【坐标图入门指南】:绘制清晰易懂的坐标图,提升数据可视化技能
发布时间: 2024-07-13 03:00:12 阅读量: 49 订阅数: 36 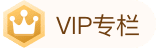
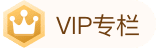
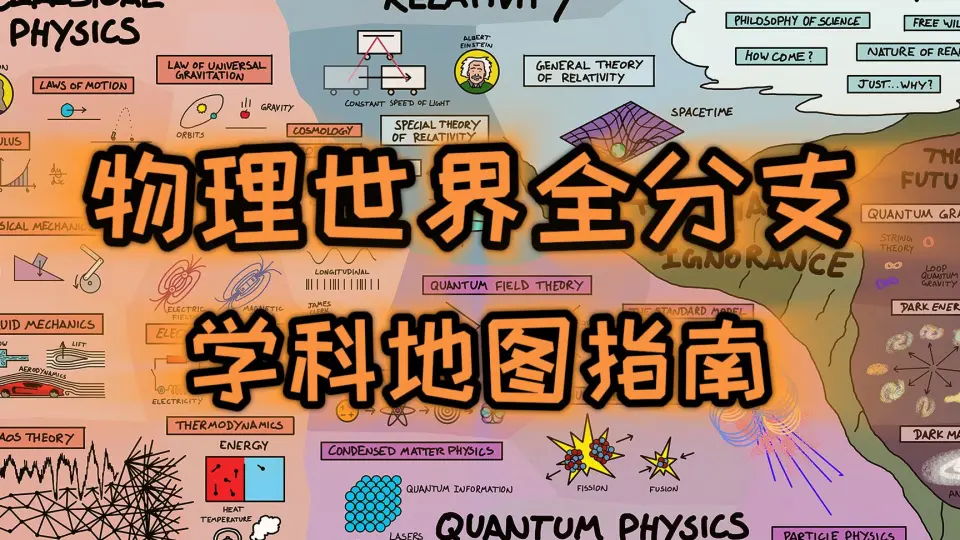
# 1. 坐标图基础理论
坐标图是一种强大的数据可视化工具,它通过在二维或三维空间中绘制数据点来展示数据。其基本原理是将数据值映射到坐标轴上,从而创建可视化表示。
坐标图由以下关键元素组成:
- **坐标轴:**定义数据点的参考框架,通常使用水平(x 轴)和垂直(y 轴)轴。
- **刻度:**沿坐标轴显示的标记,表示数据值的范围和间隔。
- **数据点:**表示单个数据值的点,通常用点、线或条形图表示。
# 2. 坐标图实践技巧
### 2.1 坐标系的建立和配置
#### 2.1.1 坐标轴的定义和刻度设置
**代码块:**
```python
import matplotlib.pyplot as plt
# 创建一个坐标系
fig, ax = plt.subplots()
# 设置 x 轴和 y 轴的标签
ax.set_xlabel("X 轴")
ax.set_ylabel("Y 轴")
# 设置 x 轴和 y 轴的刻度
ax.set_xticks([0, 1, 2, 3, 4])
ax.set_yticks([0, 2, 4, 6, 8])
# 显示坐标系
plt.show()
```
**逻辑分析:**
* `plt.subplots()` 创建一个包含一个坐标系的图形。
* `ax.set_xlabel()` 和 `ax.set_ylabel()` 设置 x 轴和 y 轴的标签。
* `ax.set_xticks()` 和 `ax.set_yticks()` 设置 x 轴和 y 轴的刻度。
* `plt.show()` 显示坐标系。
#### 2.1.2 坐标系范围的调整和缩放
**代码块:**
```python
import matplotlib.pyplot as plt
# 创建一个坐标系
fig, ax = plt.subplots()
# 设置 x 轴和 y 轴的范围
ax.set_xlim([0, 5])
ax.set_ylim([0, 10])
# 缩放 x 轴和 y 轴
ax.set_xscale("log")
ax.set_yscale("log")
# 显示坐标系
plt.show()
```
**逻辑分析:**
* `ax.set_xlim()` 和 `ax.set_ylim()` 设置 x 轴和 y 轴的范围。
* `ax.set_xscale()` 和 `ax.set_yscale()` 缩放 x 轴和 y 轴。
* `log` 缩放表示以对数刻度显示轴。
### 2.2 数据的可视化呈现
#### 2.2.1 散点图和折线图的绘制
**代码块:**
```python
import matplotlib.pyplot as plt
# 创建一个坐标系
fig, ax = plt.subplots()
# 绘制散点图
ax.scatter(x, y)
# 绘制折线图
ax.plot(x, y)
# 显示坐标系
plt.show()
```
**逻辑分析:**
* `ax.scatter()` 绘制散点图,其中 `x` 和 `y` 是数据点。
* `ax.plot()` 绘制折线图,其中 `x` 和 `y` 是折线上的数据点。
#### 2.2.2 柱状图和饼图的绘制
**代码块:**
```python
import matplotlib.pyplot as plt
# 创建一个坐标系
fig, ax = plt.subplots()
# 绘制柱状图
ax.bar(x, y)
# 绘制饼图
ax.pie(y)
# 显示坐标系
plt.show()
```
**逻辑分析:**
* `ax.bar()` 绘制柱状图,其中 `x` 是柱状图的类别,`y` 是柱状图的高度。
* `ax.pie()` 绘制饼图,其中 `y` 是饼图中每个扇区的百分比。
#### 2.2.3 混合图表的创建和定制
**代码块:**
```python
import matplotlib.pyplot as plt
# 创建一个坐标系
fig, ax = plt.subplots()
# 绘制散点图和折线图
ax.scatter(x, y1)
ax.plot(x, y2)
# 设置图例
ax.legend(["散点图", "折线图"])
# 显示坐标系
plt.show()
```
**逻辑分析:**
* `ax.scatter()` 和 `ax.plot()` 绘制散点图和折线图。
* `ax.legend()` 添加一个图例,其中包含散点图和折线图的标签。
### 2.3 图表的交互和美化
#### 2.3.1 图例和标签的添加
**代码块:**
```python
