Trie树在文本处理中的应用:高效搜索和文本压缩(文本处理神器:Trie树助你高效搜索和压缩)
发布时间: 2024-08-24 03:08:16 阅读量: 45 订阅数: 34 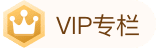
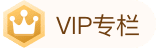
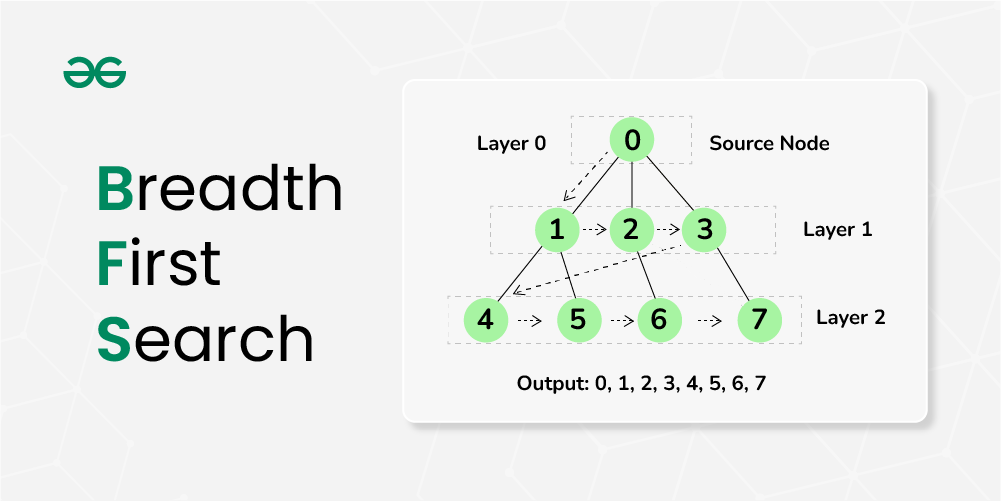
# 1. Trie树的基本概念和原理
Trie树,又称前缀树或字典树,是一种多叉树数据结构,用于高效地存储和检索字符串。它通过将字符串逐个字符插入树中,将每个前缀表示为树中的一个节点。
Trie树的每个节点代表一个字符串前缀,其子节点代表该前缀的扩展。通过这种方式,Trie树可以快速查找字符串的前缀,并支持高效的字符串匹配和搜索操作。例如,在存储单词集合的Trie树中,每个单词的前缀都表示为树中的一个路径,而单词本身则表示为从根节点到叶节点的完整路径。
# 2. Trie树在文本处理中的应用
### 2.1 Trie树在高效搜索中的应用
#### 2.1.1 前缀匹配和模糊搜索
Trie树在文本处理中的一个重要应用是前缀匹配和模糊搜索。前缀匹配是指查找以特定字符串开头的所有字符串,而模糊搜索是指查找与特定字符串相似的字符串。
**前缀匹配**
前缀匹配在搜索引擎、自动补全和拼写检查等应用中非常有用。在Trie树中,前缀匹配可以通过从根节点开始,沿着与前缀字符相对应的边向下遍历来实现。如果遍历到一个叶节点,则表示找到了以该前缀开头的字符串。
```python
def prefix_match(trie, prefix):
"""
在Trie树中进行前缀匹配
参数:
trie: Trie树对象
prefix: 要匹配的前缀字符串
返回:
以prefix开头的所有字符串的列表
"""
current_node = trie.root
for char in prefix:
if char not in current_node.children:
return []
current_node = current_node.children[char]
return current_node.get_all_words()
```
**模糊搜索**
模糊搜索在拼写检查、自然语言处理和文本挖掘等应用中非常有用。在Trie树中,模糊搜索可以通过允许在遍历过程中跳过一个或多个字符来实现。
```python
def fuzzy_search(trie, pattern):
"""
在Trie树中进行模糊搜索
参数:
trie: Trie树对象
pattern: 要匹配的模式字符串
返回:
与pattern相似的所有字符串的列表
"""
def _dfs(node, pattern, i, visited):
if i == len(pattern):
return node.get_all_words()
char = pattern[i]
if char == '?':
# 跳过一个字符
result = []
for child in node.children.values():
if child not in visited:
result += _dfs(child, pattern, i + 1, visited | {child})
return result
else:
# 匹配一个字符
if char in node.children:
return _dfs(node.children[char], pattern, i + 1, visited)
else:
return []
return _dfs(trie.root, pattern, 0, set())
```
#### 2.1.2 字典树和自动补全
字典树是Trie树的一种特殊形式,用于存储单词集合。字典树在自动补全功能中非常有用,它可以快速查找以特定前缀开头的单词。
```python
class DictionaryTree:
"""
字典树类
"""
def __init__(self):
self.root = TrieNode()
def insert(self, word):
"""
向字典树中插入一个单词
参数:
word: 要插入的单词
"""
current_node = self.root
for char in word:
if char not in current_node.children:
current_node.children[char] = TrieNode()
current_node = current_node.children[char]
current_node.is_word = True
def search(self, prefix):
"""
在字典树中查找一个前缀
参数:
prefix: 要查找的前缀
返回:
True if the prefix is in the dictionary tree, False otherwise
"""
current_node = self.root
for char in prefix:
if char not in current_node.children:
