【PHP连接MSSQL数据库指南】:从入门到精通,助力你的PHP应用程序高效连接MSSQL数据库
发布时间: 2024-08-02 10:03:16 阅读量: 14 订阅数: 13 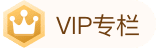
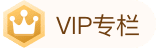
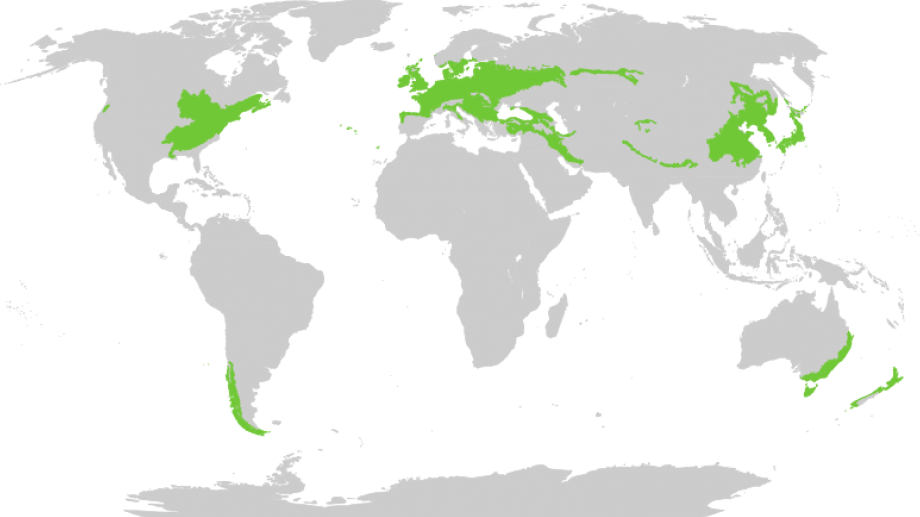
# 1. PHP连接MSSQL数据库基础
PHP连接MSSQL数据库需要使用专门的扩展,例如php_sqlsrv或pdo_sqlsrv。这些扩展提供了连接、查询和操作MSSQL数据库所需的函数和类。
连接MSSQL数据库需要一个连接字符串,其中包含服务器地址、数据库名称、用户名和密码等信息。连接字符串可以使用`sqlsrv_connect()`函数或`PDO`类进行解析。
```php
$serverName = "serverName";
$databaseName = "databaseName";
$uid = "username";
$pwd = "password";
// 使用sqlsrv_connect()函数连接MSSQL数据库
$conn = sqlsrv_connect($serverName, $databaseName, $uid, $pwd);
// 使用PDO类连接MSSQL数据库
$conn = new PDO("sqlsrv:Server=$serverName;Database=$databaseName", $uid, $pwd);
```
# 2. MSSQL数据库连接配置
### 2.1 连接字符串的组成和配置
连接字符串是建立PHP与MSSQL数据库连接的关键,它包含了连接数据库所需的信息。连接字符串的格式如下:
```
"Driver={SQL Server};Server=server_name;Database=database_name;Uid=username;Pwd=password;"
```
| 参数 | 说明 |
|---|---|
| Driver | 指定数据库驱动程序,对于MSSQL数据库为 "SQL Server" |
| Server | 数据库服务器的名称或IP地址 |
| Database | 要连接的数据库名称 |
| Uid | 数据库用户名 |
| Pwd | 数据库密码 |
**示例连接字符串:**
```
$connectionString = "Driver={SQL Server};Server=localhost;Database=test;Uid=sa;Pwd=123456;";
```
### 2.2 连接参数的设置和优化
除了基本的连接参数外,还可以通过设置其他连接参数来优化连接行为和性能。
**连接超时:**
```
$connectionOptions = array(
'ConnectTimeout' => 10, // 连接超时时间(秒)
);
```
**重试连接:**
```
$connectionOptions = array(
'RetryAttempts' => 3, // 重试连接次数
'RetryInterval' => 1, // 重试间隔时间(秒)
);
```
**字符集和排序规则:**
```
$connectionOptions = array(
'CharacterSet' => 'UTF-8', // 字符集
'Collation' => 'SQL_Latin1_General_CP1_CI_AS', // 排序规则
);
```
**加密连接:**
```
$connectionOptions = array(
'Encrypt' => true, // 是否加密连接
);
```
**示例连接参数设置:**
```
$connectionOptions = array(
'ConnectTimeout' => 10,
'RetryAttempts' => 3,
'RetryInterval' => 1,
'CharacterSet' => 'UTF-8',
'Collation' => 'SQL_Latin1_General_CP1_CI_AS',
'Encrypt' => true,
);
```
# 3. PHP与MSSQL数据库交互
### 3.1 查询和更新数据的操作
#### 查询数据
使用PHP连接MSSQL数据库进行查询操作时,可以使用`sqlsrv_query()`函数。该函数接收两个参数:连接资源和SQL查询语句。查询结果将返回一个结果集资源,可以使用`sqlsrv_fetch_array()`函数逐行获取结果。
```php
$sql = "SELECT * FROM users";
$result = sqlsrv_query($conn, $sql);
while ($row = sqlsrv_fetch_array($result, SQLSRV_FETCH_ASSOC)) {
echo $row['id'] . " " . $row['name'] . "\n";
}
```
#### 更新数据
更新数据可以使用`sqlsrv_query()`函数执行UPDATE、INSERT和DELETE语句。该函数返回受影响的行数。
```php
$sql = "UPDATE users SET name='John Doe' WHERE id=1";
$result = sqlsrv_query($conn, $sql);
if ($result) {
echo "Updated successfully";
} else {
echo "Update failed";
}
```
### 3.2 事务和并发控制
#### 事务
事务是一组原子操作,要么全部成功,要么全部失败。在PHP中,可以使用`sqlsrv_begin_transaction()`和`sqlsrv_commit()`或`sqlsrv_rollback()`函数来管理事务。
```php
sqlsrv_begin_transaction($conn);
$sql1 = "UPDATE users SET name='John Doe' WHERE id=1";
$result1 = sqlsrv_query($conn, $sql1);