import matplotlib.pyplot as plt
# 创建一个坐标系
fig, ax = plt.subplots()
# 绘制散点图
ax.scatter(x, y)
# 添加图例
ax.legend(["散点图"])
# 添加标签
ax.set_xlabel("X 轴")
ax.set_ylabel("Y 轴")
# 显示坐标系
plt.show()
```
**逻辑分析:**
* `ax.legend()` 添加一个图例,其中包含散点图的标签。
* `ax.set_xlabel()` 和 `ax.set_ylabel()` 设置 x 轴和 y 轴的标签。
#### 2.3.2 颜色和样式的自定义
**代码块:**
```python
import matplotlib.pyplot as plt
# 创建一个坐标系
fig, ax = plt.subplots()
# 绘制散点图
ax.scatter(x, y, color="red", marker="o")
# 设置线宽和线型
ax.plot(x, y, color="blue", linewidth=2, linestyle="--")
# 显示坐标系
plt.show()
```
**逻辑分析:**
* `color` 参数设置散点图和折线图的颜色。
* `marker` 参数设置散点图的形状。
* `linewidth` 和 `linestyle` 参数设置折线图的线宽和线型。
#### 2.3.3 图表的导出和保存
**代码块:**
```python
import matplotlib.pyplot as plt
# 创建一个坐标系
fig, ax = plt.subplots()
# 绘制散点图
ax.scatter(x, y)
# 保存图表为 PNG 文件
plt.savefig("scatter_plot.png")
# 显示图表
plt.show()
```
**逻辑分析:**
* `plt.savefig()` 将图表保存为 PNG 文件。
* `plt.show()` 显示图表。
# 3. 坐标图实践应用
坐标图在科学、商业和个人领域都有着广泛的应用。本章将探讨坐标图在这些领域的具体应用场景,展示其在数据分析、可视化和决策制定中的强大功能。
### 3.1 科学数据可视化
坐标图是科学数据可视化的有力工具。通过将实验或统计数据绘制成图表,科学家可以轻松识别趋势、模式和异常值。
#### 3.1.1 实验数据的绘制和分析
在实验科学中,坐标图可用于绘制实验数据,以便进行分析和比较。例如,研究人员可以将不同变量(如温度或浓度)对实验结果(如反应速率或产物产量)的影响绘制成折线图或散点图。通过观察图表,他们可以识别变量之间的关系,确定最佳条件并得出结论。
```python
import matplotlib.pyplot as plt
# 实验数据
temperatures = [10, 20, 30, 40, 50]
reaction_rates = [0.1, 0.2, 0.4, 0.8, 1.6]
# 绘制折线图
plt.plot(temperatures, reaction_rates)
plt.xlabel("温度 (℃)")
plt.ylabel("反应速率")
plt.title("温度对反应速率的影响")
plt.show()
```
**代码逻辑分析:**
* `plt.plot(temperatures, reaction_rates)`:绘制折线图,其中 `temperatures` 为 x 轴数据,`reaction_rates` 为 y 轴数据。
* `plt.xlabel("温度 (℃)")` 和 `plt.ylabel("反应速率")`:设置 x 轴和 y 轴标签。
* `plt.title("温度对反应速率的影响")`:设置图表标题。
* `plt.show()`:显示图表。
#### 3.1.2 统计数据的呈现和建模
在统计学中,坐标图可用于呈现和建模统计数据。例如,研究人员可以将人口分布绘制成直方图或频率分布图,以了解数据的分布情况。此外,他们还可以使用散点图或回归线来探索变量之间的关系,并建立预测模型。
```python
import numpy as np
import matplotlib.pyplot as plt
# 统计数据
data = np.random.normal(100, 15, 1000)
# 绘制直方图
plt.hist(data, bins=20)
plt.xlabel("数据值")
plt.ylabel("频率")
plt.title("数据分布")
plt.show()
```
**代码逻辑分析:**
* `np.random.normal(100, 15, 1000)`:生成 1000 个服从正态分布的数据点,平均值为 100,标准差为 15。
* `plt.hist(data, bins=20)`:绘制直方图,将数据分成 20 个箱。
