双链表与Java集合框架的比较:性能与适用场景分析,构建高效缓存系统
发布时间: 2024-09-11 09:42:20 阅读量: 72 订阅数: 37 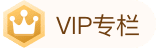
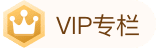
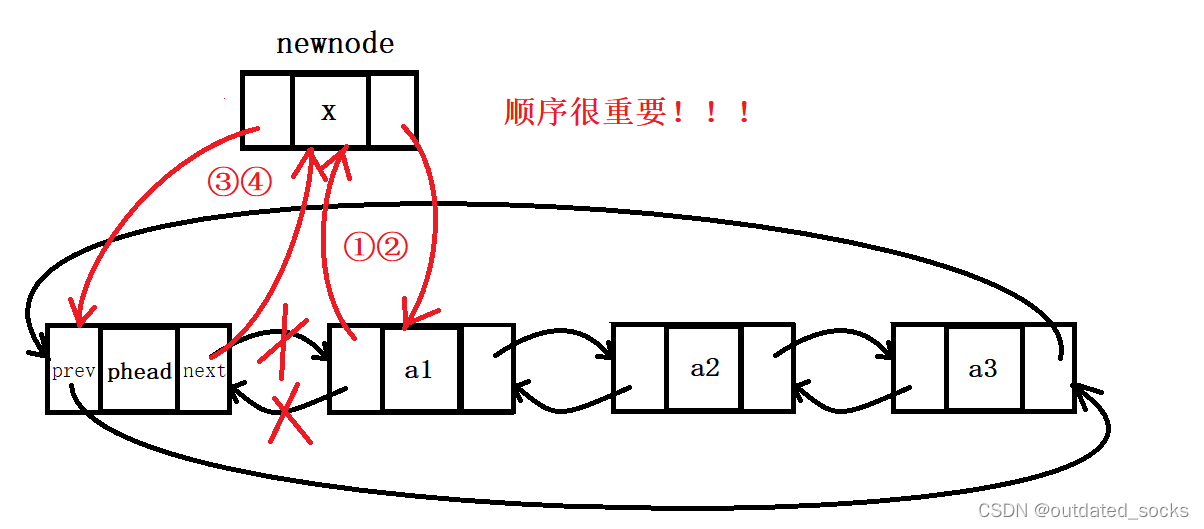
# 1. 数据结构基础 - 双链表与集合框架概述
## 1.1 数据结构与程序性能
在IT行业中,数据结构作为基础,是程序设计的核心。其选择往往直接决定了程序的效率和性能。在众多数据结构中,双链表和集合框架是两种常见的数据结构,它们各自有着独特的特性与适用场景。接下来,我们将探讨双链表与Java集合框架的基础知识,为后续章节深入分析打下坚实的基础。
## 1.2 双链表简介
双链表是一种线性数据结构,相较于单链表,它允许双向遍历,即可以从任一节点向前或向后访问。其内部由节点组成,每个节点都存储了数据以及指向前一个和后一个节点的引用。这种结构使得双链表在插入和删除操作中具有较高的效率,特别是在数据量较大且需要频繁进行此类操作的场景下。
## 1.3 Java集合框架概览
Java集合框架是一组为了表示和操作集合而设计的接口和类。它提供了一套丰富的数据结构实现,包括List、Set和Map等。这些集合类型能够容纳并管理不同类型的数据,提供了众多便利的操作方法,极大地简化了数据操作的复杂度。本文将介绍Java集合框架的基础知识,为接下来的深入探讨构建坚实的理论基础。
# 2. 双链表的内部实现与应用
双链表是一种常见的数据结构,其在计算机科学中有着广泛的应用。它的两个关键特性是节点之间的双向连接和对头尾操作的高效性,使得双链表特别适合实现如队列和栈这类需要频繁在两端添加或删除元素的数据结构。
### 2.1 双链表的概念与特性
#### 2.1.1 双链表的数据结构定义
双链表由一系列节点构成,每个节点都包含三个部分:数据域、前驱指针和后继指针。数据域存储节点实际的数据,前驱指针指向节点的前一个节点,后继指针则指向节点的后一个节点。这种结构使得在双链表中可以从任何一个节点出发,按照一个方向遍历到链表的任意一个节点。
#### 2.1.2 双链表的操作:插入、删除与遍历
双链表的操作包括插入、删除和遍历。在双链表中插入一个新节点可以发生在链表的任何位置,而在删除节点时,需要考虑被删除节点前后的节点关系。遍历双链表可以通过前驱或后继指针进行,可以实现双向遍历,即可以从头遍历到尾,也可以从尾遍历到头。
### 2.2 双链表在Java中的实现
#### 2.2.1 Java中的LinkedList类
Java中提供了`LinkedList`类来实现双链表的数据结构。它位于`java.util`包中,支持在列表的中间插入和移除元素,其内部使用`Node`类来表示链表中的每个节点。
```java
public class LinkedList<E> extends AbstractSequentialList<E> implements List<E>, Deque<E>, Cloneable, Serializable {
// ...
