【PHP数据库实战指南】:从小白到大神,解锁数据库操作秘籍
发布时间: 2024-07-28 13:40:37 阅读量: 16 订阅数: 20 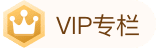
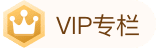
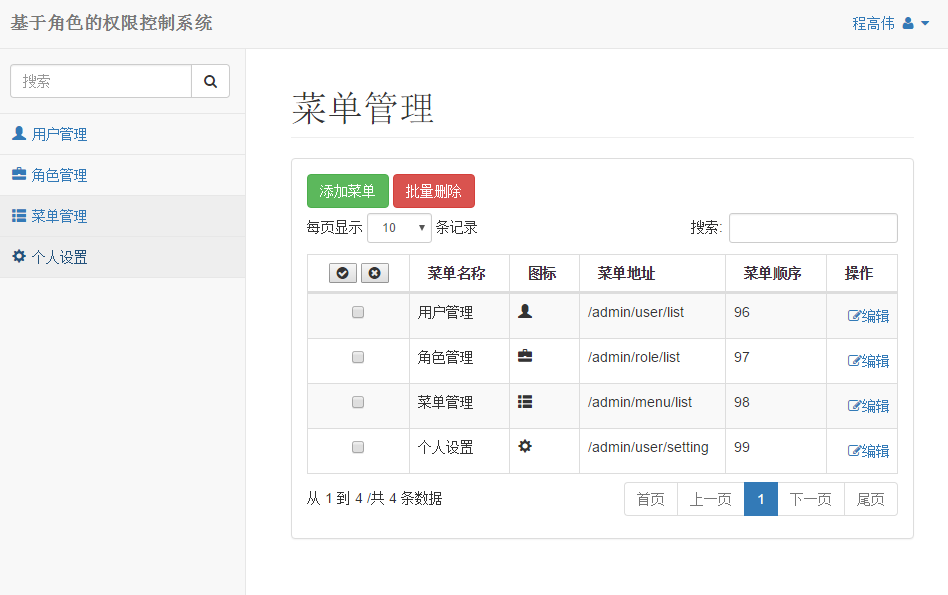
# 1. PHP数据库基础**
PHP数据库基础是理解PHP数据库操作的关键。数据库是一个存储和管理数据的系统,PHP通过数据库扩展程序与数据库进行交互。本章将介绍数据库的基本概念,包括数据库类型、数据模型和数据库管理系统(DBMS)。此外,还将讨论PHP中用于数据库操作的常用扩展程序,例如mysqli和PDO。
# 2. PHP数据库连接与操作
### 2.1 MySQL数据库连接
#### 连接数据库
```php
<?php
// 创建一个 MySQL 连接
$conn = new mysqli("localhost", "username", "password", "database_name");
// 检查连接是否成功
if ($conn->connect_error) {
die("连接失败: " . $conn->connect_error);
}
?>
```
**参数说明:**
* `localhost`: 数据库服务器地址
* `username`: 数据库用户名
* `password`: 数据库密码
* `database_name`: 要连接的数据库名称
**逻辑分析:**
1. 使用 `mysqli` 类创建新的 MySQL 连接。
2. 提供数据库服务器地址、用户名、密码和数据库名称作为参数。
3. 使用 `connect_error` 属性检查连接是否成功。
#### 断开数据库连接
```php
<?php
// 断开 MySQL 连接
$conn->close();
?>
```
**逻辑分析:**
1. 调用 `close()` 方法关闭 MySQL 连接。
### 2.2 SQL语句基础
#### 查询数据
```php
<?php
// 查询数据
$result = $conn->query("SELECT * FROM table_name");
// 检查查询是否成功
if (!$result) {
die("查询失败: " . $conn->error);
}
?>
```
**参数说明:**
* `table_name`: 要查询的表名
**逻辑分析:**
1. 使用 `query()` 方法执行 SQL 查询。
2. 将查询结果存储在 `$result` 变量中。
3. 使用 `error` 属性检查查询是否成功。
#### 插入数据
```php
<?php
// 插入数据
$sql = "INSERT INTO table_name (column1, column2) VALUES ('value1', 'value2')";
$result = $conn->query($sql);
// 检查插入是否成功
if (!$result) {
die("插入失败: " . $conn->error);
}
?>
```
**参数说明:**
* `table_name`: 要插入数据的表名
* `column1`, `column2`: 要插入数据的列名
* `value1`, `value2`: 要插入的数据值
**逻辑分析:**
1. 准备 SQL 插入语句。
2. 使用 `query()` 方法执行插入语句。
3. 将插入结果存储在 `$result` 变量中。
4. 使用 `error` 属性检查插入是否成功。
### 2.3 数据查询与操作
#### 遍历查询结果
```php
<?php
// 遍历查询结果
while ($row = $result->fetch_assoc()) {
// 访问结果中的每一行
echo $row['column_name'];
}
?>
```
**参数说明:**
* `$result`: 查询结果对象
**逻辑分析:**
1. 使用 `fetch_assoc()` 方法获取查询结果的每一行。
2. 将每一行存储在 `$row` 数组中。
3. 使用 `$row['column_name']` 访问每一行的列值。
#### 更新数据
```php
<?php
// 更新数据
$sql = "UPDATE table_name SET column1 = 'new_value' WHERE id = 1";
$result = $conn->query($sql);
// 检查更新是否成功
if (!$result) {
die("更新失败: " . $conn->error);
}
?>
```
**参数说明:**
* `table_name`: 要更新数据的表名
