【PHP与SQL Server数据库整合指南】:揭秘无缝连接的奥秘,提升开发效率
发布时间: 2024-07-24 07:31:59 阅读量: 39 订阅数: 42 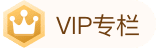
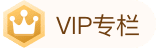
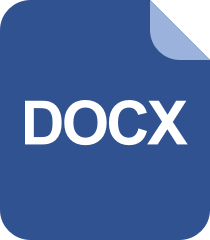
SQL Server 2005数据库备份与还原详细步骤指南
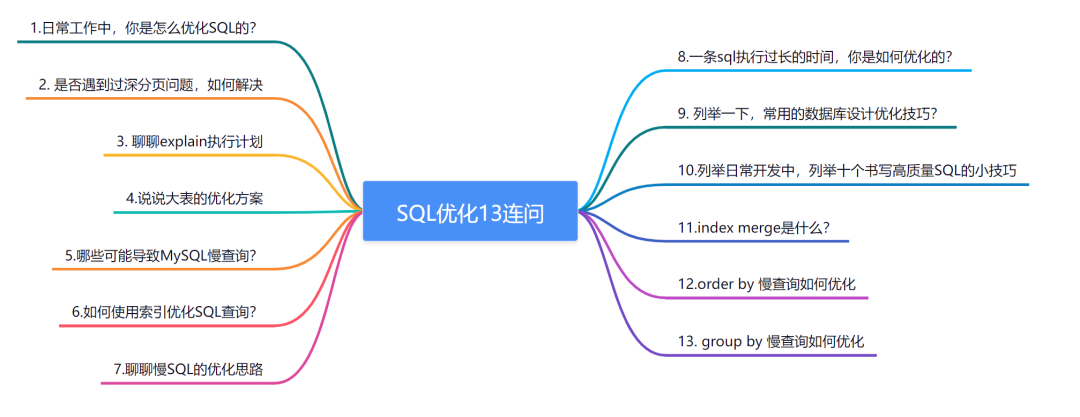
# 1. PHP与SQL Server数据库整合概述
PHP是一种广泛使用的脚本语言,它提供了与各种数据库系统集成的功能,其中包括SQL Server。通过将PHP与SQL Server数据库集成,开发人员可以构建强大的Web应用程序,这些应用程序可以存储、管理和操作数据。
本章将概述PHP与SQL Server数据库集成的基本概念,包括连接、操作和事务处理。它还将讨论一些与集成相关的最佳实践和安全注意事项。通过理解这些基础知识,开发人员可以创建高效且安全的PHP应用程序,这些应用程序充分利用了SQL Server数据库的功能。
# 2. PHP与SQL Server数据库连接
### 2.1 SQL Server数据库连接方式
#### 2.1.1 PDO连接
PDO(PHP Data Objects)是PHP中面向对象的数据访问接口,它提供了统一的API来连接和操作不同的数据库系统,包括SQL Server。使用PDO连接SQL Server的步骤如下:
```php
// 创建PDO对象
$dsn = 'sqlsrv:Server=localhost;Database=myDB';
$user = 'myUser';
$password = 'myPassword';
$options = [
PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION,
PDO::ATTR_PERSISTENT => true
];
$conn = new PDO($dsn, $user, $password, $options);
```
**参数说明:**
* `$dsn`:数据源名称,指定数据库类型、服务器地址和数据库名称。
* `$user`:数据库用户名。
* `$password`:数据库密码。
* `$options`:PDO连接选项,包括错误模式和持久连接。
#### 2.1.2 mysqli连接
mysqli是PHP中另一个流行的数据库连接扩展,它专门用于连接MySQL和MariaDB数据库。虽然它也可以用于连接SQL Server,但它不是推荐的方法。如果需要使用mysqli连接SQL Server,可以使用以下步骤:
```php
// 创建mysqli对象
$host = 'localhost';
$user = 'myUser';
$password = 'myPassword';
$database = 'myDB';
$conn = new mysqli($host, $user, $password, $database);
```
**参数说明:**
* `$host`:数据库服务器地址。
* `$user`:数据库用户名。
* `$password`:数据库密码。
* `$database`:数据库名称。
### 2.2 连接参数配置
连接SQL Server数据库时,需要配置以下参数:
#### 2.2.1 服务器地址
服务器地址指定数据库服务器的IP地址或主机名。例如:
```php
$dsn = 'sqlsrv:Server=localhost';
```
#### 2.2.2 数据库名称
数据库名称指定要连接的数据库。例如:
```php
$dsn = 'sqlsrv:Server=localhost;Database=myDB';
```
#### 2.2.3 用户名和密码
用户名和密码用于验证数据库连接。例如:
```php
$user = 'myUser';
$password = 'myPassword';
```
# 3. PHP与SQL Server数据库操作
### 3.1 数据查询
#### 3.1.1 查询语句的编写
PHP中使用SQL语句查询SQL Server数据库的数据,需要使用`mysqli_query()`函数。该函数的语法格式如下:
```php
mysqli_query(mysqli $link, string $query) : mysqli_result|false
```
其中:
- `$link`:代表与SQL Server数据库的连接句柄。
- `$query`:要执行的SQL查询语句。
**示例:**
```php
$query = "SELECT * FROM users";
$result = mysqli_query($link, $query);
```
#### 3.1.2 查询结果的获取
`mysqli_query()`函数执行查询语句后,会返回一个`mysqli_result`对象,该对象包含了查询结果。可以使用`mysqli_fetch_assoc()`函数获取查询结果中的每一行数据,并以关联数组的形式返回。
```php
while ($row = mysqli_fetch_assoc($result)) {
// 处理每一行数据
}
```
### 3.2 数据插入
#### 3.2.1 插入语句的编写
使用`mysqli_query()`函数插入数据到SQL Server数据库中,需要使用`INSERT INTO`语句。该语句的语法格式如下:
```sql
INSERT INTO table_name (column1, column2, ...) VALUES (value1, value2, ...)
