Understanding Fourier Series and Their Applications
发布时间: 2024-09-15 05:28:42 阅读量: 24 订阅数: 29 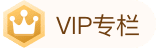
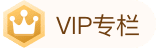
# 1. Fundamental Concepts of Fourier Series
## 1.1 What is a Fourier Series?
A Fourier Series is a method to decompose a periodic function into a series of sine and cosine terms. By expressing the original function as the sum of these fundamental frequency components, we can better understand and analyze the characteristics of periodic signals.
## 1.2 Historical Background of Fourier Series
The Fourier Series was first proposed by the French mathematician Joseph Fourier in the early 19th century to study problems related to heat conduction. It has since been widely applied in mathematics, physics, engineering, and other fields.
## 1.3 Mathematical Principles of Fourier Series
Fourier Series utilizes sine and cosine functions as the basis functions and expands periodic functions into an infinite series of trigonometric functions through the Fourier Series formula. This involves complex-valued Fourier transforms and spectral analysis. The mathematical principles of Fourier Series are crucial for understanding its applications.
# 2. Expansion and Convergence of Fourier Series
The expansion form of a Fourier Series describes how a periodic function can be represented as a linear combination of a set of sine and cosine functions. In practical applications, Fourier Series expansion can decompose complex periodic signals into multiple simple sine and cosine signals, facilitating analysis and processing.
### 2.1 Expansion Form of Fourier Series
The mathematical expression of the Fourier Series expansion for a function f(t) with a period T is as follows:
\[ f(t) = a_0 + \sum_{n=1}^{\infty}(a_n \cos(n\omega t) + b_n \sin(n\omega t)) \]
Where a0 is the DC component, an and bn are the coefficients of the sine and cosine terms, respectively, and ω=2π/T is the angular frequency.
### 2.2 Concepts of Convergence and Convergence Domain
The convergence of a Fourier Series refers to the conditions under which the series in the expansion converges to the original function. Typically, the function must be absolutely integrable over one period to ensure the convergence of the series.
The convergence domain refers to the range within which the Fourier Series expansion converges to the original function. Generally, Fourier Series have better convergence for functions that are continuous and differentiable over their defined domain.
### 2.3 Convergence Conditions of Fourier Series
The convergence conditions of a Fourier Series mainly depend on the properties of the original function, such as continuity and differentiability. Special functions like square waves may require the generalized form of Fourier Series to handle convergence issues.
In practical applications, understanding the convergence conditions of Fourier Series is crucial for correctly applying Fourier transforms and their inverse transforms, ensuring accurate signal reconstruction and processing.
# 3. Discrete and Continuous Forms of Fourier Series
Fourier Series exists in both discrete and continuous forms, each with different applications and characteristics. The following will detail the discrete and continuous forms of Fourier Series and their practical applications.
#### 3.1 Applications of Discrete Fourier Series
Discrete Fourier Series is widely used in the field of digital signal processing, especially in spectral analysis, filtering, and data compression. It can decompose signals into multiple frequency components, helping us understand the signal's frequency domain characteristics and thus implement signal processing and analysis.
```python
# Python code example: Calculate Discrete Fourier Transform
import numpy as np
# Generate a random sequence as the input signal
x = np.array([1, 2, 3, 4, 3, 2])
# Compute Discrete Fourier Transform
X = np.fft.fft(x)
# Output spectrum magnitude
print(np.abs(X))
```
**Code Summary:**
- Generate a random sequence as the input signal
- Use the `fft` function from NumPy library to compute the Discrete Fourier Transform
- Output the spectrum magnitude to view the signal's frequency domain characteristics
**Result Explanation:**
By computing the Discrete Fourier Transform, we can obtain the spectrum magnitude of the input signal, thus understanding the frequency domain components of the signal.
#### 3.2 Applications of Continuous Fourier Series
Continuous Fourier Series play a significant role in signal processing, communication systems, and control engineering. It can decompose continuous signals into sine and cosine wave components of different frequencies, used for analyzing the signal's spectrum and implementing filtering, modulation, and other operations.
