【Python爬虫与Selenium的强强联合】:揭秘爬取网页数据的利器组合
发布时间: 2024-06-25 00:22:20 阅读量: 7 订阅数: 10 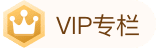
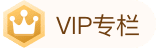
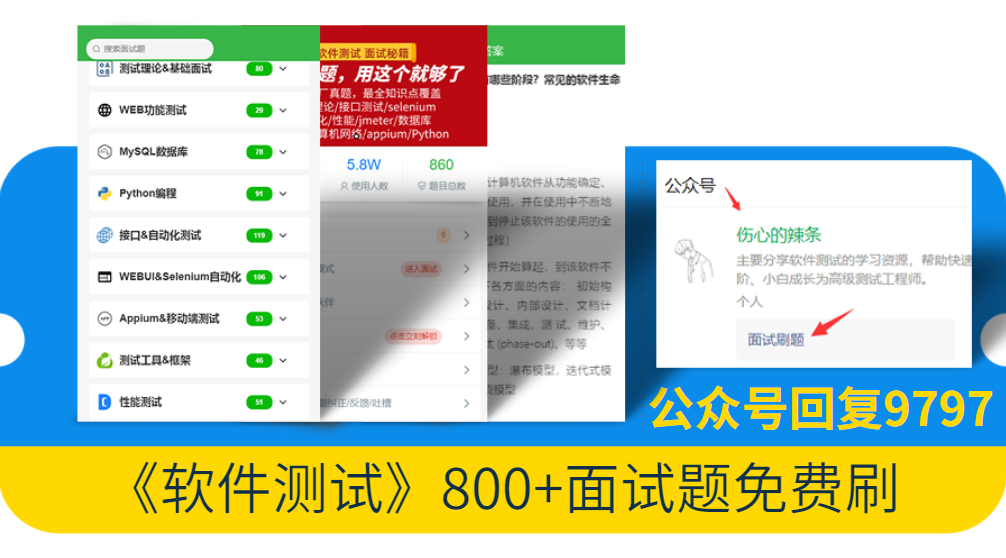
# 1. Python爬虫基础**
**1.1 Python爬虫简介**
Python爬虫是一种使用Python语言编写的程序,用于从网站上自动提取和解析数据。它广泛应用于网络数据采集、信息聚合和自动化测试等领域。
**1.2 常见爬虫库介绍**
Python提供了丰富的爬虫库,其中最常用的包括:
* **Beautiful Soup:**一个流行的HTML解析库,可轻松从HTML文档中提取数据。
* **Requests:**一个HTTP请求库,用于向网站发送请求并获取响应。
* **Scrapy:**一个功能强大的爬虫框架,提供了一系列用于构建和管理爬虫的工具。
# 2. Selenium自动化测试
### 2.1 Selenium简介
Selenium是一个用于自动化网页测试的开源框架。它允许您控制浏览器并执行各种操作,例如单击按钮、填写表单和验证页面内容。Selenium支持多种编程语言,包括Python。
### 2.2 Selenium的安装和使用
要安装Selenium,请使用以下命令:
```
pip install selenium
```
要使用Selenium,首先需要创建一个WebDriver对象。WebDriver对象代表一个浏览器实例,您可以使用它来控制浏览器。以下是如何创建一个WebDriver对象:
```python
from selenium import webdriver
driver = webdriver.Chrome()
```
### 2.3 Selenium的定位策略
Selenium提供了多种定位策略来查找网页元素。最常用的定位策略包括:
- **ID:**使用元素的ID属性。
- **名称:**使用元素的name属性。
- **类名:**使用元素的class属性。
- **XPath:**使用XPath表达式。
- **CSS选择器:**使用CSS选择器。
以下是如何使用ID定位策略查找元素:
```python
element = driver.find_element_by_id("my_id")
```
### 2.4 Selenium的交互操作
一旦找到元素,就可以使用Selenium执行各种交互操作。最常用的交互操作包括:
- **单击:**使用click()方法。
- **填写:**使用send_keys()方法。
- **获取文本:**使用text属性。
- **验证:**使用assert方法。
以下是如何使用Selenium单击按钮:
```python
button = driver.find_element_by_id("my_button")
button.click()
```
### 代码示例
以下是一个使用Selenium自动登录到网站的示例:
```python
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("https://www.example.com")
username_field = driver.find_element_by_id("username")
username_field.send_keys("my_username")
password_field = driver.find_element_by_id("password")
password_field.send_keys("my_password")
login_button = driver.find_element_by_id("login_button")
login_button.click()
assert "Welcome, my_username!" in driver.page_source
```
### 扩展性说明
**代码逻辑逐行解读:**
1. 使用WebDriver对象打开网站。
2. 使用ID定位策略找到用户名和密码字段。
3. 使用send_keys()方法填写用户名和密码。
4. 使用ID定位策略找到登录按钮。
5. 使用click()方法单击登录按钮。
6. 使用assert方法验证是否成功登录。
**参数说明:**
- **WebDriver对象:**代表浏览器实例。
- **find_element_by_id()方法:**用于根据ID定位元素。
- **send_keys()方法:**用于填写元素。
- **click()方法:**用于单击元素。
- **assert方法:**用于验证条件是否为真。
# 3. Python爬虫与Selenium整合
### 3.1 Python爬虫与Selenium的优势互补
Python爬虫和Selenium自动化测试工具各具优势,当两者结合使用时,可以发挥出强大的协同效应。
