大数据处理算法性能优化指南:提升算法效率与可靠性
发布时间: 2024-08-26 08:28:15 阅读量: 64 订阅数: 30 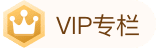
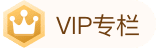
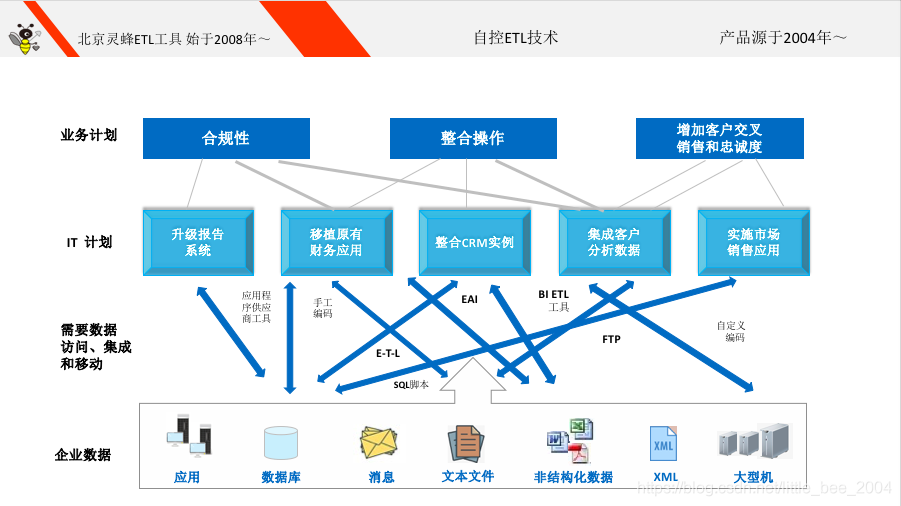
# 1. 大数据处理算法性能优化概述
大数据处理算法的性能优化对于处理海量数据和实现高效的分析至关重要。算法的性能受到多种因素的影响,包括算法复杂度、数据规模、计算资源和存储架构。
算法复杂度是衡量算法效率的一个关键指标,它描述了算法在最坏情况下执行所需的时间或空间资源。优化算法复杂度涉及选择具有较低复杂度的算法,或通过优化算法实现来降低复杂度。例如,使用分治法或快速排序算法可以降低排序算法的复杂度。
# 2. 算法性能分析与优化方法
### 2.1 算法复杂度分析
算法复杂度是衡量算法效率的重要指标,它表示算法在不同输入规模下执行所需的时间或空间。复杂度通常用大 O 表示法表示,表示算法执行时间或空间随输入规模 n 渐近增长的速率。
**时间复杂度**表示算法执行所需的时间,常见的时间复杂度有:
* O(1):常数时间复杂度,无论输入规模如何,算法执行时间都保持不变。
* O(log n):对数时间复杂度,算法执行时间随输入规模的增长呈对数增长。
* O(n):线性时间复杂度,算法执行时间随输入规模的增长呈线性增长。
* O(n^2):平方时间复杂度,算法执行时间随输入规模的平方增长。
* O(2^n):指数时间复杂度,算法执行时间随输入规模的指数增长。
**空间复杂度**表示算法执行所需的内存空间,常见的空间复杂度有:
* O(1):常数空间复杂度,无论输入规模如何,算法所需的内存空间都保持不变。
* O(n):线性空间复杂度,算法所需的内存空间随输入规模的增长呈线性增长。
* O(n^2):平方空间复杂度,算法所需的内存空间随输入规模的平方增长。
### 2.2 算法时间复杂度优化
优化算法时间复杂度的方法主要有:
* **减少循环次数:**通过使用更有效的算法或数据结构来减少循环次数。
* **减少循环内操作:**通过优化循环内的操作,减少每次循环所需的时间。
* **使用更快的算法:**选择时间复杂度更低的算法来解决问题。
* **使用数据结构:**使用合适的哈希表、树或其他数据结构来提高查找和插入效率。
* **并行化算法:**将算法分解成多个并行执行的任务,从而减少总执行时间。
### 2.3 算法空间复杂度优化
优化算法空间复杂度的方法主要有:
* **减少不必要的变量:**仅保留必要的变量,避免创建不必要的临时变量或对象。
* **使用更紧凑的数据结构:**选择占用更少空间的数据结构,例如使用位图代替布尔数组。
* **释放未使用的内存:**在不再需要时释放未使用的内存,避免内存泄漏。
* **使用内存池:**使用内存池来管理内存分配,减少内存分配和释放的开销。
* **使用虚拟内存:**将不经常使用的内存页面换出到磁盘,从而减少实际使用的内存空间。
# 3. 大数据处理算法优化实践
### 3.1 分布式算法优化
**简介**
分布式算法优化旨在通过将算法分布在多个计算节点上,提高大数据处理的性能。这种方法适用于数据量庞大、难以在单台机器上处理的情况。
**优化方法**
* **数据分区:**将数据集划分为多个较小的分区,以便在不同的节点上并行处理。
* **分布式计算:**使用分布式计算框架(如 Hadoop、Spark)将算法分布在多个节点上执行。
* **负载均衡:**确保每个节点的负载均衡,避免出现性能瓶颈。
**代码示例**
```java
import org.apache.spark.SparkConf;
import org.apache.spark.api.java.JavaRDD;
import org.apache.spark.api.java.JavaSparkContext;
public class DistributedWordCount {
public static void main(String[] args) {
// 创建 Spark 配置
SparkConf conf = new SparkConf().setAppName("DistributedWordCount");
// 创建 Spark 上下文
JavaSparkContext sc = new JavaSparkContext(conf);
// 加载文本文件
JavaRDD<String> lines = sc.textFile("input.txt");
// 将文本行拆分为单词
JavaRDD<String> words = lines.flatMap(line -> Arrays.asList(line.split(" ")).iterator());
// 统计单词出现的次数
JavaPairRDD<String, Integer> wordCounts = words.mapToPair(word -> new Tuple2<>(word, 1))
.reduceByKey((a, b) -> a + b);
// 保存结果
wordCounts.saveAsTextFile("output.txt");
}
}
```
**逻辑分析**
* `SparkConf`:配置 Spark 应用程序的设置。
