Python并发编程实战:多线程与多进程的艺术,提升代码并发能力
发布时间: 2024-06-17 18:50:09 阅读量: 67 订阅数: 31 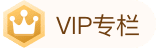
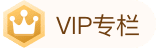

# 1. Python并发编程概述
并发编程是一种编程范式,它允许程序同时执行多个任务。在Python中,并发编程主要通过多线程和多进程来实现。
**多线程**:线程是程序中执行任务的轻量级实体。它们共享相同的内存空间,因此可以轻松地通信和同步。多线程编程适用于需要同时执行大量小任务的情况。
**多进程**:进程是程序中执行任务的独立实体。它们有自己的内存空间,因此通信和同步比多线程更复杂。多进程编程适用于需要同时执行大量耗时任务的情况。
# 2. 多线程编程实战
### 2.1 Python线程基础
#### 2.1.1 线程的概念和创建
**概念:**
线程是轻量级的执行单元,它与进程共享相同的内存空间和资源。线程可以并行执行,从而提高程序的执行效率。
**创建:**
Python中使用`threading`模块创建线程:
```python
import threading
def task():
print("子线程执行")
thread = threading.Thread(target=task)
thread.start()
```
#### 2.1.2 线程的同步和通信
**同步:**
当多个线程同时访问共享资源时,需要进行同步,以避免数据不一致。Python中常用的同步机制有锁和信号量。
**锁:**
锁是一种同步机制,它允许一次只有一个线程访问共享资源。Python中使用`threading.Lock`类实现锁:
```python
import threading
lock = threading.Lock()
def task():
with lock:
# 临界区代码
pass
```
**信号量:**
信号量是一种同步机制,它限制同时访问共享资源的线程数量。Python中使用`threading.Semaphore`类实现信号量:
```python
import threading
semaphore = threading.Semaphore(3)
def task():
with semaphore:
# 临界区代码
pass
```
**通信:**
线程之间可以通过共享变量、队列或管道进行通信。
**共享变量:**
共享变量是线程之间共享的内存空间。使用共享变量进行通信时,需要使用锁进行同步。
**队列:**
队列是一种线程安全的数据结构,它可以存储和检索数据。线程可以通过队列进行通信,而无需使用锁。
**管道:**
管道是一种线程安全的数据流,它允许线程之间传输数据。管道通常用于在不同进程或线程之间进行通信。
### 2.2 多线程编程应用
#### 2.2.1 多线程爬虫
**原理:**
多线程爬虫通过创建多个线程并行抓取网页,提高爬取效率。
**实现:**
```python
import threading
import requests
def crawl(url):
response = requests.get(url)
# 处理网页内容
urls = ["url1", "url2", "url3"]
threads = []
for url in urls:
thread = threading.Thread(target=crawl, args=(url,))
threads.append(thread)
for thread in threads:
thread.start()
for thread in threads:
thread.join()
```
#### 2.2.2 多线程图像处理
**原理:**
多线程图像处理通过创建多个线程并行处理图像,提高图像处理效率。
**实现:**
```python
import threading
from PIL import Image
def process_image(image_path):
image = Image.open(image_path)
# 处理图像
image_paths = ["image1.jpg", "image2.jpg", "image3.jpg"]
threads = []
for image_path in image_paths:
thread = threading.Thread(target=process_image, args=(image_path,))
threads.append(thread)
for thread in threads:
thread.start()
for thread in threads:
thread.join()
```
# 3.1 Python进程基础
#### 3.1.1 进程的概念和创建
**进程的概念**
进程是操作系统中执行的一个程序实例,它拥有自己的地址空间、资源和执行上下文。进程是并发编程的基础,它允许多个
0
0
相关推荐
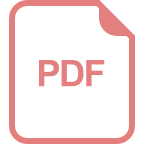
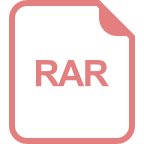
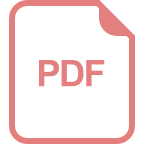
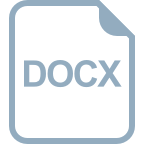
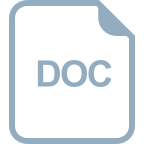
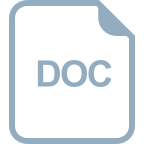
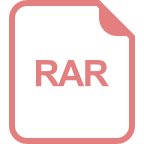
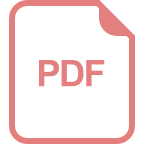
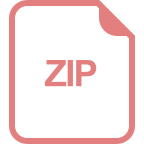