* `plt.xlabel("数据值")` 和 `plt.ylabel("频率")`:设置 x 轴和 y 轴标签。
* `plt.title("数据分布")`:设置图表标题。
* `plt.show()`:显示图表。
### 3.2 商业数据可视化
坐标图在商业领域也发挥着至关重要的作用,帮助企业分析销售数据、市场趋势和客户行为。
#### 3.2.1 销售数据的分析和预测
销售数据可视化对于了解销售业绩、识别增长机会和预测未来趋势至关重要。通过将销售数据绘制成折线图、柱状图或饼图,企业可以快速识别畅销产品、季节性趋势和区域差异。此外,他们还可以使用回归分析或机器学习算法来建立预测模型,以预测未来的销售额。
```python
import pandas as pd
import matplotlib.pyplot as plt
# 销售数据
sales_data = pd.read_csv("sales_data.csv")
# 绘制折线图
plt.plot(sales_data["Date"], sales_data["Sales"])
plt.xlabel("日期")
plt.ylabel("销售额")
plt.title("销售趋势")
plt.show()
```
**代码逻辑分析:**
* `pd.read_csv("sales_data.csv")`:从 CSV 文件中读取销售数据。
* `plt.plot(sales_data["Date"], sales_data["Sales"])`:绘制折线图,其中 `sales_data["Date"]` 为 x 轴数据,`sales_data["Sales"]` 为 y 轴数据。
* `plt.xlabel("日期")` 和 `plt.ylabel("销售额")`:设置 x 轴和 y 轴标签。
* `plt.title("销售趋势")`:设置图表标题。
* `plt.show()`:显示图表。
#### 3.2.2 市场数据的监测和评估
市场数据可视化对于监测市场趋势、评估营销活动和制定竞争策略至关重要。通过将市场数据绘制成雷达图、漏斗图或散点图,企业可以了解市场份额、客户满意度和竞争对手的优势。此外,他们还可以使用数据挖掘技术来识别市场机会和制定针对性的营销活动。
```python
import pandas as pd
import matplotlib.pyplot as plt
# 市场数据
market_data = pd.read_csv("market_data.csv")
# 绘制雷达图
plt.radar_chart(market_data[["Market Share", "Customer Satisfaction", "Competitor Advantage"]])
plt.title("市场数据雷达图")
plt.show()
```
**代码逻辑分析:**
* `pd.read_csv("market_data.csv")`:从 CSV 文件中读取市场数据。
* `plt.radar_chart(market_data[["Market Share", "Customer Satisfaction", "Competitor Advantage"]])`:绘制雷达图,其中 `market_data[["Market Share", "Customer Satisfaction", "Competitor Advantage"]]` 为雷达图的数据。
* `plt.title("市场数据雷达图")`:设置图表标题。
* `plt.show()`:显示图表。
### 3.3 个人数据可视化
坐标图也为个人提供了可视化和分析个人数据的机会。
#### 3.3.1 健康数据的追踪和管理
健康数据可视化可以帮助个人追踪他们的健康状况,管理慢性疾病并做出明智的健康决策。通过将健康数据绘制成折线图、条形图或饼图,个人可以监控体重、血压、睡眠模式和营养摄入情况。此外,他们还可以使用数据分析工具来识别趋势、设定目标并制定健康计划。
```python
import pandas as pd
import matplotlib.pyplot as plt
# 健康数据
health_data = pd.read_csv("health_data.csv")
# 绘制折线图
plt.plot(health_data["Date"], health_data["Weight"])
plt.xlabel("日期")
plt.ylabel("体重 (kg)")
plt.title("体重追踪")
plt.show()
```
**代码逻辑分析:**
* `pd.read_csv("health_data.csv")`:从 CSV 文件中读取健康数据。
* `plt.plot(health_data["Date"], health_data["Weight"])`:绘制折线图,其中 `health_data["Date"]` 为 x 轴数据,`health_data["Weight"]` 为 y 轴数据。