* `JavaSparkContext`:创建 Spark 上下文,用于管理 Spark 作业。
* `textFile`:从文件系统加载文本文件。
* `flatMap`:将文本行拆分为单词。
* `mapToPair`:将单词映射为键值对,其中单词为键,出现次数为值。
* `reduceByKey`:对键值对进行聚合,统计单词出现的次数。
* `saveAsTextFile`:将结果保存到文件系统。
### 3.2 并行算法优化
**简介**
并行算法优化通过同时使用多个处理器或线程来提高算法的性能。这种方法适用于可以并行执行的算法。
**优化方法**
* **多线程编程:**使用多线程技术将算法分解为多个线程,同时执行。
* **并行算法库:**使用并行算法库(如 OpenMP、TBB)提供并行算法的实现。
* **GPU 加速:**利用 GPU 的并行计算能力加速算法的执行。
**代码示例**
```c++
#include <iostream>
#include <thread>
#include <vector>
using namespace std;
// 线程函数
void thread_function(vector<int>& numbers, int start, int end) {
for (int i = start; i < end; i++) {
numbers[i] *= 2;
}
}
int main() {
// 创建一个整数向量
vector<int> numbers = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
// 创建线程
thread t1(thread_function, ref(numbers), 0, 5);
thread t2(thread_function, ref(numbers), 5, 10);
// 等待线程完成
t1.join();
t2.join();
// 输出结果
for (int number : numbers) {
cout << number << " ";
}
cout << endl;
return 0;
}
```
**逻辑分析**
* `thread_function`:线程函数,负责将向量中的元素乘以 2。
* `main`:主函数,创建向量、线程并等待线程完成。
* `ref`:引用传递,确保线程可以访问向量。
* `join`:等待线程完成执行。
### 3.3 流式算法优化
**简介**
流式算法优化适用于处理不断增长的数据流的情况。这种方法使用增量算法,可以实时处理数据,而无需存储整个数据集。
**优化方法**
* **滑动窗口算法:**使用滑动窗口来处理数据流中的最新数据。
* **草图数据结构:**使用草图数据结构(如 Bloom 过滤器、Count-Min Sketch)对数据流进行近似统计。
* **流式处理框架:**使用流式处理框架(如 Apache Flink、Apache Kafka Streams)来构建和管理流式算法。
**代码示例**
```python
from pyspark.sql import SparkSession
from pyspark.sql.functions import count
# 创建 Spark Session
spark = SparkSession.builder.appName("StreamingWordCount").getOrCreate()
# 创建流式数据源
stream = spark.readStream.format("socket").option("host", "localhost").option("port", 9999).load()
# 统计单词出现的次数
wordCounts = stream.selectExpr("explode(split(value, ' ')) as word") \
.groupBy("word").count()
# 输出结果
query = wordCounts.writeStream.outputMode("complete").format("console").start()
query.awaitTermination()
```
**逻辑分析**
* `SparkSession`:创建 Spark Session,用于管理 Spark 作业。
* `readStream`:创建流式数据源,从套接字连接中读取数据。
* `selectExpr`:将数据流中的值拆分为单词。
* `groupBy` 和 `count`:对单词进行分组并统计出现的次数。
* `writeStream`:将结果写入控制台。
# 4. 算法优化工具与技术
算法优化涉及使用各种工具和技术来提高算法的性能。本章将介绍一些常用的算法优化工具和技术,包括性能分析工具、算法优化库和算法优化框架。
### 4.1 性能分析工具
性能分析工具可以帮助识别和分析算法的性能瓶颈。这些工具通常提供以下功能:
* **性能剖析:**收集有关算法执行期间资源使用情况(例如 CPU 时间、内存使用情况)的数据。
* **调用跟踪:**记录算法执行期间函数调用的顺序和持续时间。
* **火焰图:**可视化算法执行期间函数调用的层次结构,突出显示最耗时的函数。
**示例:**
```
# 使用 Python 的 cProfile 模块进行性能剖析
import cProfile
def my_function():