* **Python爬虫:**擅长处理大规模数据抓取,可以轻松获取大量网页内容,但对于动态网页和复杂交互操作支持不足。
* **Selenium:**专用于自动化测试,提供丰富的定位策略和交互操作,可以轻松处理动态网页和复杂表单提交等操作。
### 3.2 Selenium在Python爬虫中的应用场景
Selenium在Python爬虫中主要应用于以下场景:
* **动态网页数据的爬取:**Selenium可以模拟浏览器行为,加载并执行JavaScript,获取动态加载的数据。
* **复杂表单数据的提交:**Selenium可以模拟用户操作,填写并提交复杂表单,获取提交后的响应。
* **JavaScript渲染后的页面爬取:**Selenium可以等待JavaScript执行完毕,获取渲染后的页面内容,避免因JavaScript异步加载导致数据缺失。
### 3.3 Python爬虫与Selenium的集成方法
Python爬虫与Selenium集成主要有两种方法:
#### 3.3.1 使用Selenium WebDriver
Selenium WebDriver是一个跨语言的API,可以控制浏览器并执行自动化操作。Python爬虫可以通过`webdriver`模块集成Selenium WebDriver。
```python
from selenium import webdriver
# 创建一个Chrome浏览器驱动
driver = webdriver.Chrome()
# 访问目标网页
driver.get("https://example.com")
# 获取网页内容
html = driver.page_source
# 关闭浏览器
driver.quit()
```
#### 3.3.2 使用Selenium Grid
Selenium Grid是一个分布式测试框架,可以并行执行Selenium测试。Python爬虫可以通过`selenium-grid`模块集成Selenium Grid。
```python
from selenium.webdriver.remote.webdriver import WebDriver
from selenium.webdriver.remote.webdriver import DesiredCapabilities
# 创建一个远程WebDriver
desired_capabilities = DesiredCapabilities.CHROME
driver = WebDriver(command_executor="http://localhost:4444/wd/hub", desired_capabilities=desired_capabilities)
# 访问目标网页
driver.get("https://example.com")
# 获取网页内容
html = driver.page_source
# 关闭浏览器
driver.quit()
```
# 4. 实践应用
### 4.1 动态网页数据的爬取
动态网页数据是指通过JavaScript动态加载或渲染的网页内容,传统爬虫无法直接获取。Selenium可以模拟浏览器的行为,执行JavaScript代码,从而获取动态加载的内容。
**使用Selenium爬取动态网页数据的步骤:**
1. **加载页面:**使用`webdriver.get()`方法加载需要爬取的动态网页。
2. **等待页面加载完成:**使用`webdriver.implicitly_wait()`方法等待页面加载完成,确保JavaScript代码执行完毕。
3. **获取动态加载的内容:**使用`webdriver.find_element()`方法定位动态加载的内容,并使用`webdriver.text`或`webdriver.get_attribute()`方法获取内容。
**示例代码:**
```python
from selenium import webdriver
# 加载页面
driver = webdriver.Chrome()
driver.get("https://example.com")
# 等待页面加载完成
driver.implicitly_wait(10)
# 获取动态加载的内容
content = driver.find_element(By.ID, "dynamic_content").text
# 打印内容
print(content)
```
### 4.2 复杂表单数据的提交
复杂表单可能包含多种输入类型,如文本框、下拉列表、复选框等。Selenium可以模拟用户操作,填写表单并提交。
**使用Selenium提交复杂表单数据的步骤:**
1. **定位表单元素:**使用`webdriver.find_element()`方法定位表单中的每个输入元素。
2. **填写表单:**根据元素类型,使用`webdriver.send_keys()`方法填写文本框,使用`webdriver.select_by_visible_text()`方法选择下拉列表,使用`webdriver.click()`方法勾选复选框。
