算法面试通关秘籍:快速掌握常见算法题目的解题思路
发布时间: 2024-09-10 19:50:25 阅读量: 69 订阅数: 37 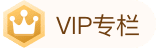
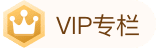
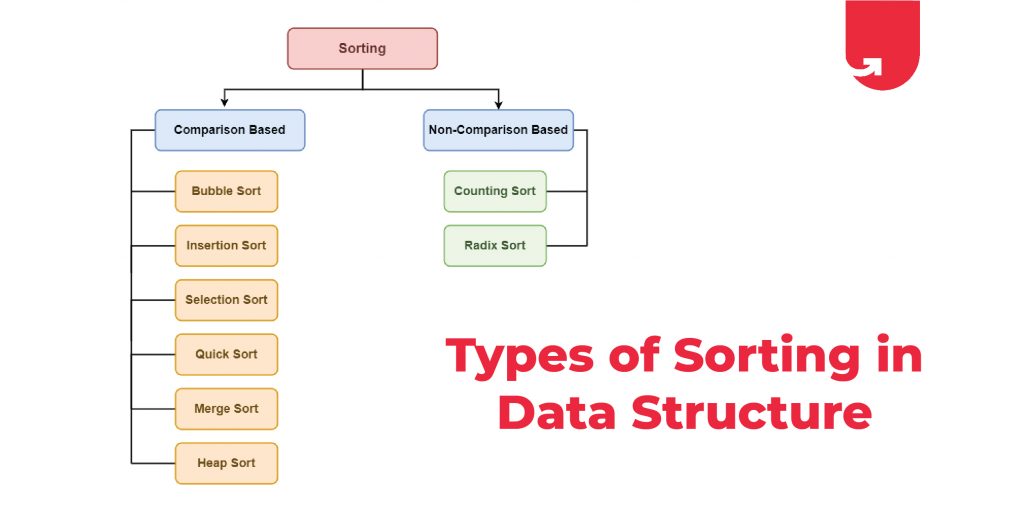
# 1. 算法面试概述
## 算法面试的重要性
算法面试是IT行业招聘流程中的核心环节,尤其对于数据科学、软件开发和系统架构师等职位至关重要。它不仅考察应聘者对算法知识的掌握程度,还反映了其解决复杂问题的逻辑思维能力和编码实践能力。
## 算法面试的准备
准备算法面试需要系统地学习常见的数据结构和算法,并且通过大量练习来熟悉不同问题的解法。这包括掌握时间复杂度和空间复杂度的概念,以及常见的算法优化技巧。
## 面试中的沟通技巧
在算法面试过程中,清晰地表达自己的思路和解题步骤同样重要。良好的沟通能力可以帮助面试官更好地理解你的解题思路,有时候即使算法实现有误,清晰的沟通也可能为你赢得加分。
# 2. 数据结构基础
## 2.1 线性结构
### 2.1.1 数组与字符串的操作技巧
数组与字符串是编程中最基础也是最常见的线性结构,广泛应用于各种算法问题中。数组是一种线性数据结构,可以存储多个相同类型的数据。而字符串可以看作是字符数组的一种特殊形式。
#### 数组的基本操作
数组操作的核心是索引访问和数据更新。在大多数编程语言中,数组都是通过下标(索引)来访问特定元素,索引通常从0开始。如在C++、Java或Python中,可以通过简单的索引操作来访问或修改数组元素。
```python
arr = [10, 20, 30, 40, 50]
# 访问第三个元素
print(arr[2]) # 输出:30
# 修改第三个元素
arr[2] = 35
# 输出修改后的数组
print(arr) # 输出:[10, 20, 35, 40, 50]
```
数组的静态分配和连续内存存储使它可以高效地进行随机访问,但其大小在初始化后通常不能动态改变,这在处理未知或变化大小的数据集时会带来局限性。这时可以考虑使用动态数组(如Python中的list),它支持动态扩容。
#### 字符串操作技巧
字符串在处理文本数据时尤其重要。许多编程语言提供了丰富的字符串操作函数库。在许多算法问题中,字符串处理是关键技能之一,尤其是涉及到模式匹配和字符串分析的问题。
```python
# 字符串连接
s1 = "Hello"
s2 = "World"
s = s1 + " " + s2 # 使用加号连接字符串
# 字符串切片
print(s[0:5]) # 输出:Hello
# 字符串长度
print(len(s)) # 输出:11
# 查找子串位置
pos = s.find("World") # 输出:6
# 字符串替换
s = s.replace("World", "Python") # 输出:Hello Python
```
字符串操作中还需要注意到一些特性,如不可变性(字符串一旦创建,其内容不可改变,修改字符串实际上是创建了一个新的字符串)以及常见的编码问题(如Unicode编码处理)。
### 2.1.2 链表的遍历与常用算法
链表是一种线性结构,由一系列节点组成,每个节点包含数据部分和指向下一个节点的指针。链表相比数组,内存分布不需要连续,因此具有更好的动态性。不过,由于访问数据需要遍历链表,所以在性能上可能不如数组。
#### 链表基本概念
链表通常可以分为单向链表、双向链表和循环链表。单向链表的节点只包含一个指向下一个节点的指针,而双向链表则有指向前一个节点和后一个节点的指针。循环链表的最后一个节点的指针指向头节点。
```python
class ListNode:
def __init__(self, value=0, next=None):
self.value = value
self.next = next
# 创建链表
node1 = ListNode(1)
node2 = ListNode(2)
node3 = ListNode(3)
node1.next = node2
node2.next = node3
```
#### 链表的遍历
遍历链表是链表操作的基础,遍历过程中通常需要使用两个指针,一个指向当前访问的节点,另一个指向下一次访问的节点。
```python
def traverse(head):
current = head
while current is not None:
print(current.value)
current = current.next
```
在遍历链表时,我们还可以实现一些高级操作,比如链表的排序、搜索特定值、插入节点、删除节点等。实现这些算法时,我们需要注意保持链表结构的完整性和指针的正确性。
#### 链表的常用算法
**反转链表**:反转链表是面试中的常考题。在反转时,需要注意调整节点的指向,同时要维护好前一个节点的引用。
```python
def reverse_list(head):
prev, current = None, head
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev
```
**链表的中间节点**:找到链表的中间节点常用于判断链表的奇偶长度以及快慢指针技巧中。
```python
def find_middle(head):
slow = head
fast = head
while fast and fast.next:
slow = slow.next
fast = fast.next.next
return slow
```
链表的其他常用算法还包括合并两个已排序链表、检测环路等,这些都需要在面试中重点掌握。
## 2.2 栈和队列
### 2.2.1 栈的基本概念与应用
栈是一种后进先出(Last In First Out, LIFO)的数据结构,只能在一端(通常称为栈顶)添加或移除元素。栈的这一特点使得它非常适合实现撤销操作、深度优先搜索等算法。
