【PHP图片上传数据库新手入门宝典】:从零开始,轻松掌握图片上传技巧
发布时间: 2024-08-01 23:21:53 阅读量: 10 订阅数: 12 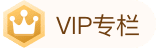
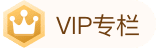

# 1. PHP图片上传概述**
PHP图片上传是一种将图片文件从客户端(如浏览器)传输到服务器(如Web服务器)的过程。它广泛应用于各种场景,如网站用户头像上传、电商平台商品图片上传等。
PHP图片上传涉及以下几个关键步骤:
* **客户端表单提交:**用户通过HTML表单选择图片文件并提交。
* **服务器端处理:**PHP脚本接收并处理上传的文件,包括验证、存储和处理。
* **数据库集成:**图片信息通常存储在数据库中,以便后续查询和检索。
# 2. PHP图片上传理论基础
### 2.1 文件上传的基本原理
#### 2.1.1 HTTP文件上传协议
HTTP文件上传协议是HTTP协议的一个扩展,它允许客户端将文件上传到服务器。该协议使用`multipart/form-data`编码类型,将文件数据与表单数据一起发送到服务器。
#### 2.1.2 PHP文件上传处理机制
PHP使用`$_FILES`超全局数组来处理文件上传。`$_FILES`数组包含有关上传文件的信息,包括文件名称、类型、大小和临时存储位置。
### 2.2 图片上传的常见格式和限制
#### 2.2.1 常用图片格式及其特点
| 格式 | 特点 |
|---|---|
| JPEG | 有损压缩,文件大小小,适合照片 |
| PNG | 无损压缩,文件大小较大,适合图形 |
| GIF | 支持动画,文件大小小,适合图标 |
| BMP | 无压缩,文件大小大,适合高分辨率图像 |
#### 2.2.2 图片上传大小、尺寸和类型限制
服务器通常会对图片上传设置大小、尺寸和类型限制。这些限制是为了防止恶意文件上传和服务器资源滥用。
```php
// 设置图片上传大小限制(单位:字节)
ini_set('upload_max_filesize', '2M');
// 设置图片上传类型限制
ini_set('upload_allowed_types', 'image/jpeg,image/png,image/gif');
```
# 3. PHP图片上传实践操作
### 3.1 使用HTML表单进行图片上传
#### 3.1.1 表单元素的设置
图片上传通常通过HTML表单来实现,表单中需要包含以下元素:
- `<form>` 元素:定义表单,指定提交方式和目标地址。
- `<input>` 元素:类型为 "file",用于选择要上传的图片。
- `<input>` 元素:类型为 "submit",用于提交表单。
```html
<form action="upload.php" method="post" enctype="multipart/form-data">
<input type="file" name="image">
<input type="submit" value="上传">
</form>
```
#### 3.1.2 文件上传字段的处理
在PHP中,通过 `$_FILES` 超全局变量来获取表单中上传的文件信息。`$_FILES` 是一个数组,其中每个元素代表一个上传的文件,键名是表单中文件字段的名称。
```php
if (isset($_FILES['image'])) {
$file = $_FILES['image'];
}
```
### 3.2 PHP处理图片上传
#### 3.2.1 文件上传数据的获取
`$_FILES` 数组中的每个元素包含以下信息:
- `name`:上传文件的原始名称。
- `type`:上传文件的MIME类型。
- `tmp_name`:上传文件在服务器上的临时存储路径。
- `error`:上传文件过程中发生的错误代码。
- `size`:上传文件的大小(以字节为单位)。
```php
$fileName = $file['name'];
$fileType = $file['type'];
$tmpName = $file['tmp_name'];
$fileSize = $file['size'];
```
#### 3.2.2 文件上传的验证和处理
在处理上传文件之前,需要进行以下验证:
- **文件类型验证:**确保上传的文件是允许的图片格式。
- **文件大小验证:**确保上传的文件不超过允许的最大尺寸。
- **错误代码检查:**确保文件上传过程中没有发生错误。
```php
// 允许的图片格式
$allowedTypes = ['image/jpeg', 'image/png', 'image/gif'];
// 验证文件类型
if (!in_array($fileType, $allowedTypes)) {
echo '不允许的文件类型';
exit;
}
// 验证文件大小
if ($fileSize > 1024000) {
echo '文件太大';
exit;
}
// 验证错误代码
if ($file['error'] !== UPLOAD_ERR_OK) {
echo '文件上传失败';
exit;
}
```
验证通过后,就可以将文件移动到永久存储位置。
```php
$targetPath = 'uploads/' . $fileName;
move_uploaded_file($tmpName, $targetPath);
```
# 4. PHP图片上传高级技巧
### 4.1 图片上传的安全防护
**4.1.1 文件类型验证**
为了防止恶意文件上传,需要对上传的文件类型进行验证。PHP提供了`getimagesize()`函数来获取图片文件的类型,并返回一个包含图片宽高和类型的数组。可以通过比较数组中的`mime`元素来判断图片类型是否合法。
```php
if (!getimagesize($_FILES['image']['tmp_name'])) {
echo 'Invalid image type';
exit;
}
```
**4.1.2 恶意代码检测**
恶意代码检测可以防止上传的文件中包含恶意代码,例如病毒或后门。PHP提供了`scandir()`函数来扫描目录中的文件,并返回一个包含文件名的数组。可以通过检查数组中的文件名是否包含可疑字符或扩展名来检测恶意代码。
```php
$files = scandir($_FILES['image']['tmp_name']);
foreach ($files as $file) {
if (preg_match('/\.php|\.exe|\.bat/', $file)) {
echo 'Malicious code detected';
exit;
}
}
```
### 4.2 图片上传的性能优化
**4.2.1 缓存机制的应用**
缓存机制可以减少图片上传时的网络请求次数,从而提高性能。PHP提供了`cache()`函数来缓存图片数据。可以通过将图片数据缓存到文件中或数据库中来实现。
```php
$cache_file = 'cache/' . $_FILES['image']['name'];
if (file_exists($cache_file)) {
echo 'Image already cached';
exit;
} else {
