【MATLAB梯度下降法精通】:从原理到实践的进阶指南
发布时间: 2024-08-30 23:24:44 阅读量: 110 订阅数: 40 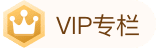
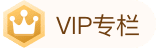
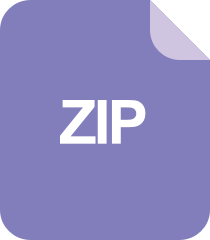
java计算器源码.zip
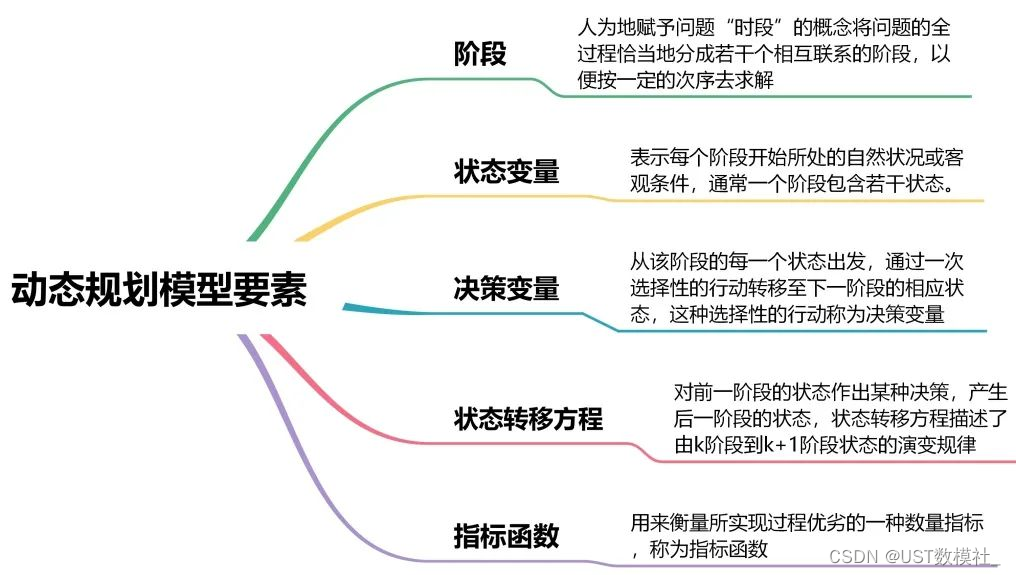
# 1. MATLAB中的梯度下降法概述
## 1.1 梯度下降法简介
梯度下降法是一种迭代优化算法,广泛应用于机器学习和深度学习中,用于最小化损失函数。其基本思想是,根据损失函数相对于模型参数的梯度,调整参数以逐步向最小值靠近。这种方法因其简单性和有效性,成为优化问题的首选算法之一。
## 1.2 梯度下降法的重要性
在MATLAB环境中,梯度下降法不仅用于基本的数值优化,而且是许多高级算法的基础。对于IT专业人士而言,掌握其原理和在MATLAB中的应用是必不可少的技能,对于解决复杂的优化问题有着重要的作用。
## 1.3 梯度下降法的MATLAB实现基础
要在MATLAB中实现梯度下降法,首先需要对目标函数进行定义,然后计算其梯度,并在每次迭代中根据梯度信息来更新参数。参数更新的核心是学习率,这需要谨慎选择,以平衡收敛速度和算法稳定性。下一章将深入探讨梯度下降法的数学原理和MATLAB的具体实现。
# 2. 梯度下降法的数学原理
## 2.1 优化问题与梯度概念
### 2.1.1 优化问题的基本形式
在数学和计算机科学领域,优化问题是指在一组给定的约束条件下,寻找最优解的问题。这里的“最优”可能代表最大、最小或者是一个特定条件下的最佳结果。常见的优化问题包括线性规划、整数规划、非线性规划等。在机器学习和深度学习中,优化问题主要关注的是最小化一个损失函数,通常形式如下:
\[
\min_{\theta} f(\theta)
\]
其中 \( f \) 代表损失函数,\( \theta \) 代表模型参数。
在机器学习中,损失函数衡量的是模型预测值与真实值之间的差异。通过最小化损失函数,我们可以得到一个较好的模型参数设置。
### 2.1.2 梯度的定义与几何意义
梯度是多元函数在某一点的方向导数中最大的那个,即函数在这一点上沿着梯度的方向增加最快。数学上,对于函数 \( f(x_1, x_2, \ldots, x_n) \),其梯度是一个向量,由函数在每个变量方向上的偏导数组成:
\[
\nabla f(x_1, x_2, \ldots, x_n) = \left( \frac{\partial f}{\partial x_1}, \frac{\partial f}{\partial x_2}, \ldots, \frac{\partial f}{\partial x_n} \right)^T
\]
几何上,梯度向量指向的是函数增长最快的方向。如果将函数 \( f \) 视为一个山坡,那么梯度就是山坡最陡峭的方向。
在优化问题中,梯度扮演了重要角色,它用于指导我们如何更新模型参数以减少损失函数的值。在数学中,梯度与优化的关系可以表示为:
\[
\theta_{new} = \theta_{old} - \alpha \nabla f(\theta_{old})
\]
这里 \( \alpha \) 是学习率,一个超参数,控制了参数更新的步长。
## 2.2 梯度下降法的工作机制
### 2.2.1 基本梯度下降算法流程
梯度下降算法是优化问题中最基本的迭代方法。它遵循以下步骤:
1. 初始化参数 \( \theta \)。
2. 计算损失函数关于参数的梯度 \( \nabla f(\theta) \)。