```
其中:
- `table_name`:要插入数据的表名。
- `column1`, `column2`, ...:要插入数据的列名。
- `value1`, `value2`, ...:要插入数据的列值。
**示例:**
```php
$query = "INSERT INTO users (username, password, email) VALUES ('user1', 'password1', 'user1@example.com')";
$result = mysqli_query($link, $query);
```
#### 3.2.2 插入数据的类型转换
在插入数据时,需要考虑数据类型的转换。如果插入的数据类型与表中列的类型不一致,则需要使用`mysqli_real_escape_string()`函数对数据进行类型转换。
```php
$username = mysqli_real_escape_string($link, $username);
$password = mysqli_real_escape_string($link, $password);
$email = mysqli_real_escape_string($link, $email);
```
### 3.3 数据更新
#### 3.3.1 更新语句的编写
使用`mysqli_query()`函数更新SQL Server数据库中的数据,需要使用`UPDATE`语句。该语句的语法格式如下:
```sql
UPDATE table_name SET column1 = value1, column2 = value2, ... WHERE condition
```
其中:
- `table_name`:要更新数据的表名。
- `column1`, `column2`, ...:要更新数据的列名。
- `value1`, `value2`, ...:要更新数据的列值。
- `condition`:更新数据的条件。
**示例:**
```php
$query = "UPDATE users SET password = 'new_password' WHERE username = 'user1'";
$result = mysqli_query($link, $query);
```
#### 3.3.2 更新数据的条件判断
在更新数据时,可以使用`WHERE`子句指定更新数据的条件。条件可以是任何SQL表达式,例如:
```sql
WHERE id = 1
WHERE username = 'user1'
WHERE created_at > '2023-01-01'
```
### 3.4 数据删除
#### 3.4.1 删除语句的编写
使用`mysqli_query()`函数删除SQL Server数据库中的数据,需要使用`DELETE`语句。该语句的语法格式如下:
```sql
DELETE FROM table_name WHERE condition
```
其中:
- `table_name`:要删除数据的表名。
- `condition`:删除数据的条件。
**示例:**
```php
$query = "DELETE FROM users WHERE username = 'user1'";
$result = mysqli_query($link, $query);
```
#### 3.4.2 删除数据的条件判断
在删除数据时,可以使用`WHERE`子句指定删除数据的条件。条件可以是任何SQL表达式,例如:
```sql
WHERE id = 1
WHERE username = 'user1'
WHERE created_at < '2023-01-01'
```
# 4. PHP与SQL Server数据库事务处理
### 4.1 事务的概念和优势
事务是数据库操作中不可分割的一个逻辑单元,它保证了数据库操作要么全部成功,要么全部失败。事务的特性包括:
- **原子性(Atomicity):**事务中的所有操作要么全部执行成功,要么全部失败,不会出现部分成功的情况。
- **一致性(Consistency):**事务执行前后,数据库必须处于一致的状态,不会因为事务的执行而破坏数据库的完整性。
- **隔离性(Isolation):**事务在执行过程中不受其他事务的影响,并且其他事务也看不到当前事务未提交的修改。
- **持久性(Durability):**一旦事务提交,其修改将永久保存到数据库中,即使系统发生故障也不会丢失。
事务的优势在于:
- **确保数据完整性:**通过原子性和一致性特性,事务保证了数据库数据的准确性和可靠性。
- **提高并发性:**通过隔离性特性,事务允许多个用户同时操作数据库,避免了数据冲突和死锁。
- **简化错误处理:**如果事务中出现错误,可以回滚整个事务,避免了手动回退操作的复杂性。
### 4.2 事务的开始、提交和回滚
在 PHP 中,可以使用 `mysqli` 或 `PDO` 扩展来开启、提交和回滚事务。
**开启事务:**
```php
// 使用 mysqli 扩展
$mysqli->begin_transaction();
// 使用 PDO 扩展
$pdo->beginTransaction();
```
**提交事务:**
```php
// 使用 mysqli 扩展
$mysqli->commit();
// 使用 PDO 扩展
$pdo->commit();
```
**回滚事务:**
```php
// 使用 mysqli 扩展
$mysqli->rollback();
// 使用 PDO 扩展
$pdo->rollBack();
```
### 4.3 事务控制语句
除了使用 `begin_transaction()`、`commit()` 和 `rollback()` 函数之外,PHP 还提供了以下事务控制语句:
