【PHP数据库JSON返回指南】:从入门到精通
发布时间: 2024-07-27 22:27:14 阅读量: 26 订阅数: 26 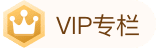
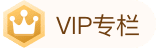
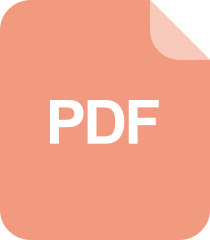
php简单实现查询数据库返回json数据
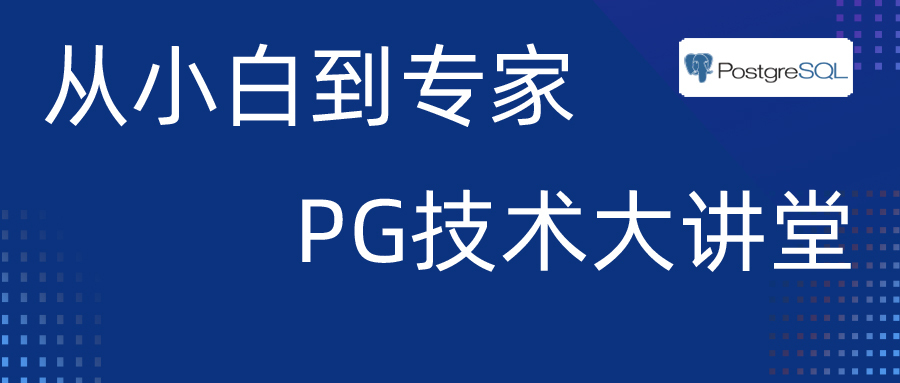
# 1. PHP与数据库交互基础**
PHP与数据库交互是Web开发中至关重要的环节。本节将介绍PHP与数据库交互的基础知识,包括数据库连接、查询执行和结果处理。
**数据库连接**
```php
$conn = mysqli_connect('localhost', 'username', 'password', 'database_name');
```
此代码使用MySQLi扩展建立与数据库的连接。其中,`localhost`为数据库服务器地址,`username`和`password`为数据库用户名和密码,`database_name`为数据库名称。
**查询执行**
```php
$sql = "SELECT * FROM table_name WHERE id = 1";
$result = mysqli_query($conn, $sql);
```
此代码执行SQL查询,其中`$sql`为查询语句,`$conn`为数据库连接。`mysqli_query()`函数执行查询并返回一个结果集对象。
# 2. JSON数据格式与处理
### 2.1 JSON语法和数据结构
JSON(JavaScript Object Notation)是一种轻量级的数据交换格式,广泛用于Web开发和数据传输。其语法简单,易于解析和生成。
**JSON语法**
JSON数据由键值对组成,键为字符串,值可以是字符串、数字、布尔值、数组或嵌套对象。键值对之间用冒号分隔,对象和数组用大括号和大括号分隔。
```json
{
"name": "John Doe",
"age": 30,
"is_active": true,
"address": {
"street": "123 Main Street",
"city": "Anytown",
"state": "CA",
"zip": "12345"
},
"hobbies": ["reading", "hiking", "coding"]
}
```
**JSON数据结构**
JSON数据可以表示为以下数据结构:
* **对象:**键值对的集合,键为字符串。
* **数组:**有序值列表,值可以是任何JSON数据类型。
* **字符串:**由双引号引用的文本。
* **数字:**整数或浮点数。
* **布尔值:**true或false。
* **null:**表示空值。
### 2.2 PHP解析和生成JSON数据
PHP提供了丰富的函数库用于解析和生成JSON数据。
**解析JSON数据**
```php
$json_string = '{"name": "John Doe", "age": 30}';
$json_data = json_decode($json_string);
echo $json_data->name; // 输出: John Doe
echo $json_data->age; // 输出: 30
```
**生成JSON数据**
```php
$data = [
"name" => "John Doe",
"age" => 30
];
$json_string = json_encode($data);
echo $json_string; // 输出: {"name": "John Doe", "age": 30}
```
**参数说明**
* `json_decode()`:解析JSON字符串并返回一个PHP对象或数组。
* `json_encode()`:将PHP对象或数组编码为JSON字符串。
**代码逻辑分析**
* `json_decode()`函数将JSON字符串解析为PHP对象或数组。
* `json_encode()`函数将PHP对象或数组编码为JSON字符串。
* `echo`语句输出解析或编码后的数据。
# 3. PHP数据库查询与JSON返回
### 3.1 数据库查询基础
数据库查询是获取存储在数据库中的数据的过程。PHP提供了多种方法来执行数据库查询,包括:
- **mysqli_query():**使用MySQLi扩展执行查询。
- **PDO:**使用PHP数据对象(PDO)执行查询,支持多种数据库类型。
- **原生PHP函数:**使用诸如`mysql_query()`和`pg_query()`之类的原生PHP函数执行查询(不推荐)。
**查询语法**
数据库查询使用SQL(结构化查询语言)编写,它是一种专门用于与数据库交互的语言。基本的查询语法如下:
```sql
SELECT column1, column2, ...