3. 更新参数 \( \theta_{new} = \theta_{old} - \alpha \nabla f(\theta_{old}) \)。
4. 重复步骤2和3直到收敛。
整个过程是通过不断迭代更新模型参数,目的是寻找损失函数的最小值。
### 2.2.2 学习率的选择与影响
学习率 \( \alpha \) 是影响梯度下降算法性能的关键超参数。如果学习率选择得太小,会导致学习过程缓慢,需要很多迭代次数才能收敛;如果选择太大,则可能会导致算法无法收敛,甚至震荡。
因此,选择合适的学习率是实现有效梯度下降的关键。
### 2.2.3 梯度下降的变种算法
标准的梯度下降法有多种变种,其中包括批量梯度下降、随机梯度下降、小批量梯度下降等。这些变种算法通过不同的策略来选择数据样本,从而计算梯度,主要差异在于计算效率和收敛速度。
### 2.3 数学原理的MATLAB实现
#### 2.3.1 梯度的MATLAB计算
MATLAB提供了许多工具来计算梯度。这里,我们将使用符号计算的方式。假设我们有一个二次函数 \( f(x, y) = x^2 + y^2 \),我们可以用以下的MATLAB代码来计算其梯度:
```matlab
syms x y; % 定义符号变量
f = x^2 + y^2; % 定义函数
grad_f = gradient(f, [x, y]); % 计算梯度
disp(grad_f); % 显示梯度结果
```
执行上述代码后,我们会得到函数 \( f(x, y) \) 的梯度 \( [2x, 2y]^T \)。
#### 2.3.2 梯度下降算法的MATLAB代码
下面是一个在MATLAB中实现的基本梯度下降算法的示例代码,用于最小化函数 \( f(x) = x^2 \):
```matlab
% 定义目标函数
f = @(x) x^2;
% 定义梯度计算函数
grad_f = @(x) 2*x;
% 初始化参数
x_old = 10;
% 设置学习率
alpha = 0.1;
% 设置迭代次数
num_iter = 100;
% 迭代过程
for i = 1:num_iter
% 更新参数
x_new = x_old - alpha * grad_f(x_old);
% 打印迭代信息
fprintf('迭代次数: %d, 参数值: %f\n', i, x_new);
% 更新旧参数值
x_old = x_new;
end
```
上述代码将输出每次迭代后参数 \( x \) 的值,并最终接近于该函数的最小值点,即 \( x = 0 \)。
以上内容详细介绍了梯度下降法的数学原理,包括优化问题的基本形式、梯度定义与几何意义,以及梯度下降法的工作机制和在MATLAB中的实现。通过这些内容的学习,我们可以为梯度下降法的实践应用打下坚实的基础。
# 3. 梯度下降法在MATLAB中的实践应用
## 3.1 线性回归问题的梯度下降实现
### 3.1.1 线性回归模型的构建
在众多的机器学习算法中,线性回归是最基本和最简单的模型之一。它假设因变量与一个或多个自变量之间存在线性关系。数学上,线性回归模型通常表示为:
y = θ0 + θ1x1 + θ2x2 + ... + θnxn
其中,y 是因变量(我们要预测的值),x1 到 xn 是自变量(特征),θ0 到 θn 是模型参数。线性回归的目标是找到一组参数θ,使得预测值 y 和真实值之间的误差最小。
### 3.1.2 梯度下降法解决线性回归
梯度下降法可用于最小化线性回归的成本函数,即最小化预测值和实际值之间差的平方和(SSE,Sum of Squared Errors)。
我们定义成本函数 J(θ) 如下:
J(θ) = 1/2m ∑(hθ(xi) - yi)²
其中,hθ(xi) 是使用当前参数θ对第i个数
0
0