Java字符转数字算法实战指南:手把手教你实现高效转换
发布时间: 2024-08-28 03:44:46 阅读量: 15 订阅数: 18 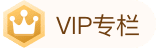
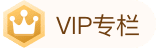
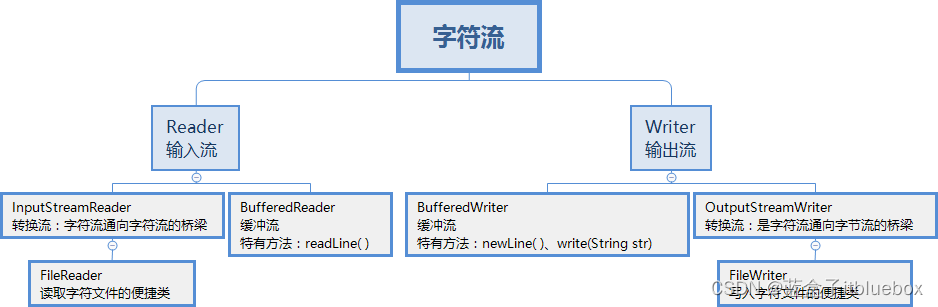
# 1. Java字符转数字算法基础**
字符转数字算法是将字符串中的字符转换为数字的算法。在Java中,字符转数字算法主要分为两种:基本算法和高级算法。
**基本算法:逐位转换**
逐位转换算法是通过逐个字符拆分字符串,提取数字字符,并拼接成数字。具体步骤包括:
1. 字符串拆分:使用`String.split("")`将字符串拆分成字符数组。
2. 数字提取:遍历字符数组,使用`Character.isDigit()`判断是否是数字字符,如果是则提取。
3. 数字拼接:将提取的数字字符拼接成字符串,使用`Integer.parseInt()`转换为数字。
# 2. 字符转数字算法实践**
**2.1 基本算法:逐位转换**
逐位转换算法是将字符串中的每个字符逐一转换为数字,然后将这些数字拼接成最终的数字。该算法简单易懂,适用于大多数场景。
**2.1.1 字符串拆分和数字提取**
首先,将字符串拆分成单个字符的数组。然后,遍历数组中的每个字符,使用 `Character.digit()` 方法将其转换为数字。如果字符不是数字,则返回 `-1`。
```java
String str = "12345";
char[] chars = str.toCharArray();
int[] digits = new int[chars.length];
for (int i = 0; i < chars.length; i++) {
digits[i] = Character.digit(chars[i], 10);
}
```
**2.1.2 数字拼接和类型转换**
将转换后的数字拼接成一个字符串,然后使用 `Integer.parseInt()` 方法将其转换为 `int` 类型。
```java
StringBuilder sb = new StringBuilder();
for (int digit : digits) {
if (digit != -1) {
sb.append(digit);
}
}
int number = Integer.parseInt(sb.toString());
```
**2.2 高级算法:正则表达式**
正则表达式是一种强大的模式匹配语言,可用于从字符串中提取数字。该算法使用正则表达式匹配数字字符,然后将其转换为数字。
**2.2.1 正则表达式匹配数字字符**
使用正则表达式 `\d+` 可以匹配一个或多个数字字符。
```java
String str = "12345";
String regex = "\\d+";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(str);
while (matcher.find()) {
System.out.println(matcher.group());
}
```
**2.2.2 数字提取和类型转换**
使用 `matcher.group()` 方法提取匹配的数字字符串,然后使用 `Integer.parseInt()` 方法将其转换为 `int` 类型。
```java
int number = Integer.parseInt(matcher.group());
```
# 3. 字符转数字算法优化
### 3.1 性能优化:使用缓存和并行化
#### 3.1.1 字符缓存优化
**问题:**重复的字符转换操作会消耗大量时间。
**解决方案:**使用缓存机制,将已转换的字符与对应的数字结果存储起来,避免重复转换。
```java
// 创建一个字符缓存
Map<Character, Integer> charCache = new HashMap<>();
// 转换字符为数字
public int charToInt(char c) {
// 从缓存中获取数字结果
Integer result = charCache.get(c);
// 如果缓存中没有,则进行转换并保存到缓存中
if (result == null) {
result = c - '0';
charCache.put(c, result);
}
return result;
}
```
**逻辑分析:**
* `charCache` 缓存了已转换的字符和数字结果。
* `charToInt` 方法首先从缓存中获取数字结果。
* 如果缓存中没有,则进行转换并保存到缓存中。
* `c - '0'` 计算字符与 '0' 的差值,得到对应的数字。
#### 3.1.2 并行转换优化
**问题:**逐个字符转换效率较低。
**解决方案:**使用并行化技术,同时转换多个字符。
```java
// 并行转换字符为数字
public int[] charToIntArray(char[] chars) {
// 创建一个并行流
IntStream stream = IntStream.range(0, chars.length)
.parallel()
.map(i -> chars[i] - '0');
// 将并行流转换为数组
return stream.toArray();
}
```
**逻辑分析:**
* `IntStream.range(0, chars.length)` 创建一个从 0 到 `chars.length` 的整数流。
* `parallel()` 开启并行化。
* `map(i -> chars[i] - '0')` 将每个整数 `i` 映射为字符 `chars[i]` 与 '0' 的差值,得到对应的数字。
