【OpenCV小车巡线实战指南】:从零开始打造你的自主巡线小车
发布时间: 2024-08-13 19:20:19 阅读量: 674 订阅数: 40 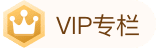
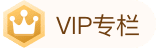
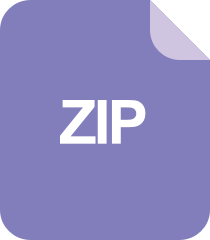
《永磁无刷直流电机控制系统与软件综合研究-集成电机计算软件、电机控制器及电磁设计软件的创新设计与实践》,永磁无刷直流电机计算与控制软件:高效电机控制器与电磁设计工具,永磁无刷直流电机计算软件,电机控

# 1. OpenCV基础**
OpenCV(Open Source Computer Vision Library)是一个开源计算机视觉库,为图像处理、计算机视觉和机器学习提供了广泛的算法和函数。它支持多种编程语言,包括C++、Python和Java。
OpenCV图像处理功能包括:
- 图像读取和写入
- 图像转换(如灰度化、二值化、腐蚀和膨胀)
- 图像增强(如锐化、平滑和对比度调整)
- 特征提取(如边缘检测、角点检测和轮廓提取)
# 2. 巡线算法理论
### 2.1 PID控制原理
PID控制是一种经典的反馈控制算法,广泛应用于各种自动控制系统中。其原理是通过测量系统输出与期望值之间的偏差,并根据偏差的大小和变化率来调整控制信号,从而使系统输出接近期望值。
#### 2.1.1 比例项、积分项、微分项
PID控制算法由三个基本项组成:比例项、积分项和微分项。
- **比例项 (P)**:与偏差成正比,即偏差越大,控制信号调整越大。
- **积分项 (I)**:与偏差的积分成正比,即偏差持续存在,控制信号将不断调整,直到偏差消除。
- **微分项 (D)**:与偏差的变化率成正比,即偏差变化越快,控制信号调整越大。
#### 2.1.2 PID参数的调优
PID控制算法的性能很大程度上取决于其参数的调优。参数调优的目标是找到一组参数,使系统响应快速、稳定且无超调。常用的调优方法包括:
- **试错法**:通过不断调整参数,观察系统响应,直到达到满意的效果。
- **齐格勒-尼科尔斯法**:基于系统阶跃响应,计算出参数的初始值,再进行微调。
- **遗传算法**:利用遗传算法优化参数,找到最优解。
### 2.2 图像处理技术
图像处理技术在巡线算法中扮演着至关重要的角色,它可以将原始图像转换为适合算法处理的形式。
#### 2.2.1 灰度化、二值化、腐蚀膨胀
- **灰度化**:将彩色图像转换为灰度图像,减少图像信息量。
- **二值化**:将灰度图像转换为二值图像,只有黑色和白色两种像素。
- **腐蚀膨胀**:通过形态学操作,去除图像中细小的噪声或填充空洞。
#### 2.2.2 轮廓提取、直线拟合
- **轮廓提取**:从二值图像中提取目标对象的边界。
- **直线拟合**:通过最小二乘法或其他算法,将轮廓拟合成一条直线。
# 3. 巡线小车硬件搭建
### 3.1 电路设计与元器件选型
巡线小车的电路设计主要包括电机驱动电路、传感器接口电路和电源电路。
**3.1.1 电机驱动电路**
电机驱动电路负责控制小车的电机转动,以实现小车的移动。常用的电机驱动芯片有L298N、TB6612FNG等。
```c++
// 使用 L298N 驱动电机
void motor_control(int left_speed, int right_speed) {
// 设置左电机速度
digitalWrite(L298N_ENA, HIGH);
digitalWrite(L298N_IN1, left_speed > 0 ? HIGH : LOW);
digitalWrite(L298N_IN2, left_speed < 0 ? HIGH : LOW);
analogWrite(L298N_PWM1, abs(left_speed));
// 设置右电机速度
digitalWrite(L298N_ENB, HIGH);
digitalWrite(L298N_IN3, right_speed > 0 ? HIGH : LOW);
digitalWrite(L298N_IN4, right_speed < 0 ? HIGH : LOW);
analogWrite(L298N_PWM2, abs(right_speed));
}
```
**参数说明:**
* `left_speed`:左电机速度,正值表示向前转,负值表示向后转
* `right_speed`:右电机速度,正值表示向前转,负值表示向后转
**3.1.2 传感器接口电路**
传感器接口电路负责将传感器信号转换为可被微控制器识别的信号。常用的传感器接口电路有ADC转换电路、比较器电路等。
```c++
// 使用 ADC 转换传感器信号
int adc_read(int channel) {
// 设置 ADC 通道
ADMUX = (ADMUX & 0xF0) | (channel & 0x0F);