3. **提交表单:**使用`webdriver.find_element()`方法定位提交按钮,并使用`webdriver.click()`方法提交表单。
**示例代码:**
```python
from selenium import webdriver
# 加载页面
driver = webdriver.Chrome()
driver.get("https://example.com/form")
# 填写表单
driver.find_element(By.ID, "name").send_keys("John Doe")
driver.find_element(By.ID, "email").send_keys("john.doe@example.com")
driver.find_element(By.ID, "country").select_by_visible_text("United States")
driver.find_element(By.ID, "terms").click()
# 提交表单
driver.find_element(By.ID, "submit").click()
```
### 4.3 JavaScript渲染后的页面爬取
JavaScript渲染后的页面是指通过JavaScript动态生成和渲染的网页内容,传统爬虫无法直接获取。Selenium可以执行JavaScript代码,从而获取JavaScript渲染后的内容。
**使用Selenium爬取JavaScript渲染后的页面数据的步骤:**
1. **加载页面:**使用`webdriver.get()`方法加载需要爬取的JavaScript渲染后的页面。
2. **执行JavaScript代码:**使用`webdriver.execute_script()`方法执行JavaScript代码,获取渲染后的内容。
3. **获取渲染后的内容:**使用`webdriver.find_element()`方法定位渲染后的内容,并使用`webdriver.text`或`webdriver.get_attribute()`方法获取内容。
**示例代码:**
```python
from selenium import webdriver
# 加载页面
driver = webdriver.Chrome()
driver.get("https://example.com/js_rendered")
# 执行JavaScript代码
content = driver.execute_script("return document.getElementById('js_rendered_content').innerHTML")
# 打印内容
print(content)
```
# 5.1 反爬虫机制应对
### 5.1.1 识别反爬虫机制
反爬虫机制通常通过以下方式识别爬虫:
- **User-Agent检测:**爬虫通常使用特定的User-Agent,反爬虫机制可以通过检测User-Agent来识别爬虫。
- **IP地址检测:**爬虫通常使用大量IP地址进行爬取,反爬虫机制可以通过检测IP地址的频繁访问来识别爬虫。
- **行为分析:**爬虫通常具有规律的爬取行为,例如快速访问大量页面、频繁提交表单等,反爬虫机制可以通过分析爬取行为来识别爬虫。
### 5.1.2 应对反爬虫机制
应对反爬虫机制需要采取以下策略:
- **伪装User-Agent:**使用随机或合法的User-Agent来伪装爬虫。
- **代理IP池:**使用代理IP池来避免IP地址被封禁。
- **模拟人类行为:**通过设置随机延迟、模拟鼠标移动和键盘输入等方式来模拟人类行为。
- **验证码识别:**使用OCR技术或机器学习模型来识别验证码。
- **分布式爬虫:**使用分布式爬虫架构来分散爬取压力,避免被反爬虫机制检测到。
### 5.1.3 代码示例
```python
import requests
import random
# 伪装User-Agent
user_agents = [
"Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36",
"Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36",
"Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36"
]
user_agent = random.choice(user_agents)
# 使用代理IP
proxies = {
"http": "http://127.0.0.1:8080",
"https": "https://127.0.0.1:8080"
}
# 模拟人类行为
import time
import random
def delay():
time.sleep(random.uniform(0.5, 1.5))
```
0
0
相关推荐
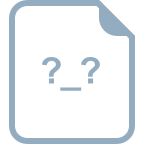
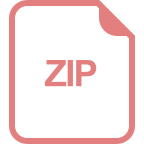





