email FROM customers ORDER BY name DESC")
```
**代码逻辑逐行解读:**
1. 在ORDER BY子句中使用DESC关键字指定降序排序。
# 3.1 使用fetch*()方法获取所有结果
#### 3.1.1 基本语法和示例
`fetch*()` 方法用于获取查询结果集中所有剩余的行。它返回一个包含所有结果的元组列表,其中每个元组代表一行数据。
```python
import mysql.connector
# 建立数据库连接
mydb = mysql.connector.connect(
host="localhost",
user="username",
password="password",
database="database_name"
)
# 创建游标对象
mycursor = mydb.cursor()
# 执行查询语句
mycursor.execute("SELECT * FROM table_name")
# 获取所有结果
results = mycursor.fetchall()
# 遍历结果并打印每一行
for row in results:
print(row)
```
#### 3.1.2 迭代处理结果
也可以使用迭代器来处理结果,这可以节省内存,尤其是在处理大型数据集时。
```python
import mysql.connector
# 建立数据库连接
mydb = mysql.connector.connect(
host="localhost",
user="username",
password="password",
database="database_name"
)
# 创建游标对象
mycursor = mydb.cursor()
# 执行查询语句
mycursor.execute("SELECT * FROM table_name")
# 迭代处理结果
for row in mycursor:
print(row)
```
### 3.2 使用fetchone()方法获取单条结果
#### 3.2.1 基本语法和示例
`fetchone()` 方法用于获取查询结果集中的一行数据。它返回一个元组,代表一行数据。如果结果集中没有更多行,则返回 `None`。
```python
import mysql.connector
# 建立数据库连接
mydb = mysql.connector.connect(
host="localhost",
user="username",
password="password",
database="database_name"
)
# 创建游标对象
mycursor = mydb.cursor()
# 执行查询语句
mycursor.execute("SELECT * FROM table_name")
# 获取单条结果
result = mycursor.fetchone()
# 打印结果
print(result)
```
#### 3.2.2 循环处理结果
可以使用循环来获取查询结果集中所有行。
```python
import mysql.connector
# 建立数据库连接
mydb = mysql.connector.connect(
host="localhost",
user="username",
password="password",
database="database_name"
)
# 创建游标对象
mycursor = mydb.cursor()
# 执行查询语句
mycursor.execute("SELECT * FROM table_name")
# 循环处理结果
while True:
result = mycursor.fetchone()
if result is None:
break
print(result)
```
### 3.3 使用fetchmany()方法获取指定数量结果
#### 3.3.1 基本语法和示例
`fetchmany()` 方法用于获取查询结果集中指定数量的行。它返回一个包含指定数量行的元组列表。如果结果集中没有更多行,则返回一个空列表。
```python
import mysql.connector
# 建立数据库连接
mydb = mysql.connector.connect(
host="localhost",
user="username",
password="password",
database="database_name"
)
# 创建游标对象
mycursor = mydb.cursor()
# 执行查询语句
mycursor.execute("SELECT * FROM table_name")
# 获取指定数量结果
results = mycursor.fetchmany(3)
# 打印结果
for row in results:
print(row)
```
#### 3.3.2 分页处理结果
可以使用 `fetchmany()` 方法来实现分页处理结果。
```python
import mysql.connector
# 建立数据库连接
mydb = mysql.connector.connect(
host="localhost",
user="username",
password="password",
database="database_name"
)
# 创建游标对象
mycursor = mydb.cursor()
# 执行查询语句
mycursor.execute("SELECT * FROM table_name")
# 分页处理结果
page_size = 10
page_number = 1
while True:
results = mycursor.fetchmany(page_size)
if not results:
break
# 处理当前页面的结果
for row in results:
print(row)
page_number += 1
```
# 4. Python读取MySQL特定列的实践应用
### 4.1 数据分析和报表生成
**4.1.1 提取特定列数据进行分析**
在数据分析中,经常需要对特定列的数据进行提取和分析。例如,在销售数据分析中,我们可能需要提取产品销量列的数据来分析产品的销售趋势。
```python
import mysql.connector
# 连接数据库
connection = mysql.connector.connect(
host="localhost",
user="root",
password="password",
database="sales_db"
)
# 创建游标
cursor = connection.cursor()
# 提取特定列数据
query = "SELECT product_name, sales_volume FROM sales_data"
cursor.execute(query)
# 获取结果
results = cursor.fetchall()
# 提取特定列数据
product_names = [result[0] for result in results]
sales_volumes = [result[1] for result in results]