FROM table_name
WHERE condition1 AND condition2 ...
```
其中:
- `SELECT`指定要检索的列。
- `FROM`指定要查询的表。
- `WHERE`指定查询条件。
**查询参数**
查询参数允许将动态值传递给查询,从而提高查询的可重用性和安全性。在PHP中,可以使用`mysqli_stmt_prepare()`和`PDO`准备语句来使用查询参数。
**查询结果**
查询执行后,将返回一个结果集,其中包含查询到的数据。可以使用`mysqli_fetch_array()`或`PDOStatement::fetch()`方法获取结果集中的行。
### 3.2 查询结果转换为JSON格式
将查询结果转换为JSON格式涉及两个步骤:
**1. 编码查询结果**
查询结果是一个PHP数组,需要使用`json_encode()`函数将其编码为JSON字符串。
```php
$json_data = json_encode($result);
```
**2. 设置响应头**
为了指示响应是JSON格式,需要设置以下HTTP响应头:
```php
header('Content-Type: application/json');
```
### 代码示例
以下代码示例演示了如何使用PHP执行数据库查询并将其结果转换为JSON格式:
```php
<?php
// 连接到数据库
$mysqli = new mysqli('localhost', 'username', 'password', 'database_name');
// 准备查询
$stmt = $mysqli->prepare("SELECT * FROM users WHERE name = ?");
// 绑定参数
$stmt->bind_param('s', $name);
// 执行查询
$stmt->execute();
// 获取结果集
$result = $stmt->get_result();
// 编码查询结果
$json_data = json_encode($result->fetch_all(MYSQLI_ASSOC));
// 设置响应头
header('Content-Type: application/json');
// 输出JSON数据
echo $json_data;
?>
```
**代码逻辑分析**
1. 使用`mysqli`类连接到数据库。
2. 使用`prepare()`方法准备查询并绑定参数。
3. 执行查询并获取结果集。
4. 使用`fetch_all()`方法获取结果集中的所有行。
5. 使用`json_encode()`函数将结果集编码为JSON字符串。
6. 设置响应头指示JSON响应。
7. 输出JSON数据。
# 4. PHP数据库JSON返回的实践应用
### 4.1 RESTful API开发
RESTful API(Representational State Transferful Application Programming Interface)是一种基于HTTP协议的API设计风格,它遵循一定的约束和规范,以实现资源的统一管理和操作。
#### RESTful API设计原则
RESTful API设计遵循以下原则:
- **资源导向:**API操作的对象是资源,资源可以通过URI(统一资源标识符)进行标识。
- **统一接口:**对不同资源的操作使用统一的HTTP方法(如GET、POST、PUT、DELETE)。
- **无状态:**服务器不保存客户端状态,每次请求都是独立的。
- **缓存性:**API响应可以被缓存,以提高性能。
- **分层系统:**API可以分层设计,以实现模块化和可扩展性。
#### PHP开发RESTful API
PHP提供了多种框架和工具,用于开发RESTful API,如Laravel、Slim和Symfony。这些框架提供了方便的路由、请求处理和响应生成机制。
**示例代码:**
```php
// 使用Laravel框架开发RESTful API
// 定义路由
Route::get('/api/users', 'UserController@index');
Route::post('/api/users', 'UserController@store');
Route::put('/api/users/{id}', 'UserController@update');
Route::delete('/api/users/{id}', 'UserController@destroy');
// UserController处理请求
class UserController extends Controller
{
public function index()
{
$users = User::all();
return response()->json($users);
}
public function store(Request $request)
{
$user = User::create($request->all());
return response()->json($user, 201);