$sql2 = "UPDATE users SET age=30 WHERE id=1";
$result2 = sqlsrv_query($conn, $sql2);
if ($result1 && $result2) {
sqlsrv_commit($conn);
echo "Transaction committed successfully";
} else {
sqlsrv_rollback($conn);
echo "Transaction rolled back";
}
```
#### 并发控制
MSSQL数据库使用锁机制来实现并发控制。当一个事务对数据进行修改时,它会获得一个锁,以防止其他事务同时修改相同的数据。锁的类型包括:
* **共享锁 (S)**:允许其他事务读取数据,但不能修改。
* **排他锁 (X)**:不允许其他事务读取或修改数据。
### 3.3 存储过程和函数的调用
#### 存储过程
存储过程是一组预编译的SQL语句,可以作为单元执行。它们可以接受参数并返回结果集。在PHP中,可以使用`sqlsrv_prepare()`和`sqlsrv_execute()`函数来调用存储过程。
```php
$sql = "EXEC GetUserDetails @id=?";
$params = array(1);
$stmt = sqlsrv_prepare($conn, $sql, $params);
sqlsrv_execute($stmt);
while ($row = sqlsrv_fetch_array($stmt, SQLSRV_FETCH_ASSOC)) {
echo $row['id'] . " " . $row['name'] . "\n";
}
```
#### 函数
函数与存储过程类似,但它们不返回结果集。在PHP中,可以使用`sqlsrv_query()`函数来调用函数。
```php
$sql = "SELECT dbo.GetFullName(1)";
$result = sqlsrv_query($conn, $sql);
if ($result) {
$row = sqlsrv_fetch_array($result, SQLSRV_FETCH_ASSOC);
echo $row['FullName'];
}
```
# 4. MSSQL数据库高级操作**
**4.1 数据类型转换和处理**
MSSQL数据库支持多种数据类型,包括整数、浮点数、字符串、日期时间和布尔值。在PHP中,可以使用PDO或ODBC扩展来处理这些数据类型。
**PDO数据类型转换**
PDO提供了内置的数据类型转换函数,用于将PHP变量转换为MSSQL数据类型。这些函数包括:
```php
PDO::PARAM_INT
PDO::PARAM_STR
PDO::PARAM_BOOL
PDO::PARAM_NULL
```
例如,以下代码将PHP变量`$value`转换为整数类型:
```php
$stmt->bindParam(':value', $value, PDO::PARAM_INT);
```
**ODBC数据类型转换**
ODBC也提供了数据类型转换函数,但需要手动指定目标数据类型。这些函数包括:
```php
SQLBindParameter()
SQLPutData()
```
例如,以下代码将PHP变量`$value`转换为整数类型:
```php
SQLBindParameter($stmt, ':value', SQL_C_LONG, SQL_INTEGER, 0, 0, $value);
```
**4.2 视图和索引的创建和管理**
**视图**
视图是虚拟表,它从一个或多个基础表中派生数据。视图可以简化复杂的查询,并提供对数据的不同视角。
**创建视图**
```sql
CREATE VIEW view_name AS
SELECT column1, column2, ...
FROM table1
WHERE condition;
```
**索引**
索引是数据库表中的一种数据结构,它可以加快对表的查询速度。索引通过创建列值的排序顺序来实现。
**创建索引**
```sql
CREATE INDEX index_name ON table_name (column1, column2, ...);
```
**4.3 查询优化和性能调优**
**查询优化**
查询优化是指通过调整查询语句来提高其性能。一些常见的查询优化技术包括:
* 使用适当的索引
* 避免不必要的连接
* 使用子查询代替嵌套循环
* 缓存查询结果
**性能调优**
性能调优涉及优化数据库服务器的配置和设置,以提高整体性能。一些常见的性能调优技术包括:
* 调整内存设置
* 优化磁盘I/O
* 监控数据库活动
* 使用性能分析工具
# 5.1 常见连接问题及解决方法
在 PHP 连接 MSSQL 数据库时,可能会遇到一些常见问题。以下是这些问题及其解决方法:
**问题 1:连接超时**
**原因:**数据库服务器不可达或响应缓慢。
**解决方法:**
- 检查数据库服务器是否正在运行。
- 确保防火墙允许对数据库服务器的连接。
- 增加连接超时时间。
**问题 2:身份验证失败**
**原因:**提供的用户名或密码不正确。
**解决方法:**
- 仔细检查用户名和密码。
- 确保用户具有连接数据库的权限。
- 重置数据库密码。
**问题 3:SQL 语法错误**
**原因:**查询中存在语法错误。
**解决方法:**
- 检查查询语法是否正确。
- 使用调试工具或错误日志来识别错误。
- 确保使用正确的 MSSQL 语法。
**问题 4:数据库连接已关闭**
**原因:**连接已超时或被服务器关闭。
**解决方法:**
- 重新打开连接。
- 增加连接超时时间。
- 使用连接池来管理连接。
**问题 5:连接池已满**
**原因:**连接池中的连接数已达到最大值。
**解决方法:**
- 增加连接池大小。
- 优化连接使用,释放不再需要的连接。
- 使用连接池管理策略来限制同时打开的连接数。
0
0
相关推荐
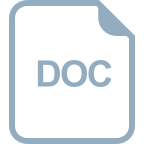
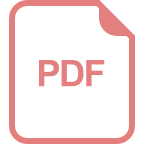