file_put_contents($cache_file, file_get_contents($_FILES['image']['tmp_name']));
echo 'Image cached successfully';
}
```
**4.2.2 异步上传技术的利用**
异步上传技术可以将图片上传过程与其他操作并行执行,从而提高性能。PHP提供了`curl_multi()`函数来实现异步上传。
```php
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, 'http://example.com/upload.php');
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $_FILES['image']);
curl_multi_add_handle($mh, $ch);
curl_multi_exec($mh, $running);
```
# 5. PHP图片上传与数据库集成
### 5.1 图片上传与数据库字段的关联
#### 5.1.1 数据库表结构设计
为了将图片上传与数据库集成,需要在数据库中设计一个表来存储图片相关信息。表结构如下:
```sql
CREATE TABLE images (
id INT NOT NULL AUTO_INCREMENT,
name VARCHAR(255) NOT NULL,
path VARCHAR(255) NOT NULL,
size INT NOT NULL,
type VARCHAR(255) NOT NULL,
created_at TIMESTAMP NOT NULL DEFAULT CURRENT_TIMESTAMP,
updated_at TIMESTAMP NOT NULL DEFAULT CURRENT_TIMESTAMP,
PRIMARY KEY (id)
);
```
其中:
* `id`:图片ID,自增主键
* `name`:图片名称
* `path`:图片路径
* `size`:图片大小(字节)
* `type`:图片类型(例如:image/jpeg、image/png)
* `created_at`:图片上传时间
* `updated_at`:图片更新时间
### 5.1.2 图片上传数据的入库处理
当图片上传成功后,需要将图片相关信息入库到数据库中。入库处理步骤如下:
1. 获取图片上传后的信息,包括图片名称、路径、大小、类型等。
2. 根据表结构,构造SQL插入语句。
3. 执行SQL插入语句,将图片信息插入到数据库中。
```php
$name = $_FILES['image']['name'];
$path = $_FILES['image']['tmp_name'];
$size = $_FILES['image']['size'];
$type = $_FILES['image']['type'];
$sql = "INSERT INTO images (name, path, size, type) VALUES (?, ?, ?, ?)";
$stmt = $conn->prepare($sql);
$stmt->bind_param('ssss', $name, $path, $size, $type);
$stmt->execute();
```
### 5.2 图片上传与数据库查询
#### 5.2.1 根据图片ID查询图片信息
根据图片ID查询图片信息,可以用于获取图片的详细信息。查询语句如下:
```sql
SELECT * FROM images WHERE id = ?;
```
```php
$id = 1;
$sql = "SELECT * FROM images WHERE id = ?";
$stmt = $conn->prepare($sql);
$stmt->bind_param('i', $id);
$stmt->execute();
$result = $stmt->get_result();
$image = $result->fetch_assoc();
```
#### 5.2.2 根据图片属性查询图片列表
根据图片属性查询图片列表,可以用于筛选和查找符合条件的图片。查询语句如下:
```sql
SELECT * FROM images WHERE name LIKE ? OR type LIKE ?;
```
```php
$name = '%avatar%';
$type = '%image/jpeg%';
$sql = "SELECT * FROM images WHERE name LIKE ? OR type LIKE ?";
$stmt = $conn->prepare($sql);
$stmt->bind_param('ss', $name, $type);
$stmt->execute();
$result = $stmt->get_result();
$images = $result->fetch_all(MYSQLI_ASSOC);
```
# 6. PHP图片上传的应用场景
### 6.1 网站用户头像上传
#### 6.1.1 头像上传的业务需求
* 允许用户上传个人头像,用于展示在网站个人主页、评论区等位置。
* 头像图片应符合一定的大小、尺寸和格式限制,以确保页面加载速度和视觉效果。
* 头像上传后应自动生成缩略图,用于在不同场景下展示。
#### 6.1.2 头像上传的实现方案
```php
// 设置表单元素
<form action="upload_avatar.php" method="post" enctype="multipart/form-data">
<input type="file" name="avatar">
<input type="submit" value="上传">
</form>
// PHP处理头像上传
if (isset($_FILES['avatar'])) {
// 获取上传文件信息
$file = $_FILES['avatar'];
// 验证文件类型
$allowedTypes = ['image/jpeg', 'image/png', 'image/gif'];
if (!in_array($file['type'], $allowedTypes)) {
echo '文件类型不正确';
exit;
}
// 验证文件大小
$maxSize = 1024 * 1024 * 2; // 2MB
if ($file['size'] > $maxSize) {
echo '文件大小超过限制';
exit;
}
// 保存头像
$fileName = uniqid() . '.' . pathinfo($file['name'], PATHINFO_EXTENSION);
$filePath = 'uploads/avatars/' . $fileName;
move_uploaded_file($file['tmp_name'], $filePath);
// 生成缩略图
$thumbPath = 'uploads/avatars/thumbs/' . $fileName;
createThumbnail($filePath, $thumbPath, 100, 100);
// 入库处理
// ...
}
```
0
0
相关推荐
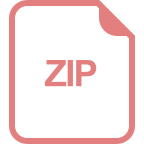
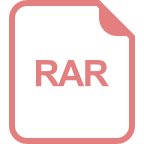