* `plt.xlabel("日期")` 和 `plt.ylabel("体重 (kg)")`:设置 x 轴和 y 轴标签。
* `plt.title("体重追踪")`:设置图表标题。
* `plt.show()`:显示图表。
#### 3.3.2 旅行数据的记录和分享
旅行数据可视化可以帮助个人记录和分享他们的旅行经历。通过将旅行数据绘制成地图、时间线或照片墙,个人可以创建交互式旅行日志,展示他们的行程、经历和见闻。此外,他们还可以使用社交媒体平台来分享他们的旅行数据,与朋友和家人联系。
```python
import folium
import pandas as pd
# 旅行数据
travel_data = pd.read_csv("travel_data.csv")
# 创建地图
map = folium.Map(location=[travel_data["Latitude"].mean(), travel_data["Longitude"].mean()], zoom_start=10)
# 添加标记
for i in range(len(travel_data)):
folium.Marker([travel_data["Latitude"][i], travel_data["Longitude"][i]], popup=travel_data["Location"][i]).add_to(map)
# 保存地图
map.save("travel_map.html")
```
**代码逻辑分析:**
* `folium.Map(location=[travel_data["Latitude"].mean(), travel_data["Longitude"].mean()], zoom_start=10)`:创建地图,其中 `travel_data["Latitude"].mean()` 和 `travel_data["Longitude"].mean()` 为地图的中心坐标,`zoom
# 4. 坐标图进阶应用
### 4.1 动态坐标图的实现
#### 4.1.1 实时数据的更新和显示
动态坐标图可以实时更新数据,从而展示不断变化的信息。这对于监控系统、数据分析和交互式可视化应用至关重要。
**实现方法:**
* **使用数据流技术:**Kafka、RabbitMQ等数据流技术可以提供实时数据流。坐标图可以订阅这些数据流,并根据接收到的数据更新显示。
* **使用轮询机制:**定期从数据源(如数据库、API)获取更新的数据。如果数据发生变化,则更新坐标图。
**代码示例:**
```python
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
# 数据流模拟
data_stream = [0]
# 创建坐标图
fig, ax = plt.subplots()
ax.set_xlim(0, 100)
ax.set_ylim(0, 100)
line, = ax.plot([], [], lw=2)
# 更新数据函数
def update(frame):
data_stream.append(data_stream[-1] + 1)
line.set_data(range(len(data_stream)), data_stream)
return line,
# 启动动画
ani = FuncAnimation(fig, update, interval=100, blit=True)
plt.show()
```
**逻辑分析:**
* `data_stream`模拟一个实时数据流,每隔100毫秒增加1。
* `update()`函数更新坐标图的数据和显示。
* `FuncAnimation()`函数启动动画,每隔100毫秒调用`update()`函数更新坐标图。
#### 4.1.2 交互式坐标图的创建
交互式坐标图允许用户与坐标图进行交互,例如缩放、平移和选择数据点。
**实现方法:**
* **使用事件处理:**坐标图库通常提供事件处理机制,允许用户在坐标图上触发事件(如鼠标点击、拖动)。
* **使用第三方库:**Plotly、Bokeh等第三方库提供开箱即用的交互式坐标图功能。
**代码示例:**
```python
import plotly.graph_objs as go
# 数据
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# 创建交互式坐标图
fig = go.Figure(data=[go.Scatter(x=x, y=y)])
fig.update_layout(dragmode='pan', hovermode='closest')
fig.show()
```
**逻辑分析:**
* `dragmode='pan'`允许用户平移坐标图。
* `hovermode='closest'`在用户悬停在数据点上时显示提示信息。