return False
current_node = current_node.children[char]
return True
def autocomplete(self, prefix):
"""
自动补全功能
参数:
prefix: 要自动补全的前缀
返回:
以prefix开头的所有单词的列表
"""
current_node = self.root
for char in prefix:
if char not in current_node.children:
return []
current_node = current_node.children[char]
return current_node.get_all_words()
```
### 2.2 Trie树在文本压缩中的应用
#### 2.2.1 哈夫曼编码和哈夫曼树
哈夫曼编码是一种无损数据压缩算法,它利用Trie树来构建哈夫曼树。哈夫曼树的每个叶节点代表一个字符,其权重表示该字符在文本中出现的频率。
```python
class HuffmanNode:
"""
哈夫曼树节点类
"""
def __init__(self, char, freq):
self.char = char
self.freq = freq
self.left = None
self.right = None
def __lt__(self, other):
return self.freq < other.freq
def build_huffman_tree(freq_table):
"""
构建哈夫曼树
参数:
freq_table: 字符及其频率的字典
返回:
哈夫曼树的根节点
"""
nodes = [HuffmanNode(char, freq) for char, freq in freq_table.items()]
while len(nodes) > 1:
nodes.sort()
left = nodes.pop(0)
right = nodes.pop(0)
parent = HuffmanNode(None, left.freq + right.freq)
parent.left = left
parent.right = right
nodes.append(parent)
return nodes[0]
```
#### 2.2.2 Lempel-Ziv算法和LZ77
Lempel-Ziv算法(LZ77)是一种无损数据压缩算法,它利用Trie树来存储重复的字符串。LZ77算法通过将重复的字符串替换为指向Trie树中相应节点的指针来实现压缩。
```python
def lz77_encode(text):
"""
使用LZ77算法对文本进行编码
参数:
text: 要编码的文本
返回:
编码后的文本
"""
trie = Trie()
encoded_text = []
i = 0
while i < len(text):
match = trie.find_longest_match(text, i)
if match is not None:
encoded_text.append((match.length, trie.get_node_id(match.node)))
i += match.length
else:
encoded_text.append(text[i])
trie.insert(text[i])
i += 1
return encoded_text
def lz77_decode(encoded_text):
"""
使用LZ77算法对文本进行解码
参数:
encoded_text: 要解码的编码文本
返回:
解码后的文本
"""
trie = Trie()
decoded_text = []
for length, node_id in encoded_text:
if node_id == -1:
decoded_text.append(length)
else:
node = trie.get_node_by_id(node_id)
decoded_text.append(node.value)
trie.insert(node.value + length)
return ''.join(decoded_text)
```
# 3. Trie树的实现和优化
### 3.1 Trie树的存储结构和算法
Trie树的存储结构主要有两种:数组实现和链表实现。
**数组实现**
数组实现是将Trie树中的每个节点存储在一个数组中,其中每个节点对应数组中的一个元素。节点的索引由其在Trie树中的位置决定。这种实现方式简单高效,适用于节点数量较少的情况。
```python
class TrieNode:
def __init__(self):
self.children = [None] * 26
self.is_word = False
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word):
node = self.root
for char in word:
index = ord(char) - ord('a')
if not node.children[index]:
