单片机C语言程序设计实训:100个案例中的图形用户界面设计
发布时间: 2024-07-08 11:21:14 阅读量: 50 订阅数: 24 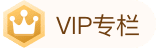
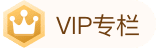
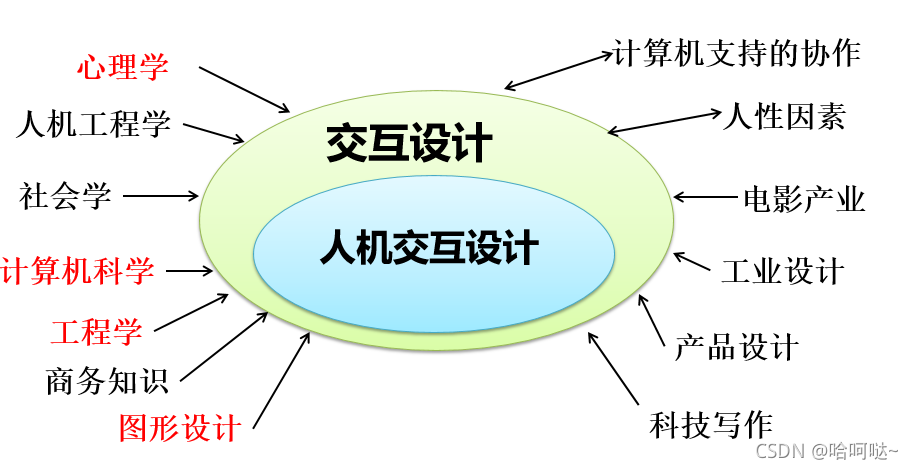
# 1. 单片机C语言程序设计基础
单片机C语言是一种专为单片机设计的C语言变体,它结合了C语言的强大功能和单片机的资源限制。本章将介绍单片机C语言程序设计的基础知识,包括:
- 单片机C语言的基本语法和数据类型
- 单片机寄存器和内存寻址方式
- 输入/输出操作和中断处理
- 编译器和调试工具的使用
# 2. 图形用户界面设计理论
### 2.1 图形用户界面的概念和组成
**概念**
图形用户界面(GUI)是一种人机交互方式,允许用户通过图形化元素(如图标、按钮、菜单等)与计算机进行交互。与传统的命令行界面相比,GUI更加直观、易用,降低了计算机的使用门槛。
**组成**
GUI通常由以下元素组成:
- **窗口:**包含其他GUI元素的矩形区域。
- **按钮:**执行特定操作的控件。
- **文本框:**用于输入和显示文本。
- **复选框:**用于选择或取消选择选项。
- **单选按钮:**用于在多个选项中选择一个。
- **菜单:**提供一系列选项的列表。
- **滚动条:**用于在内容过多时浏览内容。
### 2.2 常见的图形用户界面控件
GUI中常用的控件包括:
| 控件 | 用途 |
|---|---|
| 按钮 | 执行特定操作 |
| 文本框 | 输入和显示文本 |
| 复选框 | 选择或取消选择选项 |
| 单选按钮 | 在多个选项中选择一个 |
| 菜单 | 提供一系列选项的列表 |
| 滚动条 | 在内容过多时浏览内容 |
| 标签 | 显示文本信息 |
| 图片 | 显示图像 |
| 进度条 | 显示操作的进度 |
| 滑块 | 调整数值或选项 |
### 2.3 图形用户界面的事件处理
GUI中,用户交互会触发事件。事件处理是GUI编程的关键部分,它允许程序响应用户的操作。
**事件类型**
常见的事件类型包括:
- **鼠标事件:**单击、双击、移动、悬停等。
- **键盘事件:**按键按下、松开等。
- **窗口事件:**窗口创建、关闭、移动等。
**事件处理流程**
事件处理流程通常如下:
1. **事件发生:**用户执行操作,触发事件。
2. **事件传递:**事件传递到相关的控件或窗口。
3. **事件处理:**控件或窗口处理事件,执行相应的操作。
**代码示例**
以下代码示例展示了如何处理按钮单击事件:
```c
#include <stdio.h>
#include <stdlib.h>
#include <gtk/gtk.h>
GtkWidget *window;
GtkWidget *button;
// 按钮单击事件处理函数
static void button_clicked(GtkWidget *widget, gpointer data) {
printf("按钮被单击了!\n");
}
int main(int argc, char *argv[]) {
gtk_init(&argc, &argv);
// 创建窗口
window = gtk_window_new(GTK_WINDOW_TOPLEVEL);
gtk_window_set_title(GTK_WINDOW(window), "图形用户界面示例");
gtk_window_set_default_size(GTK_WINDOW(window), 350, 200);
// 创建按钮
button = gtk_button_new_with_label("单击我");
g_signal_connect(button, "clicked", G_CALLBACK(button_clicked), NULL);
// 将按钮添加到窗口