### 4.2 三维坐标图的绘制
#### 4.2.1 三维坐标系的建立和配置
三维坐标系允许可视化三维数据。它由x、y和z轴组成。
**实现方法:**
* **使用3D绘图库:**Matplotlib、Mayavi等3D绘图库提供三维坐标系创建和配置功能。
* **使用WebGL:**WebGL是一个JavaScript API,允许在浏览器中创建3D图形。
**代码示例:**
```python
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
# 数据
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
z = [3, 6, 9, 12, 15]
# 创建三维坐标系
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
# 绘制数据
ax.scatter(x, y, z)
plt.show()
```
**逻辑分析:**
* `projection='3d'`指定创建三维坐标系。
* `ax.scatter()`绘制三维散点图。
#### 4.2.2 三维数据的可视化呈现
三维数据可以以不同的方式可视化,包括散点图、曲面图和体积图。
**实现方法:**
* **散点图:**使用`ax.scatter()`函数绘制三维散点图。
* **曲面图:**使用`ax.plot_surface()`函数绘制三维曲面图。
* **体积图:**使用`ax.voxels()`函数绘制三维体积图。
**代码示例:**
```python
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
# 数据
x = np.linspace(-1, 1, 50)
y = np.linspace(-1, 1, 50)
X, Y = np.meshgrid(x, y)
Z = np.sqrt(X**2 + Y**2)
# 创建三维坐标系
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
# 绘制曲面图
ax.plot_surface(X, Y, Z, cmap='jet')
plt.show()
```
**逻辑分析:**
* `np.meshgrid()`创建网格数据。
* `np.sqrt()`计算曲面的z值。
* `ax.plot_surface()`绘制三维曲面图。
### 4.3 地理坐标图的应用
#### 4.3.1 地图数据的加载和处理
地理坐标图用于可视化地理数据,例如国家、城市和道路。
**实现方法:**
* **使用地理数据库:**GeoPandas、Shapely等地理数据库提供加载和处理地理数据的工具。
* **使用在线地图服务:**Google Maps、OpenStreetMap等在线地图服务提供地图数据和API。
**代码示例:**
```python
import geopandas as gpd
# 加载地图数据
world_map = gpd.read_file('world_map.shp')
# 处理地图数据
world_map['geometry'] = world_map['geometry'].simplify(tolerance=0.01)
```
**逻辑分析:**
* `gpd.read_file()`加载地图数据。
* `simplify()`简化几何形状以提高性能。
#### 4.3.2 地理信息的可视化展示
地理信息可以以不同的方式可视化,包括点图、线图和面图。
**实现方法:**
* **点图:**使用`ax.scatter()`函数绘制地理点图。
* **线图:**使用`ax.plot()`函数绘制地理线图。
* **面图:**使用`ax.fill()`函数绘制地理面图。
**代码示例:**
```python
import matplotlib.pyplot as plt
import geopandas as gpd
# 加载地图数据
world_map = gpd.read_file('world_map.shp')
# 创建坐标图
fig, ax = plt.subplots()
# 绘制面图
ax.fill(world_map['geometry'], facecolor='lightblue', edgecolor='black')
# 添加图例
plt.legend(['Land'])
plt.show()
```
**逻辑分析:**
* `ax.fill()`绘制地理面图。
* `facecolor`和`edgecolor`指定面和边的颜色。
* `plt.legend()`添加图例。
# 5.1 常见的坐标图绘制工具
在实践中,有许多流行的坐标图绘制工具可供选择,每种工具都有其独特的优势和适用场景。以下列出了几种最常见的坐标图绘制工具:
### 5.1.1 Python中的Matplotlib库
Matplotlib是一个功能强大的Python库,用于创建各种类型的静态、动画和交互式可视化。它提供了一系列广泛的绘图函数和对象,使您可以轻松地创建定制和专业的坐标图。