#### 栈的基本操作
在栈中,有两个基本操作:
- `push`:在栈顶添加一个元素。
- `pop`:移除栈顶元素并返回该元素。
```python
class Stack:
def __init__(self):
self.stack = []
def push(self, item):
self.stack.append(item)
def pop(self):
if self.is_empty():
return None
return self.stack.pop()
def is_empty(self):
return len(self.stack) == 0
```
#### 栈的应用实例
栈的一个典型应用是在表达式求值中,比如计算后缀表达式(逆波兰表达式)。在这个过程中,我们遍历表达式的每个符号,如果遇到数字则压入栈中,遇到运算符则从栈中弹出两个数字进行运算,并将结果压回栈中。
```python
def eval_postfix(expression):
stack = Stack()
for item in expression:
if item.isdigit():
stack.push(int(item))
else:
right = stack.pop()
left = stack.pop()
if item == '+':
stack.push(left + right)
elif item == '-':
stack.push(left - right)
elif item == '*':
stack.push(left * right)
elif item == '/':
stack.push(left / right)
return stack.pop()
```
### 2.2.2 队列及其在算法中的使用
队列是一种先进先出(First In First Out, FIFO)的数据结构,与栈刚好相反,在队列中,最早进入的元素将最先被访问。
#### 队列的基本操作
队列的操作主要包括:
- `enqueue`:在队列尾部添加一个元素。
- `dequeue`:移除队列头部的元素并返回该元素。
```python
class Queue:
def __init__(self):
self.queue = []
def enqueue(self, item):
self.queue.append(item)
def dequeue(self):
if self.is_empty():
return None
return self.queue.pop(0)
def is_empty(self):
return len(self.queue) == 0
```
#### 队列在算法中的应用
队列在算法中的典型应用包括广度优先搜索(Breadth-First Search, BFS)。在BFS中,我们使用队列来追踪待访问的节点,通常是在树或图的遍历中。
```python
def bfs_traversal(graph, start_node):
visited = set()
queue = Queue()
queue.enqueue(start_node)
while not queue.is_empty():
current_node = queue.dequeue()
if current_node not in visited:
visited.add(current_node)
queue.enqueue(current_node) # 对于树,这一行可以省略
# 遍历当前节点的邻居
for neighbor in graph[current_node]:
if neighbor not in visited:
queue.enqueue(neighbor)
return visited
```
通过以上实例可以看到,栈和队列的操作虽然简单,但在算法中发挥着重要的作用。掌握它们的基本概念和应用,对于处理复杂算法问题至关重要。
# 3. 排序与搜索算法
## 3.1 排序算法精讲
### 3.1.1 快速排序与归并排序
快速排序和归并排序都是高效的比较排序算法。它们的平均时间复杂度均为O(n log n),并且在实际应用中表现优秀。但在内部实现机制上有很大的差异。快速排序是一种分治策略的实现,它通过一个划分操作将数组分为两个子数组,一个子数组的所有元素都比另一个小。归并排序则是通过递归将数组分成更小的数组,然后合并这些小数组,使得合并后的数组有序。
快速排序的核心在于划分过程。在划分过程中,选定一个基准元素(pivot),然后将数组分为两部分:一部分的所有元素小于等于基准元素,另一部分的所有元素大于等于基准元素。划分操作的关键是选择合适的基准元素和处理重复元素。
归并排序的归并过程是实现的关键。在每次合并操作中,两个有序数组被合并成一个更大的有序数组。这个过程不断递归,直到所有子数组只剩一个元素,然后逐步合并回上一层。
下面是快速排序和归并排序的Python代码示例,并附带注释解析:
```python
# 快速排序的Python实现
def quick_sort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left =
```
0
0
相关推荐
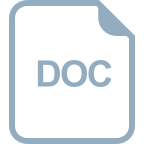
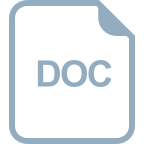
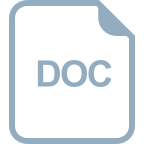
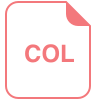
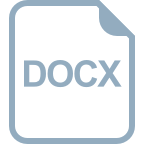
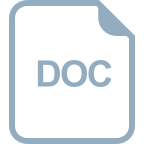
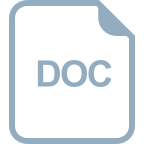
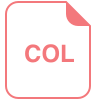
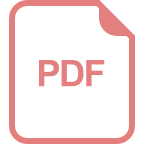