【自然语言处理的决策树】:文本分类案例与实战技巧
发布时间: 2024-09-04 23:14:47 阅读量: 222 订阅数: 39 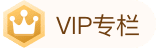
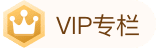
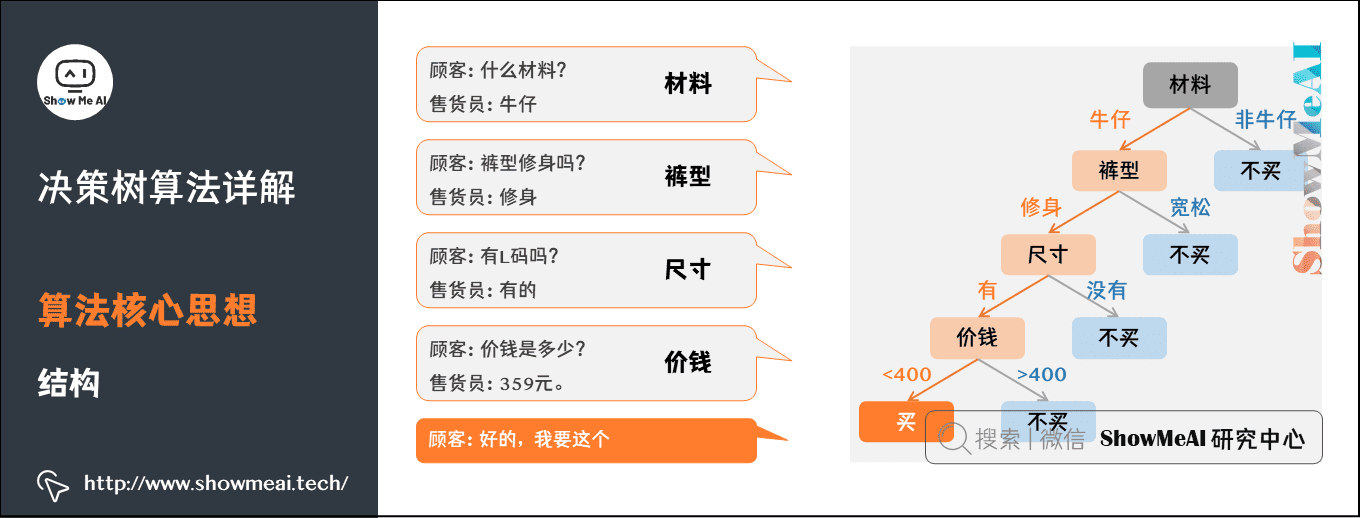
# 1. 自然语言处理与决策树基础
自然语言处理(NLP)是计算机科学和人工智能领域中的一个关键分支,旨在使计算机能够理解、解释和生成人类语言。决策树是一种常用的机器学习算法,在NLP中扮演着重要角色。它通过一系列的判断规则,将数据分配到不同的类别中,类似于人类做决策的过程。本章节将简述自然语言处理的基本概念以及决策树的基础知识,为后续章节的内容打下坚实的基础。
## 1.1 自然语言处理简介
自然语言处理是计算机与人类语言之间的桥梁。其任务涵盖了从简单的分词到复杂的语言生成等多个层面。例如,搜索引擎需要处理用户输入的查询语句,聊天机器人需要理解和回应人类的语言,机器翻译则需要将一种语言转化为另一种语言,这些应用都离不开NLP技术的支持。
## 1.2 决策树的引入
决策树模型以其直观性和高效性成为解决分类问题的有力工具。它在NLP中的应用包括文本分类、情感分析等,能够帮助系统理解文本内容并做出决策。通过决策树模型,我们可以将复杂的自然语言问题简化为树状结构的逻辑判断,进而提取出决策规则。
在深入讨论决策树的理论与构建方法之前,先了解其基础概念和在NLP中的应用是至关重要的。这不仅有助于读者更好地理解后续章节的专业内容,也为实践操作打下基础。
# 2. 决策树的理论与构建方法
## 2.1 决策树模型简介
### 2.1.1 决策树的历史和重要性
在机器学习领域,决策树是一种基本的分类与回归方法。它模拟人类在进行决策时的思考过程,是一种树形结构。尽管决策树的起源可以追溯到20世纪60年代,但其发展和完善是在80年代以后,特别是ID3算法的提出,标志着决策树方法的成熟。
决策树之所以重要,在于其模型的简洁性、解释性和较高的准确性。它不需要对数据进行规范化处理,并且对于非线性关系的建模能力较强。此外,决策树在处理大规模数据集时表现出色,且易于理解和解释,这使得决策树成为了数据挖掘和机器学习中非常流行的算法之一。
### 2.1.2 决策树的工作原理
决策树的构建过程模拟了人类做决策的思考方式。它从根节点开始,使用属性对数据集进行分割,生成子节点。这个分割过程基于特征选择的准则,不断递归地进行,直到满足停止条件。最终,决策树的每个叶节点代表一个分类结果或回归输出。
构建决策树的关键在于选择最佳特征进行分割,以及决定何时停止分割。在分类任务中,我们通常寻找使得子集纯度增加最大的属性,而在回归任务中,我们寻求最小化预测误差。
## 2.2 构建决策树的算法
### 2.2.1 ID3算法的原理和实现
ID3(Iterative Dichotomiser 3)算法是最经典的决策树学习算法之一。ID3算法的核心思想是使用信息增益作为标准来选择特征。信息增益是基于熵的概念,它反映了通过某个属性对数据集进行分割,数据集纯度的提升程度。
**代码块:ID3算法示例**
```python
import numpy as np
import pandas as pd
# 假设有一个简单的数据集
data = pd.DataFrame({
'Outlook': ['Sunny', 'Sunny', 'Overcast', 'Rain', 'Rain', 'Rain', 'Overcast', 'Sunny', 'Sunny', 'Rain', 'Sunny', 'Overcast', 'Overcast', 'Rain'],
'Temperature': ['Hot', 'Hot', 'Hot', 'Mild', 'Cool', 'Cool', 'Cool', 'Mild', 'Cool', 'Mild', 'Mild', 'Mild', 'Hot', 'Mild'],
'Humidity': ['High', 'High', 'High', 'High', 'Normal', 'Normal', 'Normal', 'High', 'Normal', 'Normal', 'Normal', 'High', 'Normal', 'High'],
'PlayTennis': ['No', 'No', 'Yes', 'Yes', 'Yes', 'No', 'Yes', 'No', 'Yes', 'Yes', 'Yes', 'Yes', 'Yes', 'No']
})
# 计算熵
def entropy(data, target_col):
entropy_val = 0
unique_vals = data[target_col].unique()
for val in unique_vals:
p = len(data[data[target_col] == val]) / len(data)
entropy_val -= p * np.log2(p)
return entropy_val
# 计算信息增益
def information_gain(data, split_name, target_col):