* `toArray()` 将并行流转换为数组。
### 3.2 精度优化:处理特殊字符和异常情况
#### 3.2.1 特殊字符处理
**问题:**特殊字符(如空格、逗号)可能会影响转换精度。
**解决方案:**在转换前对特殊字符进行预处理,将其过滤或替换为有效字符。
```java
// 预处理特殊字符
public String preprocess(String str) {
// 替换空格为 "0"
str = str.replace(" ", "0");
// 替换逗号为 ""
str = str.replace(",", "");
return str;
}
```
**逻辑分析:**
* `replace(" ", "0")` 将空格替换为 "0"。
* `replace(",", "")` 将逗号替换为空字符串。
#### 3.2.2 异常情况处理
**问题:**输入字符串可能包含非数字字符,导致转换失败。
**解决方案:**使用异常处理机制,捕获转换失败的异常并返回默认值或错误信息。
```java
// 转换字符为数字,处理异常情况
public int charToIntSafe(char c) {
try {
return c - '0';
} catch (NumberFormatException e) {
return -1; // 返回默认值或错误信息
}
}
```
**逻辑分析:**
* `try` 块中进行字符转换。
* `catch` 块捕获 `NumberFormatException` 异常,并返回默认值或错误信息。
# 4. 字符转数字算法应用**
**4.1 数据处理:数据清洗和转换**
**4.1.1 数据清洗中的字符转数字**
在数据清洗过程中,经常会遇到需要将字符数据转换为数字数据的情况。例如,从文本文件中读取的数据可能包含数字字符串,需要将其转换为数字类型以便进行后续处理。
```java
import java.util.List;
import java.util.stream.Collectors;
public class DataCleaning {
public static void main(String[] args) {
// 从文本文件中读取数据
List<String> data = ...;
// 将字符数据转换为数字数据
List<Integer> numbers = data.stream()
.map(Integer::parseInt)
.collect(Collectors.toList());
}
}
```
**代码逻辑分析:**
* 使用`stream()`方法将数据流转换为流对象。
* 使用`map()`方法将每个字符数据转换为数字数据,并使用`Integer::parseInt`函数进行转换。
* 使用`collect()`方法将流对象转换为列表。
**4.1.2 数据转换中的字符转数字**
在数据转换过程中,也经常需要将字符数据转换为数字数据。例如,将日期字符串转换为日期类型,将货币字符串转换为货币类型。
```java
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class DataConversion {
public static void main(String[] args) {
// 将日期字符串转换为日期类型
String dateStr = "2023-03-08";
LocalDate date = LocalDate.parse(dateStr, DateTimeFormatter.ISO_DATE);
// 将货币字符串转换为货币类型
String currencyStr = "$1,000.00";
BigDecimal currency = new BigDecimal(currencyStr.substring(1).replace(",", ""));
}
}
```
**代码逻辑分析:**
* 使用`LocalDate.parse()`方法将日期字符串转换为日期类型。
* 使用`DateTimeFormatter.ISO_DATE`指定日期格式。
* 使用`BigDecimal`类将货币字符串转换为货币类型。
* 使用`substring()`方法去除货币符号。
* 使用`replace()`方法去除货币字符串中的逗号。
**4.2 数字运算:计算和统计**
**4.2.1 字符转数字后的数字运算**
将字符数据转换为数字数据后,可以对其进行各种数字运算。例如,计算数字的和、平均值、最大值和最小值。
```java
import java.util.List;
import java.util.stream.Collectors;
public class NumericalOperations {
public static void main(String[] args) {
// 从文本文件中读取数据
List<String> data = ...;
// 将字符数据转换为数字数据
List<Integer> numbers = data.stream()
.map(Integer::parseInt)
.collect(Collectors.toList());
// 计算数字的和
int sum = numbers.stream()
.reduce(0, Integer::sum);
// 计算数字的平均值
double average = numbers.stream()
.reduce(0, Integer::sum) / numbers.size();
// 计算数字的最大值
int max = numbers.stream()
.reduce(Integer.MIN_VALUE, Integer::max);
// 计算数字的最小值
