单片机C语言程序设计实训:100个案例中的物联网应用开发
发布时间: 2024-07-08 11:29:10 阅读量: 62 订阅数: 24 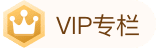
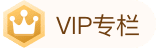
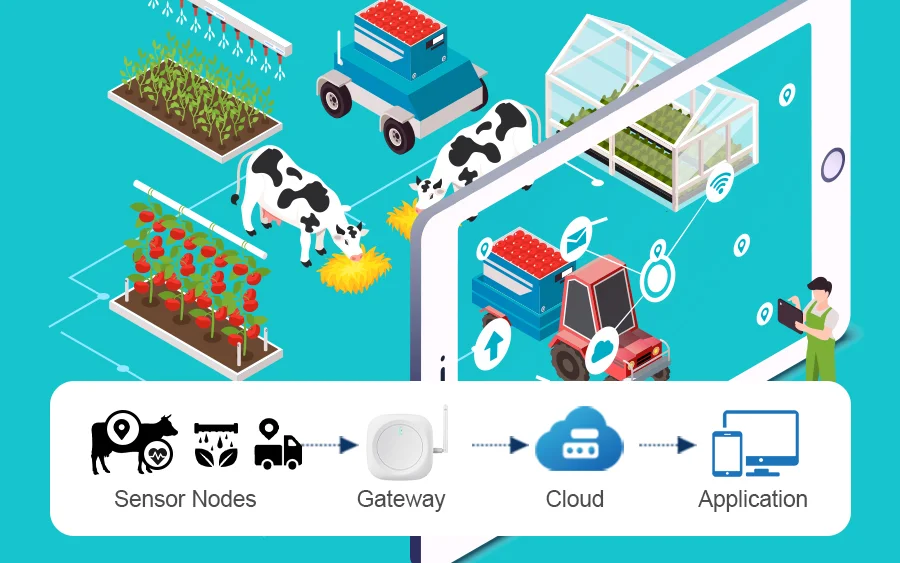
# 1. 单片机C语言基础与开发环境
### 1.1 单片机简介
单片机是一种集成了中央处理器、存储器和输入/输出接口等功能于一体的微型计算机。它具有体积小、功耗低、成本低等优点,广泛应用于各种电子设备中。
### 1.2 C语言简介
C语言是一种结构化的高级编程语言,具有简洁、高效、可移植性好等特点。它广泛用于单片机、嵌入式系统和操作系统等领域。
### 1.3 开发环境搭建
单片机C语言开发需要一个集成的开发环境(IDE),它提供了代码编辑、编译、调试等功能。常用的IDE包括Keil uVision、IAR Embedded Workbench和Code Composer Studio(CCS)。
# 2. 单片机C语言程序设计基础
### 2.1 数据类型和变量
#### 2.1.1 数据类型
单片机C语言中,数据类型用于指定变量可以存储的数据类型。主要的数据类型包括:
- 整型:用于存储整数,包括有符号整数(int)和无符号整数(unsigned int)。
- 浮点型:用于存储浮点数,包括单精度浮点数(float)和双精度浮点数(double)。
- 字符型:用于存储单个字符,使用单引号表示。
- 布尔型:用于存储逻辑值,只有 true 和 false 两个值。
- 指针型:用于存储其他变量的地址。
#### 2.1.2 变量
变量是用来存储数据的命名内存单元。在使用变量之前,需要先声明变量类型和变量名。变量声明的语法如下:
```c
数据类型 变量名;
```
例如:
```c
int num;
float temp;
```
### 2.2 运算符和表达式
#### 2.2.1 算术运算符
算术运算符用于对数值进行算术运算,包括:
- 加法(+):将两个值相加。
- 减法(-):将第二个值从第一个值中减去。
- 乘法(*):将两个值相乘。
- 除法(/):将第一个值除以第二个值。
- 取模(%):将第一个值除以第二个值,并返回余数。
#### 2.2.2 关系运算符
关系运算符用于比较两个值,并返回一个布尔值。关系运算符包括:
- 等于(==):检查两个值是否相等。
- 不等于(!=):检查两个值是否不相等。
- 大于(>):检查第一个值是否大于第二个值。
- 小于(<):检查第一个值是否小于第二个值。
- 大于等于(>=):检查第一个值是否大于或等于第二个值。
- 小于等于(<=):检查第一个值是否小于或等于第二个值。
### 2.3 控制语句
#### 2.3.1 if语句
if语句用于根据条件执行不同的代码块。语法如下:
```c
if (条件) {
// 条件为 true 时执行的代码块
} else {
// 条件为 false 时执行的代码块
}
```
例如:
```c
int num = 10;
if (num > 5) {
printf("num 大于 5");
} else {
printf("num 小于或等于 5");
}
```
#### 2.3.2 switch语句
switch语句用于根据变量的值执行不同的代码块。语法如下:
```c
switch (变量名) {
case 值1:
// 变量名等于值1 时执行的代码块
break;
case 值2:
// 变量名等于值2 时执行的代码块
break;
default:
// 变量名不等于任何值时执行的代码块
}
```
例如:
```c
int num = 1;
switch (num) {
case 1:
printf("num 等于 1");
break;
case 2:
printf("num 等于 2");
break;
default:
printf("num 不等于 1 或 2");
}
```
### 2.4 函数和数组
#### 2.4.1 函数
函数是代码的独立模块,可以重复使用。函数可以接收参数,并返回一个值。函数的语法如下:
```c
返回类型 函数名(参数列表) {
// 函数体
}
```
例如:
```c
int sum(int a, int b) {
return a + b;
}
```
#### 2.4.2 数组
数组是存储相同数据类型元素的集合。数组的元素使用索引访问。数组的语法如下:
```c
数据类型 数组名[大小];
```
例如:
```c
int numbers[5];
```
# 3. 单片机C语言外设接口编程
### 3.1 GPIO编程
#### 3.1.1 GPIO的概念
GPIO(General Purpose Input/Output)通用输入/输出端口,是单片机与外部设备通信的桥梁。GPIO端口可以被配置为输入或输出模式,从而实现数据的输入或输出功能。
#### 3.1.2 GPIO的配置和使用
**GPIO配置**
```c
// 设置GPIOA的第0位为输出模式
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
```
**参数说明:**
- `GPIO_Pin`:要配置的GPIO引脚,如`GPIO_Pin_0`表示GPIOA的第0位
- `GPIO_Mode`:配置的模式,如`GPIO_Mode_Out_PP`表示推挽输出模式
**GPIO使用**
```c
// 设置GPIOA的第0位输出高电平
GPIO_SetBits(GPIOA, GPIO_Pin_0);
// 设置GPIOA的第0位输出低电平
GPIO_ResetBits(GPIOA, GPIO_Pin_0);
```
### 3.2 定时器编程
#### 3.2.1 定时器的概念
定时器是单片机中用于产生定时脉冲或测量时间间隔的硬件模块。定时器可以被配置为不同的模式,如计数模式、比较模式等。
#### 3.2.2 定时器的配置和使用
**定时器配置**
```c
// 配置TIM2为向上计数模式,时钟源为APB1时钟,预分频系数为1000
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
TIM_TimeBaseStructure.TIM_Period = 1000;
TIM_TimeBaseStructure.TIM_Prescaler = 1000;
TIM_TimeBaseStructure.TIM_ClockDivision = TIM_CKD_DIV1;
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseInit(TIM2, &TIM_TimeBaseStruc
```
0
0
相关推荐
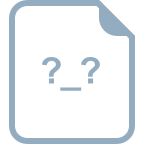
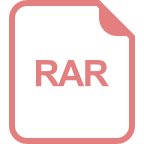
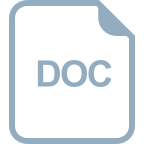
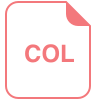
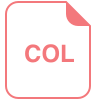
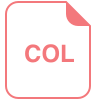
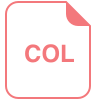
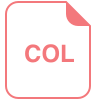
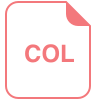