node.children[index] = TrieNode()
node = node.children[index]
node.is_word = True
```
**链表实现**
链表实现是将Trie树中的每个节点存储在一个链表中。每个节点包含指向其子节点的指针和一个标记,表示该节点是否是一个单词的结尾。这种实现方式适用于节点数量较多的情况,可以节省空间。
```python
class TrieNode:
def __init__(self):
self.children = {}
self.is_word = False
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word):
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_word = True
```
### 3.1.2 压缩存储和空间优化
为了进一步优化Trie树的空间占用,可以采用压缩存储技术。一种常用的方法是**哈夫曼编码**。哈夫曼编码将出现频率较高的字符分配较短的编码,出现频率较低的字符分配较长的编码。
```python
class TrieNode:
def __init__(self):
self.children = {}
self.is_word = False
self.code = None
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word):
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_word = True
def compress(self):
# 计算每个字符的出现频率
char_freq = {}
self._count_chars(self.root, char_freq)
# 根据频率生成哈夫曼编码
codes = {}
self._generate_codes(char_freq, codes)
# 为每个节点分配压缩编码
self._assign_codes(self.root, codes)
def _count_chars(self, node, char_freq):
if node.is_word:
char_freq[""] += 1
for char, child in node.children.items():
char_freq[char] += 1
self._count_chars(child, char_freq)
def _generate_codes(self, char_freq, codes):
# 使用哈夫曼编码生成编码
huffman_tree = HuffmanTree(char_freq)
huffman_tree.build()
for char, code in huffman_tree.codes.items():
codes[char] = code
def _assign_codes(self, node, codes):
if node.is_word:
node.code = codes[""]
for char, child in node.children.items():
child.code = codes[char]
self._assign_codes(child, codes)
```
### 3.2 Trie树的性能优化和并行处理
为了提高Trie树的性能,可以采用多种优化技术。
**缓存机制和预处理**
缓存机制可以将频繁访问的节点存储在内存中,从而减少对磁盘或其他慢速存储介质的访问。预处理可以将一些耗时的操作,如哈夫曼编码,提前进行,从而提高运行时性能。
**多线程和分布式处理**
对于大型Trie树,可以采用多线程或分布式处理技术来提高查询和插入效率。通过将Trie树划分为多个子树,可以在不同的线程或机器上并行处理操作。
```python
import threading
class Trie:
def __init__(self):
self.root = TrieNode()
self.lock = threading.Lock()
def insert(self, word):
with self.lock:
node = self.root
for char in word:
index = ord(char) - ord('a')
if not node.children[index]:
node.children[index] = TrieNode()
node = node.children[index]
node.is_word = True
def search(self, word):
with self.lock:
node = self.root
for char in word:
index = ord(char) - ord('a')
if not node.children[index]:
return False
node = node.children[index]
return node.is_word
```