```python
import matplotlib.pyplot as plt
# 创建一个散点图
plt.scatter(x, y)
# 设置坐标轴标签
plt.xlabel('x-axis')
plt.ylabel('y-axis')
# 显示图表
plt.show()
```
**参数说明:**
* `x` 和 `y`:要绘制的数据序列。
* `xlabel` 和 `ylabel`:坐标轴标签。
**代码逻辑分析:**
此代码使用Matplotlib的`scatter()`函数创建一个散点图,其中`x`和`y`是数据序列。然后,它使用`xlabel`和`ylabel`设置坐标轴标签,最后使用`show()`函数显示图表。
### 5.1.2 JavaScript中的D3.js库
D3.js是一个强大的JavaScript库,用于创建动态、交互式和数据驱动的可视化。它提供了操作文档对象模型(DOM)的强大功能,使您可以创建复杂的坐标图,并响应用户交互进行更新。
```javascript
// 创建一个SVG元素
var svg = d3.select('body').append('svg');
// 添加一个散点图组
var scatterplot = svg.append('g')
.attr('class', 'scatterplot');
// 添加数据点
scatterplot.selectAll('circle')
.data(data)
.enter()
.append('circle')
.attr('cx', function(d) { return xScale(d.x); })
.attr('cy', function(d) { return yScale(d.y); })
.attr('r', 5);
```
**参数说明:**
* `data`:要可视化的数据。
* `xScale`和`yScale`:用于转换数据值的比例尺。
**代码逻辑分析:**
此代码使用D3.js创建了一个散点图。首先,它创建一个SVG元素并添加一个散点图组。然后,它使用`selectAll()`和`data()`函数将数据绑定到散点图组。最后,它使用`enter()`和`append()`函数添加数据点,并使用`attr()`函数设置数据点的属性,例如位置和半径。
### 5.1.3 R语言中的ggplot2包
ggplot2是R语言中用于创建统计图形的高级语法。它提供了一个一致且直观的接口,使您可以轻松地创建各种类型的坐标图,包括散点图、折线图、柱状图和饼图。
```r
library(ggplot2)
# 创建一个散点图
ggplot(data, aes(x, y)) +
geom_point() +
labs(title = 'Scatterplot',
x = 'x-axis',
y = 'y-axis')
```
**参数说明:**
* `data`:要可视化的数据。
* `aes()`:美学映射,指定如何将数据映射到坐标图的属性。
* `geom_point()`:几何对象,用于绘制数据点。
* `labs()`:标签,用于设置图表标题和坐标轴标签。
**代码逻辑分析:**
此代码使用ggplot2创建一个散点图。首先,它使用`ggplot()`函数创建一个ggplot对象,并使用`aes()`函数指定美学映射。然后,它使用`geom_point()`函数添加数据点,并使用`labs()`函数设置图表标题和坐标轴标签。
# 6. 坐标图的未来发展
### 6.1 人工智能与坐标图
人工智能(AI)正在改变各个行业,包括数据可视化。AI技术可以应用于坐标图领域,以增强其功能和自动化程度。
#### 6.1.1 自动化坐标图的生成
AI算法可以自动化坐标图的生成过程,从数据准备到图表布局和样式选择。这可以节省大量时间和精力,特别是对于需要处理大量数据的复杂图表。
#### 6.1.2 智能化的数据分析和可视化
AI可以帮助分析数据并确定最合适的可视化方式。它可以识别模式、趋势和异常值,并建议最佳的图表类型和配置。这可以确保图表有效地传达数据中的见解。
### 6.2 增强现实与坐标图
增强现实(AR)技术可以将坐标图与现实世界融合在一起,创造身临其境的体验。
#### 6.2.1 沉浸式坐标图的体验
AR可以创建交互式坐标图,用户可以与之进行交互并探索数据。例如,用户可以放大或缩小图表,旋转它以查看不同的角度,或通过触摸或手势控制数据点。
#### 6.2.2 坐标图与现实世界的交互
AR还可以将坐标图叠加在现实世界中,以便用户可以将其与物理对象进行比较。这在建筑、工程和规划等领域特别有用,用户可以可视化设计并将其与现有环境进行交互。
0
0
相关推荐
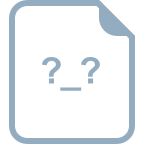
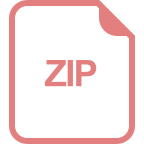