#### 4.3.1 BEGIN TRANSACTION
`BEGIN TRANSACTION` 语句显式地开启一个事务。它可以用于显式控制事务的开始和结束。
```php
// 开启一个事务
$mysqli->query("BEGIN TRANSACTION");
// 执行事务中的操作
// 提交事务
$mysqli->commit();
```
#### 4.3.2 COMMIT
`COMMIT` 语句提交当前事务,将所有未提交的修改永久保存到数据库中。
```php
// 提交事务
$mysqli->query("COMMIT");
```
#### 4.3.3 ROLLBACK
`ROLLBACK` 语句回滚当前事务,撤销所有未提交的修改。
```php
// 回滚事务
$mysqli->query("ROLLBACK");
```
**示例:使用事务控制语句**
```php
// 开启一个事务
$mysqli->query("BEGIN TRANSACTION");
// 执行事务中的操作
$mysqli->query("INSERT INTO users (name, email) VALUES ('John Doe', 'john.doe@example.com')");
$mysqli->query("INSERT INTO orders (user_id, product_id, quantity) VALUES (1, 1, 10)");
// 提交事务
$mysqli->query("COMMIT");
```
在上面的示例中,`BEGIN TRANSACTION` 语句显式地开启了一个事务,然后执行了两个 `INSERT` 语句。最后,`COMMIT` 语句提交了事务,将这两个插入操作永久保存到数据库中。
# 5. PHP与SQL Server数据库安全
### 5.1 SQL注入攻击原理
SQL注入攻击是一种常见的网络安全威胁,它利用应用程序中的漏洞向数据库服务器发送恶意SQL查询。攻击者通过在用户输入中注入恶意代码,从而控制数据库并窃取敏感数据或破坏数据库结构。
### 5.2 预处理语句的使用
预处理语句是一种防止SQL注入攻击的有效方法。它允许您在执行查询之前准备SQL语句,并使用占位符来表示用户输入。当执行查询时,数据库引擎将使用占位符替换用户输入,从而防止恶意代码被注入到查询中。
```php
$stmt = $conn->prepare("SELECT * FROM users WHERE username = ?");
$stmt->bind_param("s", $username);
$stmt->execute();
```
### 5.3 参数化查询
参数化查询与预处理语句类似,但它使用命名参数而不是占位符。这可以提高代码的可读性和可维护性。
```php
$stmt = $conn->prepare("SELECT * FROM users WHERE username = :username");
$stmt->bindParam(":username", $username);
$stmt->execute();
```
### 5.4 存储过程的使用
存储过程是预编译的SQL语句,存储在数据库服务器上。它们提供了一种安全的方法来执行复杂的操作,因为它们在执行前已经过编译和优化。
```php
$stmt = $conn->prepare("CALL GetUserData(?)");
$stmt->bind_param("s", $username);
$stmt->execute();
```
# 6. PHP与SQL Server数据库性能优化
### 6.1 索引的使用
索引是数据库中一种数据结构,用于快速查找数据。通过在表中创建索引,可以显著提高查询性能。
**创建索引**
```sql
CREATE INDEX index_name ON table_name (column_name);
```
例如,在 `customers` 表中创建 `last_name` 索引:
```sql
CREATE INDEX last_name_idx ON customers (last_name);
```
### 6.2 查询语句的优化
优化查询语句可以减少数据库服务器的处理时间。以下是一些优化技巧:
- **使用适当的连接类型**:`INNER JOIN`、`LEFT JOIN` 和 `RIGHT JOIN` 具有不同的性能特征。选择最适合特定查询的连接类型。
- **避免使用 `SELECT *`**:只选择需要的列,以减少数据传输量。
- **使用 `WHERE` 子句**:过滤不需要的数据,以减少查询时间。
- **使用 `LIMIT` 子句**:限制返回的结果数量,以提高性能。
### 6.3 数据库连接池的使用
数据库连接池是一种机制,用于管理数据库连接。它可以减少创建和销毁连接的开销,从而提高性能。
**使用 PHP 连接池**
```php
$pool = new \PDOPool('mysql:host=localhost;dbname=test', 'root', 'password');
$connection = $pool->getConnection();
```
### 6.4 缓存机制的应用
缓存机制可以将经常访问的数据存储在内存中,以减少数据库访问次数。这可以显著提高性能。
**使用 PHP 缓存**
```php
$cache = new \Doctrine\Common\Cache\ApcuCache();
$cache->save('key', 'value');
$value = $cache->fetch('key');
```
0
0
相关推荐