}
public function update(Request $request, $id)
{
$user = User::findOrFail($id);
$user->update($request->all());
return response()->json($user);
}
public function destroy($id)
{
$user = User::findOrFail($id);
$user->delete();
return response()->json(null, 204);
}
}
```
**逻辑分析:**
- 路由定义了API的端点和处理请求的控制器方法。
- 控制器方法处理请求,查询数据库,并返回JSON响应。
- 响应代码根据HTTP状态码(如201表示创建成功、204表示删除成功)进行设置。
### 4.2 AJAX交互与数据传输
AJAX(Asynchronous JavaScript and XML)是一种技术,允许在不重新加载整个页面的情况下,与服务器进行异步通信。它广泛用于Web应用程序中,用于实现动态交互和数据传输。
#### PHP处理AJAX请求
PHP可以使用$_POST、$_GET或$_REQUEST全局变量来接收AJAX请求数据。它还可以使用header()函数设置响应头,并使用echo或json_encode()函数返回JSON响应。
**示例代码:**
```php
// 处理AJAX请求
if (isset($_POST['action']) && $_POST['action'] == 'get_users') {
$users = User::all();
echo json_encode($users);
exit;
}
```
**逻辑分析:**
- 检查是否存在名为"action"的POST参数,并验证其值为"get_users"。
- 查询数据库获取用户数据。
- 使用json_encode()函数将用户数据编码为JSON字符串。
- 使用echo输出JSON响应。
#### AJAX数据传输
AJAX请求可以传输各种数据类型,包括JSON、XML、文本和二进制数据。在PHP中,可以使用jQuery或其他JavaScript库来发送AJAX请求,并处理服务器返回的响应。
**示例代码:**
```javascript
// 使用jQuery发送AJAX请求
$.ajax({
url: '/api/users',
method: 'GET',
success: function(data) {
// 处理返回的JSON数据
}
});
```
**逻辑分析:**
- 使用jQuery的ajax()函数发送AJAX请求。
- 指定请求的URL和方法。
- 在success回调函数中处理服务器返回的JSON数据。
# 5. PHP数据库JSON返回的高级技巧
### 5.1 数据安全与防注入
在处理数据库查询和JSON返回时,数据安全至关重要。防止SQL注入攻击是首要任务,它可以利用恶意输入来操纵数据库查询并获取未经授权的数据。
**防注入措施:**
- **使用参数化查询:**使用PDO或mysqli_stmt等API来准备查询,并使用参数绑定来替换用户输入。这可以防止恶意字符被解释为SQL语句的一部分。
- **转义用户输入:**在将用户输入添加到查询之前,使用mysqli_real_escape_string()或addslashes()等函数对其进行转义。这将替换特殊字符,使其不会被解释为SQL命令。
- **限制用户权限:**只授予用户执行特定查询和访问特定数据的最低权限。这可以限制潜在攻击的范围。
- **验证用户输入:**在处理用户输入之前,使用正则表达式或其他验证方法检查其有效性。这可以防止恶意输入到达数据库。
### 5.2 性能优化与缓存机制
优化PHP数据库JSON返回的性能对于提高应用程序响应时间至关重要。以下是一些优化技巧:
**缓存查询结果:**将经常执行的查询结果存储在缓存中,例如Memcached或Redis。这可以避免重复执行相同的查询,从而提高性能。
- **使用索引:**在数据库表上创建索引,以便快速查找数据。这可以显著提高查询速度。
- **优化查询:**使用EXPLAIN语句分析查询并识别性能瓶颈。调整查询以提高效率。
- **使用批处理:**将多个查询组合成一个批处理操作。这可以减少与数据库的交互次数,从而提高性能。
0
0
相关推荐
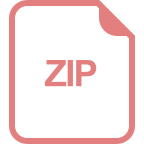
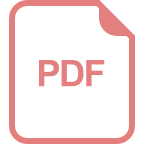
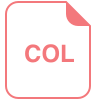
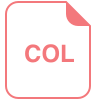
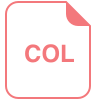
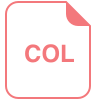
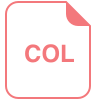
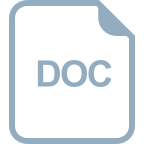