图像特征提取与匹配:OpenCV摄像头图像处理的识别与分类之本
发布时间: 2024-08-07 07:00:04 阅读量: 13 订阅数: 16 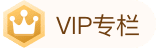
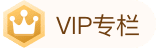
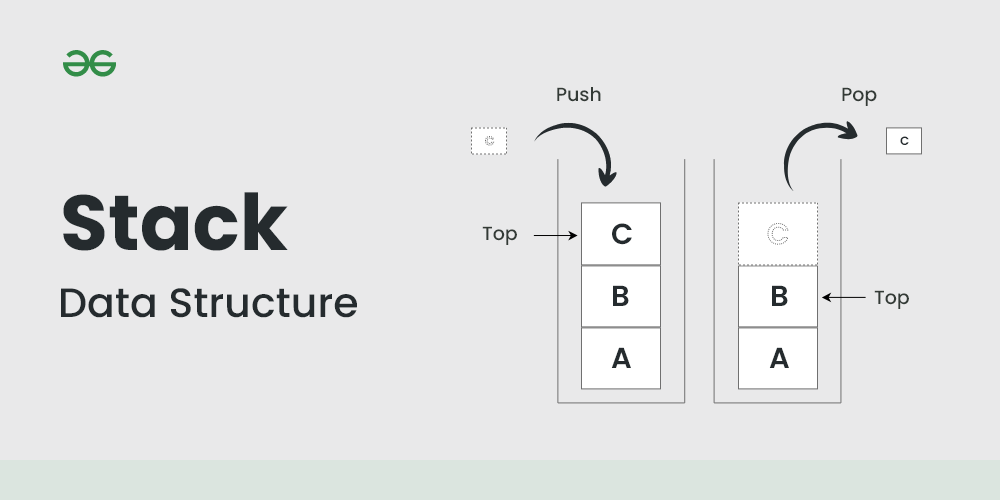
# 1. 图像特征提取的基础**
图像特征提取是计算机视觉领域中一项基本技术,它从图像中提取出能够描述其内容的关键信息。这些特征可以用来识别、分类和匹配图像。
图像特征可以分为基于像素、基于边缘和基于区域三大类。基于像素的特征提取方法直接操作图像的像素值,如灰度直方图和局部二进制模式。基于边缘的特征提取方法检测图像中的边缘,如Sobel算子和Canny算子。基于区域的特征提取方法将图像分割成不同的区域,如轮廓检测和连通域分析。
# 2. OpenCV图像特征提取技术**
**2.1 基于像素的特征提取**
基于像素的特征提取技术从图像的像素值中提取特征。这些技术对图像的亮度和颜色分布敏感,适用于图像分类和检索等任务。
**2.1.1 灰度直方图**
灰度直方图是图像中像素灰度值分布的统计表示。它将图像划分为多个灰度级,并计算每个灰度级中像素的数量。灰度直方图可以反映图像的整体亮度和对比度。
```python
import cv2
import numpy as np
# 读取图像
image = cv2.imread('image.jpg')
# 计算灰度直方图
hist = cv2.calcHist([image], [0], None, [256], [0, 256])
# 绘制灰度直方图
plt.plot(hist)
plt.show()
```
**2.1.2 局部二进制模式**
局部二进制模式(LBP)是一种基于像素邻域的特征提取技术。它将图像中的每个像素与周围的像素进行比较,并根据像素值的变化情况生成一个二进制模式。LBP可以捕捉图像的纹理和边缘信息。
```python
import cv2
# 读取图像
image = cv2.imread('image.jpg')
# 计算局部二进制模式
lbp = cv2.xfeatures2d.LBP_create(radius=1, points=8)
lbp_features = lbp.compute(image)
# 输出局部二进制模式特征
print(lbp_features)
```
**2.2 基于边缘的特征提取**
基于边缘的特征提取技术从图像中提取边缘和轮廓信息。这些技术对图像中的物体边界和形状敏感,适用于目标检测和分割等任务。
**2.2.1 Sobel算子**
Sobel算子是一种边缘检测算子,它使用两个卷积核来分别计算图像中水平和垂直方向的梯度。Sobel算子可以检测图像中的水平和垂直边缘。
```python
import cv2
# 读取图像
image = cv2.imread('image.jpg')
# 使用Sobel算子计算水平和垂直梯度
sobelx = cv2.Sobel(image, cv2.CV_64F, 1, 0, ksize=5)
sobely = cv2.Sobel(image, cv2.CV_64F, 0, 1, ksize=5)
# 计算梯度幅值
gradient_magnitude = np.sqrt(sobelx**2 + sobely**2)
# 输出梯度幅值
print(gradient_magnitude)
```
**2.2.2 Canny算子**
Canny算子是一种边缘检测算子,它使用多级边缘检测算法来检测图像中的边缘。Canny算子可以检测图像中的强边缘,并抑制噪声和弱边缘。
```python
import cv2
# 读取图像
image = cv2.imread('image.jpg')
# 使用Canny算子检测边缘
edges = cv2.Canny(image, 100, 200)
# 输出边缘图像
cv2.imshow('Edges', edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**2.3 基于区域的特征提取**
基于区域的特征提取技术从图像中提取区域和形状信息。这些技术对图像中的物体和区域的形状和大小敏感,适用于图像分割和对象识别等任务。
**2.3.1 轮廓检测**
轮廓检测是一种基于区域的特征提取技术,它从图像中提取物体和区域的边界。轮廓检测可以识别图像中的连通区域,并输出这些区域的边界信息。
```python
import cv2
# 读取图像
image = cv2.imread('image.jpg')
# 灰度化图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 二值化图像
thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)[1]
# 寻找轮廓
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 绘制轮廓
cv2.drawContours(image, contours, -1, (0, 255, 0), 2)
# 输出轮廓图像
cv2.imshow('Contours', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**2.3.2 连通域分析**
连通域分析是一种基于区域的特征提取技术,它从图像中识别和分析连通的区域。连通域分析可以将图像中的连通区域分组,并输出这些区域的属性信息。
```python
import cv2
import numpy as np
# 读取图像
image = cv2.imread('image.jpg')
# 灰度化图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 二值化图像
thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)[1]
# 进行连通域分析
num_labels, labels, stats, centroids = cv2.connectedComponentsWithStats(thresh)
# 输出连通域信息
for i in range(1, num_labels):
print(f"连通域 {i}:")
print(f"面积:{stats[i, cv2.CC_STAT_AREA]}")
print(f"质心:{centroids[i]}")
```
# 3. 图像特征匹配算法
图像特征匹配算法是图像处理中至关重要的一个环节,其目的是在两幅或多幅图像中找到相似的特征点,从而建立图像之间的对应关系。在图像识别、分类、跟踪等任务中,特征匹配算法起着至关重要的作用。
### 3.1 欧氏距离
欧氏距离是最常用的图像特征匹配算法之一,它计算两点之间的直线距离。对于两个特征点 $p_1 = (x_1
0
0
相关推荐
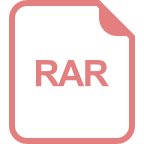
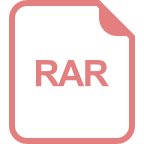
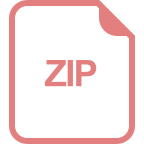





