Python数据结构与算法:掌握高效数据处理的利器,提升代码性能
发布时间: 2024-06-17 18:54:58 阅读量: 13 订阅数: 14 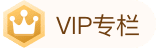
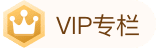
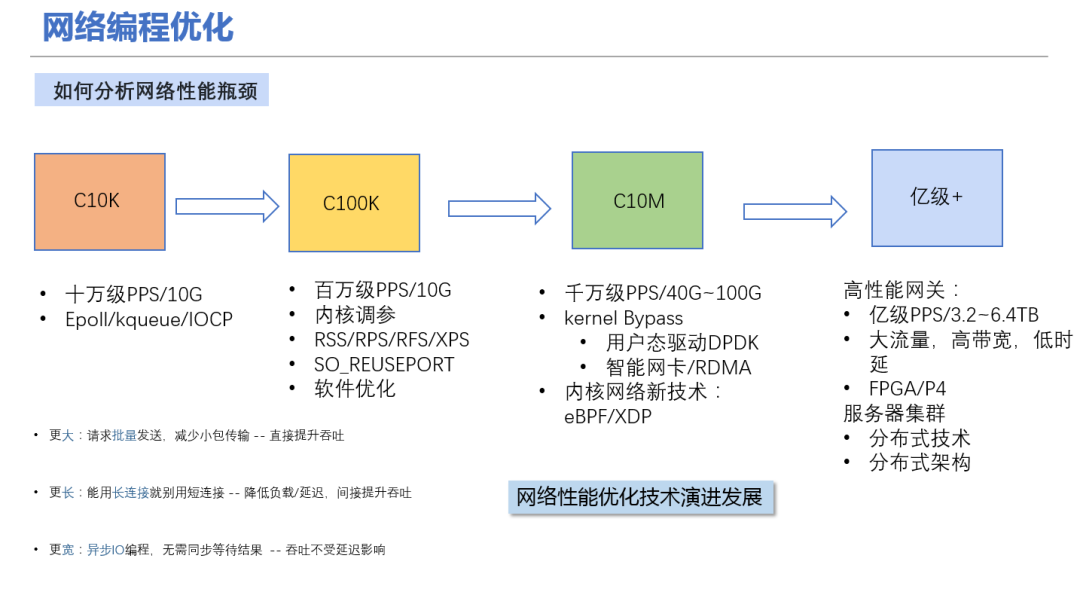
# 1. Python数据结构基础**
Python数据结构是存储和组织数据的基本单位,对于高效地管理和处理数据至关重要。Python提供了丰富的内置数据结构,包括列表、元组、字典和集合,每个数据结构都有其独特的特性和用途。
列表是可变的、有序的元素集合,可以存储各种数据类型。元组是不可变的、有序的元素集合,主要用于存储不可更改的数据。字典是键值对的集合,其中键是唯一的,而值可以是任何数据类型。集合是无序的、唯一的元素集合,用于快速查找和删除元素。
# 2. Python数据结构进阶**
**2.1 链表与栈**
**2.1.1 链表的结构和操作**
链表是一种线性数据结构,由一组节点组成,每个节点包含一个值和指向下一个节点的指针。链表具有以下特点:
- 节点可以动态分配和释放,无需预先分配内存。
- 链表可以高效地插入和删除元素,因为不需要移动其他元素。
- 链表的查找效率较低,因为需要遍历整个链表。
**链表操作:**
- **创建链表:**创建一个头节点,指向第一个元素。
- **插入元素:**在指定位置创建新节点,并更新指针。
- **删除元素:**找到要删除的元素,并更新指针跳过该元素。
- **查找元素:**遍历链表,比较每个元素的值。
**代码示例:**
```python
class Node:
def __init__(self, value):
self.value = value
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def insert(self, value):
new_node = Node(value)
if self.head is None:
self.head = new_node
else:
current = self.head
while current.next is not None:
current = current.next
current.next = new_node
def delete(self, value):
if self.head is None:
return
if self.head.value == value:
self.head = self.head.next
return
current = self.head
while current.next is not None:
if current.next.value == value:
current.next = current.next.next
return
current = current.next
def find(self, value):
current = self.head
while current is not None:
if current.value == value:
return True
current = current.next
return False
```
**2.1.2 栈的结构和操作**
栈是一种后进先出(LIFO)的数据结构,由一组元素组成,遵循以下规则:
- 只能从栈顶访问元素。
- 新元素只能添加到栈顶。
- 从栈中删除元素只能从栈顶。
**栈操作:**
- **压栈(push):**将元素添加到栈顶。
- **弹栈(pop):**从栈顶移除元素。
- **栈顶(peek):**查看栈顶元素,但不移除。
- **栈空(isEmpty):**检查栈是否为空。
**代码示例:**
```python
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
if not self.isEmpty():
return self.items.pop()
else:
raise IndexError("Cannot pop from an empty stack.")
def peek(self):
if not self.i
```
0
0
相关推荐
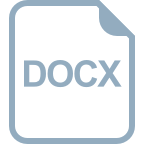
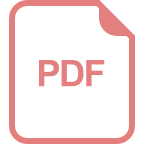
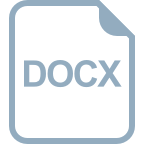





