揭秘Trie树的构建原理:从理论到实践(Trie树构建秘籍:从理论到实践)
发布时间: 2024-08-24 03:19:08 阅读量: 9 订阅数: 13 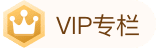
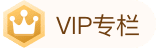
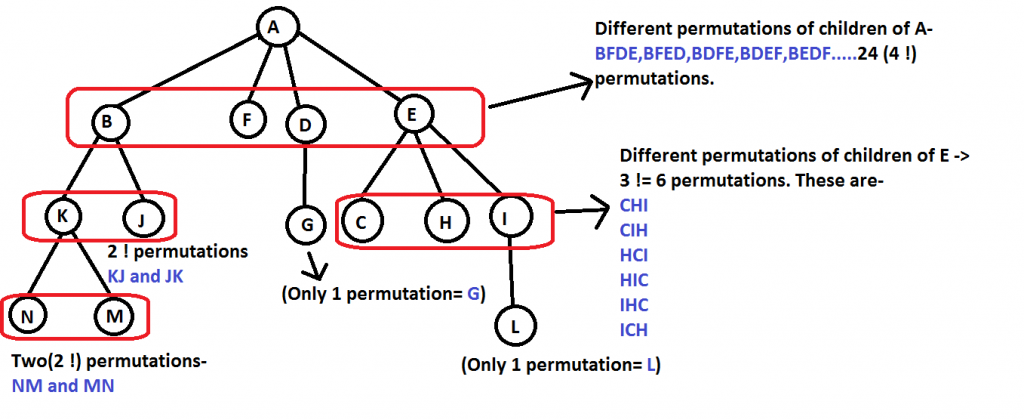
# 1. Trie树的基本原理
Trie树,又称前缀树或单词查找树,是一种高效的数据结构,用于存储字符串集合。其基本原理在于:
- **前缀共享:**Trie树将字符串的前缀存储在同一路径上,从而减少空间占用。例如,字符串"apple"和"application"的前缀"app"将存储在同一节点。
- **快速查找:**Trie树利用前缀共享,可以快速查找字符串。通过沿着前缀路径向下查找,可以高效地确定字符串是否存在或找到匹配的前缀。
# 2. Trie树的构建
### 2.1 递归构建
**2.1.1 递归构建的步骤**
递归构建Trie树遵循以下步骤:
1. **创建根节点:**首先,创建一个根节点,它表示Trie树的起点。
2. **遍历单词:**对于要插入Trie树的每个单词,执行以下步骤:
- 从根节点开始。
- 对于单词中的每个字符:
- 检查根节点是否有指向该字符的子节点。
- 如果有,则移动到该子节点。
- 如果没有,则创建一个指向该字符的新子节点,并移动到该子节点。
3. **标记叶子节点:**当到达单词的最后一个字符时,将当前节点标记为叶子节点,表示单词已插入。
**代码块:**
```python
def insert_recursive(self, word):
"""
Recursively inserts a word into the Trie.
Args:
word (str): The word to insert.
"""
# Check if the word is empty
if not word:
return
# Get the first character of the word
char = word[0]
# Check if the root node has a child for the first character
if char not in self.children:
# If not, create a new child node
self.children[char] = TrieNode()
# Recursively insert the rest of the word into the child node
self.children[char].insert_recursive(word[1:])
# Mark the current node as a leaf node if this is the last character of the word
if len(word) == 1:
self.is_leaf = True
```
**逻辑分析:**
此代码块实现了递归构建Trie树的方法。它首先检查单词是否为空,如果是,则返回。然后,它获取单词的第一个字符并检查根节点是否有指向该字符的子节点。如果没有,它创建一个新的子节点。接下来,它递归地将单词的其余部分插入子节点。最后,如果这是单词的最后一个字符,它将当前节点标记为叶子节点。
**2.1.2 递归构建的复杂度分析**
递归构建Trie树的复杂度为 O(n*m),其中 n 是单词的平均长度,m 是单词的数量。这是因为对于每个单词,我们必须遍历单词中的每个字符,并且我们必须为每个字符创建一个新的节点(如果它不存在)。
### 2.2 迭代构建
**2.2.1 迭代构建的步骤**
迭代构建Trie树遵循以下步骤:
1. **创建根节点:**首先,创建一个根节点,它表示Trie树的起点。
2. **遍历单词:**对于要插入Trie树的每个单词,执行以下步骤:
- 从根节点开始。
- 对于单词中的每个字符:
- 检查当前节点是否有指向该字符的子节点。
- 如果有,则移动到该子节点。
- 如果没有,则创建一个指向该字符的新子节点,并移动到该子节点。
3. **标记叶子节点:**当到达单词的最后一个字符时,将当前节点标记为叶子节点,表示单词已插入。
**代码块:**
```python
def insert_iterative(self, word):
"""
Iteratively inserts a word into the Trie.
Args:
word (str): The word to insert.
"""
# Check if the word is empty
if not word:
return
# Start at the root node
current_node = self
# Iterate over the characters in the word
for char in word:
# Check if the current node has
```
0
0
相关推荐
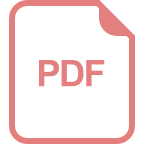
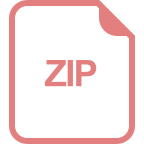
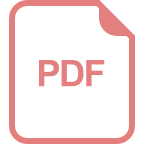
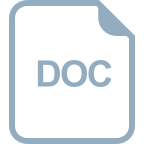
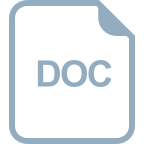
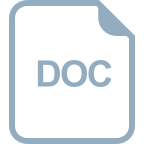
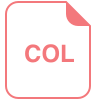
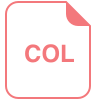
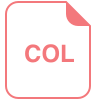