total_entropy = entropy(data, target_col)
vals, counts = np.unique(data[split_name], return_counts=True)
Weighted_Entropy = 0
for val, count in zip(vals, counts):
Weighted_Entropy += (count / len(data)) * entropy(data.where(data[split_name] == val).dropna(), target_col)
Information_Gain = total_entropy - Weighted_Entropy
return Information_Gain
# 以'Outlook'为例来选择最佳分割点
best_feature = max(data.columns[:-1], key=lambda v: information_gain(data, v, 'PlayTennis'))
print(f"Best feature for split: {best_feature}")
```
在上述代码中,我们定义了`entropy`函数来计算数据集的熵,以及`information_gain`函数来计算分割后的信息增益。然后我们通过计算各个特征的信息增益来选择最佳分割特征。
### 2.2.2 C4.5算法的原理和实现
C4.5算法是ID3的后继版本,它继承了ID3的决策树构建方法,但在处理连续值属性和缺失值问题上有很大的改进。C4.5使用增益率(gain ratio)来选择特征,它还引入了剪枝机制,通过剪枝来解决过拟合的问题。
### 2.2.3 CART算法的原理和实现
分类与回归树(Classification and Regression Tree,CART)是一种可以用于回归和分类任务的决策树算法。CART使用二分法来分割特征空间,构建二叉树结构。相比ID3和C4.5,CART算法在处理数值型属性和缺失数据方面更加灵活。
## 2.3 决策树的剪枝技术
### 2.3.1 过拟合的概念
过拟合是机器学习中的一个重要概念,指的是模型在训练数据上表现得很好,但在新的、未见过的数据上表现不佳的现象。过拟合通常发生在模型过于复杂时,如决策树过于深入,导致模型学到了训练数据中的噪声和异常点。
### 2.3.2 剪枝的策略和方法
剪枝是一种避免过拟合的技术,它通过去除决策树中某些部分来简化模型。剪枝可以分为预剪枝和后剪枝。预剪枝是在决策树构建过程中提前停止树的增长,而后剪枝是在构建完整树之后,通过剪去一些节点来简化树的复杂度。
**mermaid流程图:剪枝技术的决策过程**
```mermaid
graph TD;
A[开始剪枝] --> B{是否满足剪枝条件};
B -- 是 --> C[剪去节点];
B -- 否 --> D[继续构建决策树];
C --> E[更新决策树];
D --> F{是否到达停止条件};
F -- 是 --> G[结束];
F -- 否 --> B;
E --> F;
```
在上述mermaid流程图中,我们展示了剪枝技术的决策过程。算法首先判断是否满足剪枝条件,如果满足,则剪去一些节点;如果不满足,则继续构建决策树。这个过程一直持续到满足停止条件为止。
在接下来的章节中,我们将探讨如何使用决策树进行文本分类,以及文本分类中的实践技巧,这将为我们深入了解NLP决策树模型的进阶应用打下坚实的基础。
# 3. 文本分类的实践技巧
随着自然语言处理技术的不断发展,文本分类作为基础而广泛的应用,已经深入到新闻筛选、垃圾邮件检测、情感分析、话题识别等多个领域。文本分类任务旨在根据文本内容自动将文本划分到一个或多个预定义的类别中,而决策树模型因其结构清晰、易于解释的特点,在文本分类任务中表现出了独特的优势。
## 3.1 文本预处理
在将文本数据输入到决策树模型之前,需要经过一系列预处理步骤以提高模型性能。文本预处理主要包含以下几个方面:
### 3.1.1 分词和向量化
分词是将句子或段落分割成单词、短语或其他有意义元素的过程,它是进行文本分析前的基础步骤。在英文中,分词较为简单,通常以空格为分隔符,但中文分词要复杂得多,需要根据语言学知识或统计模型来识别词语边界。
向量化是将分词后的文本转化为数学模型可以处理的格式,常用的有词袋模型(BOW)、TF-IDF模型等。向量化可以采用Python的`sklearn.feature_extraction.text`模块实现,代码示例如下:
```python
from sklearn.feature_extraction.text import TfidfVectorizer
# 假设corpus是包含多篇文本的列表
corpus = [
"This is the first document.",
"This document is the se
```
0
0
相关推荐
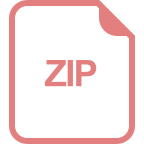
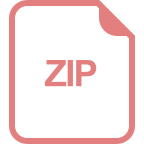
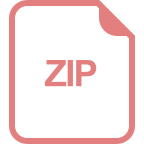