gtk_container_add(GTK_CONTAINER(window), button);
// 显示窗口
gtk_widget_show_all(window);
// 运行主事件循环
gtk_main();
return 0;
}
```
**逻辑分析**
代码中,`button_clicked`函数被注册为按钮单击事件的处理函数。当按钮被单击时,`button_clicked`函数会被调用,打印一条消息到控制台。
**参数说明**
- `widget`:触发事件的控件。
- `data`:传递给事件处理函数的附加数据。
# 3. 单片机C语言图形用户界面编程
### 3.1 单片机图形用户界面开发环境
**Keil uVision5**
Keil uVision5是一款集成开发环境(IDE),专为单片机开发设计。它提供了一个直观的界面,包含代码编辑器、调试器、仿真器和其他工具。Keil uVision5支持多种单片机架构,包括ARM Cortex-M系列。
**IAR Embedded Workbench**
IAR Embedded Workbench是另一个流行的单片机IDE。它提供了一个强大的代码编辑器、调试器和分析器。IAR Embedded Workbench支持多种单片机架构,包括ARM Cortex-M系列、8051和PIC。
**CodeSourcery CodeBench**
CodeSourcery CodeBench是一个开源的单片机IDE。它基于Eclipse平台,提供了一个可扩展的界面。CodeSourcery CodeBench支持多种单片机架构,包括ARM Cortex-M系列、PowerPC和MIPS。
### 3.2 图形用户界面控件的创建和使用
**创建控件**
要创建图形用户界面控件,可以使用IDE提供的控件库。控件库包含各种控件,如按钮、文本框、列表框和滚动条。要创建控件,只需将控件从控件库拖放到设计表面即可。
**设置控件属性**
创建控件后,可以设置其属性。属性决定控件的外观和行为。例如,可以设置按钮的文本、字体和颜色。还可以设置文本框的文本、字体和对齐方式。
### 3.3 图形用户界面事件的处理
**事件**
事件是用户与图形用户界面交互时发生的。事件可以是单击按钮、移动鼠标或按键盘。
**事件处理**
要处理事件,需要为每个事件编写事件处理程序。事件处理程序是当事件发生时调用的函数。例如,可以为按钮单击事件编写一个事件处理程序,该事件处理程序在用户单击按钮时执行。
**事件处理机制**
单片机图形用户界面使用消息队列来处理事件。当发生事件时,系统会将事件消息添加到消息队列中。事件处理程序从消息队列中获取事件消息并执行相应的操作。
**代码示例**
```c
// 按钮单击事件处理程序
void OnButtonClick(void)
{
// 执行按钮单击操作
}
```
**逻辑分析**
此代码定义了一个按钮单击事件处理程序。当用户单击按钮时,将调用此事件处理程序。事件处理程序执行按钮单击操作。
# 4. 单片机C语言图形用户界面案例
本章节将通过三个案例,分别介绍单片机C语言图形用户界面在数字时钟显示、音乐播放器和游戏中的应用。
### 4.1 数字时钟显示案例
#### 4.1.1 功能描述
该案例实现了一个简单的数字时钟,可以显示当前时间。
#### 4.1.2 实现步骤
1. 创建一个新的工程,并选择图形用户界面模板。
2. 在主窗口中添加一个文本控件,用于显示时间。
3. 在定时器中断服务程序中,更新文本控件中的时间。
#### 4.1.3 代码示例
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
// 定时器中断服务程序
void timer_isr() {
// 获取当前时间
time_t t = time(NULL);
struct tm
```
0
0
相关推荐
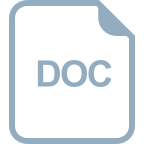
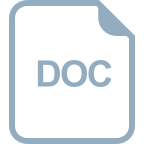