# 数据分析
# ...
```
**4.1.2 生成基于特定列数据的报表**
基于特定列数据生成的报表可以帮助我们快速了解数据的分布和趋势。例如,我们可以生成基于产品销量列数据的销售报表。
```python
import pandas as pd
# 创建DataFrame
df = pd.DataFrame(results, columns=["product_name", "sales_volume"])
# 生成报表
report = df.groupby("product_name").sum()
print(report)
```
### 4.2 数据导出和导入
**4.2.1 导出特定列数据到文件或数据库**
将特定列数据导出到文件或其他数据库可以用于数据备份、数据交换或进一步处理。
```python
# 导出到文件
with open("sales_data.csv", "w") as f:
f.write("product_name,sales_volume\n")
for result in results:
f.write(f"{result[0]},{result[1]}\n")
# 导出到另一个数据库
target_connection = mysql.connector.connect(
host="remote_host",
user="target_user",
password="target_password",
database="target_db"
)
target_cursor = target_connection.cursor()
query = "INSERT INTO sales_data (product_name, sales_volume) VALUES (%s, %s)"
for result in results:
target_cursor.execute(query, result)
target_connection.commit()
```
**4.2.2 导入特定列数据到MySQL数据库**
从文件或其他数据库导入特定列数据可以用于数据更新、数据合并或数据恢复。
```python
# 从文件导入
with open("sales_data.csv", "r") as f:
lines = f.readlines()
for line in lines[1:]:
product_name, sales_volume = line.split(",")
query = "INSERT INTO sales_data (product_name, sales_volume) VALUES (%s, %s)"
cursor.execute(query, (product_name, sales_volume))
# 从另一个数据库导入
query = "SELECT product_name, sales_volume FROM sales_data"
source_cursor = source_connection.cursor()
source_cursor.execute(query)
source_results = source_cursor.fetchall()
for result in source_results:
query = "INSERT INTO sales_data (product_name, sales_volume) VALUES (%s, %s)"
cursor.execute(query, result)
connection.commit()
```
### 4.3 数据验证和清理
**4.3.1 使用特定列数据进行数据验证**
特定列数据可以用于验证数据的完整性和准确性。例如,在客户数据中,我们可以使用电子邮件列数据来验证电子邮件地址的有效性。
```python
import re
# 验证电子邮件地址
for result in results:
email = result[0]
if not re.match(r"^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$", email):
print(f"Invalid email address: {email}")
```
**4.3.2 清理特定列中的异常数据**
特定列数据可以用于识别和清理异常数据。例如,在销售数据中,我们可以使用价格列数据来识别异常值。
```python
# 识别异常值
for result in results:
price = result[0]
if price < 0 or price > 1000:
print(f"Abnormal price: {price}")
```
# 5. Python读取MySQL特定列的优化技巧
### 5.1 使用索引优化查询速度
索引是数据库中一种特殊的数据结构,用于快速查找数据。为经常查询的特定列创建索引可以显著提高查询速度。
**示例:**
```python
# 创建索引
cursor.execute("CREATE INDEX idx_name ON table_name(column_name)")
```
### 5.2 使用批量处理减少网络开销
批量处理是指一次性处理多个查询或操作,而不是逐个执行。这可以减少网络开销,提高整体性能。
**示例:**
```python
# 批量插入数据
cursor.executemany("INSERT INTO table_name (column1, column2) VALUES (%s, %s)", data_list)
```
### 5.3 使用连接池减少连接开销
连接池是一种预先建立和维护的数据库连接集合。使用连接池可以避免每次查询都建立新的连接,从而减少连接开销。
**示例:**
```python
import mysql.connector
# 创建连接池
pool = mysql.connector.connect(
host="localhost",
user="root",
password="password",
database="database_name",
)
# 获取连接
connection = pool.getconn()
```
### 5.4 使用缓存机制减少查询次数
缓存机制可以将查询结果存储在内存中,以便后续查询直接从缓存中获取。这可以减少对数据库的查询次数,提高性能。
**示例:**
```python
import cachetools
# 创建缓存
cache = cachetools.LRUCache(maxsize=100)
# 获取缓存数据
data = cache.get(query)
# 如果缓存中没有数据,则查询数据库并缓存结果
if data is None:
data = cursor.execute(query).fetchall()
cache[query] = data
```
0
0
相关推荐
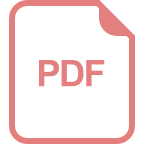
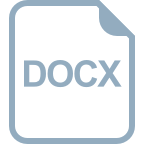
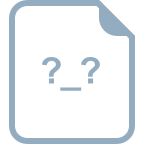