```java
// Java code example: ***
***plex;
***mons.math3.transform.*;
// Generate a sine signal as input
double[] x = {0, 1, 2, 1, 0, -1, -2, -1};
// Define a Fourier Transform object
FastFourierTransformer transformer = new FastFourierTransformer(DftNormalization.STANDARD);
// Execute Continuous Fourier Transform
Complex[] X = transformer.transform(x, TransformType.FORWARD);
// Output spectrum magnitude
for (Complex c : X) {
System.out.println(c.abs());
}
```
**Code Summary:**
- Generate a sine signal as the input
- Use the `FastFourierTransformer` class from the Apache Commons Math library to calculate Continuous Fourier Transform
- Output spectrum magnitude to analyze the signal's frequency domain characteristics
**Result Explanation:**
Continuous Fourier Transform helps analyze the signal's frequency domain characteristics, thereby implementing signal processing and frequency domain operations.
# 4. Applications of Fourier Series in Signal Processing
Fourier Series has a wide range of applications in signal processing, helping us better understand the frequency spectrum characteristics of signals, perform signal analysis and filtering, and achieve signal reconstruction and synthesis. The following will discuss the specific applications of Fourier Series in the field of signal processing in detail.
#### 4.1 Fourier Series and Frequency Domain Analysis
In signal processing, Fourier Series is extensively used to analyze the frequency domain characteristics of signals. By decomposing the signal into sine and cosine functions of different frequencies, the signal's spectral structure is revealed, helping us understand the contribution of each frequency component contained in the signal.
```python
import numpy as np
import matplotlib.pyplot as plt
# Generate a signal with multiple frequency components
t = np.linspace(0, 1, 500)
frequencies = [1, 5, 10] # Three frequency components
signal = np.sum([np.sin(2 * np.pi * f * t) for f in frequencies], axis=0)
# Perform Fourier Transform on the signal
fft_result = np.fft.fft(signal)
freqs = np.fft.fftfreq(len(signal))
# Plot the signal's time domain and frequency domain representation
plt.figure(figsize=(12, 6))
plt.subplot(2, 1, 1)
plt.plot(t, signal)
plt.title('Time Domain Signal')
plt.xlabel('Time')
plt.ylabel('Amplitude')
plt.subplot(2, 1, 2)
plt.stem(freqs, np.abs(fft_result))
plt.title('Frequency Domain Signal')
plt.xlabel('Frequency (Hz)')
plt.ylabel('Amplitude')
plt.show()
```
With the above code, we can generate a signal with multiple frequency components and perform Fourier Transform on it. We can then draw the signal's representation in the time and frequency domains, clearly showing the signal's spectral characteristics.
#### 4.2 Calculation and Analysis of Signal Spectrum
Fourier Series can also be used to calculate and analyze the spectral characteristics of signals. By performing Fourier Transform on a signal, we can obtain its representation in the frequency domain and then perform spectral analysis, finding the main frequency components in the signal, as well as their intensity and phase information.
```python
from scipy import signal
# Generate a signal
t = np.linspace(0, 1, 1000)
signal = np.sin(2 * np.pi * 5 * t) + 0.5 * np.sin(2 * np.pi * 10 * t)
# Calculate the power spectral density of the signal
frequencies, psd = signal.welch(signal)
# Plot the power spectral density graph
plt.figure(figsize=(8, 4))
plt.plot(frequencies, psd)
plt.title('Power Spectral Density')
plt.xlabel('Frequency (Hz)')
plt.ylabel('Power')
plt.show()
```
In the above code, we generated a signal with two frequency components and used the `signal.welch()` function to calculate the power spectral density of the signal. We then plotted the power spectral density graph to help us analyze the energy distribution of different frequency components in the signal.
#### 4.3 Signal Filtering and Reconstruction
In signal processing, Fourier Series is also commonly used for signal filtering and reconstruction. By performing filtering operations in the frequency domain, noise or specific frequency components can be removed from the signal, achieving clear signal processing; at the same time, we can reconstruct the processed frequency domain signal back into the time domain signal through the inverse Fourier Transform, ultimately obtaining the desired signal.