// 启动 ADC 转换
ADCSRA |= (1 << ADSC);
// 等待转换完成
while (ADCSRA & (1 << ADSC));
// 读取转换结果
return ADC;
}
```
**参数说明:**
* `channel`:ADC 通道号
### 3.2 车体组装与调试
**3.2.1 机械结构设计**
巡线小车的机械结构主要包括车架、轮子、电机和传感器。车架可以采用亚克力板、铝合金等材料制作,轮子可以选择橡胶轮或尼龙轮。电机和传感器需要根据具体需求选择合适的型号。
**3.2.2 电机安装与校准**
电机安装需要保证电机与轮子之间的同轴度,以避免小车运行时出现抖动。电机校准需要调整电机的位置和角度,以确保小车在直线行驶时不会偏离航线。
```mermaid
sequenceDiagram
participant User
participant Motor
User->Motor: Send command to move forward
Motor->User: Receive command
Motor: Set motor speed
Motor: Move forward
```
**流程图说明:**
该流程图展示了用户向电机发送移动命令,电机接收命令并设置电机速度,最终电机驱动小车向前移动的过程。
# 4.1 OpenCV图像处理实现
### 4.1.1 图像采集与预处理
**图像采集**
```python
import cv2
# 打开摄像头
cap = cv2.VideoCapture(0)
# 循环读取帧
while True:
# 读取一帧图像
ret, frame = cap.read()
# 如果读取失败,退出循环
if not ret:
break
# 显示图像
cv2.imshow('frame', frame)
# 按下 'q' 键退出循环
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 释放摄像头
cap.release()
# 销毁所有窗口
cv2.destroyAllWindows()
```
**代码逻辑分析:**
1. 导入 OpenCV 库。
2. 打开摄像头,并创建一个视频捕捉对象。
3. 循环读取帧,直到读取失败或按下 'q' 键退出。
4. 显示当前帧。
5. 释放摄像头并销毁所有窗口。
**参数说明:**
* `cap.read()`:读取一帧图像,返回一个布尔值 `ret` 表示是否读取成功和一个图像 `frame`。
* `cv2.imshow()`:显示图像。
* `cv2.waitKey()`:等待按键输入,返回按下的键的 ASCII 码。
**图像预处理**
```python
# 灰度化
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 二值化
thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)[1]
# 腐蚀
kernel = np.ones((5, 5), np.uint8)
eroded = cv2.erode(thresh, kernel)
# 膨胀
dilated = cv2.dilate(eroded, kernel)
```
**代码逻辑分析:**
1. 将图像转换为灰度图像。
2. 对灰度图像进行二值化,阈值设为 127。
3. 对二值图像进行腐蚀操作,去除噪声。
4. 对腐蚀后的图像进行膨胀操作,恢复轮廓。
**参数说明:**
* `cv2.cvtColor()`:将图像转换为指定的颜色空间。
* `cv2.threshold()`:对图像进行阈值化。
* `cv2.erode()`:对图像进行腐蚀操作。
* `cv2.dilate()`:对图像进行膨胀操作。
* `kernel`:腐蚀和膨胀操作的内核。
### 4.1.2 巡线目标识别
**轮廓提取**
```python
# 查找轮廓
contours, hierarchy = cv2.findContours(dilated, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
```
**代码逻辑分析:**
1. 在膨胀后的图像中查找轮廓。
2. `cv2.RETR_EXTERNAL` 表示只查找外部轮廓。
3. `cv2.CHAIN_APPROX_SIMPLE` 表示只存储轮廓的端点。
**参数说明:**
* `cv2.findContours()`:查找图像中的轮廓。
* `contours`:存储轮廓的列表。
* `hierarchy`:存储轮廓层次结构的列表。
**直线拟合**
```python
# 找到最大的轮廓
max_contour = max(contours, key=cv2.contourArea)