# ...
cProfile.run('my_function()')
```
**逻辑分析:**
`cProfile.run()` 函数执行 `my_function()` 并收集有关其执行的性能数据。生成的报告显示函数的调用次数、执行时间和累积时间。
### 4.2 算法优化库
算法优化库提供预先实现的优化算法和数据结构,可以显著减少开发和优化算法所需的时间和精力。这些库通常包括以下内容:
* **排序算法:**快速排序、归并排序、堆排序等。
* **搜索算法:**二分查找、哈希表等。
* **数据结构:**树、图、队列、栈等。
**示例:**
```
# 使用 Python 的 NumPy 库进行矩阵乘法
import numpy as np
A = np.array([[1, 2], [3, 4]])
B = np.array([[5, 6], [7, 8]])
C = np.dot(A, B)
```
**逻辑分析:**
`np.dot()` 函数使用优化过的矩阵乘法算法计算矩阵 `A` 和 `B` 的乘积。这比手动实现矩阵乘法算法要高效得多。
### 4.3 算法优化框架
算法优化框架提供了一个结构化的环境,用于开发和优化算法。这些框架通常包括以下功能:
* **算法选择:**提供各种算法的集合,并根据输入数据和性能要求自动选择最佳算法。
* **并行化:**支持算法的并行执行,以利用多核处理器或分布式系统。
* **性能监控:**提供工具来监控算法的性能并识别优化机会。
**示例:**
```
# 使用 Apache Spark 框架进行分布式算法优化
from pyspark.sql import SparkSession
spark = SparkSession.builder.appName("My App").getOrCreate()
df = spark.createDataFrame([(1, 2), (3, 4)], ["a", "b"])
df.groupBy("a").sum().show()
```
**逻辑分析:**
Apache Spark 框架自动将 `groupBy()` 和 `sum()` 操作分布到多个工作节点上,从而提高了对大数据集的处理速度。
# 5.1 算法优化最佳实践
在进行算法优化时,遵循以下最佳实践可以帮助提高算法的性能:
* **选择合适的算法:**根据问题的具体要求,选择最合适的算法。考虑算法的复杂度、数据结构和内存使用情况。
* **优化数据结构:**选择合适的数据结构可以显著提高算法的性能。例如,使用哈希表进行快速查找,使用树或图进行层次遍历。
* **避免不必要的计算:**通过缓存结果、使用懒惰求值或应用剪枝策略来避免重复计算。
* **并行化算法:**如果可能,将算法并行化以利用多核处理器或分布式计算环境。
* **使用优化库:**利用现有的优化库,例如 NumPy、SciPy 和 TensorFlow,可以节省时间和精力。
* **持续性能监控:**定期监控算法的性能,以识别瓶颈并进行进一步优化。
* **关注局部性:**优化算法以最大化数据和指令在缓存中的局部性,从而减少内存访问延迟。
* **使用算法优化工具:**利用性能分析工具,例如 gprof 和 Valgrind,来识别算法中的瓶颈并指导优化工作。
* **遵循算法设计原则:**遵循算法设计原则,例如分治、贪心和动态规划,可以帮助开发高效的算法。
* **考虑硬件限制:**了解目标硬件的限制,例如内存大小和处理器速度,并优化算法以适应这些限制。
0
0
相关推荐
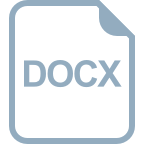
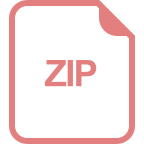
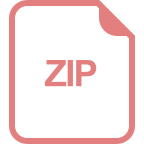