```python
# Perform low-pass filtering on the signal
cutoff_freq = 7 # Cutoff frequency is 7Hz
b, a = signal.butter(4, cutoff_freq, 'low', fs=1000)
filtered_signal = signal.filtfilt(b, a, signal)
# Plot the comparison of the signal before and after filtering
plt.figure(figsize=(8, 4))
plt.plot(t, signal, label='Original Signal')
plt.plot(t, filtered_signal, label='Filtered Signal')
plt.title('Signal Filtering')
plt.xlabel('Time')
plt.ylabel('Amplitude')
plt.legend()
plt.show()
```
The above code achieves a low-pass filtering operation on the signal, removing frequency components higher than 7Hz, and plots a comparison of the signal before and after filtering, showing the effect of signal processing after filtering.
# 5. Applications of Fourier Series in Image Processing
Fourier Series has a wide range of applications in image processing. Through Fourier Transform, the image can be converted to the frequency domain for analysis and processing, thus achieving operations such as image filtering, denoising, compression, and reconstruction. The following will detail the specific applications of Fourier Series in the field of image processing:
### 5.1 Fourier Transform and Image Frequency Domain Analysis
In image processing, Fourier Transform is widely used to convert images into the frequency domain for analysis. Through the analysis of the frequency domain characteristics of the image, the spectral information of the image can be obtained, understanding the distribution of different frequency components in the image, providing a basis for subsequent processing.
```python
import cv2
import numpy as np
from matplotlib import pyplot as plt
img = cv2.imread('image.jpg', 0)
f = np.fft.fft2(img)
fshift = np.fft.fftshift(f)
magnitude_spectrum = 20 * np.log(np.abs(fshift))
plt.subplot(121), plt.imshow(img, cmap='gray')
plt.title('Input Image'), plt.xticks([]), plt.yticks([])
plt.subplot(122), plt.imshow(magnitude_spectrum, cmap='gray')
plt.title('Magnitude Spectrum'), plt.xticks([]), plt.yticks([])
plt.show()
```
The above code demonstrates performing Fourier Transform on a grayscale image and drawing its spectral information. By observing the spectrum, the frequency domain characteristics of the image can be analyzed.
### 5.2 Image Filtering and Denoising Processing
Using Fourier Transform, ***mon filters include low-pass filters and high-pass filters, which can achieve smoothing and sharpening of the image, and can also be used to remove noise from the image.
```python
rows, cols = img.shape
crow, ccol = rows // 2, cols // 2
fshift[crow - 30:crow + 30, ccol - 30:ccol + 30] = 0
f_ishift = np.fft.ifftshift(fshift)
img_back = np.fft.ifft2(f_ishift)
img_back = np.abs(img_back)
plt.subplot(121), plt.imshow(img, cmap='gray')
plt.title('Input Image'), plt.xticks([]), plt.yticks([])
plt.subplot(122), plt.imshow(img_back, cmap='gray')
plt.title('Image after HPF'), plt.xticks([]), plt.yticks([])
plt.show()
```
The above code demonstrates filtering the high-frequency components in the frequency domain and then obtaining the filtered image through the inverse Fourier Transform, thus achieving image denoising processing.
### 5.3 Image Compression and Reconstruction
Using Fourier Transform, image compression and reconstruction can also be achieved. By retaining the main energy information in the image's frequency domain, the image can be compressed to reduce storage space occupancy. At the same time, the compressed data can be reconstructed through the inverse Fourier Transform.
```python
fshift[crow - 30:crow + 30, ccol - 30:ccol + 30] = fshift.min()
f_ishift = np.fft.ifftshift(fshift)
img_back = np.fft.ifft2(f_ishift)
img_back = np.abs(img_back)
plt.subplot(121), plt.imshow(img, cmap='gray')
plt.title('Input Image'), plt.xticks([]), plt.yticks([])
plt.subplot(122), plt.imshow(img_back, cmap='gray')
plt.title('Image after Compression and Reconstruction'), plt.xticks([]), plt.yticks([])
plt.show()
```
The above code demonstrates compressing the image in the frequency domain and reconstructing the compressed image through the inverse Fourier Transform, achieving the process of image compression and reconstruction.
