字典树性能优化技巧:空间和时间复杂度分析,提升效率
发布时间: 2024-08-24 04:19:24 阅读量: 12 订阅数: 20 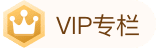
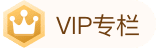
# 1. 字典树简介
字典树,又称前缀树或单词查找树,是一种高效的数据结构,用于存储和检索字符串。它由一系列节点组成,每个节点代表一个字符,节点之间的连接构成一个有向无环图。
字典树的主要优点在于其空间和时间效率。由于前缀共享,它可以有效地存储大量字符串。此外,由于其树形结构,它支持快速查找、插入和删除操作。
# 2. 字典树性能优化技巧
### 2.1 空间优化
#### 2.1.1 节点合并
**优化原理:**
节点合并是一种通过合并具有相同子节点的节点来减少字典树空间消耗的技术。当两个节点具有相同的子节点时,它们可以合并为一个节点,从而减少树中节点的数量。
**代码示例:**
```python
class Node:
def __init__(self, char):
self.char = char
self.children = {}
self.is_word = False
def merge_nodes(node1, node2):
for char, child in node2.children.items():
if char not in node1.children:
node1.children[char] = child
node1.is_word |= node2.is_word
```
**逻辑分析:**
* `merge_nodes` 函数接收两个节点 `node1` 和 `node2`,并将 `node2` 的子节点合并到 `node1` 中。
* 如果 `node2` 的某个子节点不在 `node1` 中,则将其添加到 `node1` 的子节点中。
* 更新 `node1` 的 `is_word` 属性,使其包含 `node1` 和 `node2` 的 `is_word` 属性的逻辑或值。
#### 2.1.2 前缀共享
**优化原理:**
前缀共享是一种通过存储公共前缀来减少字典树空间消耗的技术。当多个单词具有相同的公共前缀时,可以创建一个公共前缀节点来存储该前缀,从而避免重复存储。
**代码示例:**
```python
class Trie:
def __init__(self):
self.root = Node('')
def insert_word(self, word):
current_node = self.root
for char in word:
if char not in current_node.children:
current_node.children[char] = Node(char)
current_node = current_node.children[char]
current_node.is_word = True
```
**逻辑分析:**
* `insert_word` 函数将单词 `word` 插入字典树中。
* 对于单词中的每个字符 `char`,如果 `current_node` 的子节点中没有 `char`,则创建一个新的节点并将其添加到子节点中。
* 将 `current_node` 更新为子节点,并继续遍历单词的剩余字符。
* 当到达单词的最后一个字符时,将 `current_node` 的 `is_word` 属性设置为 `True`。
### 2.2 时间优化
#### 2.2.1 哈希函数
**优化原理:**
哈希函数是一种通过将键映射到固定大小数组中索引的技术。在字典树中,哈希函数可用于快速查找子节点,从而减少查找时间。
**代码示例:**
```python
class Node:
def __init__(self, char):
self.char = char
self.children = {}
self.is_word = False
def get_child(self, char):
return self.children.get(char, None)
```
**逻辑分析:**
* `get_child` 函数使用哈希函数从 `self.children` 字典中获取子节点。
* 如果 `char` 在字典中,则返回相应的子节点;否则,返回 `None`。
#### 2.2.2 字典树的平衡
**优化原理:**
字典树的平衡是一种通过调整节点的顺序来减少搜索时间和空间消耗的技术。平衡的字典树具有更均匀的深度,从而提高了查找效率。
**代码示例:**
```python
class Trie:
def __init__(self):
self.root = Node('')
def balance(self):
self._balance_helper(self.root)
def _balance_helper(self, node):
if not node:
return
children = sorted(node.children.items(), key=lambda x: x[0])
node.children = dict(children)
for _, child in children:
self._balance_helper(child)
```
**逻辑分析:**
* `balance` 函数调用 `_balance_helper` 函数对字典树进行平衡。
* `_balance_helper` 函数对节点的子节点进行排序,并更新节点的子节点字典。
* 该函数递归地对每个子节点调用 `_balance_helper` 函数,从而平衡整个字典树。
#### 2.2.3 懒惰删除
**优化原理:**
懒惰删除是一种通过延迟删除节点来减少删除操作时间的技术。当删除一个单词时,字典树不会立即删除节点,而是将其标记为已删除。在后续查找操作中,已删除的节点将被跳过。
**代码示例:**
```python
class Node:
def __init__(self, char):
self.char = char
self.children
```
0
0
相关推荐
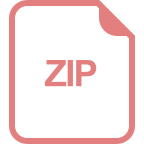
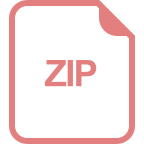
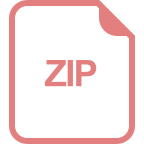