private static class Node<E> {
E item;
LinkedList.Node<E> next;
LinkedList.Node<E> prev;
Node(LinkedList.Node<E> prev, E element, LinkedList.Node<E> next) {
this.item = element;
this.next = next;
this.prev = prev;
}
}
// ...
}
```
#### 2.2.2 LinkedList的源码分析与性能考量
`LinkedList`类的源码主要由内部的`Node`类、构造器、常用方法如`add`、`remove`、`get`和`set`等构成。性能考量方面,对于频繁在两端进行插入和删除操作的场景,`LinkedList`提供了O(1)时间复杂度的性能优势。然而,由于链表的每个节点都需要额外存储指针信息,因此在空间占用上不如数组结构高效。
### 2.3 双链表的适用场景分析
#### 2.3.1 双链表与单链表的对比
双链表与单链表的主要区别在于双链表提供了双向遍历的能力。如果应用场景中对节点的前驱信息有频繁访问的需求,那么双链表将是更佳的选择。相对地,单链表的每个节点只保留了对下一个节点的引用,节省了内存空间,在内存占用较为敏感的环境下更为适用。
#### 2.3.2 双链表在缓存系统中的应用实例
在缓存系统中,双链表可以用来实现LRU(最近最少使用)缓存策略。利用双链表的特性,可以快速地将访问过的元素移动到链表头部,同时淘汰尾部的元素,实现快速的缓存更新和数据淘汰。
```java
public class LRUCache<K, V> {
private final int capacity;
private final Map<K, Node<K, V>> cache;
private final LinkedList<Node<K, V>> list;
public LRUCache(int capacity) {
this.capacity = capacity;
cache = new HashMap<>();
list = new LinkedList<>();
}
// ...
}
```
此代码块展示了双链表实现LRU缓存的一个简化的例子,包括`capacity`容量限制,`cache`映射和`list`双链表结构。
通过以上对双链表的内部实现与应用的探讨,我们了解了双链表作为数据结构的特性和在Java中的具体实现方式。接下来,我们将深入研究Java集合框架,并对比其与双链表的性能表现。
# 3. Java集合框架深度解析
Java集合框架是Java编程中不可或缺的一部分,为开发者提供了大量预先实现的数据结构。这些数据结构包括不同类型的List, Set, Map等接口,支持不同场景下的数据存储、管理和处理。深入了解Java集合框架的内部实现机制、特点和性能优化策略,对于编写高效、稳定的代码至关重要。
## 3.1 Java集合框架概述
### 3.1.1 集合框架的架构与分类
Java集合框架按结构可以分为两大类:Collection和Map。Collection集合主要用于存储单一对象,Map集合用于存储键值对。
- **Collection接口**:它是单个元素的集合,它有两个主要的子接口,List和Set。
- **List接口**:List是有序集合,可以包含重复元素,常用的实现类有ArrayList和LinkedList。
- **Set接口**:Set是不允许重复元素的集合,常用的实现类有HashSet、LinkedHashSet和TreeSet。
- **Map接口**:Map存储的是键值对,每个键最多映射到一个值,常用的
0
0
相关推荐
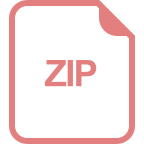
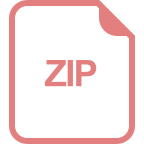
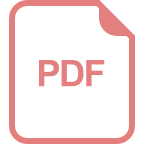
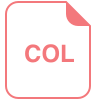
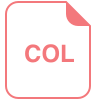
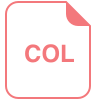
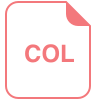