Through the above code examples and explanations, we can clearly understand the applications of Fourier Series in image processing, including frequency domain analysis, filtering and denoising, compression, and reconstruction, among other aspects.
# 6. Applications of Fourier Series in Engineering Practice
As a powerful signal processing tool, Fourier Series has a wide range of applications in the field of engineering. The following are some specific application cases of Fourier Series in engineering practice:
#### 6.1 Application Cases of Fourier Series in the Field of Signal Processing
In communication systems, Fourier Series is widely used in signal modulation, demodulation, encoding, and decoding processes. For example, in modulation, Fourier Series can transform signals from the time domain to the frequency domain for spectral analysis and signal processing.
```python
# Signal modulation example code
import numpy as np
import matplotlib.pyplot as plt
# Generate signal
t = np.linspace(0, 1, 1000)
f_signal = 5 # Signal frequency is 5Hz
signal = np.sin(2 * np.pi * f_signal * t)
# Fourier Transform
frequencies = np.fft.fftfreq(len(t), t[1] - t[0])
fft_values = np.fft.fft(signal)
# Display signal spectrum
plt.figure()
plt.plot(frequencies, np.abs(fft_values))
plt.title('Signal Spectrum')
plt.xlabel('Frequency (Hz)')
plt.ylabel('Amplitude')
plt.show()
```
Through the processing of Fourier Series, the spectral characteristics of the signal can be better understood, and the signal can be effectively analyzed and processed.
#### 6.2 Application Cases of Fourier Series in the Field of Image Processing
In digital image processing, Fourier Series is widely used in frequency domain analysis, filtering processing, image enhancement, and other aspects. For example, Fourier Transform can be used to analyze and process frequency domain information.
```java
// ***
***plex;
// Read image data
BufferedImage image = ImageIO.read(new File("image.jpg"));
int width = image.getWidth();
int height = image.getHeight();
// Convert image to grayscale
int[][] grayImage = convertToGrayscale(image);
Complex[][] complexImage = new Complex[height][width];
// Perform two-dimensional Fourier Transform
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
complexImage[i][j] = new Complex(grayImage[i][j], 0);
}
}
Complex[][] frequencyDomainImage = twoDimensionalFFT(complexImage);
// Perform filtering operations on the frequency domain image, etc.
// Reconstruct the image after frequency domain analysis
BufferedImage reconstructedImage = reconstructImage(frequencyDomainImage);
```
Through the application of Fourier Series, in-depth analysis and processing of image frequency domain information can be achieved, resulting in clearer images with specific features.
#### 6.3 Other Applications and Development Trends of Fourier Series in Other Fields
In addition to the fields of signal and image processing, Fourier Series also has a wide range of applications in other engineering fields. With the continuous development of science and technology, the applications of Fourier Series in audio processing, video processing, medical image processing, and other fields are becoming increasingly important. In the future, with the development of artificial intelligence, big data, and other technologies, the applications of Fourier Series will be further expanded and deepened, bringing more innovations and breakthroughs to engineering practice.
In engineering practice, fully understanding and applying Fourier Series can not only improve the efficiency and quality of engineering processing but also promote the progress and development of engineering technology. Therefore, understanding Fourier Series and its applications is of great significance. Engineers should continuously learn and explore, maximize the advantages of Fourier Series, and create more engineering marvels.
0
0
相关推荐
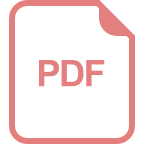
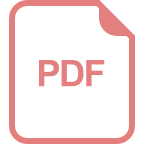
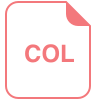
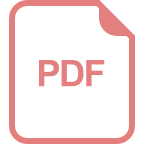
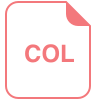
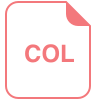
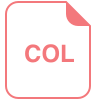
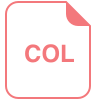