* `column1`: 要更新的列名
* `new_value`: 要更新的数据值
* `id`: 更新条件
**逻辑分析:**
1. 准备 SQL 更新语句。
2. 使用 `query()` 方法执行更新语句。
3. 将更新结果存储在 `$result` 变量中。
4. 使用 `error` 属性检查更新是否成功。
#### 删除数据
```php
<?php
// 删除数据
$sql = "DELETE FROM table_name WHERE id = 1";
$result = $conn->query($sql);
// 检查删除是否成功
if (!$result) {
die("删除失败: " . $conn->error);
}
?>
```
**参数说明:**
* `table_name`: 要删除数据的表名
* `id`: 删除条件
**逻辑分析:**
1. 准备 SQL 删除语句。
2. 使用 `query()` 方法执行删除语句。
3. 将删除结果存储在 `$result` 变量中。
4. 使用 `error` 属性检查删除是否成功。
# 3.1 事务处理
事务是数据库中一组原子操作的集合,要么全部成功,要么全部失败。事务处理可以保证数据库数据的完整性和一致性。
### 事务的特性
事务具有以下特性:
- **原子性(Atomicity):**事务中的所有操作要么全部成功,要么全部失败。
- **一致性(Consistency):**事务执行后,数据库必须处于一个一致的状态,即满足所有业务规则。
- **隔离性(Isolation):**并发执行的事务之间是相互隔离的,一个事务的执行不会影响其他事务。
- **持久性(Durability):**一旦事务提交,其对数据库的修改将永久保存,即使系统发生故障。
### 事务的隔离级别
数据库系统提供不同的隔离级别来控制事务之间的隔离程度。常见的隔离级别包括:
| 隔离级别 | 描述 |
|---|---|
| **Read Uncommitted** | 事务可以读取其他未提交事务的数据。 |
| **Read Committed** | 事务只能读取已提交事务的数据。 |
| **Repeatable Read** | 事务可以读取已提交事务的数据,但其他事务不能在该事务执行期间修改这些数据。 |
| **Serializable** | 事务可以读取已提交事务的数据,并且其他事务不能在该事务执行期间修改这些数据,也不能插入与该事务读取的数据冲突的新数据。 |
### 事务的实现
在 PHP 中,可以使用 `PDO` 扩展来实现事务处理。以下代码示例演示了如何使用 `PDO` 开始、提交和回滚事务:
```php
<?php
$pdo = new PDO('mysql:host=localhost;dbname=test', 'root', 'password');
// 开始事务
$pdo->beginTransaction();
// 执行查询或更新操作
// 提交事务
$pdo->commit();
// 回滚事务
$pdo->rollBack();
?>
```
### 事务处理的应用场景
事务处理在以下场景中非常有用:
- **确保数据一致性:**当需要确保多个操作要么全部成功,要么全部失败时,可以使用事务处理。
- **防止并发冲突:**当多个用户同时访问数据库时,可以使用事务处理来防止并发冲突。
- **提高性能:**在某些情况下,使用事务处理可以提高性能,因为可以减少数据库操作的次数。
# 4. PHP数据库实战应用
### 4.1 用户管理系统
#### 4.1.1 用户注册
用户注册是用户管理系统中的基本功能。以下是一个简单的用户注册代码示例:
```php
<?php
// 连接数据库
$conn = new mysqli("localhost", "root", "password", "database");
// 获取表单数据
$username = $_POST['username'];
$password = $_POST['password'];
$email = $_POST['email'];
// 验证表单数据
if (empty($username) || empty($password) || empty($email)) {
echo "请填写所有字段。";
exit;
}
// 检查用户名是否已存在
$sql = "SELECT * FROM users WHERE username = ?";
$stmt = $conn->prepare($sql);
$stmt->bind_param("s", $username);
$stmt->execute();
$result = $stmt->get_result();
if ($result->num_rows > 0) {
echo "用户名已存在。";
exit;
}