# 4. Trie树在其他领域的应用
### 4.1 Trie树在网络路由中的应用
#### 4.1.1 路由表存储和查找
Trie树在网络路由中扮演着至关重要的角色,用于存储和查找路由表。路由表包含了网络中每个目的地址对应的下一跳地址。
Trie树的结构非常适合存储路由表。每个节点代表一个网络前缀,子节点则代表更具体的子前缀。通过从根节点开始逐层匹配前缀,可以高效地查找给定目的地址的下一跳地址。
例如,考虑一个路由表:
| 目的地址 | 下一跳地址 |
|---|---|
| 192.168.1.0/24 | 192.168.1.1 |
| 192.168.2.0/24 | 192.168.2.1 |
| 192.168.3.0/24 | 192.168.3.1 |
可以将此路由表存储在 Trie树中,如下所示:
```mermaid
graph LR
subgraph 192.168
A[192.168]
B[1.0] --> A
C[2.0] --> A
D[3.0] --> A
end
```
要查找目的地址 192.168.2.10 的下一跳地址,只需从根节点开始逐层匹配前缀。首先匹配 "192.168",然后匹配 "192.168.2",最终到达节点 "C",对应下一跳地址 192.168.2.1。
#### 4.1.2 前缀匹配和最长前缀匹配
Trie树在网络路由中还用于前缀匹配和最长前缀匹配。前缀匹配是指查找路由表中具有最长匹配前缀的条目。最长前缀匹配是指在路由表中查找具有最长匹配前缀的条目,并将其作为下一跳地址。
例如,考虑一个路由表:
| 目的地址 | 下一跳地址 |
|---|---|
| 192.168.1.0/24 | 192.168.1.1 |
| 192.168.2.0/24 | 192.168.2.1 |
| 192.168.2.10/32 | 192.168.2.10 |
对于目的地址 192.168.2.10,前缀匹配将匹配 "192.168.2.0/24" 条目,而最长前缀匹配将匹配 "192.168.2.10/32" 条目。
### 4.2 Trie树在机器学习中的应用
#### 4.2.1 特征提取和模式识别
Trie树在机器学习中广泛用于特征提取和模式识别。通过将文本或数据转换为 Trie树,可以提取出有用的特征,用于训练机器学习模型。
例如,考虑一个文本数据集,其中包含以下句子:
* 我喜欢吃苹果。
* 我喜欢吃香蕉。
* 我喜欢吃橘子。
可以将此数据集转换为 Trie树,如下所示:
```mermaid
graph LR
A[我]
B[喜欢] --> A
C[吃] --> B
D[苹果] --> C
E[香蕉] --> C
F[橘子] --> C
```
通过遍历 Trie树,可以提取出以下特征:
* 单词频率:每个单词在文本中出现的次数。
* 单词共现:哪些单词经常一起出现。
* 单词顺序:单词在文本中的顺序。
这些特征可以用于训练机器学习模型来识别文本模式,例如情感分析或主题分类。
#### 4.2.2 文本分类和情感分析
Trie树在文本分类和情感分析中也发挥着重要作用。通过将文本转换为 Trie树,可以提取出有用的特征,用于训练机器学习模型来对文本进行分类或分析其情感。
例如,考虑一个包含正面和负面评论的文本数据集。可以将此数据集转换为 Trie树,并提取出以下特征:
* 正面单词频率:文本中正面单词出现的次数。
* 负面单词频率:文本中负面单词出现的次数。
* 正面单词共现:哪些正面单词经常一起出现。
* 负面单词共现:哪些负面单词经常一起出现。
这些特征可以用于训练机器学习模型来对文本进行分类为正面或负面,或分析其情感。
# 5.1 Patricia树和Radix树
### 5.1.1 Patricia树的结构和算法
Patricia树(又称PATRICIA树)是Trie树的一种变种,它通过压缩相邻的相同前缀节点来优化存储空间。Patricia树的每个节点存储一个字符和一个指向子树的指针。如果两个节点具有相同的父节点,并且它们的字符相同,那么它们将合并为一个节点,指向相同的子树。
Patricia树的算法与Trie树类似,但它在插入和查找操作中进行了优化。在插入操作中,如果遇到一个已经存在的节点,则直接将新节点插入到该节点的子树中。在查找操作中,如果遇到一个合并的节点,则可以跳过与该节点字符相同的字符,直接跳转到子树中。
### 5.1.2 Radix树的改进和应用
Radix树是Patricia树的进一步改进,它通过将每个节点存储多个字符来进一步优化存储空间。Radix树的每个节点存储一个字符数组,其中每个字符代表一个子树的分支。
Radix树的算法与Patricia树类似,但它在插入和查找操作中进行了进一步的优化。在插入操作中,如果遇到一个已经存在的字符数组,则直接将新字符添加到数组中。在查找操作中,如果遇到一个Radix树节点,则可以同时比较多个字符,从而加快查找速度。
Patricia树和Radix树在网络路由、IP地址查找和字符串匹配等领域有着广泛的应用。它们可以有效地存储和查找大量字符串,并提供高效的查询性能。
0
0
相关推荐
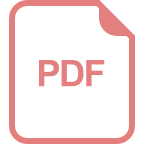
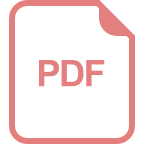
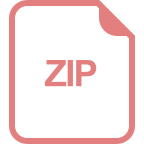





