递归算法的陷阱与规避:避免死循环,提升代码质量
发布时间: 2024-08-24 23:57:18 阅读量: 26 订阅数: 24 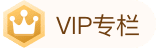
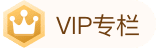
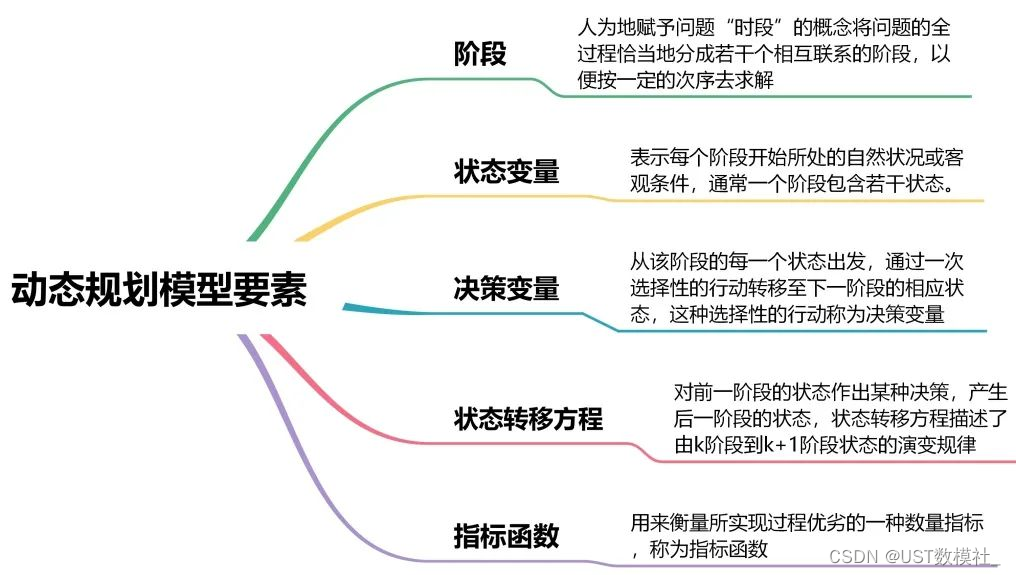
# 1. 递归算法的基本概念**
递归算法是一种通过自身调用自身来解决问题的算法。它具有以下特点:
* **自相似性:**递归函数的结构与它所解决的问题相似。
* **终止条件:**递归函数必须有一个明确的终止条件,以防止死循环。
* **递归调用:**递归函数调用自身,并传入不同的参数,将问题分解成更小的子问题。
# 2. 递归算法的陷阱
递归算法是一种强大的工具,但如果使用不当,也可能导致严重的陷阱。本章将深入探讨递归算法中常见的陷阱,并提供规避这些陷阱的实践方法。
### 2.1 死循环的成因
死循环是递归算法中最常见的陷阱之一。死循环是指算法不断调用自身,而没有明确的终止条件,导致算法无限执行。死循环可能由以下原因造成:
#### 2.1.1 缺乏明确的终止条件
明确的终止条件是递归算法的关键。如果没有明确的终止条件,算法将继续递归调用自身,直到达到系统资源限制。例如,以下代码中的递归函数没有明确的终止条件,将导致死循环:
```python
def factorial(n):
if n > 0:
return n * factorial(n - 1)
```
#### 2.1.2 过度嵌套的递归调用
过度嵌套的递归调用也会导致死循环。当递归函数被嵌套调用过多时,系统堆栈空间可能会耗尽,导致堆栈溢出。例如,以下代码中的递归函数过度嵌套,可能会导致堆栈溢出:
```python
def fibonacci(n):
if n == 0:
return 0
elif n == 1:
return 1
else:
return fibonacci(n - 1) + fibonacci(n - 2)
```
### 2.2 堆栈溢出的风险
堆栈溢出是另一个常见的递归算法陷阱。堆栈溢出是指系统堆栈空间耗尽,导致算法无法继续执行。堆栈溢出可能由以下原因造成:
#### 2.2.1 递归深度过大
递归深度过大是指递归函数调用自身过多,导致系统堆栈空间耗尽。例如,以下代码中的递归函数递归深度过大,可能会导致堆栈溢出:
```python
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n - 1)
```
#### 2.2.2 复杂数据结构导致的内存消耗
复杂数据结构,例如树或图,在递归算法中可能导致大量的内存消耗。当递归函数遍历复杂数据结构时,每个递归调用都会在堆栈中创建一个新的数据结构副本。如果数据结构非常大,这可能会导致堆栈溢出。
# 3. 规避递归陷阱的实践
### 3.1 设定明确的终止条件
递归算法的陷阱之一是缺乏明确的终止条件,导致程序陷入死循环。为了避免这种情况,必须为递归函数设定明确的终止条件。终止条件是指函数何时停止递归调用的条件。
例如,考虑一个计算阶乘的递归函数:
```python
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
```
在这个函数中,终止条件是 `n == 0`。当 `n` 等于 0 时,函数返回 1,停止递归调用。如果没有这个终止条件,函数将无限递归,导致堆栈溢出。
### 3.2 限制递归深度
另一个陷阱是递归深度过大,导致堆栈溢出。堆栈溢出是指当函数调用的深度超过系统允许的最大深度时发生的一种错误。
为了避免堆栈溢出,可以限制递归深度。一种方法是使用迭代算法代替递归算法。迭代算法使用循环而不是递归来解决问题,因此不会遇到堆栈溢出的问题。
例如,以下迭代算法可以计算阶乘:
```python
def factorial_iterative(n):
result = 1
for i in range(1, n+1):
result *= i
return result
```
### 3.3 使用尾递归优化
尾递归优化是一种技术,可以将递归调用优化为循环。尾递归是指递归函数的最后一步是递归调用。
例如,以下递归函数计算斐波那契数列:
```python
def fibonacci(n):
if n == 0:
return 0
elif n == 1:
return 1
else:
return fibonacci(n-1) + fibonacci(n-2)
```
在这个函数中,递归调用是函数的最后一步。因此,我们可以使用尾递归优化将它转换为循环:
```python
def fibonacci_iterative(n):
a, b = 0, 1
for _ in range(n):
a, b = b, a + b
return a
```
### 3.4 考虑迭代算法作为替代
在某些情况下,迭代算法可能是递归算法的更好选择。迭代算法使用循环而不是递归来解决问题,因此不会遇到堆栈溢出的问题。
例如,以下迭代算法可以计算阶乘:
```python
def factorial_iterative(n):
result = 1
for i in range(1, n+1):
result *= i
return result
```
与递归算法相比,迭代算法通常更简单、更高效。但是,递归算法有时更易于理解和实现。因此,在选择算法时,需要权衡递归和迭代的利弊。
# 4. 递归算法的优化
### 4.1
0
0
相关推荐
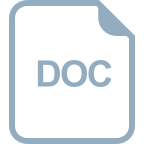
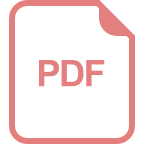
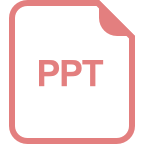
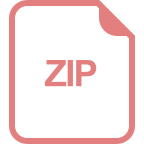
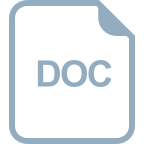
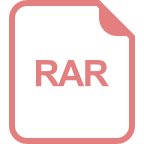
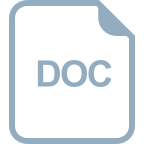