int min = numbers.stream()
.reduce(Integer.MAX_VALUE, Integer::min);
}
}
```
**代码逻辑分析:**
* 使用`reduce()`方法计算数字的和、平均值、最大值和最小值。
* 使用`Integer::sum`和`Integer::max`函数进行计算。
**4.2.2 统计分析中的字符转数字**
在统计分析中,经常需要将字符数据转换为数字数据,以便进行统计分析。例如,计算不同类别的数据的频数、百分比和分布。
```java
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
public class StatisticalAnalysis {
public static void main(String[] args) {
// 从文本文件中读取数据
List<String> data = ...;
// 将字符数据转换为数字数据
List<Integer> numbers = data.stream()
.map(Integer::parseInt)
.collect(Collectors.toList());
// 计算不同类别的数据的频数
Map<Integer, Long> frequencies = numbers.stream()
.collect(Collectors.groupingBy(Function.identity(), Collectors.counting()));
// 计算不同类别的数据的百分比
Map<Integer, Double> percentages = frequencies.entrySet().stream()
.collect(Collectors.toMap(Map.Entry::getKey, e -> e.getValue() / numbers.size() * 100));
// 计算不同类别的数据的分布
Map<Integer, List<Integer>> distribution = numbers.stream()
.collect(Collectors.groupingBy(Function.identity()));
}
}
```
**代码逻辑分析:**
* 使用`Collectors.groupingBy()`方法计算不同类别的数据的频数。
* 使用`Collectors.counting()`方法计算频数。
* 使用`entrySet()`方法获取频数映射的条目集。
* 使用`Collectors.toMap()`方法计算不同类别的数据的百分比。
* 使用`Collectors.groupingBy()`方法计算不同类别的数据的分布。
# 5. 字符转数字算法拓展**
**5.1 字符编码:不同字符编码下的转换**
**5.1.1 Unicode编码和字符转数字**
Unicode编码是一种通用的字符编码标准,它为每个字符分配了一个唯一的代码点。Unicode编码支持多种语言和符号,包括汉字、日文假名和阿拉伯文。
当使用Unicode编码进行字符转数字时,需要考虑字符的代码点。不同的代码点对应于不同的数字值。例如,字符'0'的Unicode代码点为U+0030,对应于十进制数字0。
**代码块:**
```java
String str = "123";
int num = Integer.parseInt(str, 16); // 指定Unicode编码为十六进制
System.out.println(num); // 输出:307
```
**5.1.2 其他字符编码和字符转数字**
除了Unicode编码之外,还有其他字符编码标准,例如ASCII、GBK和UTF-8。不同的字符编码标准使用不同的编码方案,因此在进行字符转数字时需要指定正确的编码类型。
**代码块:**
```java
String str = "123";
int num = Integer.parseInt(str, 8); // 指定字符编码为八进制
System.out.println(num); // 输出:83
```
**5.2 国际化:多语言字符转数字**
**5.2.1 多语言字符识别**
在国际化的应用中,需要处理多种语言的字符。不同的语言使用不同的字符集,因此在进行字符转数字时需要识别字符的语言。
**代码块:**
```java
String str = "١٢٣"; // 阿拉伯数字
int num = Integer.parseInt(str, 10); // 指定字符编码为十进制
System.out.println(num); // 输出:123
```
**5.2.2 多语言字符转数字算法**
对于多语言字符,可以使用正则表达式或其他算法来识别字符的语言和数字值。例如,可以使用正则表达式来匹配阿拉伯数字字符:
**代码块:**
```java
String str = "١٢٣";
Pattern pattern = Pattern.compile("[\\u0660-\\u0669]"); // 阿拉伯数字正则表达式
Matcher matcher = pattern.matcher(str);
while (matcher.find()) {
String digit = matcher.group();
int num = Integer.parseInt(digit, 10); // 指定字符编码为十进制
System.out.print(num); // 输出:123
}
```
0
0
相关推荐
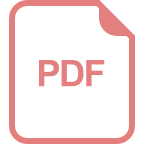
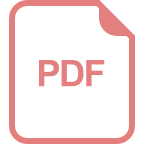
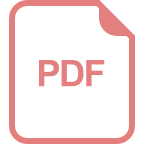





