Frequency Domain Filtering: Case Studies Analysis
发布时间: 2024-09-15 05:37:53 阅读量: 24 订阅数: 29 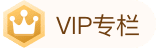
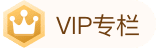
# 1. Introduction
This chapter presents the background, overview, and structural outline of frequency domain filtering.
# 2. Fundamentals of Frequency Domain Filtering
- 2.1 Introduction to Time Domain and Frequency Domain Concepts
- 2.2 Principles of Fourier Transform
- 2.3 Principles of Frequency Domain Filtering
# 3. Frequency Domain Filtering Algorithms
Commonly used frequency domain filtering algorithms include Discrete Fourier Transform (DFT) and Fast Fourier Transform (FFT). The principles and applications of these methods will be discussed in detail below.
#### 3.1 Discrete Fourier Transform (DFT)
The Discrete Fourier Transform (DFT) is a method that converts a discrete sequence into another discrete sequence of the same length. In frequency domain filtering, by performing a DFT transformation on the signal, it can be converted from the time domain to the frequency domain, thus achieving frequency domain filtering.
```python
import numpy as np
def DFT(signal):
N = len(signal)
n = np.arange(N)
k = n.reshape((N, 1))
exp_term = np.exp(-2j * np.pi * k * n / N)
return np.dot(exp_term, signal)
# Example code
signal = np.array([1, 2, 3, 4])
dft_result = DFT(signal)
print("DFT result:", dft_result)
```
#### 3.2 Fast Fourier Transform (FFT)
The Fast Fourier Transform (FFT) is an efficient algorithm for computing DFT, capable of quickly analyzing signals in the frequency domain. In frequency domain filtering, FFT is more commonly used than DFT due to its faster computation speed, making it suitable for processing large-scale data in the frequency domain.
```python
from scipy.fft import fft
# Example code
signal = np.array([1, 2, 3, 4])
fft_result = fft(signal)
print("FFT result:", fft_result)
```
#### 3.3 Comparison of Frequency Domain Filtering Methods
In practical applications, different frequency domain filtering methods can be chosen based on the requirements and data characteristics. DFT is suitable for frequency domain analysis of small-scale data, while FFT is better for processing large-scale data. When making a choice, it is necessary to consider both computational efficiency and accuracy requirements comprehensively.
With the above introduction, we have gained an understanding of the key methods in frequency domain
0
0
相关推荐
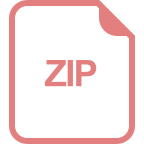
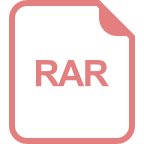
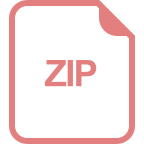
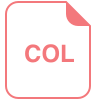
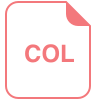
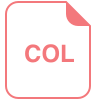
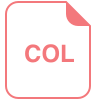
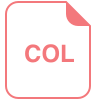
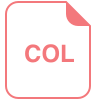