# 拟合直线
(x, y), (MA, ma), angle = cv2.fitLine(max_contour, cv2.DIST_L2, 0, 0.01, 0.01)
```
**代码逻辑分析:**
1. 根据轮廓面积找到最大的轮廓。
2. 使用最小二乘法拟合直线。
3. `cv2.DIST_L2` 表示使用欧氏距离作为度量。
4. `0, 0.01, 0.01` 表示拟合直线的参数。
**参数说明:**
* `cv2.contourArea()`:计算轮廓的面积。
* `cv2.fitLine()`:拟合直线。
* `(x, y)`:拟合直线的起点。
* `(MA, ma)`:拟合直线的斜率和截距。
* `angle`:拟合直线的角度。
# 5. 巡线小车实战应用**
**5.1 障碍物避障算法**
巡线小车在实际应用中,难免会遇到障碍物阻挡,因此需要设计障碍物避障算法来保证小车的安全运行。
**5.1.1 超声波传感器检测**
超声波传感器是一种常见的障碍物检测设备,它通过发射超声波并接收反射波来测量障碍物与传感器的距离。
**代码块:**
```python
import RPi.GPIO as GPIO
import time
# 超声波传感器引脚定义
TRIG_PIN = 23
ECHO_PIN = 24
# 设置GPIO模式
GPIO.setmode(GPIO.BCM)
# 设置超声波传感器引脚模式
GPIO.setup(TRIG_PIN, GPIO.OUT)
GPIO.setup(ECHO_PIN, GPIO.IN)
# 距离计算函数
def get_distance():
# 发射超声波脉冲
GPIO.output(TRIG_PIN, True)
time.sleep(0.00001)
GPIO.output(TRIG_PIN, False)
# 等待回波信号
while GPIO.input(ECHO_PIN) == 0:
pass
# 记录回波信号开始时间
start_time = time.time()
# 等待回波信号结束
while GPIO.input(ECHO_PIN) == 1:
pass
# 记录回波信号结束时间
end_time = time.time()
# 计算距离
distance = (end_time - start_time) * 34300 / 2
return distance
# 清理GPIO
GPIO.cleanup()
```
**5.1.2 路径规划与避障策略**
当超声波传感器检测到障碍物时,巡线小车需要根据当前位置和障碍物位置进行路径规划和避障策略。
**代码块:**
```python
import numpy as np
# 路径规划函数
def path_planning(current_position, obstacle_position):
# 计算障碍物与当前位置的相对位置
relative_position = obstacle_position - current_position
# 根据相对位置确定避障策略
if relative_position[0] > 0: # 障碍物在右侧
new_position = current_position + np.array([0, -1])
elif relative_position[0] < 0: # 障碍物在左侧
new_position = current_position + np.array([0, 1])
else: # 障碍物在前方
new_position = current_position + np.array([1, 0])
return new_position
```
**5.2 无线通信与远程控制**
为了方便巡线小车的远程控制和数据传输,可以采用蓝牙或WiFi通信模块。
**5.2.1 蓝牙或WiFi通信模块**
**代码块:**
```python
import bluetooth
# 蓝牙通信模块初始化
bluetooth.init()
# 查找蓝牙设备
devices = bluetooth.discover_devices()
# 连接到指定设备
target_device = "00:11:22:33:44:55"
bluetooth.connect(target_device)
```
**5.2.2 远程控制界面设计**
远程控制界面可以通过网页或移动应用程序实现,提供对巡线小车的控制和数据显示功能。
**代码块:**
```html
<!DOCTYPE html>
<html>
<head>
<title>巡线小车远程控制</title>
</head>
<body>
<button onclick="forward()">前进</button>
<button onclick="backward()">后退</button>
<button onclick="left()">左转</button>
<button onclick="right()">右转</button>
</body>
</html>
```
0
0