// 插入新用户
$sql = "INSERT INTO users (username, password, email) VALUES (?, ?, ?)";
$stmt = $conn->prepare($sql);
$stmt->bind_param("sss", $username, $password, $email);
$stmt->execute();
// 关闭连接
$stmt->close();
$conn->close();
// 注册成功,跳转到登录页面
header("Location: login.php");
?>
```
**代码逻辑分析:**
1. 连接数据库。
2. 获取表单数据。
3. 验证表单数据。
4. 检查用户名是否已存在。
5. 插入新用户。
6. 关闭连接。
7. 注册成功,跳转到登录页面。
**参数说明:**
* `$conn`:数据库连接对象。
* `$username`:用户名。
* `$password`:密码。
* `$email`:邮箱地址。
#### 4.1.2 用户登录
用户登录是用户管理系统中的另一个基本功能。以下是一个简单的用户登录代码示例:
```php
<?php
// 连接数据库
$conn = new mysqli("localhost", "root", "password", "database");
// 获取表单数据
$username = $_POST['username'];
$password = $_POST['password'];
// 验证表单数据
if (empty($username) || empty($password)) {
echo "请填写所有字段。";
exit;
}
// 检查用户是否存在
$sql = "SELECT * FROM users WHERE username = ?";
$stmt = $conn->prepare($sql);
$stmt->bind_param("s", $username);
$stmt->execute();
$result = $stmt->get_result();
if ($result->num_rows == 0) {
echo "用户不存在。";
exit;
}
// 验证密码
$user = $result->fetch_assoc();
if (!password_verify($password, $user['password'])) {
echo "密码错误。";
exit;
}
// 登录成功,跳转到主页
header("Location: index.php");
?>
```
**代码逻辑分析:**
1. 连接数据库。
2. 获取表单数据。
3. 验证表单数据。
4. 检查用户是否存在。
5. 验证密码。
6. 登录成功,跳转到主页。
**参数说明:**
* `$conn`:数据库连接对象。
* `$username`:用户名。
* `$password`:密码。
# 5.1 数据库索引
数据库索引是一种数据结构,它可以快速查找和检索数据,从而提高数据库查询性能。索引通过创建指向数据表中特定列或列组合的指针来实现这一目标。
### 索引类型
MySQL支持多种索引类型,包括:
- **B-Tree索引:**最常用的索引类型,它使用平衡树结构来存储数据。
- **哈希索引:**使用哈希函数将数据映射到索引项,从而实现快速查找。
- **全文索引:**用于对文本数据进行搜索,支持模糊查询和全文搜索。
### 索引创建
要创建索引,可以使用以下语法:
```sql
CREATE INDEX index_name ON table_name (column_name);
```
例如,要为`users`表中的`username`列创建索引,可以使用以下语句:
```sql
CREATE INDEX username_index ON users (username);
```
### 索引选择
选择要创建的索引时,需要考虑以下因素:
- **查询模式:**索引应该针对最常见的查询模式进行优化。
- **数据分布:**索引应该针对数据分布进行优化,例如,如果数据分布不均匀,可以使用哈希索引。
- **列选择:**索引应该创建在经常用于查询或连接的列上。
### 索引优化
索引可以优化数据库性能,但如果索引过多或不当,也会导致性能下降。因此,需要定期检查和优化索引:
- **删除未使用索引:**删除不再使用的索引,以减少数据库开销。
- **重建索引:**随着时间的推移,索引可能会变得碎片化,需要重建以提高性能。
- **监控索引使用情况:**使用数据库监控工具监控索引使用情况,并根据需要进行调整。
0
0
相关推荐
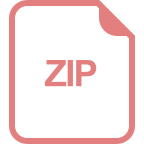
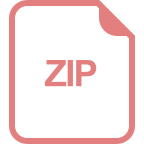





